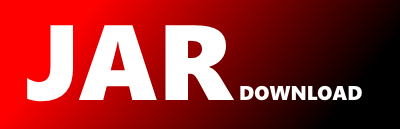
org.scalactic.anyvals.NegZInt.scala Maven / Gradle / Ivy
/* * Copyright 2001-2016 Artima, Inc. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package org.scalactic.anyvals import scala.collection.immutable.Range import scala.language.implicitConversions import scala.util.{Try, Success, Failure} import org.scalactic.{Validation, Pass, Fail} import org.scalactic.{Or, Good, Bad} /** * An
Float in Scala can lose precision.) This makes it convenient to * use aAnyVal
for non-positiveInt
s. * * * ** Because
* *NegZInt
is anAnyVal
it will usually be * as efficient as anInt
, being boxed only when anInt
* would have been boxed. ** The
* *NegZInt.apply
factory method is implemented in terms of a macro that * checks literals for validity at compile time. CallingNegZInt.apply
with * a literalInt
value will either produce a validNegZInt
instance * at run time or an error at compile time. Here's an example: ** scala> import anyvals._ * import anyvals._ * * scala> NegZInt(-42) * res0: org.scalactic.anyvals.NegZInt = NegZInt(-42) * * scala> NegZInt(1) * <console>:14: error: NegZInt.apply can only be invoked on a non-positive (i <= 0) literal, like NegZInt(-42). * NegZInt(1) * ^ ** **
* *NegZInt.apply
cannot be used if the value being passed is a variable (i.e., not a literal), because * the macro cannot determine the validity of variables at compile time (just literals). If you try to pass a variable * toNegZInt.apply
, you'll get a compiler error that suggests you use a different factor method, *NegZInt.from
, instead: ** scala> val x = 1 * x: Int = 1 * * scala> NegZInt(x) * <console>:15: error: NegZInt.apply can only be invoked on a non-positive integer literal, like NegZInt(-42). Please use NegZInt.from instead. * NegZInt(x) * ^ ** ** The
* *NegZInt.from
factory method will inspect the value at runtime and return anOption[NegZInt]
. If * the value is valid,NegZInt.from
will return aSome[NegZInt]
, else it will return aNone
. * Here's an example: ** scala> NegZInt.from(x) * res3: Option[org.scalactic.anyvals.NegZInt] = Some(NegZInt(1)) * * scala> val y = 0 * y: Int = 0 * * scala> NegZInt.from(y) * res4: Option[org.scalactic.anyvals.NegZInt] = None ** ** The
* *NegZInt.apply
factory method is marked implicit, so that you can pass literalInt
s * into methods that requireNegZInt
, and get the same compile-time checking you get when calling *NegZInt.apply
explicitly. Here's an example: ** scala> def invert(pos: NegZInt): Int = Int.MaxValue - pos * invert: (pos: org.scalactic.anyvals.NegZInt)Int * * scala> invert(1) * res0: Int = 2147483646 * * scala> invert(Int.MaxValue) * res1: Int = 0 * * scala> invert(0) * <console>:15: error: NegZInt.apply can only be invoked on a non-positive (i <= 0) integer literal, like NegZInt(-42). * invert(0) * ^ * * scala> invert(-1) * <console>:15: error: NegZInt.apply can only be invoked on a non-positive (i <= 0) integer literal, like NegZInt(-42). * invert(-1) * ^ * ** ** This example also demonstrates that the
NegZInt
companion object also defines implicit widening conversions * when either no loss of precision will occur or a similar conversion is provided in Scala. (For example, the implicit * conversion fromInt
toNegZInt
where anInt
or wider type is needed. An example is the subtraction in the body * of theinvert
method defined above,Int.MaxValue - pos
. AlthoughInt.MaxValue
is * anInt
, which has no-
method that takes aNegZInt
(the type ofpos
), * you can still subtractpos
, because theNegZInt
will be implicitly widened toInt
. * * * @param value TheInt
value underlying thisNegZInt
. */ final class NegZInt private (val value: Int) extends AnyVal { /** * A string representation of thisNegZInt
. */ override def toString: String = s"NegZInt(${value.toString()})" /** * Converts thisNegZInt
to aByte
. */ def toByte: Byte = value.toByte /** * Converts thisNegZInt
to aShort
. */ def toShort: Short = value.toShort /** * Converts thisNegZInt
to aChar
. */ def toChar: Char = value.toChar /** * Converts thisNegZInt
to anInt
. */ def toInt: Int = value.toInt /** * Converts thisNegZInt
to aLong
. */ def toLong: Long = value.toLong /** * Converts thisNegZInt
to aFloat
. */ def toFloat: Float = value.toFloat /** * Converts thisNegZInt
to aDouble
. */ def toDouble: Double = value.toDouble /** * Returns the bitwise negation of this value. * @example {{{ * ~5 == -6 * // in binary: ~00000101 == * // 11111010 * }}} */ def unary_~ : Int = ~value /** Returns this value, unmodified. */ def unary_+ : NegZInt = this /** Returns the negation of this value. */ def unary_- : Int = -value /** * Converts thisNegZInt
's value to a string then concatenates the given string. */ def +(x: String): String = s"${value.toString()}${x.toString()}" /** * Returns this value bit-shifted left by the specified number of bits, * filling in the new right bits with zeroes. * @example {{{ 6 << 3 == 48 // in binary: 0110 << 3 == 0110000 }}} */ def <<(x: Int): Int = value << x /** * Returns this value bit-shifted left by the specified number of bits, * filling in the new right bits with zeroes. * @example {{{ 6 << 3 == 48 // in binary: 0110 << 3 == 0110000 }}} */ def <<(x: Long): Int = value << x /** * Returns this value bit-shifted right by the specified number of bits, * filling the new left bits with zeroes. * @example {{{ 21 >>> 3 == 2 // in binary: 010101 >>> 3 == 010 }}} * @example {{{ * -21 >>> 3 == 536870909 * // in binary: 11111111 11111111 11111111 11101011 >>> 3 == * // 00011111 11111111 11111111 11111101 * }}} */ def >>>(x: Int): Int = value >>> x /** * Returns this value bit-shifted right by the specified number of bits, * filling the new left bits with zeroes. * @example {{{ 21 >>> 3 == 2 // in binary: 010101 >>> 3 == 010 }}} * @example {{{ * -21 >>> 3 == 536870909 * // in binary: 11111111 11111111 11111111 11101011 >>> 3 == * // 00011111 11111111 11111111 11111101 * }}} */ def >>>(x: Long): Int = value >>> x /** * Returns this value bit-shifted left by the specified number of bits, * filling in the right bits with the same value as the left-most bit of this. * The effect of this is to retain the sign of the value. * @example {{{ * -21 >> 3 == -3 * // in binary: 11111111 11111111 11111111 11101011 >> 3 == * // 11111111 11111111 11111111 11111101 * }}} */ def >>(x: Int): Int = value >> x /** * Returns this value bit-shifted left by the specified number of bits, * filling in the right bits with the same value as the left-most bit of this. * The effect of this is to retain the sign of the value. * @example {{{ * -21 >> 3 == -3 * // in binary: 11111111 11111111 11111111 11101011 >> 3 == * // 11111111 11111111 11111111 11111101 * }}} */ def >>(x: Long): Int = value >> x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Byte): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Short): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Char): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Int): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Long): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Float): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Double): Boolean = value < x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Byte): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Short): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Char): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Int): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Long): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Float): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Double): Boolean = value <= x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Byte): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Short): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Char): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Int): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Long): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Float): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Double): Boolean = value > x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Byte): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Short): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Char): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Int): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Long): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Float): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Double): Boolean = value >= x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Byte): Int = value | x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Short): Int = value | x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Char): Int = value | x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Int): Int = value | x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Long): Long = value | x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Byte): Int = value & x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Short): Int = value & x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Char): Int = value & x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Int): Int = value & x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Long): Long = value & x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Byte): Int = value ^ x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Short): Int = value ^ x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Char): Int = value ^ x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Int): Int = value ^ x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Long): Long = value ^ x /** Returns the sum of this value and `x`. */ def +(x: Byte): Int = value + x /** Returns the sum of this value and `x`. */ def +(x: Short): Int = value + x /** Returns the sum of this value and `x`. */ def +(x: Char): Int = value + x /** Returns the sum of this value and `x`. */ def +(x: Int): Int = value + x /** Returns the sum of this value and `x`. */ def +(x: Long): Long = value + x /** Returns the sum of this value and `x`. */ def +(x: Float): Float = value + x /** Returns the sum of this value and `x`. */ def +(x: Double): Double = value + x /** Returns the difference of this value and `x`. */ def -(x: Byte): Int = value - x /** Returns the difference of this value and `x`. */ def -(x: Short): Int = value - x /** Returns the difference of this value and `x`. */ def -(x: Char): Int = value - x /** Returns the difference of this value and `x`. */ def -(x: Int): Int = value - x /** Returns the difference of this value and `x`. */ def -(x: Long): Long = value - x /** Returns the difference of this value and `x`. */ def -(x: Float): Float = value - x /** Returns the difference of this value and `x`. */ def -(x: Double): Double = value - x /** Returns the product of this value and `x`. */ def *(x: Byte): Int = value * x /** Returns the product of this value and `x`. */ def *(x: Short): Int = value * x /** Returns the product of this value and `x`. */ def *(x: Char): Int = value * x /** Returns the product of this value and `x`. */ def *(x: Int): Int = value * x /** Returns the product of this value and `x`. */ def *(x: Long): Long = value * x /** Returns the product of this value and `x`. */ def *(x: Float): Float = value * x /** Returns the product of this value and `x`. */ def *(x: Double): Double = value * x /** Returns the quotient of this value and `x`. */ def /(x: Byte): Int = value / x /** Returns the quotient of this value and `x`. */ def /(x: Short): Int = value / x /** Returns the quotient of this value and `x`. */ def /(x: Char): Int = value / x /** Returns the quotient of this value and `x`. */ def /(x: Int): Int = value / x /** Returns the quotient of this value and `x`. */ def /(x: Long): Long = value / x /** Returns the quotient of this value and `x`. */ def /(x: Float): Float = value / x /** Returns the quotient of this value and `x`. */ def /(x: Double): Double = value / x /** Returns the remainder of the division of this value by `x`. */ def %(x: Byte): Int = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Short): Int = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Char): Int = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Int): Int = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Long): Long = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Float): Float = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Double): Double = value % x // Stuff from RichInt: /** * Returns a string representation of thisNegZInt
's underlyingInt
as an * unsigned integer in base 2. * ** The unsigned integer value is the argument plus 232 * if this
* * @return the string representation of the unsigned integer value * represented by thisNegZInt
's underlyingInt
is negative; otherwise it is equal to the * underlyingInt
. This value is converted to a string of ASCII digits * in binary (base 2) with no extra leading0
s. * If the unsigned magnitude is zero, it is represented by a * single zero character'0'
* ('\u0030'
); otherwise, the first character of * the representation of the unsigned magnitude will not be the * zero character. The characters'0'
* ('\u0030'
) and'1'
* ('\u0031'
) are used as binary digits. *NegZInt
's underlyingInt
in binary (base 2). */ def toBinaryString: String = java.lang.Integer.toBinaryString(value) /** * Returns a string representation of thisNegZInt
's underlyingInt
as an * unsigned integer in base 16. * ** The unsigned integer value is the argument plus 232 * if this
* *NegZInt
's underlyingInt
is negative; otherwise, it is equal to the * thisNegZInt
's underlyingInt
This value is converted to a string of ASCII digits * in hexadecimal (base 16) with no extra leading *0
s. If the unsigned magnitude is zero, it is * represented by a single zero character'0'
* ('\u0030'
); otherwise, the first character of * the representation of the unsigned magnitude will not be the * zero character. The following characters are used as * hexadecimal digits: *** * These are the characters0123456789abcdef
*'\u0030'
through *'\u0039'
and'\u0061'
through *'\u0066'
. If uppercase letters are * desired, thetoUpperCase
method may * be called on the result. * * @return the string representation of the unsigned integer value * represented by thisNegZInt
's underlyingInt
in hexadecimal (base 16). */ def toHexString: String = java.lang.Integer.toHexString(value) /** * Returns a string representation of thisNegZInt
's underlyingInt
as an * unsigned integer in base 8. * *The unsigned integer value is this
NegZInt
's underlyingInt
plus 232 * if the underlyingInt
is negative; otherwise, it is equal to the * underlyingInt
. This value is converted to a string of ASCII digits * in octal (base 8) with no extra leading0
s. * *If the unsigned magnitude is zero, it is represented by a * single zero character
'0'
* ('\u0030'
); otherwise, the first character of * the representation of the unsigned magnitude will not be the * zero character. The following characters are used as octal * digits: * *** * These are the characters01234567
*'\u0030'
through *'\u0037'
. * * @return the string representation of the unsigned integer value * represented by thisNegZInt
's underlyingInt
in octal (base 8). */ def toOctalString: String = java.lang.Integer.toOctalString(value) /** * Create aRange
from thisNegZInt
value * until the specifiedend
(exclusive) with step value 1. * * @param end The final bound of the range to make. * @return A [[scala.collection.immutable.Range]] from `this` up to but * not including `end`. */ def until(end: Int): Range = Range(value, end) /** * Create aRange
from thisNegZInt
value * until the specifiedend
(exclusive) with the specifiedstep
value. * * @param end The final bound of the range to make. * @param step The number to increase by for each step of the range. * @return A [[scala.collection.immutable.Range]] from `this` up to but * not including `end`. */ def until(end: Int, step: Int): Range = Range(value, end, step) /** * Create an inclusiveRange
from thisNegZInt
value * to the specifiedend
with step value 1. * * @param end The final bound of the range to make. * @return A [[scala.collection.immutable.Range]] from `'''this'''` up to * and including `end`. */ def to(end: Int): Range.Inclusive = Range.inclusive(value, end) /** * Create an inclusiveRange
from thisNegZInt
value * to the specifiedend
with the specifiedstep
value. * * @param end The final bound of the range to make. * @param step The number to increase by for each step of the range. * @return A [[scala.collection.immutable.Range]] from `'''this'''` up to * and including `end`. */ def to(end: Int, step: Int): Range.Inclusive = Range.inclusive(value, end, step) /** * Returnsthis
ifthis > that
orthat
otherwise. */ def max(that: NegZInt): NegZInt = if (math.max(value, that.value) == value) this else that /** * Returnsthis
ifthis < that
orthat
otherwise. */ def min(that: NegZInt): NegZInt = if (math.min(value, that.value) == value) this else that /** * Applies the passedInt => Int
function to the underlyingInt
* value, and if the result is positive, returns the result wrapped in aNegZInt
, * else throwsAssertionError
. * * A factory/assertion method that produces aPosInt
given a * validInt
value, or throwsAssertionError
, * if given an invalidInt
value. * * Note: you should use this method only when you are convinced that it will * always succeed, i.e., never throw an exception. It is good practice to * add a comment near the invocation of this method indicating ''why'' you think * it will always succeed to document your reasoning. If you are not sure an * `ensuringValid` call will always succeed, you should use one of the other * factory or validation methods provided on this object instead: `isValid`, * `tryingValid`, `passOrElse`, `goodOrElse`, or `rightOrElse`. * ** This method will inspect the result of applying the given function to this *
* *NegZInt
's underlyingInt
value and if the result * is non-positive, it will return aNegZInt
representing that value. * Otherwise, theInt
value returned by the given function is * not non-positive, so this method will throwAssertionError
. ** This method differs from a vanilla
* * @param f theassert
orensuring
* call in that you get something you didn't already have if the assertion * succeeds: a type that promises anInt
is non-positive. * With this method, you are asserting that you are convinced the result of * the computation represented by applying the given function to thisNegZInt
's * value will not overflow. Instead of overflowing silently likeInt
, this * method will signal an overflow with a loudAssertionError
. *Int => Int
function to apply to thisNegZInt
's * underlyingInt
value. * @return the result of applying thisNegZInt
's underlyingInt
value to * to the passed function, wrapped in aNegZInt
if it is non-positive (else throwsAssertionError
). * @throws AssertionError if the result of applying thisNegZInt
's underlyingInt
value to * to the passed function is not non-positive. */ def ensuringValid(f: Int => Int): NegZInt = { val candidateResult: Int = f(value) if (NegZIntMacro.isValid(candidateResult)) new NegZInt(candidateResult) else throw new AssertionError(s"${candidateResult.toString()}, the result of applying the passed function to ${value.toString()}, was not a valid NegZInt") } } /** * The companion object forNegZInt
that offers factory methods that * produceNegZInt
s, implicit widening conversions fromNegZInt
* to other numeric types, and maximum and minimum constant values forNegZInt
. */ object NegZInt { /** * The largest value representable as a non-positiveInt
, which isNegZInt(0)
. */ final val MaxValue: NegZInt = NegZInt.ensuringValid(0) /** * The smallest value representable as a non-positiveInt
, which isNegZInt(-2147483648)
. */ final val MinValue: NegZInt = NegZInt.ensuringValid(Int.MinValue) // Can't use the macro here /** * A factory method that produces anOption[NegZInt]
given an *Int
value. * ** This method will inspect the passed
* *Int
value and if * it is a non-positiveInt
, i.e., a non-positive integer value, * it will return aNegZInt
representing that value, * wrapped in aSome
. Otherwise, the passedInt
* value is not non-positive integer value, so this method will returnNone
. ** This factory method differs from the
* * @param value theapply
factory method * in thatapply
is implemented via a macro that inspects *Int
literals at compile time, whereasfrom
inspects *Int
values at run time. *Int
to inspect, and if non-positive, return * wrapped in aSome[NegZInt]
. * @return the specifiedInt
value wrapped * in aSome[NegZInt]
, if it is non-positive, elseNone
. */ def from(value: Int): Option[NegZInt] = if (NegZIntMacro.isValid(value)) Some(new NegZInt(value)) else None /** * A factory/assertion method that produces aNegZInt
given a * validInt
value, or throwsAssertionError
, * if given an invalidInt
value. * * Note: you should use this method only when you are convinced that it will * always succeed, i.e., never throw an exception. It is good practice to * add a comment near the invocation of this method indicating ''why'' you think * it will always succeed to document your reasoning. If you are not sure an * `ensuringValid` call will always succeed, you should use one of the other * factory or validation methods provided on this object instead: `isValid`, * `tryingValid`, `passOrElse`, `goodOrElse`, or `rightOrElse`. * ** This method will inspect the passed
* *Int
value and if * it is a non-positiveInt
, it will return aNegZInt
* representing that value. Otherwise, the passedInt
value is not non-positive, so this * method will throwAssertionError
. ** This factory method differs from the
* * @param value theapply
factory method * in thatapply
is implemented via a macro that inspects *Int
literals at compile time, whereas this method inspects *Int
values at run time. * It differs from a vanillaassert
orensuring
* call in that you get something you didn't already have if the assertion * succeeds: a type that promises anInt
is non-positive. *Int
to inspect, and if non-positive, return * wrapped in aNegZInt
. * @return the specifiedInt
value wrapped * in aNegZInt
, if it is non-positive, else throwsAssertionError
. * @throws AssertionError if the passed value is not non-positive */ def ensuringValid(value: Int): NegZInt = if (NegZIntMacro.isValid(value)) new NegZInt(value) else { throw new AssertionError(s"${value.toString()} was not a valid NegZInt") } /** * A factory/validation method that produces aNegZInt
, wrapped * in aSuccess
, given a validInt
value, or if the * givenInt
is invalid, anAssertionError
, wrapped * in aFailure
. * ** This method will inspect the passed
* *Int
value and if * it is a non-positiveInt
, it will return aNegZInt
* representing that value, wrapped in aSuccess
. * Otherwise, the passedInt
value is not non-positive, so this * method will return anAssertionError
, wrapped in aFailure
. ** This factory method differs from the
* * @param value theapply
factory method * in thatapply
is implemented via a macro that inspects *Int
literals at compile time, whereas this method inspects *Int
values at run time. *Int
to inspect, and if non-positive, return * wrapped in aSuccess(NegZInt)
. * @return the specifiedInt
value wrapped * in aSuccess(NegZInt)
, if it is non-positive, else aFailure(AssertionError)
. */ def tryingValid(value: Int): Try[NegZInt] = if (NegZIntMacro.isValid(value)) Success(new NegZInt(value)) else Failure(new AssertionError(s"${value.toString()} was not a valid NegZInt")) /** * A validation method that produces aPass
* given a validInt
value, or * an error value of typeE
produced by passing the * given invalidInt
value * to the given functionf
, wrapped in aFail
. * ** This method will inspect the passed
* *Int
value and if * it is a non-positiveInt
, it will return aPass
. * Otherwise, the passedInt
value is non-positive, so this * method will return a result of typeE
obtained by passing * the invalidInt
value to the given functionf
, * wrapped in a `Fail`. ** This factory method differs from the
* * @param value the `Int` to validate that it is non-positive. * @return a `Pass` if the specified `Int` value is non-positive, * else a `Fail` containing an error value produced by passing the * specified `Int` to the given function `f`. */ def passOrElse[E](value: Int)(f: Int => E): Validation[E] = if (NegZIntMacro.isValid(value)) Pass else Fail(f(value)) /** * A factory/validation method that produces aapply
factory method * in thatapply
is implemented via a macro that inspects *Int
literals at compile time, whereas this method inspects *Int
values at run time. *NegZInt
, wrapped * in aGood
, given a validInt
value, or if the * givenInt
is invalid, an error value of typeB
* produced by passing the given invalidInt
value * to the given functionf
, wrapped in aBad
. * ** This method will inspect the passed
* *Int
value and if * it is a non-positiveInt
, it will return aNegZInt
* representing that value, wrapped in aGood
. * Otherwise, the passedInt
value is not non-positive, so this * method will return a result of typeB
obtained by passing * the invalidInt
value to the given functionf
, * wrapped in a `Bad`. ** This factory method differs from the
* * @param value theapply
factory method * in thatapply
is implemented via a macro that inspects *Int
literals at compile time, whereas this method inspects *Int
values at run time. *Int
to inspect, and if non-positive, return * wrapped in aGood(NegZInt)
. * @return the specifiedInt
value wrapped * in aGood(NegZInt)
, if it is non-positive, else aBad(f(value))
. */ def goodOrElse[B](value: Int)(f: Int => B): NegZInt Or B = if (NegZIntMacro.isValid(value)) Good(NegZInt.ensuringValid(value)) else Bad(f(value)) /** * A factory/validation method that produces aNegZInt
, wrapped * in aRight
, given a validInt
value, or if the * givenInt
is invalid, an error value of typeL
* produced by passing the given invalidInt
value * to the given functionf
, wrapped in aLeft
. * ** This method will inspect the passed
* *Int
value and if * it is a non-positiveInt
, it will return aNegZInt
* representing that value, wrapped in aRight
. * Otherwise, the passedInt
value is not non-positive, so this * method will return a result of typeL
obtained by passing * the invalidInt
value to the given functionf
, * wrapped in a `Left`. ** This factory method differs from the
* * @param value theapply
factory method * in thatapply
is implemented via a macro that inspects *Int
literals at compile time, whereas this method inspects *Int
values at run time. *Int
to inspect, and if non-positive, return * wrapped in aRight(NegZInt)
. * @return the specifiedInt
value wrapped * in aRight(NegZInt)
, if it is non-positive, else aLeft(f(value))
. */ def rightOrElse[L](value: Int)(f: Int => L): Either[L, NegZInt] = if (NegZIntMacro.isValid(value)) Right(NegZInt.ensuringValid(value)) else Left(f(value)) /** * A predicate method that returns true if a given *Int
value is non-positive. * * @param value theInt
to inspect, and if non-positive, return true. * @return true if the specifiedInt
is non-positive, else false. */ def isValid(value: Int): Boolean = NegZIntMacro.isValid(value) /** * A factory method that produces aNegZInt
given a *Int
value and a defaultNegZInt
. * ** This method will inspect the passed
* *Int
value and if * it is a positiveInt
, i.e., a value greater * than 0.0, it will return aNegZInt
representing that value. * Otherwise, the passedInt
value is 0 or negative, so this * method will return the passeddefault
value. ** This factory method differs from the
* * @param value theapply
* factory method in thatapply
is implemented * via a macro that inspectsInt
literals at * compile time, whereasfrom
inspects *Int
values at run time. *Int
to inspect, and if positive, return. * @param default theNegZInt
to return if the passed *Int
value is not positive. * @return the specifiedInt
value wrapped in a *NegZInt
, if it is positive, else the *default
NegZInt
value. */ def fromOrElse(value: Int, default: => NegZInt): NegZInt = if (NegZIntMacro.isValid(value)) new NegZInt(value) else default import language.experimental.macros /** * A factory method, implemented via a macro, that produces aNegZInt
* if passed a validInt
literal, otherwise a compile time error. * ** The macro that implements this method will inspect the specified
* *Int
* expression at compile time. If * the expression is a positiveInt
literal, i.e., with a * value greater than 0, it will return aNegZInt
representing that value. * Otherwise, the passedInt
* expression is either a literal that is 0 or negative, or is not a literal, so * this method will give a compiler error. ** This factory method differs from the
* * @param value thefrom
factory method * in that this method is implemented via a macro that inspects *Int
literals at compile time, whereasfrom
inspects *Int
values at run time. *Int
literal expression to inspect at compile time, * and if positive, to return wrapped in aNegZInt
at run time. * @return the specified, validInt
literal value wrapped * in aNegZInt
. (If the specified expression is not a valid *Int
literal, the invocation of this method will not * compile.) */ inline implicit def apply(value: => Int): NegZInt = ${ NegZIntMacro('{value}) } /** * Implicit widening conversion fromNegZInt
toInt
. * * @param pos theNegZInt
to widen * @return theInt
value underlying the specifiedNegZInt
. */ implicit def widenToInt(pos: NegZInt): Int = pos.value /** * Implicit widening conversion fromNegZInt
toLong
. * * @param pos theNegZInt
to widen * @return theInt
value underlying the specifiedNegZInt
, * widened toLong
. */ implicit def widenToLong(pos: NegZInt): Long = pos.value /** * Implicit widening conversion fromNegZInt
toFloat
. * * @param pos theNegZInt
to widen * @return theInt
value underlying the specifiedNegZInt
, * widened toFloat
. */ implicit def widenToFloat(pos: NegZInt): Float = pos.value /** * Implicit widening conversion fromNegZInt
toDouble
. * * @param pos theNegZInt
to widen * @return theInt
value underlying the specifiedNegZInt
, * widened toDouble
. */ implicit def widenToDouble(pos: NegZInt): Double = pos.value /** * Implicit widening conversion fromNegZInt
toNegZLong
. * * @param pos theNegZInt
to widen * @return theInt
value underlying the specifiedNegZInt
, * widened toLong
and wrapped in aNegZLong
. */ implicit def widenToNegZLong(pos: NegZInt): NegZLong = NegZLong.ensuringValid(pos.value) /** * Implicit widening conversion fromNegZInt
toNegZFloat
. * * @param pos theNegZInt
to widen * @return theInt
value underlying the specifiedNegZInt
, * widened toFloat
and wrapped in aNegZFloat
. */ implicit def widenToNegZFloat(pos: NegZInt): NegZFloat = NegZFloat.ensuringValid(pos.value) /** * Implicit widening conversion fromNegZInt
toNegZDouble
. * * @param pos theNegZInt
to widen * @return theInt
value underlying the specifiedNegZInt
, * widened toDouble
and wrapped in aNegZDouble
. */ implicit def widenToNegZDouble(pos: NegZInt): NegZDouble = NegZDouble.ensuringValid(pos.value) /** * Implicit Ordering instance. */ implicit val ordering: Ordering[NegZInt] = new Ordering[NegZInt] { def compare(x: NegZInt, y: NegZInt): Int = x.toInt.compare(y) } }