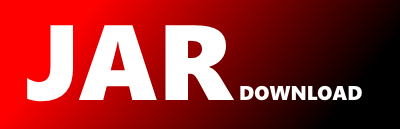
org.scalactic.anyvals.NonEmptyVector.scala Maven / Gradle / Ivy
/*
* Copyright 2001-2013 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalactic.anyvals
import scala.annotation.unchecked.{ uncheckedVariance => uV }
import scala.collection.GenIterable
import scala.collection.GenSeq
import scala.collection.GenTraversableOnce
import scala.collection.generic.CanBuildFrom
import scala.collection.mutable.Buffer
import scala.reflect.ClassTag
import scala.collection.immutable
import scala.collection.mutable.ArrayBuffer
import org.scalactic.Every
// Can't be a LinearSeq[T] because Builder would be able to create an empty one.
/**
* A non-empty list: an ordered, immutable, non-empty collection of elements with LinearSeq
performance characteristics.
*
*
* The purpose of NonEmptyVector
is to allow you to express in a type that a Vector
is non-empty, thereby eliminating the
* need for (and potential exception from) a run-time check for non-emptiness. For a non-empty sequence with IndexedSeq
* performance, see Every
.
*
*
* Constructing NonEmptyVector
s
*
*
* You can construct a NonEmptyVector
by passing one or more elements to the NonEmptyVector.apply
factory method:
*
*
*
* scala> NonEmptyVector(1, 2, 3)
* res0: org.scalactic.anyvals.NonEmptyVector[Int] = NonEmptyVector(1, 2, 3)
*
*
*
* Alternatively you can cons elements onto the End
singleton object, similar to making a Vector
starting with Nil
:
*
*
*
* scala> 1 :: 2 :: 3 :: Nil
* res0: Vector[Int] = Vector(1, 2, 3)
*
* scala> 1 :: 2 :: 3 :: End
* res1: org.scalactic.NonEmptyVector[Int] = NonEmptyVector(1, 2, 3)
*
*
*
* Note that although Nil
is a Vector[Nothing]
, End
is
* not a NonEmptyVector[Nothing]
, because no empty NonEmptyVector
exists. (A non-empty list is a series
* of connected links; if you have no links, you have no non-empty list.)
*
*
*
* scala> val nil: Vector[Nothing] = Nil
* nil: Vector[Nothing] = Vector()
*
* scala> val nada: NonEmptyVector[Nothing] = End
* <console>:16: error: type mismatch;
* found : org.scalactic.anyvals.End.type
* required: org.scalactic.anyvals.NonEmptyVector[Nothing]
* val nada: NonEmptyVector[Nothing] = End
* ^
*
*
* Working with NonEmptyVector
s
*
*
* NonEmptyVector
does not extend Scala's Seq
or Traversable
traits because these require that
* implementations may be empty. For example, if you invoke tail
on a Seq
that contains just one element,
* you'll get an empty Seq
:
*
*
*
* scala> Vector(1).tail
* res6: Vector[Int] = Vector()
*
*
*
* On the other hand, many useful methods exist on Seq
that when invoked on a non-empty Seq
are guaranteed
* to not result in an empty Seq
. For convenience, NonEmptyVector
defines a method corresponding to every such Seq
* method. Here are some examples:
*
*
*
* NonEmptyVector(1, 2, 3).map(_ + 1) // Result: NonEmptyVector(2, 3, 4)
* NonEmptyVector(1).map(_ + 1) // Result: NonEmptyVector(2)
* NonEmptyVector(1, 2, 3).containsSlice(NonEmptyVector(2, 3)) // Result: true
* NonEmptyVector(1, 2, 3).containsSlice(NonEmptyVector(3, 4)) // Result: false
* NonEmptyVector(-1, -2, 3, 4, 5).minBy(_.abs) // Result: -1
*
*
*
* NonEmptyVector
does not currently define any methods corresponding to Seq
methods that could result in
* an empty Seq
. However, an implicit converison from NonEmptyVector
to Vector
* is defined in the NonEmptyVector
companion object that will be applied if you attempt to call one of the missing methods. As a
* result, you can invoke filter
on an NonEmptyVector
, even though filter
could result
* in an empty sequence—but the result type will be Vector
instead of NonEmptyVector
:
*
*
*
* NonEmptyVector(1, 2, 3).filter(_ < 10) // Result: Vector(1, 2, 3)
* NonEmptyVector(1, 2, 3).filter(_ > 10) // Result: Vector()
*
*
*
*
* You can use NonEmptyVector
s in for
expressions. The result will be an NonEmptyVector
unless
* you use a filter (an if
clause). Because filters are desugared to invocations of filter
, the
* result type will switch to a Vector
at that point. Here are some examples:
*
*
*
* scala> import org.scalactic.anyvals._
* import org.scalactic.anyvals._
*
* scala> for (i <- NonEmptyVector(1, 2, 3)) yield i + 1
* res0: org.scalactic.anyvals.NonEmptyVector[Int] = NonEmptyVector(2, 3, 4)
*
* scala> for (i <- NonEmptyVector(1, 2, 3) if i < 10) yield i + 1
* res1: Vector[Int] = Vector(2, 3, 4)
*
* scala> for {
* | i <- NonEmptyVector(1, 2, 3)
* | j <- NonEmptyVector('a', 'b', 'c')
* | } yield (i, j)
* res3: org.scalactic.anyvals.NonEmptyVector[(Int, Char)] =
* NonEmptyVector((1,a), (1,b), (1,c), (2,a), (2,b), (2,c), (3,a), (3,b), (3,c))
*
* scala> for {
* | i <- NonEmptyVector(1, 2, 3) if i < 10
* | j <- NonEmptyVector('a', 'b', 'c')
* | } yield (i, j)
* res6: Vector[(Int, Char)] =
* Vector((1,a), (1,b), (1,c), (2,a), (2,b), (2,c), (3,a), (3,b), (3,c))
*
*
* @tparam T the type of elements contained in this NonEmptyVector
*/
final class NonEmptyVector[+T] private (val toVector: Vector[T]) extends AnyVal {
/**
* Returns a new NonEmptyVector
containing the elements of this NonEmptyVector
followed by the elements of the passed NonEmptyVector
.
* The element type of the resulting NonEmptyVector
is the most specific superclass encompassing the element types of this and the passed NonEmptyVector
.
*
* @tparam U the element type of the returned NonEmptyVector
* @param other the NonEmptyVector
to append
* @return a new NonEmptyVector
that contains all the elements of this NonEmptyVector
followed by all elements of other
.
*/
def ++[U >: T](other: NonEmptyVector[U]): NonEmptyVector[U] = new NonEmptyVector(toVector ++ other.toVector)
/**
* Returns a new NonEmptyVector
containing the elements of this NonEmptyVector
followed by the elements of the passed Every
.
* The element type of the resulting NonEmptyVector
is the most specific superclass encompassing the element types of this NonEmptyVector
and the passed Every
.
*
* @tparam U the element type of the returned NonEmptyVector
* @param other the Every
to append
* @return a new NonEmptyVector
that contains all the elements of this NonEmptyVector
followed by all elements of other
.
*/
def ++[U >: T](other: Every[U]): NonEmptyVector[U] = new NonEmptyVector(toVector ++ other.toVector)
// TODO: Have I added these extra ++, etc. methods to Every that take a NonEmptyVector?
/**
* Returns a new NonEmptyVector
containing the elements of this NonEmptyVector
followed by the elements of the passed GenTraversableOnce
.
* The element type of the resulting NonEmptyVector
is the most specific superclass encompassing the element types of this NonEmptyVector
* and the passed GenTraversableOnce
.
*
* @tparam U the element type of the returned NonEmptyVector
* @param other the GenTraversableOnce
to append
* @return a new NonEmptyVector
that contains all the elements of this NonEmptyVector
followed by all elements of other
.
*/
def ++[U >: T](other: GenTraversableOnce[U]): NonEmptyVector[U] =
if (other.isEmpty) this else new NonEmptyVector(toVector ++ other.toIterable)
/**
* Returns a new NonEmptyVector
with the given element prepended.
*
*
* Note that :-ending operators are right associative. A mnemonic for +:
vs. :+
is: the COLon goes on the COLlection side.
*
*
* @param element the element to prepend to this NonEmptyVector
* @return a new NonEmptyVector
consisting of element
followed by all elements of this NonEmptyVector
.
*/
final def +:[U >: T](element: U): NonEmptyVector[U] = new NonEmptyVector(element +: toVector)
/**
* Adds an element to the beginning of this NonEmptyVector
.
*
*
* Note that :-ending operators are right associative. A mnemonic for +:
vs. :+
is: the COLon goes on the COLlection side.
*
*
* @param element the element to prepend to this NonEmptyVector
* @return a NonEmptyVector
that contains element
as first element and that continues with this NonEmptyVector
.
*/
final def ::[U >: T](element: U): NonEmptyVector[U] = new NonEmptyVector(element +: toVector)
/**
* Returns a new NonEmptyVector
with the given element appended.
*
*
* Note a mnemonic for +:
vs. :+
is: the COLon goes on the COLlection side.
*
*
* @param element the element to append to this NonEmptyVector
* @return a new NonEmptyVector
consisting of all elements of this NonEmptyVector
followed by element
.
*/
def :+[U >: T](element: U): NonEmptyVector[U] = new NonEmptyVector(toVector :+ element)
/**
* Appends all elements of this NonEmptyVector
to a string builder. The written text will consist of a concatenation of the result of invoking toString
* on of every element of this NonEmptyVector
, without any separator string.
*
* @param sb the string builder to which elements will be appended
* @return the string builder, sb
, to which elements were appended.
*/
final def addString(sb: StringBuilder): StringBuilder = toVector.addString(sb)
/**
* Appends all elements of this NonEmptyVector
to a string builder using a separator string. The written text will consist of a concatenation of the
* result of invoking toString
* on of every element of this NonEmptyVector
, separated by the string sep
.
*
* @param sb the string builder to which elements will be appended
* @param sep the separator string
* @return the string builder, sb
, to which elements were appended.
*/
final def addString(sb: StringBuilder, sep: String): StringBuilder = toVector.addString(sb, sep)
/**
* Appends all elements of this NonEmptyVector
to a string builder using start, end, and separator strings. The written text will consist of a concatenation of
* the string start
; the result of invoking toString
on all elements of this NonEmptyVector
,
* separated by the string sep
; and the string end
*
* @param sb the string builder to which elements will be appended
* @param start the starting string
* @param sep the separator string
* @param start the ending string
* @return the string builder, sb
, to which elements were appended.
*/
final def addString(sb: StringBuilder, start: String, sep: String, end: String): StringBuilder = toVector.addString(sb, start, sep, end)
/**
* Selects an element by its index in the NonEmptyVector
.
*
* @return the element of this NonEmptyVector
at index idx
, where 0 indicates the first element.
*/
final def apply(idx: Int): T = toVector(idx)
/**
* Finds the first element of this NonEmptyVector
for which the given partial function is defined, if any, and applies the partial function to it.
*
* @param pf the partial function
* @return an Option
containing pf
applied to the first element for which it is defined, or None
if
* the partial function was not defined for any element.
*/
final def collectFirst[U](pf: PartialFunction[T, U]): Option[U] = toVector.collectFirst(pf)
/**
* Indicates whether this NonEmptyVector
contains a given value as an element.
*
* @param elem the element to look for
* @return true if this NonEmptyVector
has an element that is equal (as determined by ==)
to elem
, false otherwise.
*/
final def contains(elem: Any): Boolean = toVector.contains(elem)
/**
* Indicates whether this NonEmptyVector
contains a given GenSeq
as a slice.
*
* @param that the GenSeq
slice to look for
* @return true if this NonEmptyVector
contains a slice with the same elements as that
, otherwise false
.
*/
final def containsSlice[B](that: GenSeq[B]): Boolean = toVector.containsSlice(that)
/**
* Indicates whether this NonEmptyVector
contains a given Every
as a slice.
*
* @param that the Every
slice to look for
* @return true if this NonEmptyVector
contains a slice with the same elements as that
, otherwise false
.
*/
final def containsSlice[B](that: Every[B]): Boolean = toVector.containsSlice(that.toVector)
/**
* Indicates whether this NonEmptyVector
contains a given NonEmptyVector
as a slice.
*
* @param that the NonEmptyVector
slice to look for
* @return true if this NonEmptyVector
contains a slice with the same elements as that
, otherwise false
.
*/
final def containsSlice[B](that: NonEmptyVector[B]): Boolean = toVector.containsSlice(that.toVector)
/**
* Copies values of this NonEmptyVector
to an array. Fills the given array arr
with values of this NonEmptyVector
. Copying
* will stop once either the end of the current NonEmptyVector
is reached, or the end of the array is reached.
*
* @param arr the array to fill
*/
final def copyToArray[U >: T](arr: Array[U]): Unit = toVector.copyToArray(arr)
/**
* Copies values of this NonEmptyVector
to an array. Fills the given array arr
with values of this NonEmptyVector
, beginning at
* index start
. Copying will stop once either the end of the current NonEmptyVector
is reached, or the end of the array is reached.
*
* @param arr the array to fill
* @param start the starting index
*/
final def copyToArray[U >: T](arr: Array[U], start: Int): Unit = toVector.copyToArray(arr, start)
/**
* Copies values of this NonEmptyVector
to an array. Fills the given array arr
with at most len
elements of this NonEmptyVector
, beginning at
* index start
. Copying will stop once either the end of the current NonEmptyVector
is reached, the end of the array is reached, or
* len
elements have been copied.
*
* @param arr the array to fill
* @param start the starting index
* @param len the maximum number of elements to copy
*/
final def copyToArray[U >: T](arr: Array[U], start: Int, len: Int): Unit = toVector.copyToArray(arr, start, len)
/**
* Copies all elements of this NonEmptyVector
to a buffer.
*
* @param buf the buffer to which elements are copied
*/
final def copyToBuffer[U >: T](buf: Buffer[U]): Unit = toVector.copyToBuffer(buf)
/**
* Indicates whether every element of this NonEmptyVector
relates to the corresponding element of a given GenSeq
by satisfying a given predicate.
*
* @tparam B the type of the elements of that
* @param that the GenSeq
to compare for correspondence
* @param p the predicate, which relates elements from this NonEmptyVector
and the passed GenSeq
* @return true if this NonEmptyVector
and the passed GenSeq
have the same length and p(x, y)
is true
* for all corresponding elements x
of this NonEmptyVector
and y
of that, otherwise false
.
*/
final def corresponds[B](that: GenSeq[B])(p: (T, B) => Boolean): Boolean = toVector.corresponds(that)(p)
/**
* Indicates whether every element of this NonEmptyVector
relates to the corresponding element of a given Every
by satisfying a given predicate.
*
* @tparam B the type of the elements of that
* @param that the Every
to compare for correspondence
* @param p the predicate, which relates elements from this NonEmptyVector
and the passed Every
* @return true if this NonEmptyVector
and the passed Every
have the same length and p(x, y)
is true
* for all corresponding elements x
of this NonEmptyVector
and y
of that, otherwise false
.
*/
final def corresponds[B](that: Every[B])(p: (T, B) => Boolean): Boolean = toVector.corresponds(that.toVector)(p)
/**
* Indicates whether every element of this NonEmptyVector
relates to the corresponding element of a given NonEmptyVector
by satisfying a given predicate.
*
* @tparam B the type of the elements of that
* @param that the NonEmptyVector
to compare for correspondence
* @param p the predicate, which relates elements from this and the passed NonEmptyVector
* @return true if this and the passed NonEmptyVector
have the same length and p(x, y)
is true
* for all corresponding elements x
of this NonEmptyVector
and y
of that, otherwise false
.
*/
final def corresponds[B](that: NonEmptyVector[B])(p: (T, B) => Boolean): Boolean = toVector.corresponds(that.toVector)(p)
/**
* Counts the number of elements in this NonEmptyVector
that satisfy a predicate.
*
* @param p the predicate used to test elements.
* @return the number of elements satisfying the predicate p
.
*/
final def count(p: T => Boolean): Int = toVector.count(p)
/**
* Builds a new NonEmptyVector
from this NonEmptyVector
without any duplicate elements.
*
* @return A new NonEmptyVector
that contains the first occurrence of every element of this NonEmptyVector
.
*/
final def distinct: NonEmptyVector[T] = new NonEmptyVector(toVector.distinct)
/**
* Indicates whether this NonEmptyVector
ends with the given GenSeq
.
*
* @param that the sequence to test
* @return true
if this NonEmptyVector
has that
as a suffix, false
otherwise.
*/
final def endsWith[B](that: GenSeq[B]): Boolean = toVector.endsWith(that)
/**
* Indicates whether this NonEmptyVector
ends with the given Every
.
*
* @param that the Every
to test
* @return true
if this NonEmptyVector
has that
as a suffix, false
otherwise.
*/
final def endsWith[B](that: Every[B]): Boolean = toVector.endsWith(that.toVector)
// TODO: Search for that: Every in here and add a that: NonEmptyVector in Every.
/**
* Indicates whether this NonEmptyVector
ends with the given NonEmptyVector
.
*
* @param that the NonEmptyVector
to test
* @return true
if this NonEmptyVector
has that
as a suffix, false
otherwise.
*/
final def endsWith[B](that: NonEmptyVector[B]): Boolean = toVector.endsWith(that.toVector)
/*
override def equals(o: Any): Boolean =
o match {
case nonEmptyVector: NonEmptyVector[_] => toVector == nonEmptyVector.toVector
case _ => false
}
*/
/**
* Indicates whether a predicate holds for at least one of the elements of this NonEmptyVector
.
*
* @param the predicate used to test elements.
* @return true
if the given predicate p
holds for some of the elements of this NonEmptyVector
, otherwise false
.
*/
final def exists(p: T => Boolean): Boolean = toVector.exists(p)
/**
* Finds the first element of this NonEmptyVector
that satisfies the given predicate, if any.
*
* @param p the predicate used to test elements
* @return an Some
containing the first element in this NonEmptyVector
that satisfies p
, or None
if none exists.
*/
final def find(p: T => Boolean): Option[T] = toVector.find(p)
/**
* Builds a new NonEmptyVector
by applying a function to all elements of this NonEmptyVector
and using the elements of the resulting NonEmptyVector
s.
*
* @tparam U the element type of the returned NonEmptyVector
* @param f the function to apply to each element.
* @return a new NonEmptyVector
containing elements obtained by applying the given function f
to each element of this NonEmptyVector
and concatenating
* the elements of resulting NonEmptyVector
s.
*/
final def flatMap[U](f: T => NonEmptyVector[U]): NonEmptyVector[U] = {
val buf = new ArrayBuffer[U]
for (ele <- toVector)
buf ++= f(ele).toVector
new NonEmptyVector(buf.toVector)
}
/**
* Converts this NonEmptyVector
of NonEmptyVector
s into a NonEmptyVector
* formed by the elements of the nested NonEmptyVector
s.
*
*
* Note: You cannot use this flatten
method on a NonEmptyVector
that contains a GenTraversableOnce
s, because
* if all the nested GenTraversableOnce
s were empty, you'd end up with an empty NonEmptyVector
.
*
*
* @tparm B the type of the elements of each nested NonEmptyVector
* @return a new NonEmptyVector
resulting from concatenating all nested NonEmptyVector
s.
*/
final def flatten[B](implicit ev: T <:< NonEmptyVector[B]): NonEmptyVector[B] = flatMap(ev)
/**
* Folds the elements of this NonEmptyVector
using the specified associative binary operator.
*
*
* The order in which operations are performed on elements is unspecified and may be nondeterministic.
*
*
* @tparam U a type parameter for the binary operator, a supertype of T.
* @param z a neutral element for the fold operation; may be added to the result an arbitrary number of
* times, and must not change the result (e.g., Nil
for list concatenation,
* 0 for addition, or 1 for multiplication.)
* @param op a binary operator that must be associative
* @return the result of applying fold operator op
between all the elements and z
*/
final def fold[U >: T](z: U)(op: (U, U) => U): U = toVector.fold(z)(op)
/**
* Applies a binary operator to a start value and all elements of this NonEmptyVector
, going left to right.
*
* @tparam B the result type of the binary operator.
* @param z the start value.
* @param op the binary operator.
* @return the result of inserting op
between consecutive elements of this NonEmptyVector
, going left to right, with the start value,
* z
, on the left:
*
*
* op(...op(op(z, x_1), x_2), ..., x_n)
*
*
*
* where x1, ..., xn are the elements of this NonEmptyVector
.
*
*/
final def foldLeft[B](z: B)(op: (B, T) => B): B = toVector.foldLeft(z)(op)
/**
* Applies a binary operator to all elements of this NonEmptyVector
and a start value, going right to left.
*
* @tparam B the result of the binary operator
* @param z the start value
* @param op the binary operator
* @return the result of inserting op
between consecutive elements of this NonEmptyVector
, going right to left, with the start value,
* z
, on the right:
*
*
* op(x_1, op(x_2, ... op(x_n, z)...))
*
*
*
* where x1, ..., xn are the elements of this NonEmptyVector
.
*
*/
final def foldRight[B](z: B)(op: (T, B) => B): B = toVector.foldRight(z)(op)
/**
* Indicates whether a predicate holds for all elements of this NonEmptyVector
.
*
* @param p the predicate used to test elements.
* @return true
if the given predicate p
holds for all elements of this NonEmptyVector
, otherwise false
.
*/
final def forall(p: T => Boolean): Boolean = toVector.forall(p)
/**
* Applies a function f
to all elements of this NonEmptyVector
.
*
* @param f the function that is applied for its side-effect to every element. The result of function f
is discarded.
*/
final def foreach(f: T => Unit): Unit = toVector.foreach(f)
/**
* Partitions this NonEmptyVector
into a map of NonEmptyVector
s according to some discriminator function.
*
* @tparam K the type of keys returned by the discriminator function.
* @param f the discriminator function.
* @return A map from keys to NonEmptyVector
s such that the following invariant holds:
*
*
* (nonEmptyVector.toVector partition f)(k) = xs filter (x => f(x) == k)
*
*
*
* That is, every key k
is bound to a NonEmptyVector
of those elements x
for which f(x)
equals k
.
*
*/
final def groupBy[K](f: T => K): Map[K, NonEmptyVector[T]] = {
val mapKToVector = toVector.groupBy(f)
mapKToVector.mapValues { list => new NonEmptyVector(list) }.toMap
}
/**
* Partitions elements into fixed size NonEmptyVector
s.
*
* @param size the number of elements per group
* @return An iterator producing NonEmptyVector
s of size size
, except the last will be truncated if the elements don't divide evenly.
*/
final def grouped(size: Int): Iterator[NonEmptyVector[T]] = {
val itOfVector = toVector.grouped(size)
itOfVector.map { list => new NonEmptyVector(list) }
}
/**
* Returns true
to indicate this NonEmptyVector
has a definite size, since all NonEmptyVector
s are strict collections.
*/
final def hasDefiniteSize: Boolean = true
// override def hashCode: Int = toVector.hashCode
/**
* Selects the first element of this NonEmptyVector
.
*
* @return the first element of this NonEmptyVector
.
*/
final def head: T = toVector.head
// Methods like headOption I can't get rid of because of the implicit conversion to GenTraversable.
// Users can call any of the methods I've left out on a NonEmptyVector, and get whatever Vector would return
// for that method call. Eventually I'll probably implement them all to save the implicit conversion.
/**
* Selects the first element of this NonEmptyVector
and returns it wrapped in a Some
.
*
* @return the first element of this NonEmptyVector
, wrapped in a Some
.
*/
final def headOption: Option[T] = toVector.headOption
/**
* Finds index of first occurrence of some value in this NonEmptyVector
.
*
* @param elem the element value to search for.
* @return the index of the first element of this NonEmptyVector
that is equal (as determined by ==
) to elem
,
* or -1
, if none exists.
*/
final def indexOf[U >: T](elem: U): Int = toVector.indexOf(elem, 0)
/**
* Finds index of first occurrence of some value in this NonEmptyVector
after or at some start index.
*
* @param elem the element value to search for.
* @param from the start index
* @return the index >=
from
of the first element of this NonEmptyVector
that is equal (as determined by ==
) to elem
,
* or -1
, if none exists.
*/
final def indexOf[U >: T](elem: U, from: Int): Int = toVector.indexOf(elem, from)
/**
* Finds first index where this NonEmptyVector
contains a given GenSeq
as a slice.
*
* @param that the GenSeq
defining the slice to look for
* @return the first index at which the elements of this NonEmptyVector
starting at that index match the elements of
* GenSeq
that
, or -1
of no such subsequence exists.
*/
final def indexOfSlice[U >: T](that: GenSeq[U]): Int = toVector.indexOfSlice(that)
/**
* Finds first index after or at a start index where this NonEmptyVector
contains a given GenSeq
as a slice.
*
* @param that the GenSeq
defining the slice to look for
* @param from the start index
* @return the first index >=
from
at which the elements of this NonEmptyVector
starting at that index match the elements of
* GenSeq
that
, or -1
of no such subsequence exists.
*/
final def indexOfSlice[U >: T](that: GenSeq[U], from: Int): Int = toVector.indexOfSlice(that, from)
/**
* Finds first index where this NonEmptyVector
contains a given Every
as a slice.
*
* @param that the Every
defining the slice to look for
* @return the first index such that the elements of this NonEmptyVector
starting at this index match the elements of
* Every
that
, or -1
of no such subsequence exists.
*/
final def indexOfSlice[U >: T](that: Every[U]): Int = toVector.indexOfSlice(that.toVector)
/**
* Finds first index where this NonEmptyVector
contains a given NonEmptyVector
as a slice.
*
* @param that the NonEmptyVector
defining the slice to look for
* @return the first index such that the elements of this NonEmptyVector
starting at this index match the elements of
* NonEmptyVector
that
, or -1
of no such subsequence exists.
*/
final def indexOfSlice[U >: T](that: NonEmptyVector[U]): Int = toVector.indexOfSlice(that.toVector)
/**
* Finds first index after or at a start index where this NonEmptyVector
contains a given Every
as a slice.
*
* @param that the Every
defining the slice to look for
* @param from the start index
* @return the first index >=
from
such that the elements of this NonEmptyVector
starting at this index match the elements of
* Every
that
, or -1
of no such subsequence exists.
*/
final def indexOfSlice[U >: T](that: Every[U], from: Int): Int = toVector.indexOfSlice(that.toVector, from)
/**
* Finds first index after or at a start index where this NonEmptyVector
contains a given NonEmptyVector
as a slice.
*
* @param that the NonEmptyVector
defining the slice to look for
* @param from the start index
* @return the first index >=
from
such that the elements of this NonEmptyVector
starting at this index match the elements of
* NonEmptyVector
that
, or -1
of no such subsequence exists.
*/
final def indexOfSlice[U >: T](that: NonEmptyVector[U], from: Int): Int = toVector.indexOfSlice(that.toVector, from)
/**
* Finds index of the first element satisfying some predicate.
*
* @param p the predicate used to test elements.
* @return the index of the first element of this NonEmptyVector
that satisfies the predicate p
,
* or -1
, if none exists.
*/
final def indexWhere(p: T => Boolean): Int = toVector.indexWhere(p)
/**
* Finds index of the first element satisfying some predicate after or at some start index.
*
* @param p the predicate used to test elements.
* @param from the start index
* @return the index >=
from
of the first element of this NonEmptyVector
that satisfies the predicate p
,
* or -1
, if none exists.
*/
final def indexWhere(p: T => Boolean, from: Int): Int = toVector.indexWhere(p, from)
/**
* Produces the range of all indices of this NonEmptyVector
.
*
* @return a Range
value from 0
to one less than the length of this NonEmptyVector
.
*/
final def indices: Range = toVector.indices
/**
* Tests whether this NonEmptyVector
contains given index.
*
* @param idx the index to test
* @return true if this NonEmptyVector
contains an element at position idx
, false
otherwise.
*/
final def isDefinedAt(idx: Int): Boolean = toVector.isDefinedAt(idx)
/**
* Returns false
to indicate this NonEmptyVector
, like all NonEmptyVector
s, is non-empty.
*
* @return false
*/
final def isEmpty: Boolean = false
/**
* Returns true
to indicate this NonEmptyVector
, like all NonEmptyVector
s, can be traversed repeatedly.
*
* @return true
*/
final def isTraversableAgain: Boolean = true
/**
* Creates and returns a new iterator over all elements contained in this NonEmptyVector
.
*
* @return the new iterator
*/
final def iterator: Iterator[T] = toVector.iterator
/**
* Selects the last element of this NonEmptyVector
.
*
* @return the last element of this NonEmptyVector
.
*/
final def last: T = toVector.last
/**
* Finds the index of the last occurrence of some value in this NonEmptyVector
.
*
* @param elem the element value to search for.
* @return the index of the last element of this NonEmptyVector
that is equal (as determined by ==
) to elem
,
* or -1
, if none exists.
*/
final def lastIndexOf[U >: T](elem: U): Int = toVector.lastIndexOf(elem)
/**
* Finds the index of the last occurrence of some value in this NonEmptyVector
before or at a given end
index.
*
* @param elem the element value to search for.
* @param end the end index.
* @return the index >=
end
of the last element of this NonEmptyVector
that is equal (as determined by ==
)
* to elem
, or -1
, if none exists.
*/
final def lastIndexOf[U >: T](elem: U, end: Int): Int = toVector.lastIndexOf(elem, end)
/**
* Finds the last index where this NonEmptyVector
contains a given GenSeq
as a slice.
*
* @param that the GenSeq
defining the slice to look for
* @return the last index at which the elements of this NonEmptyVector
starting at that index match the elements of
* GenSeq
that
, or -1
of no such subsequence exists.
*/
final def lastIndexOfSlice[U >: T](that: GenSeq[U]): Int = toVector.lastIndexOfSlice(that)
/**
* Finds the last index before or at a given end index where this NonEmptyVector
contains a given GenSeq
as a slice.
*
* @param that the GenSeq
defining the slice to look for
* @param end the end index
* @return the last index >=
end
at which the elements of this NonEmptyVector
starting at that index match the elements of
* GenSeq
that
, or -1
of no such subsequence exists.
*/
final def lastIndexOfSlice[U >: T](that: GenSeq[U], end: Int): Int = toVector.lastIndexOfSlice(that, end)
/**
* Finds the last index where this NonEmptyVector
contains a given Every
as a slice.
*
* @param that the Every
defining the slice to look for
* @return the last index at which the elements of this NonEmptyVector
starting at that index match the elements of
* Every
that
, or -1
of no such subsequence exists.
*/
final def lastIndexOfSlice[U >: T](that: Every[U]): Int = toVector.lastIndexOfSlice(that.toVector)
/**
* Finds the last index where this NonEmptyVector
contains a given NonEmptyVector
as a slice.
*
* @param that the NonEmptyVector
defining the slice to look for
* @return the last index at which the elements of this NonEmptyVector
starting at that index match the elements of
* NonEmptyVector
that
, or -1
of no such subsequence exists.
*/
final def lastIndexOfSlice[U >: T](that: NonEmptyVector[U]): Int = toVector.lastIndexOfSlice(that.toVector)
/**
* Finds the last index before or at a given end index where this NonEmptyVector
contains a given Every
as a slice.
*
* @param that the Every
defining the slice to look for
* @param end the end index
* @return the last index >=
end
at which the elements of this NonEmptyVector
starting at that index match the elements of
* Every
that
, or -1
of no such subsequence exists.
*/
final def lastIndexOfSlice[U >: T](that: Every[U], end: Int): Int = toVector.lastIndexOfSlice(that.toVector, end)
/**
* Finds the last index before or at a given end index where this NonEmptyVector
contains a given NonEmptyVector
as a slice.
*
* @param that the NonEmptyVector
defining the slice to look for
* @param end the end index
* @return the last index >=
end
at which the elements of this NonEmptyVector
starting at that index match the elements of
* NonEmptyVector
that
, or -1
of no such subsequence exists.
*/
final def lastIndexOfSlice[U >: T](that: NonEmptyVector[U], end: Int): Int = toVector.lastIndexOfSlice(that.toVector, end)
/**
* Finds index of last element satisfying some predicate.
*
* @param p the predicate used to test elements.
* @return the index of the last element of this NonEmptyVector
that satisfies the predicate p
, or -1
, if none exists.
*/
final def lastIndexWhere(p: T => Boolean): Int = toVector.lastIndexWhere(p)
/**
* Finds index of last element satisfying some predicate before or at given end index.
*
* @param p the predicate used to test elements.
* @param end the end index
* @return the index >=
end
of the last element of this NonEmptyVector
that satisfies the predicate p
,
* or -1
, if none exists.
*/
final def lastIndexWhere(p: T => Boolean, end: Int): Int = toVector.lastIndexWhere(p, end)
/**
* Returns the last element of this NonEmptyVector
, wrapped in a Some
.
*
* @return the last element, wrapped in a Some
.
*/
final def lastOption: Option[T] = toVector.lastOption // Will always return a Some
/**
* The length of this NonEmptyVector
.
*
*
* Note: length
and size
yield the same result, which will be >
= 1.
*
*
* @return the number of elements in this NonEmptyVector
.
*/
final def length: Int = toVector.length
/**
* Compares the length of this NonEmptyVector
to a test value.
*
* @param len the test value that gets compared with the length.
* @return a value x
where
*
*
* x < 0 if this.length < len
* x == 0 if this.length == len
* x > 0 if this.length > len
*
*/
final def lengthCompare(len: Int): Int = toVector.lengthCompare(len)
/**
* Builds a new NonEmptyVector
by applying a function to all elements of this NonEmptyVector
.
*
* @tparam U the element type of the returned NonEmptyVector
.
* @param f the function to apply to each element.
* @return a new NonEmptyVector
resulting from applying the given function f
to each element of this NonEmptyVector
and collecting the results.
*/
final def map[U](f: T => U): NonEmptyVector[U] =
new NonEmptyVector(toVector.map(f))
/**
* Finds the largest element.
*
* @return the largest element of this NonEmptyVector
.
*/
final def max[U >: T](implicit cmp: Ordering[U]): T = toVector.max(cmp)
/**
* Finds the largest result after applying the given function to every element.
*
* @return the largest result of applying the given function to every element of this NonEmptyVector
.
*/
final def maxBy[U](f: T => U)(implicit cmp: Ordering[U]): T = toVector.maxBy(f)(cmp)
/**
* Finds the smallest element.
*
* @return the smallest element of this NonEmptyVector
.
*/
final def min[U >: T](implicit cmp: Ordering[U]): T = toVector.min(cmp)
/**
* Finds the smallest result after applying the given function to every element.
*
* @return the smallest result of applying the given function to every element of this NonEmptyVector
.
*/
final def minBy[U](f: T => U)(implicit cmp: Ordering[U]): T = toVector.minBy(f)(cmp)
/**
* Displays all elements of this NonEmptyVector
in a string.
*
* @return a string representation of this NonEmptyVector
. In the resulting string, the result of invoking toString
on all elements of this
* NonEmptyVector
follow each other without any separator string.
*/
final def mkString: String = toVector.mkString
/**
* Displays all elements of this NonEmptyVector
in a string using a separator string.
*
* @param sep the separator string
* @return a string representation of this NonEmptyVector
. In the resulting string, the result of invoking toString
on all elements of this
* NonEmptyVector
are separated by the string sep
.
*/
final def mkString(sep: String): String = toVector.mkString(sep)
/**
* Displays all elements of this NonEmptyVector
in a string using start, end, and separator strings.
*
* @param start the starting string.
* @param sep the separator string.
* @param end the ending string.
* @return a string representation of this NonEmptyVector
. The resulting string begins with the string start
and ends with the string
* end
. Inside, In the resulting string, the result of invoking toString
on all elements of this NonEmptyVector
are
* separated by the string sep
.
*/
final def mkString(start: String, sep: String, end: String): String = toVector.mkString(start, sep, end)
/**
* Returns true
to indicate this NonEmptyVector
, like all NonEmptyVector
s, is non-empty.
*
* @return true
*/
final def nonEmpty: Boolean = true
/**
* A copy of this NonEmptyVector
with an element value appended until a given target length is reached.
*
* @param len the target length
* @param elem he padding value
* @return a new NonEmptyVector
consisting of all elements of this NonEmptyVector
followed by the minimal number of occurrences
* of elem
so that the resulting NonEmptyVector
has a length of at least len
.
*/
final def padTo[U >: T](len: Int, elem: U): NonEmptyVector[U] =
new NonEmptyVector(toVector.padTo(len, elem))
/**
* Produces a new NonEmptyVector
where a slice of elements in this NonEmptyVector
is replaced by another NonEmptyVector
*
* @param from the index of the first replaced element
* @param that the NonEmptyVector
whose elements should replace a slice in this NonEmptyVector
* @param replaced the number of elements to drop in the original NonEmptyVector
*/
final def patch[U >: T](from: Int, that: NonEmptyVector[U], replaced: Int): NonEmptyVector[U] =
new NonEmptyVector(toVector.patch(from, that.toVector, replaced))
/**
* Iterates over distinct permutations.
*
*
* Here's an example:
*
*
*
* NonEmptyVector('a', 'b', 'b').permutations.toVector = Vector(NonEmptyVector(a, b, b), NonEmptyVector(b, a, b), NonEmptyVector(b, b, a))
*
*
* @return an iterator that traverses the distinct permutations of this NonEmptyVector
.
*/
final def permutations: Iterator[NonEmptyVector[T]] = {
val it = toVector.permutations
it map { list => new NonEmptyVector(list) }
}
/**
* Returns the length of the longest prefix whose elements all satisfy some predicate.
*
* @param p the predicate used to test elements.
* @return the length of the longest prefix of this NonEmptyVector
such that every element
* of the segment satisfies the predicate p
.
*/
final def prefixLength(p: T => Boolean): Int = toVector.prefixLength(p)
/**
* The result of multiplying all the elements of this NonEmptyVector
.
*
*
* This method can be invoked for any NonEmptyVector[T]
for which an implicit Numeric[T]
exists.
*
*
* @return the product of all elements
*/
final def product[U >: T](implicit num: Numeric[U]): U = toVector.product(num)
/**
* Reduces the elements of this NonEmptyVector
using the specified associative binary operator.
*
*
* The order in which operations are performed on elements is unspecified and may be nondeterministic.
*
*
* @tparam U a type parameter for the binary operator, a supertype of T.
* @param op a binary operator that must be associative.
* @return the result of applying reduce operator op
between all the elements of this NonEmptyVector
.
*/
final def reduce[U >: T](op: (U, U) => U): U = toVector.reduce(op)
/**
* Applies a binary operator to all elements of this NonEmptyVector
, going left to right.
*
* @tparam U the result type of the binary operator.
* @param op the binary operator.
* @return the result of inserting op
between consecutive elements of this NonEmptyVector
, going left to right:
*
*
* op(...op(op(x_1, x_2), x_3), ..., x_n)
*
*
*
* where x1, ..., xn are the elements of this NonEmptyVector
.
*
*/
final def reduceLeft[U >: T](op: (U, T) => U): U = toVector.reduceLeft(op)
/**
* Applies a binary operator to all elements of this NonEmptyVector
, going left to right, returning the result in a Some
.
*
* @tparam U the result type of the binary operator.
* @param op the binary operator.
* @return a Some
containing the result of reduceLeft(op)
*
*/
final def reduceLeftOption[U >: T](op: (U, T) => U): Option[U] = toVector.reduceLeftOption(op)
final def reduceOption[U >: T](op: (U, U) => U): Option[U] = toVector.reduceOption(op)
/**
* Applies a binary operator to all elements of this NonEmptyVector
, going right to left.
*
* @tparam U the result of the binary operator
* @param op the binary operator
* @return the result of inserting op
between consecutive elements of this NonEmptyVector
, going right to left:
*
*
* op(x_1, op(x_2, ... op(x_{n-1}, x_n)...))
*
*
*
* where x1, ..., xn are the elements of this NonEmptyVector
.
*
*/
final def reduceRight[U >: T](op: (T, U) => U): U = toVector.reduceRight(op)
/**
* Applies a binary operator to all elements of this NonEmptyVector
, going right to left, returning the result in a Some
.
*
* @tparam U the result of the binary operator
* @param op the binary operator
* @return a Some
containing the result of reduceRight(op)
*/
final def reduceRightOption[U >: T](op: (T, U) => U): Option[U] = toVector.reduceRightOption(op)
/**
* Returns new NonEmptyVector
with elements in reverse order.
*
* @return a new NonEmptyVector
with all elements of this NonEmptyVector
in reversed order.
*/
final def reverse: NonEmptyVector[T] =
new NonEmptyVector(toVector.reverse)
/**
* An iterator yielding elements in reverse order.
*
*
* Note: nonEmptyVector.reverseIterator
is the same as nonEmptyVector.reverse.iterator
, but might be more efficient.
*
*
* @return an iterator yielding the elements of this NonEmptyVector
in reversed order
*/
final def reverseIterator: Iterator[T] = toVector.reverseIterator
/**
* Builds a new NonEmptyVector
by applying a function to all elements of this NonEmptyVector
and collecting the results in reverse order.
*
*
* Note: nonEmptyVector.reverseMap(f)
is the same as nonEmptyVector.reverse.map(f)
, but might be more efficient.
*
*
* @tparam U the element type of the returned NonEmptyVector
.
* @param f the function to apply to each element.
* @return a new NonEmptyVector
resulting from applying the given function f
to each element of this NonEmptyVector
* and collecting the results in reverse order.
*/
final def reverseMap[U](f: T => U): NonEmptyVector[U] =
new NonEmptyVector(toVector.reverseMap(f))
/**
* Checks if the given GenIterable
contains the same elements in the same order as this NonEmptyVector
.
*
* @param that the GenIterable
with which to compare
* @return true
, if both this NonEmptyVector
and the given GenIterable
contain the same elements
* in the same order, false
otherwise.
*/
final def sameElements[U >: T](that: GenIterable[U]): Boolean = toVector.sameElements(that)
/**
* Checks if the given Every
contains the same elements in the same order as this NonEmptyVector
.
*
* @param that the Every
with which to compare
* @return true
, if both this and the given Every
contain the same elements
* in the same order, false
otherwise.
*/
final def sameElements[U >: T](that: Every[U]): Boolean = toVector.sameElements(that.toVector)
/**
* Checks if the given NonEmptyVector
contains the same elements in the same order as this NonEmptyVector
.
*
* @param that the NonEmptyVector
with which to compare
* @return true
, if both this and the given NonEmptyVector
contain the same elements
* in the same order, false
otherwise.
*/
final def sameElements[U >: T](that: NonEmptyVector[U]): Boolean = toVector.sameElements(that.toVector)
/**
* Computes a prefix scan of the elements of this NonEmptyVector
.
*
*
* Note: The neutral element z may be applied more than once.
*
*
*
* Here are some examples:
*
*
*
* NonEmptyVector(1, 2, 3).scan(0)(_ + _) == NonEmptyVector(0, 1, 3, 6)
* NonEmptyVector(1, 2, 3).scan("z")(_ + _.toString) == NonEmptyVector("z", "z1", "z12", "z123")
*
*
* @tparam U a type parameter for the binary operator, a supertype of T, and the type of the resulting NonEmptyVector
.
* @param z a neutral element for the scan operation; may be added to the result an arbitrary number of
* times, and must not change the result (e.g., Nil
for list concatenation,
* 0 for addition, or 1 for multiplication.)
* @param op a binary operator that must be associative
* @return a new NonEmptyVector
containing the prefix scan of the elements in this NonEmptyVector
*/
final def scan[U >: T](z: U)(op: (U, U) => U): NonEmptyVector[U] = new NonEmptyVector(toVector.scan(z)(op))
/**
* Produces a NonEmptyVector
containing cumulative results of applying the operator going left to right.
*
*
* Here are some examples:
*
*
*
* NonEmptyVector(1, 2, 3).scanLeft(0)(_ + _) == NonEmptyVector(0, 1, 3, 6)
* NonEmptyVector(1, 2, 3).scanLeft("z")(_ + _) == NonEmptyVector("z", "z1", "z12", "z123")
*
*
* @tparam B the result type of the binary operator and type of the resulting NonEmptyVector
* @param z the start value.
* @param op the binary operator.
* @return a new NonEmptyVector
containing the intermediate results of inserting op
between consecutive elements of this NonEmptyVector
,
* going left to right, with the start value, z
, on the left.
*/
final def scanLeft[B](z: B)(op: (B, T) => B): NonEmptyVector[B] = new NonEmptyVector(toVector.scanLeft(z)(op))
/**
* Produces a NonEmptyVector
containing cumulative results of applying the operator going right to left.
*
*
* Here are some examples:
*
*
*
* NonEmptyVector(1, 2, 3).scanRight(0)(_ + _) == NonEmptyVector(6, 5, 3, 0)
* NonEmptyVector(1, 2, 3).scanRight("z")(_ + _) == NonEmptyVector("123z", "23z", "3z", "z")
*
*
* @tparam B the result of the binary operator and type of the resulting NonEmptyVector
* @param z the start value
* @param op the binary operator
* @return a new NonEmptyVector
containing the intermediate results of inserting op
between consecutive elements of this NonEmptyVector
,
* going right to left, with the start value, z
, on the right.
*/
final def scanRight[B](z: B)(op: (T, B) => B): NonEmptyVector[B] = new NonEmptyVector(toVector.scanRight(z)(op))
/**
* Computes length of longest segment whose elements all satisfy some predicate.
*
* @param p the predicate used to test elements.
* @param from the index where the search starts.
* @param the length of the longest segment of this NonEmptyVector
starting from index from
such that every element of the
* segment satisfies the predicate p
.
*/
final def segmentLength(p: T => Boolean, from: Int): Int = toVector.segmentLength(p, from)
/**
* Groups elements in fixed size blocks by passing a “sliding window” over them (as opposed to partitioning them, as is done in grouped.)
*
* @param size the number of elements per group
* @return an iterator producing NonEmptyVector
s of size size
, except the last and the only element will be truncated
* if there are fewer elements than size
.
*/
final def sliding(size: Int): Iterator[NonEmptyVector[T]] = toVector.sliding(size).map(new NonEmptyVector(_))
/**
* Groups elements in fixed size blocks by passing a “sliding window” over them (as opposed to partitioning them, as is done in grouped.),
* moving the sliding window by a given step
each time.
*
* @param size the number of elements per group
* @param step the distance between the first elements of successive groups
* @return an iterator producing NonEmptyVector
s of size size
, except the last and the only element will be truncated
* if there are fewer elements than size
.
*/
final def sliding(size: Int, step: Int): Iterator[NonEmptyVector[T]] = toVector.sliding(size, step).map(new NonEmptyVector(_))
/**
* The size of this NonEmptyVector
.
*
*
* Note: length
and size
yield the same result, which will be >
= 1.
*
*
* @return the number of elements in this NonEmptyVector
.
*/
final def size: Int = toVector.size
/**
* Sorts this NonEmptyVector
according to the Ordering
of the result of applying the given function to every element.
*
* @tparam U the target type of the transformation f
, and the type where the Ordering
ord
is defined.
* @param f the transformation function mapping elements to some other domain U
.
* @param ord the ordering assumed on domain U
.
* @return a NonEmptyVector
consisting of the elements of this NonEmptyVector
sorted according to the Ordering
where
* x < y if ord.lt(f(x), f(y))
.
*/
final def sortBy[U](f: T => U)(implicit ord: Ordering[U]): NonEmptyVector[T] = new NonEmptyVector(toVector.sortBy(f))
/**
* Sorts this NonEmptyVector
according to a comparison function.
*
*
* The sort is stable. That is, elements that are equal (as determined by lt
) appear in the same order in the
* sorted NonEmptyVector
as in the original.
*
*
* @param the comparison function that tests whether its first argument precedes its second argument in the desired ordering.
* @return a NonEmptyVector
consisting of the elements of this NonEmptyVector
sorted according to the comparison function lt
.
*/
final def sortWith(lt: (T, T) => Boolean): NonEmptyVector[T] = new NonEmptyVector(toVector.sortWith(lt))
/**
* Sorts this NonEmptyVector
according to an Ordering
.
*
*
* The sort is stable. That is, elements that are equal (as determined by lt
) appear in the same order in the
* sorted NonEmptyVector
as in the original.
*
*
* @param ord the Ordering
to be used to compare elements.
* @param the comparison function that tests whether its first argument precedes its second argument in the desired ordering.
* @return a NonEmptyVector
consisting of the elements of this NonEmptyVector
sorted according to the comparison function lt
.
*/
final def sorted[U >: T](implicit ord: Ordering[U]): NonEmptyVector[U] = new NonEmptyVector(toVector.sorted(ord))
/**
* Indicates whether this NonEmptyVector
starts with the given GenSeq
.
*
* @param that the GenSeq
slice to look for in this NonEmptyVector
* @return true
if this NonEmptyVector
has that
as a prefix, false
otherwise.
*/
final def startsWith[B](that: GenSeq[B]): Boolean = toVector.startsWith(that)
/**
* Indicates whether this NonEmptyVector
starts with the given GenSeq
at the given index.
*
* @param that the GenSeq
slice to look for in this NonEmptyVector
* @param offset the index at which this NonEmptyVector
is searched.
* @return true
if this NonEmptyVector
has that
as a slice at the index offset
, false
otherwise.
*/
final def startsWith[B](that: GenSeq[B], offset: Int): Boolean = toVector.startsWith(that, offset)
/**
* Indicates whether this NonEmptyVector
starts with the given Every
.
*
* @param that the Every
to test
* @return true
if this collection has that
as a prefix, false
otherwise.
*/
final def startsWith[B](that: Every[B]): Boolean = toVector.startsWith(that.toVector)
/**
* Indicates whether this NonEmptyVector
starts with the given NonEmptyVector
.
*
* @param that the NonEmptyVector
to test
* @return true
if this collection has that
as a prefix, false
otherwise.
*/
final def startsWith[B](that: NonEmptyVector[B]): Boolean = toVector.startsWith(that.toVector)
/**
* Indicates whether this NonEmptyVector
starts with the given Every
at the given index.
*
* @param that the Every
slice to look for in this NonEmptyVector
* @param offset the index at which this NonEmptyVector
is searched.
* @return true
if this NonEmptyVector
has that
as a slice at the index offset
, false
otherwise.
*/
final def startsWith[B](that: Every[B], offset: Int): Boolean = toVector.startsWith(that.toVector, offset)
/**
* Indicates whether this NonEmptyVector
starts with the given NonEmptyVector
at the given index.
*
* @param that the NonEmptyVector
slice to look for in this NonEmptyVector
* @param offset the index at which this NonEmptyVector
is searched.
* @return true
if this NonEmptyVector
has that
as a slice at the index offset
, false
otherwise.
*/
final def startsWith[B](that: NonEmptyVector[B], offset: Int): Boolean = toVector.startsWith(that.toVector, offset)
/**
* Returns "NonEmptyVector"
, the prefix of this object's toString
representation.
*
* @return the string "NonEmptyVector"
*/
def stringPrefix: String = "NonEmptyVector"
/**
* The result of summing all the elements of this NonEmptyVector
.
*
*
* This method can be invoked for any NonEmptyVector[T]
for which an implicit Numeric[T]
exists.
*
*
* @return the sum of all elements
*/
final def sum[U >: T](implicit num: Numeric[U]): U = toVector.sum(num)
import scala.language.higherKinds
/**
* Converts this NonEmptyVector
into a collection of type Col
by copying all elements.
*
* @tparam Col the collection type to build.
* @return a new collection containing all elements of this NonEmptyVector
.
*/
final def to[Col[_]](factory: org.scalactic.ColCompatHelper.Factory[T, Col[T @ uV]]): Col[T @ uV] =
toVector.to(factory)
/**
* Converts this NonEmptyVector
to an array.
*
* @return an array containing all elements of this NonEmptyVector
. A ClassTag
must be available for the element type of this NonEmptyVector
.
*/
final def toArray[U >: T](implicit classTag: ClassTag[U]): Array[U] = toVector.toArray
/**
* Converts this NonEmptyVector
to a Vector
.
*
* @return a Vector
containing all elements of this NonEmptyVector
.
*/
final def toList: List[T] = toVector.toList
/**
* Converts this NonEmptyVector
to a mutable buffer.
*
* @return a buffer containing all elements of this NonEmptyVector
.
*/
final def toBuffer[U >: T]: Buffer[U] = toVector.toBuffer
/**
* Converts this NonEmptyVector
to an immutable IndexedSeq
.
*
* @return an immutable IndexedSeq
containing all elements of this NonEmptyVector
.
*/
final def toIndexedSeq: collection.immutable.IndexedSeq[T] = toVector.toVector
/**
* Converts this NonEmptyVector
to an iterable collection.
*
* @return an Iterable
containing all elements of this NonEmptyVector
.
*/
final def toIterable: Iterable[T] = toVector.toIterable
/**
* Returns an Iterator
over the elements in this NonEmptyVector
.
*
* @return an Iterator
containing all elements of this NonEmptyVector
.
*/
final def toIterator: Iterator[T] = toVector.toIterator
/**
* Converts this NonEmptyVector
to a list.
*
* @return a list containing all elements of this NonEmptyVector
.
*/
// final def toVector: Vector[T] = toVector
/**
* Converts this NonEmptyVector
to a map.
*
*
* This method is unavailable unless the elements are members of Tuple2
, each ((K, V))
becoming a key-value pair
* in the map. Duplicate keys will be overwritten by later keys.
*
*
* @return a map of type immutable.Map[K, V]
containing all key/value pairs of type (K, V)
of this NonEmptyVector
.
*/
final def toMap[K, V](implicit ev: T <:< (K, V)): Map[K, V] = toVector.toMap
/**
* Converts this NonEmptyVector
to an immutable IndexedSeq
.
*
* @return an immutable IndexedSeq
containing all elements of this NonEmptyVector
.
*/
final def toSeq: collection.immutable.Seq[T] = toVector
/**
* Converts this NonEmptyVector
to a set.
*
* @return a set containing all elements of this NonEmptyVector
.
*/
final def toSet[U >: T]: Set[U] = toVector.toSet
/**
* Converts this NonEmptyVector
to a stream.
*
* @return a stream containing all elements of this NonEmptyVector
.
*/
final def toStream: Stream[T] = toVector.toStream
/**
* Returns a string representation of this NonEmptyVector
.
*
* @return the string "NonEmptyVector"
followed by the result of invoking toString
on
* this NonEmptyVector
's elements, surrounded by parentheses.
*/
override def toString: String = "NonEmptyVector(" + toVector.mkString(", ") + ")"
final def transpose[U](implicit ev: T <:< NonEmptyVector[U]): NonEmptyVector[NonEmptyVector[U]] = {
val asVectors = toVector.map(ev)
val list = asVectors.transpose
new NonEmptyVector(list.map(new NonEmptyVector(_)))
}
/**
* Produces a new NonEmptyVector
that contains all elements of this NonEmptyVector
and also all elements of a given Every
.
*
*
* nonEmptyVectorX
union
everyY
is equivalent to nonEmptyVectorX
++
everyY
.
*
*
*
* Another way to express this is that nonEmptyVectorX
union
everyY
computes the order-presevring multi-set union
* of nonEmptyVectorX
and everyY
. This union
method is hence a counter-part of diff
and intersect
that
* also work on multi-sets.
*
*
* @param that the Every
to add.
* @return a new NonEmptyVector
that contains all elements of this NonEmptyVector
followed by all elements of that
Every
.
*/
final def union[U >: T](that: Every[U]): NonEmptyVector[U] = new NonEmptyVector(toVector union that.toVector)
/**
* Produces a new NonEmptyVector
that contains all elements of this NonEmptyVector
and also all elements of a given NonEmptyVector
.
*
*
* nonEmptyVectorX
union
nonEmptyVectorY
is equivalent to nonEmptyVectorX
++
nonEmptyVectorY
.
*
*
*
* Another way to express this is that nonEmptyVectorX
union
nonEmptyVectorY
computes the order-presevring multi-set union
* of nonEmptyVectorX
and nonEmptyVectorY
. This union
method is hence a counter-part of diff
and intersect
that
* also work on multi-sets.
*
*
* @param that the NonEmptyVector
to add.
* @return a new NonEmptyVector
that contains all elements of this NonEmptyVector
followed by all elements of that
.
*/
final def union[U >: T](that: NonEmptyVector[U]): NonEmptyVector[U] = new NonEmptyVector(toVector union that.toVector)
/**
* Produces a new NonEmptyVector
that contains all elements of this NonEmptyVector
and also all elements of a given GenSeq
.
*
*
* nonEmptyVectorX
union
ys
is equivalent to nonEmptyVectorX
++
ys
.
*
*
*
* Another way to express this is that nonEmptyVectorX
union
ys
computes the order-presevring multi-set union
* of nonEmptyVectorX
and ys
. This union
method is hence a counter-part of diff
and intersect
that
* also work on multi-sets.
*
*
* @param that the GenSeq
to add.
* @return a new NonEmptyVector
that contains all elements of this NonEmptyVector
followed by all elements of that
GenSeq
.
*/
final def union[U >: T](that: GenSeq[U])(implicit cbf: CanBuildFrom[Vector[T], U, Vector[U]]): NonEmptyVector[U] = new NonEmptyVector(toVector.union(that))
/**
* Converts this NonEmptyVector
of pairs into two NonEmptyVector
s of the first and second half of each pair.
*
* @tparam L the type of the first half of the element pairs
* @tparam R the type of the second half of the element pairs
* @param asPair an implicit conversion that asserts that the element type of this NonEmptyVector
is a pair.
* @return a pair of NonEmptyVector
s, containing the first and second half, respectively, of each element pair of this NonEmptyVector
.
*/
final def unzip[L, R](implicit asPair: T => (L, R)): (NonEmptyVector[L], NonEmptyVector[R]) = {
val unzipped = toVector.unzip
(new NonEmptyVector(unzipped._1), new NonEmptyVector(unzipped._2))
}
/**
* Converts this NonEmptyVector
of triples into three NonEmptyVector
s of the first, second, and and third element of each triple.
*
* @tparam L the type of the first member of the element triples
* @tparam R the type of the second member of the element triples
* @tparam R the type of the third member of the element triples
* @param asTriple an implicit conversion that asserts that the element type of this NonEmptyVector
is a triple.
* @return a triple of NonEmptyVector
s, containing the first, second, and third member, respectively, of each element triple of this NonEmptyVector
.
*/
final def unzip3[L, M, R](implicit asTriple: T => (L, M, R)): (NonEmptyVector[L], NonEmptyVector[M], NonEmptyVector[R]) = {
val unzipped = toVector.unzip3
(new NonEmptyVector(unzipped._1), new NonEmptyVector(unzipped._2), new NonEmptyVector(unzipped._3))
}
/**
* A copy of this NonEmptyVector
with one single replaced element.
*
* @param idx the position of the replacement
* @param elem the replacing element
* @throws IndexOutOfBoundsException if the passed index is greater than or equal to the length of this NonEmptyVector
* @return a copy of this NonEmptyVector
with the element at position idx
replaced by elem
.
*/
final def updated[U >: T](idx: Int, elem: U): NonEmptyVector[U] =
try new NonEmptyVector(toVector.updated(idx, elem))
catch { case _: UnsupportedOperationException => throw new IndexOutOfBoundsException(idx.toString) } // This is needed for 2.10 support. Can drop after.
// Because 2.11 throws IndexOutOfBoundsException.
/**
* Returns a NonEmptyVector
formed from this NonEmptyVector
and an iterable collection by combining corresponding
* elements in pairs. If one of the two collections is shorter than the other, placeholder elements will be used to extend the
* shorter collection to the length of the longer.
*
* @tparm O the type of the second half of the returned pairs
* @tparm U the type of the first half of the returned pairs
* @param other the Iterable
providing the second half of each result pair
* @param thisElem the element to be used to fill up the result if this NonEmptyVector
is shorter than that
Iterable
.
* @param thatElem the element to be used to fill up the result if that
Iterable
is shorter than this NonEmptyVector
.
* @return a new NonEmptyVector
containing pairs consisting of corresponding elements of this NonEmptyVector
and that
. The
* length of the returned collection is the maximum of the lengths of this NonEmptyVector
and that
. If this NonEmptyVector
* is shorter than that
, thisElem
values are used to pad the result. If that
is shorter than this
* NonEmptyVector
, thatElem
values are used to pad the result.
*/
final def zipAll[O, U >: T](other: collection.Iterable[O], thisElem: U, otherElem: O): NonEmptyVector[(U, O)] =
new NonEmptyVector(toVector.zipAll(other, thisElem, otherElem))
/**
* Zips this NonEmptyVector
with its indices.
*
* @return A new NonEmptyVector
containing pairs consisting of all elements of this NonEmptyVector
paired with their index. Indices start at 0.
*/
final def zipWithIndex: NonEmptyVector[(T, Int)] = new NonEmptyVector(toVector.zipWithIndex)
}
/**
* Companion object for class NonEmptyVector
.
*/
object NonEmptyVector {
/**
* Constructs a new NonEmptyVector
given at least one element.
*
* @tparam T the type of the element contained in the new NonEmptyVector
* @param firstElement the first element (with index 0) contained in this NonEmptyVector
* @param otherElements a varargs of zero or more other elements (with index 1, 2, 3, ...) contained in this NonEmptyVector
*/
def apply[T](firstElement: T, otherElements: T*): NonEmptyVector[T] = new NonEmptyVector(Vector(firstElement) ++ otherElements.toVector)
/**
* Variable argument extractor for NonEmptyVector
s.
*
* @param nonEmptyVector: the NonEmptyVector
containing the elements to extract
* @return an Seq
containing this NonEmptyVector
s elements, wrapped in a Some
*/
def unapplySeq[T](nonEmptyVector: NonEmptyVector[T]): Option[Seq[T]] = Some(nonEmptyVector.toVector)
/*
// TODO: Figure out how to get case NonEmptyVector() to not compile
def unapplySeq[T](nonEmptyVector: NonEmptyVector[T]): Option[(T, Seq[T])] = Some(nonEmptyVector.head, nonEmptyVector.tail)
*/
/**
* Optionally construct a NonEmptyVector
containing the elements, if any, of a given GenSeq
.
*
* @param seq the GenSeq
with which to construct a NonEmptyVector
* @return a NonEmptyVector
containing the elements of the given GenSeq
, if non-empty, wrapped in
* a Some
; else None
if the GenSeq
is empty
*/
def from[T](seq: GenSeq[T]): Option[NonEmptyVector[T]] =
seq.headOption match {
case None => None
case Some(first) => Some(new NonEmptyVector(Vector(first) ++ seq.tail.toVector))
}
import scala.language.implicitConversions
/**
* Implicit conversion from NonEmptyVector
to Vector
.
*
*
* One use case for this implicit conversion is to enable GenSeq[NonEmptyVector]
s to be flattened.
* Here's an example:
*
*
*
* scala> Vector(NonEmptyVector(1, 2, 3), NonEmptyVector(3, 4), NonEmptyVector(5, 6, 7, 8)).flatten
* res0: scala.collection.immutable.Vector[Int] = Vector(1, 2, 3, 3, 4, 5, 6, 7, 8)
*
*
* @param nonEmptyVector the NonEmptyVector
to convert to a Vector
* @return a Vector
containing the elements, in order, of this NonEmptyVector
*/
implicit def nonEmptyVectorToVector[E](nonEmptyVector: NonEmptyVector[E]): scala.collection.immutable.Vector[E] = nonEmptyVector.toVector
}