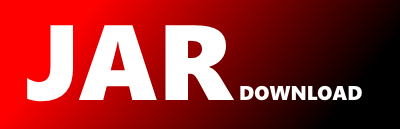
org.scalactic.anyvals.NumericChar.scala Maven / Gradle / Ivy
/*
* Copyright 2001-2017 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalactic.anyvals
import scala.language.implicitConversions
import scala.util.{Try, Success, Failure}
import org.scalactic.{Or, Good, Bad}
import org.scalactic.{Validation, Pass, Fail}
/**
* An AnyVal
for numeric Char
s.
*
* Note: a NumericChar
has a value between '0' and '9'.
*
*
* Because NumericChar
is an AnyVal
it will usually
* be as efficient as a Char
, being boxed only when a
* Char
would have been boxed.
*
*
*
* The NumericChar.apply
factory method is implemented in terms
* of a macro that checks literals for validity at compile time. Calling
* NumericChar.apply
with a literal Char
value will
* either produce a valid NumericChar
instance at run time or an
* error at compile time. Here's an example:
*
*
*
* scala> import anyvals._
* import anyvals._
*
* scala> NumericChar('4')
* res0: org.scalactic.anyvals.NumericChar = NumericChar('4')
*
* scala> NumericChar('a')
* <console>:14: error: NumericChar.apply can only be invoked on Char literals that are numeric, like NumericChar('4').
* NumericChar('a')
* ^
*
*
*
* NumericChar.apply
cannot be used if the value being passed
* is a variable (i.e., not a literal), because the macro cannot
* determine the validity of variables at compile time (just literals).
* If you try to pass a variable to NumericChar.apply
, you'll
* get a compiler error that suggests you use a different factory method,
* NumericChar.from
, instead:
*
*
*
* scala> val x = '1'
* x: Char = 1
*
* scala> NumericChar(x)
* <console>:15: error: NumericChar.apply can only be invoked on Char literals that are numeric, like NumericChar('4'). Please use NumericChar.from instead.
* NumericChar(x)
* ^
*
*
*
* The NumericChar.from
factory method will inspect the value at
* runtime and return an Option[NumericChar]
. If the value is
* valid, NumericChar.from
will return a
* Some[NumericChar]
, else it will return a None
.
* Here's an example:
*
*
*
* scala> NumericChar.from(x)
* res3: Option[org.scalactic.anyvals.NumericChar] = Some(NumericChar('1'))
*
* scala> val y = 'a'
* y: Char = a
*
* scala> NumericChar.from(y)
* res4: Option[org.scalactic.anyvals.NumericChar] = None
*
*
*
* The NumericChar.apply
factory method is marked implicit, so
* that you can pass literal Char
s into methods that require
* NumericChar
, and get the same compile-time checking you get
* when calling NumericChar.apply
explicitly. Here's an example:
*
*
*
* scala> def invert(ch: NumericChar): Char = ('9' - ch + '0').toChar
* invert: (ch: org.scalactic.anyvals.NumericChar)Char
*
* scala> invert('1')
* res6: Char = 8
*
* scala> scala> invert('9')
* res7: Char = 0
*
* scala> invert('a')
* <console>:12: error: NumericChar.apply can only be invoked on Char literals that are numeric, like NumericChar('4').
* invert('a')
* ^
*
*
* @param value The Char
value underlying this
* NumericChar
.
*/
final class NumericChar private (val value: Char) extends AnyVal {
/**
* A string representation of this NumericChar
.
*/
override def toString: String = s"NumericChar('${value.toString()}')"
/**
* Converts this NumericChar
to a Byte
.
*/
def toByte: Byte = value.toByte
/**
* Converts this NumericChar
to a Short
.
*/
def toShort: Short = value.toShort
/**
* Converts this NumericChar
to a Char
.
*/
def toChar: Char = value.toChar
/**
* Converts this NumericChar
to an Int
.
*/
def toInt: Int = value.toInt
/**
* Converts this NumericChar
to a Long
.
*/
def toLong: Long = value.toLong
/**
* Converts this NumericChar
to a Float
.
*/
def toFloat: Float = value.toFloat
/**
* Converts this NumericChar
to a Double
.
*/
def toDouble: Double = value.toDouble
def max(that: NumericChar): NumericChar =
if (math.max(value.toInt, that.value.toInt) == value.toInt) this
else that
def min(that: NumericChar): NumericChar =
if (math.min(value.toInt, that.value.toInt) == value.toInt) this
else that
def asDigit: Int = Character.digit(value, Character.MAX_RADIX) // from RichChar
def asDigitPosZInt: PosZInt = PosZInt.ensuringValid(asDigit)
/**
* Returns the bitwise negation of this value.
* @example {{{
* ~5 == -6
* // in binary: ~00000101 ==
* // 11111010
* }}}
*/
def unary_~ : Int = ~value
/** Returns this value, unmodified. */
def unary_+ : NumericChar = this
/** Returns the negation of this value. */
def unary_- : NegZInt = NegZInt.ensuringValid(-value)
/**
* Prepends this NumericChar
's value to a string.
*/
def +(x: String): String = s"${value.toString()}${x.toString()}"
/**
* Returns this value bit-shifted left by the specified number of bits,
* filling in the new right bits with zeroes.
* @example {{{ 6 << 3 == 48 // in binary: 0110 << 3 == 0110000 }}}
*/
def <<(x: Int): Int = value << x
/**
* Returns this value bit-shifted left by the specified number of bits,
* filling in the new right bits with zeroes.
* @example {{{ 6 << 3 == 48 // in binary: 0110 << 3 == 0110000 }}}
*/
def <<(x: Long): Int = value << x
/**
* Returns this value bit-shifted right by the specified number of bits,
* filling the new left bits with zeroes.
* @example {{{ 21 >>> 3 == 2 // in binary: 010101 >>> 3 == 010 }}}
* @example {{{
* -21 >>> 3 == 536870909
* // in binary: 11111111 11111111 11111111 11101011 >>> 3 ==
* // 00011111 11111111 11111111 11111101
* }}}
*/
def >>>(x: Int): Int = value >>> x
/**
* Returns this value bit-shifted right by the specified number of bits,
* filling the new left bits with zeroes.
* @example {{{ 21 >>> 3 == 2 // in binary: 010101 >>> 3 == 010 }}}
* @example {{{
* -21 >>> 3 == 536870909
* // in binary: 11111111 11111111 11111111 11101011 >>> 3 ==
* // 00011111 11111111 11111111 11111101
* }}}
*/
def >>>(x: Long): Int = value >>> x
/**
* Returns this value bit-shifted left by the specified number of bits,
* filling in the right bits with the same value as the left-most bit of this.
* The effect of this is to retain the sign of the value.
* @example {{{
* -21 >> 3 == -3
* // in binary: 11111111 11111111 11111111 11101011 >> 3 ==
* // 11111111 11111111 11111111 11111101
* }}}
*/
def >>(x: Int): Int = value >> x
/**
* Returns this value bit-shifted left by the specified number of bits,
* filling in the right bits with the same value as the left-most bit of this.
* The effect of this is to retain the sign of the value.
* @example {{{
* -21 >> 3 == -3
* // in binary: 11111111 11111111 11111111 11101011 >> 3 ==
* // 11111111 11111111 11111111 11111101
* }}}
*/
def >>(x: Long): Int = value >> x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Byte): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Short): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Char): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Int): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Long): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Float): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Double): Boolean = value < x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Byte): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Short): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Char): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Int): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Long): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Float): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Double): Boolean = value <= x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Byte): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Short): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Char): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Int): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Long): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Float): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Double): Boolean = value > x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Byte): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Short): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Char): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Int): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Long): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Float): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Double): Boolean = value >= x
/**
* Returns the bitwise OR of this value and `x`.
* @example {{{
* (0xf0 | 0xaa) == 0xfa
* // in binary: 11110000
* // | 10101010
* // --------
* // 11111010
* }}}
*/
def |(x: Byte): Int = value | x
/**
* Returns the bitwise OR of this value and `x`.
* @example {{{
* (0xf0 | 0xaa) == 0xfa
* // in binary: 11110000
* // | 10101010
* // --------
* // 11111010
* }}}
*/
def |(x: Short): Int = value | x
/**
* Returns the bitwise OR of this value and `x`.
* @example {{{
* (0xf0 | 0xaa) == 0xfa
* // in binary: 11110000
* // | 10101010
* // --------
* // 11111010
* }}}
*/
def |(x: Char): Int = value | x
/**
* Returns the bitwise OR of this value and `x`.
* @example {{{
* (0xf0 | 0xaa) == 0xfa
* // in binary: 11110000
* // | 10101010
* // --------
* // 11111010
* }}}
*/
def |(x: Int): Int = value | x
/**
* Returns the bitwise OR of this value and `x`.
* @example {{{
* (0xf0 | 0xaa) == 0xfa
* // in binary: 11110000
* // | 10101010
* // --------
* // 11111010
* }}}
*/
def |(x: Long): Long = value | x
/**
* Returns the bitwise AND of this value and `x`.
* @example {{{
* (0xf0 & 0xaa) == 0xa0
* // in binary: 11110000
* // & 10101010
* // --------
* // 10100000
* }}}
*/
def &(x: Byte): Int = value & x
/**
* Returns the bitwise AND of this value and `x`.
* @example {{{
* (0xf0 & 0xaa) == 0xa0
* // in binary: 11110000
* // & 10101010
* // --------
* // 10100000
* }}}
*/
def &(x: Short): Int = value & x
/**
* Returns the bitwise AND of this value and `x`.
* @example {{{
* (0xf0 & 0xaa) == 0xa0
* // in binary: 11110000
* // & 10101010
* // --------
* // 10100000
* }}}
*/
def &(x: Char): Int = value & x
/**
* Returns the bitwise AND of this value and `x`.
* @example {{{
* (0xf0 & 0xaa) == 0xa0
* // in binary: 11110000
* // & 10101010
* // --------
* // 10100000
* }}}
*/
def &(x: Int): Int = value & x
/**
* Returns the bitwise AND of this value and `x`.
* @example {{{
* (0xf0 & 0xaa) == 0xa0
* // in binary: 11110000
* // & 10101010
* // --------
* // 10100000
* }}}
*/
def &(x: Long): Long = value & x
/**
* Returns the bitwise XOR of this value and `x`.
* @example {{{
* (0xf0 ^ 0xaa) == 0x5a
* // in binary: 11110000
* // ^ 10101010
* // --------
* // 01011010
* }}}
*/
def ^(x: Byte): Int = value ^ x
/**
* Returns the bitwise XOR of this value and `x`.
* @example {{{
* (0xf0 ^ 0xaa) == 0x5a
* // in binary: 11110000
* // ^ 10101010
* // --------
* // 01011010
* }}}
*/
def ^(x: Short): Int = value ^ x
/**
* Returns the bitwise XOR of this value and `x`.
* @example {{{
* (0xf0 ^ 0xaa) == 0x5a
* // in binary: 11110000
* // ^ 10101010
* // --------
* // 01011010
* }}}
*/
def ^(x: Char): Int = value ^ x
/**
* Returns the bitwise XOR of this value and `x`.
* @example {{{
* (0xf0 ^ 0xaa) == 0x5a
* // in binary: 11110000
* // ^ 10101010
* // --------
* // 01011010
* }}}
*/
def ^(x: Int): Int = value ^ x
/**
* Returns the bitwise XOR of this value and `x`.
* @example {{{
* (0xf0 ^ 0xaa) == 0x5a
* // in binary: 11110000
* // ^ 10101010
* // --------
* // 01011010
* }}}
*/
def ^(x: Long): Long = value ^ x
/** Returns the sum of this value and `x`. */
def +(x: Byte): Int = value + x
/** Returns the sum of this value and `x`. */
def +(x: Short): Int = value + x
/** Returns the sum of this value and `x`. */
def +(x: Char): Int = value + x
/** Returns the sum of this value and `x`. */
def +(x: Int): Int = value + x
/** Returns the sum of this value and `x`. */
def +(x: Long): Long = value + x
/** Returns the sum of this value and `x`. */
def +(x: Float): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Double): Double = value + x
/** Returns the difference of this value and `x`. */
def -(x: Byte): Int = value - x
/** Returns the difference of this value and `x`. */
def -(x: Short): Int = value - x
/** Returns the difference of this value and `x`. */
def -(x: Char): Int = value - x
/** Returns the difference of this value and `x`. */
def -(x: Int): Int = value - x
/** Returns the difference of this value and `x`. */
def -(x: Long): Long = value - x
/** Returns the difference of this value and `x`. */
def -(x: Float): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Double): Double = value - x
/** Returns the product of this value and `x`. */
def *(x: Byte): Int = value * x
/** Returns the product of this value and `x`. */
def *(x: Short): Int = value * x
/** Returns the product of this value and `x`. */
def *(x: Char): Int = value * x
/** Returns the product of this value and `x`. */
def *(x: Int): Int = value * x
/** Returns the product of this value and `x`. */
def *(x: Long): Long = value * x
/** Returns the product of this value and `x`. */
def *(x: Float): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Double): Double = value * x
/** Returns the quotient of this value and `x`. */
def /(x: Byte): Int = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Short): Int = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Char): Int = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Int): Int = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Long): Long = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Float): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Double): Double = value / x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Byte): Int = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Short): Int = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Char): Int = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Int): Int = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Long): Long = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Float): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Double): Double = value % x
}
/**
* The companion object for NumericChar
that offers factory
* methods that produce NumericChar
s and maximum and minimum
* constant values for NumericChar
.
*/
object NumericChar {
/**
* A factory method that produces an Option[NumericChar]
given
* a Char
value.
*
*
* This method will inspect the passed Char
value and if
* it is a numeric Char
, i.e., between '0' and '9',
* it will return a NumericChar
representing that value,
* wrapped in a Some
. Otherwise, the passed Char
* value is not a numeric character value, so this method will return
* None
.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Char
literals at compile time, whereas from
* inspects Char
values at run time.
*
*
* @param value the Char
to inspect, and if numeric, return
* wrapped in a Some[NumericChar]
.
* @return the specified Char
value wrapped
* in a Some[NumericChar]
, if it is numeric, else
* None
.
*/
def from(value: Char): Option[NumericChar] =
if (NumericCharMacro.isValid(value)) Some(new NumericChar(value)) else None
/**
* A factory/assertion method that produces a NumericChar
given
* a valid Char
value, or throws AssertionError
,
* if given an invalid Char
value.
*
* Note: you should use this method only when you are convinced that it will
* always succeed, i.e., never throw an exception. It is good practice to
* add a comment near the invocation of this method indicating ''why'' you
* think it will always succeed to document your reasoning. If you are not
* sure an `ensuringValid` call will always succeed, you should use one of
* the other factory or validation methods provided on this object instead:
* `isValid`, `tryingValid`, `passOrElse`, `goodOrElse`, or `rightOrElse`.
*
*
* This method will inspect the passed Char
value and if
* it is a numeric Char
, it will return a
* NumericChar
representing that value. Otherwise, the
* passed Char
value is not numeric, so this method will
* throw AssertionError
.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Char
literals at compile time, whereas this method inspects
* Char
values at run time.
* It differs from a vanilla assert
or ensuring
* call in that you get something you didn't already have if the assertion
* succeeds: a type that promises a Char
is numeric.
*
*
* @param value the Char
to inspect, and if numeric, return
* wrapped in a NumericChar
.
* @return the specified Char
value wrapped
* in a NumericChar
, if it is numeric, else throws
* AssertionError
.
* @throws AssertionError if the passed value is not numeric
*/
def ensuringValid(value: Char): NumericChar =
if (NumericCharMacro.isValid(value)) new NumericChar(value) else {
throw new AssertionError(s"${value.toString()} was not a valid NumericChar")
}
import scala.language.experimental.macros
/**
* A factory method, implemented via a macro, that produces a
* NumericChar
if passed a valid Char
literal,
* otherwise a compile time error.
*
*
* The macro that implements this method will inspect the specified
* Char
expression at compile time. If the expression is a
* numeric Char
literal, i.e., a value between '0'
* and '9', it will return a NumericChar
representing that
* value. Otherwise, the passed Char
expression is either a
* literal that is not numeric, or is not a literal, so this method will
* give a compiler error.
*
*
*
* This factory method differs from the from
factory method
* in that this method is implemented via a macro that inspects
* Char
literals at compile time, whereas from
* inspects Char
values at run time.
*
*
* @param value the Char
literal expression to inspect at
* compile time, and if numeric, to return wrapped in a
* NumericChar
at run time.
* @return the specified, valid Char
literal value wrapped
* in a NumericChar
. (If the specified expression is not
* a valid Char
literal, the invocation of this method
* will not compile.)
*/
inline implicit def apply(value: => Char): NumericChar = ${ NumericCharMacro('{value}) }
/** The smallest value representable as a NumericChar. */
final val MinValue: NumericChar = NumericChar.ensuringValid('0')
/** The largest value representable as a NumericChar. */
final val MaxValue: NumericChar = NumericChar.ensuringValid('9')
/**
* A factory/validation method that produces a NumericChar
,
* wrapped in a Good
, given a valid Char
value,
* or if the given Char
is invalid, an error value of type
* B
produced by passing the given invalid
* Char
value to the given function f
, wrapped
* in a Bad
.
*
*
* This method will inspect the passed Char
value and if
* it is a numeric Char
, it will return a
* NumericChar
representing that value, wrapped in a
* Good
. Otherwise, the passed Char
value is
* NOT numeric, so this method will return a result of type B
* obtained by passing the invalid Char
value to the given
* function f
, wrapped in a `Bad`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Char
literals at compile time, whereas this method inspects
* Char
values at run time.
*
*
* @param value the Char
to inspect, and if numeric, return
* wrapped in a Good(NumericChar)
.
* @return the specified Char
value wrapped
* in a Good(NumericChar)
, if it is numeric, else a
* Bad(f(value))
.
*/
def goodOrElse[B](value: Char)(f: Char => B): NumericChar Or B =
if (NumericCharMacro.isValid(value)) Good(NumericChar.ensuringValid(value))
else Bad(f(value))
/**
* A validation method that produces a Pass
given a valid
* Char
value, or an error value of type E
* produced by passing the given invalid Char
value
* to the given function f
, wrapped in a Fail
.
*
*
* This method will inspect the passed Char
value and if
* it is a numeric Char
(between '0' and '9'), it will return
* a Pass
. Otherwise, the passed Char
value is
* non-numeric, so this method will return a result of type E
* obtained by passing the invalid Char
value to the given
* function f
, wrapped in a `Fail`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Char
literals at compile time, whereas this method inspects
* Char
values at run time.
*
*
* @param value the `Char` to validate that it is numeric.
* @return a `Pass` if the specified `Char` value is numeric,
* else a `Fail` containing an error value produced by passing the
* specified `Char` to the given function `f`.
*/
def passOrElse[E](value: Char)(f: Char => E): Validation[E] =
if (NumericCharMacro.isValid(value)) Pass else Fail(f(value))
/**
* A factory/validation method that produces a NumericChar
,
* wrapped in a Right
, given a valid Char
value,
* or if the given Char
is invalid, an error value of type
* L
produced by passing the given invalid
* Char
value to the given function f
, wrapped
* in a Left
.
*
*
* This method will inspect the passed Char
value and if
* it is a numeric Char
(between '0' and '9'), it will return a
* NumericChar
representing that value, wrapped in a
* Right
. Otherwise, the passed Char
value is
* NOT numeric, so this method will return a result of type L
* obtained by passing the invalid Char
value to the given
* function f
, wrapped in a `Left`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Char
literals at compile time, whereas this method inspects
* Char
values at run time.
*
*
* @param value the Char
to inspect, and if numeric, return
* wrapped in a Right(NumericChar)
.
* @return the specified Char
value wrapped
* in a Right(NumericChar)
, if it is numeric, else a
* Left(f(value))
.
*/
def rightOrElse[L](value: Char)(f: Char => L): Either[L, NumericChar] =
if (NumericCharMacro.isValid(value)) Right(NumericChar.ensuringValid(value))
else Left(f(value))
/**
* A factory/validation method that produces a NumericChar
,
* wrapped in a Success
, given a valid Char
* value, or if the given Char
is invalid, an
* AssertionError
, wrapped in a Failure
.
*
*
* This method will inspect the passed Char
value and if
* it represents a numeric value (between '0' and '9'), it will return a
* NumericChar
representing that value, wrapped in a
* Success
. Otherwise, the passed Char
value is
* not numeric, so this method will return an AssertionError
,
* wrapped in a Failure
.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Char
literals at compile time, whereas this method inspects
* Char
values at run time.
*
*
* @param value the Char
to inspect, and if numeric, return
* wrapped in a Success(NumericChar)
.
* @return the specified Char
value wrapped
* in a Success(NumericChar)
, if it is numeric, else a
* Failure(AssertionError)
.
*/
def tryingValid(value: Char): Try[NumericChar] =
if (NumericCharMacro.isValid(value)) Success(new NumericChar(value))
else Failure(new AssertionError(s"${value.toString()} was not a valid NumericChar"))
/**
* A factory method that produces a NumericChar
given a
* Char
value and a default NumericChar
.
*
*
* This method will inspect the passed Char
value and if
* it is a valid numeric Char (between '0' and '9'), it will return a
* NumericChar
representing that value. Otherwise, the passed
* Char
value is a non-digit character, so this method will
* return the passed default
value.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Char
literals at compile time, whereas
* fromOrElse
inspects Char
values at run time.
*
*
* @param value the Char
to inspect, and if numeric, return.
* @param default the NumericChar
to return if the passed
* Char
value is not numeric.
* @return the specified Char
value wrapped in a
* NumericChar
, if it is numeric, else the
* default
NumericChar
value.
*/
def fromOrElse(value: Char, default: => NumericChar): NumericChar =
if (NumericCharMacro.isValid(value)) new NumericChar(value)
else default
/**
* A predicate method that returns true if a given Char
value
* is between '0' and '9'.
*
* @param value the Char
to inspect, and if numeric, return true.
* @return true if the specified Char
is numeric, else false.
*/
def isValid(value: Char): Boolean = NumericCharMacro.isValid(value)
/** Language mandated coercions from Char to "wider" types. */
/**
* Implicit widening conversion from NumericChar
to Int
.
*
* @param value the NumericChar
to widen
* @return the Int
widen from the specified NumericChar
.
*/
implicit def widenToInt(value: NumericChar): Int = value.toInt
/**
* Implicit widening conversion from NumericChar
to Long
.
*
* @param value the NumericChar
to widen
* @return the Long
widen from the specified NumericChar
.
*/
implicit def widenToLong(value: NumericChar): Long = value.toLong
/**
* Implicit widening conversion from NumericChar
to Float
.
*
* @param value the NumericChar
to widen
* @return the Float
widen from the specified NumericChar
.
*/
implicit def widenToFloat(value: NumericChar): Float = value.toFloat
/**
* Implicit widening conversion from NumericChar
to Double
.
*
* @param value the NumericChar
to widen
* @return the Double
widen from the specified NumericChar
.
*/
implicit def widenToDouble(value: NumericChar): Double = value.toDouble
/**
* Implicit widening conversion from NumericChar
to FiniteFloat
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Float
and wrapped in a FiniteFloat
.
*/
implicit def widenToFiniteFloat(pos: NumericChar): FiniteFloat = FiniteFloat.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to FiniteDouble
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Double
and wrapped in a FiniteDouble
.
*/
implicit def widenToFiniteDouble(pos: NumericChar): FiniteDouble = FiniteDouble.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosInt
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Int
and wrapped in a PosInt
.
*/
implicit def widenToPosInt(pos: NumericChar): PosInt = PosInt.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosLong
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Long
and wrapped in a PosLong
.
*/
implicit def widenToPosLong(pos: NumericChar): PosLong = PosLong.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosFloat
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Float
and wrapped in a PosFloat
.
*/
implicit def widenToPosFloat(pos: NumericChar): PosFloat = PosFloat.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosDouble
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Double
and wrapped in a PosDouble
.
*/
implicit def widenToPosDouble(pos: NumericChar): PosDouble = PosDouble.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosZInt
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Int
and wrapped in a PosZInt
.
*/
implicit def widenToPosZInt(pos: NumericChar): PosZInt = PosZInt.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosZLong
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Long
and wrapped in a PosZLong
.
*/
implicit def widenToPosZLong(pos: NumericChar): PosZLong = PosZLong.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosZFloat
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Float
and wrapped in a PosZFloat
.
*/
implicit def widenToPosZFloat(pos: NumericChar): PosZFloat = PosZFloat.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosZDouble
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Double
and wrapped in a PosZDouble
.
*/
implicit def widenToPosZDouble(pos: NumericChar): PosZDouble = PosZDouble.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosFiniteFloat
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Float
and wrapped in a PosFiniteFloat
.
*/
implicit def widenToPosFiniteFloat(pos: NumericChar): PosFiniteFloat = PosFiniteFloat.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosFiniteDouble
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Double
and wrapped in a PosFiniteDouble
.
*/
implicit def widenToPosFiniteDouble(pos: NumericChar): PosFiniteDouble = PosFiniteDouble.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosZFiniteFloat
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Float
and wrapped in a PosZFiniteFloat
.
*/
implicit def widenToPosZFiniteFloat(pos: NumericChar): PosZFiniteFloat = PosZFiniteFloat.ensuringValid(pos.value)
/**
* Implicit widening conversion from NumericChar
to PosZFiniteDouble
.
*
* @param pos the NumericChar
to widen
* @return the Int
value underlying the specified NumericChar
,
* widened to Double
and wrapped in a PosZFiniteDouble
.
*/
implicit def widenToPosZFiniteDouble(pos: NumericChar): PosZFiniteDouble = PosZFiniteDouble.ensuringValid(pos.value)
}