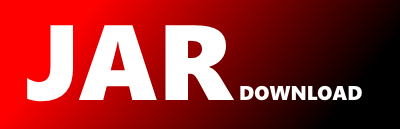
org.scalactic.anyvals.PosZFloat.scala Maven / Gradle / Ivy
/*
* Copyright 2001-2016 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalactic.anyvals
import scala.collection.immutable.NumericRange
import scala.language.implicitConversions
import scala.util.{Try, Success, Failure}
import org.scalactic.{Validation, Pass, Fail}
import org.scalactic.{Or, Good, Bad}
/**
* An AnyVal
for non-negative Float
s.
*
*
*
*
*
*
* Because PosZFloat
is an AnyVal
it
* will usually be as efficient as an Float
, being
* boxed only when an Float
would have been boxed.
*
*
*
* The PosZFloat.apply
factory method is implemented
* in terms of a macro that checks literals for validity at
* compile time. Calling PosZFloat.apply
with a
* literal Float
value will either produce a valid
* PosZFloat
instance at run time or an error at
* compile time. Here's an example:
*
*
*
* scala> import anyvals._
* import anyvals._
*
* scala> PosZFloat(1.1fF)
* res0: org.scalactic.anyvals.PosZFloat = PosZFloat(1.1f)
*
* scala> PosZFloat(-1.1fF)
* <console>:14: error: PosZFloat.apply can only be invoked on a non-negative (i >= 0.0f) floating point literal, like PosZFloat(1.1fF).
* PosZFloat(1.1fF)
* ^
*
*
*
* PosZFloat.apply
cannot be used if the value being
* passed is a variable (i.e., not a literal), because
* the macro cannot determine the validity of variables at
* compile time (just literals). If you try to pass a variable
* to PosZFloat.apply
, you'll get a compiler error
* that suggests you use a different factor method,
* PosZFloat.from
, instead:
*
*
*
* scala> val x = 1.1fF
* x: Float = 1.1f
*
* scala> PosZFloat(x)
* <console>:15: error: PosZFloat.apply can only be invoked on a floating point literal, like PosZFloat(1.1fF). Please use PosZFloat.from instead.
* PosZFloat(x)
* ^
*
*
*
* The PosZFloat.from
factory method will inspect
* the value at runtime and return an
* Option[PosZFloat]
. If the value is valid,
* PosZFloat.from
will return a
* Some[PosZFloat]
, else it will return a
* None
. Here's an example:
*
*
*
* scala> PosZFloat.from(x)
* res3: Option[org.scalactic.anyvals.PosZFloat] = Some(PosZFloat(1.1f))
*
* scala> val y = -1.1fF
* y: Float = -1.1f
*
* scala> PosZFloat.from(y)
* res4: Option[org.scalactic.anyvals.PosZFloat] = None
*
*
*
* The PosZFloat.apply
factory method is marked
* implicit, so that you can pass literal Float
s
* into methods that require PosZFloat
, and get the
* same compile-time checking you get when calling
* PosZFloat.apply
explicitly. Here's an example:
*
*
*
* scala> def invert(pos: PosZFloat): Float = Float.MaxValue - pos
* invert: (pos: org.scalactic.anyvals.PosZFloat)Float
*
* scala> invert(1.1fF)
* res5: Float = 3.4028235E38
*
* scala> invert(Float.MaxValue)
* res6: Float = 0.0
*
* scala> invert(-1.1fF)
* <console>:15: error: PosZFloat.apply can only be invoked on a non-negative (i >= 0.0f) floating point literal, like PosZFloat(1.1fF).
* invert(0.0F)
* ^
*
* scala> invert(-1.1fF)
* <console>:15: error: PosZFloat.apply can only be invoked on a non-negative (i >= 0.0f) floating point literal, like PosZFloat(1.1fF).
* invert(-1.1fF)
* ^
*
*
*
*
* This example also demonstrates that the PosZFloat
* companion object also defines implicit widening conversions
* when no loss of precision will occur. This makes it convenient to use a
* PosZFloat
where a Float
or wider
* type is needed. An example is the subtraction in the body of
* the invert
method defined above,
* Float.MaxValue - pos
. Although
* Float.MaxValue
is a Float
, which
* has no -
method that takes a
* PosZFloat
(the type of pos
), you can
* still subtract pos
, because the
* PosZFloat
will be implicitly widened to
* Float
.
*
*
* @param value The Float
value underlying this PosZFloat
.
*/
final class PosZFloat private (val value: Float) extends AnyVal {
/**
* A string representation of this PosZFloat
.
*/
override def toString: String = s"PosZFloat(${value.toString()}f)"
/**
* Converts this PosZFloat
to a Byte
.
*/
def toByte: Byte = value.toByte
/**
* Converts this PosZFloat
to a Short
.
*/
def toShort: Short = value.toShort
/**
* Converts this PosZFloat
to a Char
.
*/
def toChar: Char = value.toChar
/**
* Converts this PosZFloat
to an Int
.
*/
def toInt: Int = value.toInt
/**
* Converts this PosZFloat
to a Long
.
*/
def toLong: Long = value.toLong
/**
* Converts this PosZFloat
to a Float
.
*/
def toFloat: Float = value.toFloat
/**
* Converts this PosZFloat
to a Double
.
*/
def toDouble: Double = value.toDouble
/** Returns this value, unmodified. */
def unary_+ : PosZFloat = this
/** Returns the negation of this value. */
def unary_- : NegZFloat = NegZFloat.ensuringValid(-value)
/**
* Converts this PosZFloat
's value to a string then concatenates the given string.
*/
def +(x: String): String = s"${value.toString()}${x.toString()}"
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Byte): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Short): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Char): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Int): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Long): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Float): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Double): Boolean = value < x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Byte): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Short): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Char): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Int): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Long): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Float): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Double): Boolean = value <= x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Byte): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Short): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Char): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Int): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Long): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Float): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Double): Boolean = value > x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Byte): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Short): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Char): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Int): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Long): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Float): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Double): Boolean = value >= x
/** Returns the sum of this value and `x`. */
def +(x: Byte): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Short): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Char): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Int): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Long): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Float): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Double): Double = value + x
/** Returns the difference of this value and `x`. */
def -(x: Byte): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Short): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Char): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Int): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Long): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Float): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Double): Double = value - x
/** Returns the product of this value and `x`. */
def *(x: Byte): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Short): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Char): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Int): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Long): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Float): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Double): Double = value * x
/** Returns the quotient of this value and `x`. */
def /(x: Byte): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Short): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Char): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Int): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Long): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Float): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Double): Double = value / x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Byte): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Short): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Char): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Int): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Long): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Float): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Double): Double = value % x
// Stuff from RichFloat
/**
* Returns this
if this > that
or that
otherwise.
*/
def max(that: PosZFloat): PosZFloat = if (math.max(value, that.value) == value) this else that
/**
* Returns this
if this < that
or that
otherwise.
*/
def min(that: PosZFloat): PosZFloat = if (math.min(value, that.value) == value) this else that
/**
* Indicates whether this `PosZFloat` has a value that is a whole number: it is finite and it has no fraction part.
*/
def isWhole = {
val longValue = value.toLong
longValue.toFloat == value || longValue == Long.MaxValue && value < Float.PositiveInfinity || longValue == Long.MinValue && value > Float.NegativeInfinity
}
/** Converts an angle measured in degrees to an approximately equivalent
* angle measured in radians.
*
* @return the measurement of the angle x in radians.
*/
def toRadians: Float = math.toRadians(value.toDouble).toFloat
/** Converts an angle measured in radians to an approximately equivalent
* angle measured in degrees.
* @return the measurement of the angle x in degrees.
*/
def toDegrees: Float = math.toDegrees(value.toDouble).toFloat
/**
* Applies the passed Float => Float
function to the underlying Float
* value, and if the result is positive, returns the result wrapped in a PosZFloat
,
* else throws AssertionError
.
*
*
* This method will inspect the result of applying the given function to this
* PosZFloat
's underlying Float
value and if the result
* is non-negative, it will return a PosZFloat
representing that value.
* Otherwise, the Float
value returned by the given function is
* not non-negative, so this method will throw AssertionError
.
*
*
*
* This method differs from a vanilla assert
or ensuring
* call in that you get something you didn't already have if the assertion
* succeeds: a type that promises an Float
is non-negative.
* With this method, you are asserting that you are convinced the result of
* the computation represented by applying the given function to this PosZFloat
's
* value will not produce invalid value.
* Instead of producing such invalid values, this method will throw AssertionError
.
*
*
* @param f the Float => Float
function to apply to this PosZFloat
's
* underlying Float
value.
* @return the result of applying this PosZFloat
's underlying Float
value to
* to the passed function, wrapped in a PosZFloat
if it is non-negative (else throws AssertionError
).
* @throws AssertionError if the result of applying this PosZFloat
's underlying Float
value to
* to the passed function is not non-negative.
*/
def ensuringValid(f: Float => Float): PosZFloat = {
val candidateResult: Float = f(value)
if (PosZFloatMacro.isValid(candidateResult)) new PosZFloat(candidateResult)
else throw new AssertionError(s"${candidateResult.toString()}, the result of applying the passed function to ${value.toString()}, was not a valid PosZFloat")
}
/**
* Rounds this `PosZFloat` value to the nearest whole number value that can be expressed as an `PosZInt`, returning the result as a `PosZInt`.
*/
def round: PosZInt = PosZInt.ensuringValid(math.round(value))
/**
* Returns the smallest (closest to 0) `PosZFloat` that is greater than or equal to this `PosZFloat`
* and represents a mathematical integer.
*/
def ceil: PosZFloat = PosZFloat.ensuringValid(math.ceil(value).toFloat)
/**
* Returns the greatest (closest to infinity) `PosZFloat` that is less than or equal to
* this `PosZFloat` and represents a mathematical integer.
*/
def floor: PosZFloat = PosZFloat.ensuringValid(math.floor(value).toFloat)
/**
* Returns the PosZFloat
sum of this value and `x`.
*
*
* This method will always succeed (not throw an exception) because
* adding a non-negative Float to another non-negative Float
* will always result in another non-negative Float
* value (though the result may be infinity).
*
*/
def plus(x: PosZFloat): PosZFloat = PosZFloat.ensuringValid(value + x)
/**
* True if this PosZFloat
value represents positive infinity, else false.
*/
def isPosInfinity: Boolean = Float.PositiveInfinity == value
/**
* True if this PosZFloat
value is any finite value (i.e., it is neither positive nor negative infinity), else false.
*/
def isFinite: Boolean = !value.isInfinite
}
/**
* The companion object for PosZFloat
that offers
* factory methods that produce PosZFloat
s,
* implicit widening conversions from PosZFloat
to
* other numeric types, and maximum and minimum constant values
* for PosZFloat
.
*/
object PosZFloat {
/**
* The largest value representable as a non-negative Float
,
* which is PosZFloat(3.4028235E38)
.
*/
final val MaxValue: PosZFloat = PosZFloat.ensuringValid(Float.MaxValue)
/**
* The smallest value representable as a non-negative
* Float
, which is PosZFloat(0.0f)
.
*/
final val MinValue: PosZFloat = PosZFloat.ensuringValid(0.0f) // Can't use the macro here
/**
* A factory method that produces an Option[PosZFloat]
given a
* Float
value.
*
*
* This method will inspect the passed Float
value and if
* it is a non-negative Float
, it will return a PosZFloat
* representing that value wrapped in a Some
. Otherwise, the passed Float
* value is not non-negative, so this method will return None
.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Float
literals at
* compile time, whereas from
inspects
* Float
values at run time.
*
*
* @param value the Float
to inspect, and if non-negative, return
* wrapped in a Some[PosZFloat]
.
* @return the specified Float
value wrapped in a
* Some[PosZFloat]
, if it is non-negative, else
* None
.
*/
def from(value: Float): Option[PosZFloat] =
if (PosZFloatMacro.isValid(value)) Some(new PosZFloat(value)) else None
/**
* A factory/assertion method that produces a PosZFloat
given a
* valid Float
value, or throws AssertionError
,
* if given an invalid Float
value.
*
* Note: you should use this method only when you are convinced that it will
* always succeed, i.e., never throw an exception. It is good practice to
* add a comment near the invocation of this method indicating ''why'' you think
* it will always succeed to document your reasoning. If you are not sure an
* `ensuringValid` call will always succeed, you should use one of the other
* factory or validation methods provided on this object instead: `isValid`,
* `tryingValid`, `passOrElse`, `goodOrElse`, or `rightOrElse`.
*
*
* This method will inspect the passed Float
value and if
* it is a non-negative Float
, it will return a PosZFloat
representing that value.
* Otherwise, the passed Float
value is not non-negative, so
* this method will throw AssertionError
.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Float
literals at
* compile time, whereas from
inspects
* Float
values at run time.
* It differs from a vanilla assert
or ensuring
* call in that you get something you didn't already have if the assertion
* succeeds: a type that promises a Float
is positive.
*
*
* @param value the Float
to inspect, and if non-negative, return
* wrapped in a PosZFloat
.
* @return the specified Float
value wrapped in a
* PosZFloat
, if it is non-negative, else
* throws AssertionError
.
* @throws AssertionError if the passed value is not non-negative
*/
def ensuringValid(value: Float): PosZFloat =
if (PosZFloatMacro.isValid(value)) new PosZFloat(value) else {
throw new AssertionError(s"${value.toString()} was not a valid PosZFloat")
}
/**
* A factory/validation method that produces a PosZFloat
, wrapped
* in a Success
, given a valid Float
value, or if the
* given Float
is invalid, an AssertionError
, wrapped
* in a Failure
.
*
*
* This method will inspect the passed Float
value and if
* it is a non-negative Float
, it will return a PosZFloat
* representing that value, wrapped in a Success
.
* Otherwise, the passed Float
value is not non-negative, so this
* method will return an AssertionError
, wrapped in a Failure
.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Float
literals at compile time, whereas this method inspects
* Float
values at run time.
*
*
* @param value the Float
to inspect, and if non-negative, return
* wrapped in a Success(PosZFloat)
.
* @return the specified Float
value wrapped
* in a Success(PosZFloat)
, if it is non-negative, else a Failure(AssertionError)
.
*/
def tryingValid(value: Float): Try[PosZFloat] =
if (PosZFloatMacro.isValid(value))
Success(new PosZFloat(value))
else
Failure(new AssertionError(s"${value.toString()} was not a valid PosZFloat"))
/**
* A validation method that produces a Pass
* given a valid Float
value, or
* an error value of type E
produced by passing the
* given invalid Int
value
* to the given function f
, wrapped in a Fail
.
*
*
* This method will inspect the passed Float
value and if
* it is a non-negative Float
, it will return a Pass
.
* Otherwise, the passed Float
value is non-negative, so this
* method will return a result of type E
obtained by passing
* the invalid Float
value to the given function f
,
* wrapped in a `Fail`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Float
literals at compile time, whereas this method inspects
* Float
values at run time.
*
*
* @param value the `Float` to validate that it is non-negative.
* @return a `Pass` if the specified `Float` value is non-negative,
* else a `Fail` containing an error value produced by passing the
* specified `Float` to the given function `f`.
*/
def passOrElse[E](value: Float)(f: Float => E): Validation[E] =
if (PosZFloatMacro.isValid(value)) Pass else Fail(f(value))
/**
* A factory/validation method that produces a PosZFloat
, wrapped
* in a Good
, given a valid Float
value, or if the
* given Float
is invalid, an error value of type B
* produced by passing the given invalid Float
value
* to the given function f
, wrapped in a Bad
.
*
*
* This method will inspect the passed Float
value and if
* it is a non-negative Float
, it will return a PosZFloat
* representing that value, wrapped in a Good
.
* Otherwise, the passed Float
value is not non-negative, so this
* method will return a result of type B
obtained by passing
* the invalid Float
value to the given function f
,
* wrapped in a `Bad`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Float
literals at compile time, whereas this method inspects
* Float
values at run time.
*
*
* @param value the Float
to inspect, and if non-negative, return
* wrapped in a Good(PosZFloat)
.
* @return the specified Float
value wrapped
* in a Good(PosZFloat)
, if it is non-negative, else a Bad(f(value))
.
*/
def goodOrElse[B](value: Float)(f: Float => B): PosZFloat Or B =
if (PosZFloatMacro.isValid(value)) Good(PosZFloat.ensuringValid(value)) else Bad(f(value))
/**
* A factory/validation method that produces a PosZFloat
, wrapped
* in a Right
, given a valid Int
value, or if the
* given Int
is invalid, an error value of type L
* produced by passing the given invalid Int
value
* to the given function f
, wrapped in a Left
.
*
*
* This method will inspect the passed Int
value and if
* it is a non-negative Int
, it will return a PosZFloat
* representing that value, wrapped in a Right
.
* Otherwise, the passed Int
value is not non-negative, so this
* method will return a result of type L
obtained by passing
* the invalid Int
value to the given function f
,
* wrapped in a `Left`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Int
literals at compile time, whereas this method inspects
* Int
values at run time.
*
*
* @param value the Int
to inspect, and if non-negative, return
* wrapped in a Right(PosZFloat)
.
* @return the specified Int
value wrapped
* in a Right(PosZFloat)
, if it is non-negative, else a Left(f(value))
.
*/
def rightOrElse[L](value: Float)(f: Float => L): Either[L, PosZFloat] =
if (PosZFloatMacro.isValid(value)) Right(PosZFloat.ensuringValid(value)) else Left(f(value))
/**
* A predicate method that returns true if a given
* Float
value is non-negative.
*
* @param value the Float
to inspect, and if non-negative, return true.
* @return true if the specified Float
is non-negative, else false.
*/
def isValid(value: Float): Boolean = PosZFloatMacro.isValid(value)
/**
* A factory method that produces a PosZFloat
given a
* Float
value and a default PosZFloat
.
*
*
* This method will inspect the passed Float
value and if
* it is a non-negative Float
, it will return a PosZFloat
representing that value.
* Otherwise, the passed Float
value is not non-negative, so this
* method will return the passed default
value.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Float
literals at
* compile time, whereas from
inspects
* Float
values at run time.
*
*
* @param value the Float
to inspect, and if non-negative, return.
* @param default the PosZFloat
to return if the passed
* Float
value is not non-negative.
* @return the specified Float
value wrapped in a
* PosZFloat
, if it is non-negative, else the
* default
PosZFloat
value.
*/
def fromOrElse(value: Float, default: => PosZFloat): PosZFloat =
if (PosZFloatMacro.isValid(value)) new PosZFloat(value) else default
import language.experimental.macros
import scala.language.implicitConversions
/**
* A factory method, implemented via a macro, that produces a
* PosZFloat
if passed a valid Float
* literal, otherwise a compile time error.
*
*
* The macro that implements this method will inspect the
* specified Float
expression at compile time. If
* the expression is a non-negative Float
literal,
* it will return a PosZFloat
representing that value.
* Otherwise, the passed Float
expression is either a literal
* that is not non-negative, or is not a literal, so this method
* will give a compiler error.
*
*
*
* This factory method differs from the from
* factory method in that this method is implemented via a
* macro that inspects Float
literals at compile
* time, whereas from
inspects Float
* values at run time.
*
*
* @param value the Float
literal expression to
* inspect at compile time, and if non-negative, to return
* wrapped in a PosZFloat
at run time.
* @return the specified, valid Float
literal
* value wrapped in a PosZFloat
. (If the
* specified expression is not a valid Float
* literal, the invocation of this method will not
* compile.)
*/
inline implicit def apply(value: => Float): PosZFloat = ${ PosZFloatMacro('{value}) }
/**
* Implicit widening conversion from PosZFloat
to
* Float
.
*
* @param pos the PosZFloat
to widen
* @return the Float
value underlying the
* specified PosZFloat
*/
implicit def widenToFloat(pos: PosZFloat): Float = pos.value
/**
* Implicit widening conversion from PosZFloat
to
* Double
.
*
* @param pos the PosZFloat
to widen
* @return the Float
value underlying the
* specified PosZFloat
, widened to
* Double
.
*/
implicit def widenToDouble(pos: PosZFloat): Double = pos.value
/**
* Implicit widening conversion from PosZFloat
to PosZDouble
.
*
* @param pos the PosZFloat
to widen
* @return the Float
value underlying the specified PosZFloat
,
* widened to Double
and wrapped in a PosZDouble
.
*/
implicit def widenToPosZDouble(pos: PosZFloat): PosZDouble = PosZDouble.ensuringValid(pos.value)
/**
* Implicit Ordering instance.
*/
implicit val ordering: Ordering[PosZFloat] =
new Ordering[PosZFloat] {
def compare(x: PosZFloat, y: PosZFloat): Int = x.toFloat.compare(y)
}
/**
* The positive infinity value, which is PosZFloat.ensuringValid(Float.PositiveInfinity)
.
*/
final val PositiveInfinity: PosZFloat = PosZFloat.ensuringValid(Float.PositiveInfinity) // Can't use the macro here
/**
* The smallest positive value greater than 0.0d representable as a PosZFloat
, which is PosZFloat(1.4E-45).
*/
final val MinPositiveValue: PosZFloat = PosZFloat.ensuringValid(Float.MinPositiveValue)
/**
* The formerly implicit posZFloatOrd
field has been deprecated and will be removed in a future version of ScalaTest. Please use the ordering
field instead.
*/
@deprecated("The formerly implicit posZFloatOrd field has been deprecated and will be removed in a future version of ScalaTest. Please use the ordering field instead.")
val posZFloatOrd: Ordering[PosZFloat] =
new Ordering[PosZFloat] {
def compare(x: PosZFloat, y: PosZFloat): Int = ordering.compare(x, y)
}
}