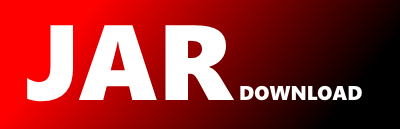
org.scalactic.anyvals.PosZLong.scala Maven / Gradle / Ivy
/* * Copyright 2001-2016 Artima, Inc. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package org.scalactic.anyvals import scala.collection.immutable.NumericRange import scala.language.implicitConversions import scala.util.{Try, Success, Failure} import org.scalactic.{Validation, Pass, Fail} import org.scalactic.{Or, Good, Bad} /** * An
Double in * Scala can lose precision.) This makes it convenient to use a *AnyVal
for non-negativeLong
s. * * * ** Because
* *PosZLong
is anAnyVal
it * will usually be as efficient as anLong
, being * boxed only when anLong
would have been boxed. ** The
* *PosZLong.apply
factory method is implemented * in terms of a macro that checks literals for validity at * compile time. CallingPosZLong.apply
with a * literalLong
value will either produce a valid *PosZLong
instance at run time or an error at * compile time. Here's an example: ** scala> import anyvals._ * import anyvals._ * * scala> PosZLong(42) * res0: org.scalactic.anyvals.PosZLong = PosZLong(42) * * scala> PosZLong(-1) * <console>:14: error: PosZLong.apply can only be invoked on a non-negative (i >= 0L) integer literal, like PosZLong(42). * PosZLong(-1) * ^ ** **
* *PosZLong.apply
cannot be used if the value being * passed is a variable (i.e., not a literal), because * the macro cannot determine the validity of variables at * compile time (just literals). If you try to pass a variable * toPosZLong.apply
, you'll get a compiler error * that suggests you use a different factor method, *PosZLong.from
, instead: ** scala> val x = 42L * x: Long = 42 * * scala> PosZLong(x) * <console>:15: error: PosZLong.apply can only be invoked on an long literal, like PosZLong(42). Please use PosZLong.from instead. * PosZLong(x) * ^ ** ** The
* *PosZLong.from
factory method will inspect the * value at runtime and return an *Option[PosZLong]
. If the value is valid, *PosZLong.from
will return a *Some[PosZLong]
, else it will return a *None
. Here's an example: ** scala> PosZLong.from(x) * res3: Option[org.scalactic.anyvals.PosZLong] = Some(PosZLong(42)) * * scala> val y = -1L * y: Long = -1 * * scala> PosZLong.from(y) * res4: Option[org.scalactic.anyvals.PosZLong] = None ** ** The
* *PosZLong.apply
factory method is marked * implicit, so that you can pass literalLong
s * into methods that requirePosZLong
, and get the * same compile-time checking you get when calling *PosZLong.apply
explicitly. Here's an example: ** scala> def invert(pos: PosZLong): Long = Long.MaxValue - pos * invert: (pos: org.scalactic.anyvals.PosZLong)Long * * scala> invert(1L) * res5: Long = 9223372036854775806 * * scala> invert(Long.MaxValue) * res6: Long = 0 * * scala> invert(-1L) * <console>:15: error: PosZLong.apply can only be invoked on a non-negative (i >= 0L) integer literal, like PosZLong(42L). * invert(-1L) * ^ * ** ** This example also demonstrates that the
PosZLong
* companion object also defines implicit widening conversions * when either no loss of precision will occur or a similar * conversion is provided in Scala. (For example, the implicit * conversion fromLong
toPosZLong
where aLong
or wider type * is needed. An example is the subtraction in the body of the *invert
method defined above,Long.MaxValue * - pos
. AlthoughLong.MaxValue
is a *Long
, which has no-
method that * takes aPosZLong
(the type ofpos
), * you can still subtractpos
, because the *PosZLong
will be implicitly widened to *Long
. * * * @param value TheLong
value underlying thisPosZLong
. */ final class PosZLong private (val value: Long) extends AnyVal { /** * A string representation of thisPosZLong
. */ override def toString: String = s"PosZLong(${value}L)" /** * Converts thisPosZLong
to aByte
. */ def toByte: Byte = value.toByte /** * Converts thisPosZLong
to aShort
. */ def toShort: Short = value.toShort /** * Converts thisPosZLong
to aChar
. */ def toChar: Char = value.toChar /** * Converts thisPosZLong
to anInt
. */ def toInt: Int = value.toInt /** * Converts thisPosZLong
to aLong
. */ def toLong: Long = value.toLong /** * Converts thisPosZLong
to aFloat
. */ def toFloat: Float = value.toFloat /** * Converts thisPosZLong
to aDouble
. */ def toDouble: Double = value.toDouble /** * Returns the bitwise negation of this value. * @example {{{ * ~5 == -6 * // in binary: ~00000101 == * // 11111010 * }}} */ def unary_~ : Long = ~value /** Returns this value, unmodified. */ def unary_+ : PosZLong = this /** Returns the negation of this value. */ def unary_- : NegZLong = NegZLong.ensuringValid(-value) /** * Converts thisPosZLong
's value to a string then concatenates the given string. */ def +(x: String): String = s"${value.toString()}$x" /** * Returns this value bit-shifted left by the specified number of bits, * filling in the new right bits with zeroes. * @example {{{ 6 << 3 == 48 // in binary: 0110 << 3 == 0110000 }}} */ def <<(x: Int): Long = value << x /** * Returns this value bit-shifted left by the specified number of bits, * filling in the new right bits with zeroes. * @example {{{ 6 << 3 == 48 // in binary: 0110 << 3 == 0110000 }}} */ def <<(x: Long): Long = value << x /** * Returns this value bit-shifted right by the specified number of bits, * filling the new left bits with zeroes. * @example {{{ 21 >>> 3 == 2 // in binary: 010101 >>> 3 == 010 }}} * @example {{{ * -21 >>> 3 == 536870909 * // in binary: 11111111 11111111 11111111 11101011 >>> 3 == * // 00011111 11111111 11111111 11111101 * }}} */ def >>>(x: Int): Long = value >>> x /** * Returns this value bit-shifted right by the specified number of bits, * filling the new left bits with zeroes. * @example {{{ 21 >>> 3 == 2 // in binary: 010101 >>> 3 == 010 }}} * @example {{{ * -21 >>> 3 == 536870909 * // in binary: 11111111 11111111 11111111 11101011 >>> 3 == * // 00011111 11111111 11111111 11111101 * }}} */ def >>>(x: Long): Long = value >>> x /** * Returns this value bit-shifted left by the specified number of bits, * filling in the right bits with the same value as the left-most bit of this. * The effect of this is to retain the sign of the value. * @example {{{ * -21 >> 3 == -3 * // in binary: 11111111 11111111 11111111 11101011 >> 3 == * // 11111111 11111111 11111111 11111101 * }}} */ def >>(x: Int): Long = value >> x /** * Returns this value bit-shifted left by the specified number of bits, * filling in the right bits with the same value as the left-most bit of this. * The effect of this is to retain the sign of the value. * @example {{{ * -21 >> 3 == -3 * // in binary: 11111111 11111111 11111111 11101011 >> 3 == * // 11111111 11111111 11111111 11111101 * }}} */ def >>(x: Long): Long = value >> x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Byte): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Short): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Char): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Int): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Long): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Float): Boolean = value < x /** Returns `true` if this value is less than x, `false` otherwise. */ def <(x: Double): Boolean = value < x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Byte): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Short): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Char): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Int): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Long): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Float): Boolean = value <= x /** Returns `true` if this value is less than or equal to x, `false` otherwise. */ def <=(x: Double): Boolean = value <= x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Byte): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Short): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Char): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Int): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Long): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Float): Boolean = value > x /** Returns `true` if this value is greater than x, `false` otherwise. */ def >(x: Double): Boolean = value > x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Byte): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Short): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Char): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Int): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Long): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Float): Boolean = value >= x /** Returns `true` if this value is greater than or equal to x, `false` otherwise. */ def >=(x: Double): Boolean = value >= x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Byte): Long = value | x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Short): Long = value | x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Char): Long = value | x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Int): Long = value | x /** * Returns the bitwise OR of this value and `x`. * @example {{{ * (0xf0 | 0xaa) == 0xfa * // in binary: 11110000 * // | 10101010 * // -------- * // 11111010 * }}} */ def |(x: Long): Long = value | x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Byte): Long = value & x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Short): Long = value & x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Char): Long = value & x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Int): Long = value & x /** * Returns the bitwise AND of this value and `x`. * @example {{{ * (0xf0 & 0xaa) == 0xa0 * // in binary: 11110000 * // & 10101010 * // -------- * // 10100000 * }}} */ def &(x: Long): Long = value & x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Byte): Long = value ^ x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Short): Long = value ^ x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Char): Long = value ^ x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Int): Long = value ^ x /** * Returns the bitwise XOR of this value and `x`. * @example {{{ * (0xf0 ^ 0xaa) == 0x5a * // in binary: 11110000 * // ^ 10101010 * // -------- * // 01011010 * }}} */ def ^(x: Long): Long = value ^ x /** Returns the sum of this value and `x`. */ def +(x: Byte): Long = value + x /** Returns the sum of this value and `x`. */ def +(x: Short): Long = value + x /** Returns the sum of this value and `x`. */ def +(x: Char): Long = value + x /** Returns the sum of this value and `x`. */ def +(x: Int): Long = value + x /** Returns the sum of this value and `x`. */ def +(x: Long): Long = value + x /** Returns the sum of this value and `x`. */ def +(x: Float): Float = value + x /** Returns the sum of this value and `x`. */ def +(x: Double): Double = value + x /** Returns the difference of this value and `x`. */ def -(x: Byte): Long = value - x /** Returns the difference of this value and `x`. */ def -(x: Short): Long = value - x /** Returns the difference of this value and `x`. */ def -(x: Char): Long = value - x /** Returns the difference of this value and `x`. */ def -(x: Int): Long = value - x /** Returns the difference of this value and `x`. */ def -(x: Long): Long = value - x /** Returns the difference of this value and `x`. */ def -(x: Float): Float = value - x /** Returns the difference of this value and `x`. */ def -(x: Double): Double = value - x /** Returns the product of this value and `x`. */ def *(x: Byte): Long = value * x /** Returns the product of this value and `x`. */ def *(x: Short): Long = value * x /** Returns the product of this value and `x`. */ def *(x: Char): Long = value * x /** Returns the product of this value and `x`. */ def *(x: Int): Long = value * x /** Returns the product of this value and `x`. */ def *(x: Long): Long = value * x /** Returns the product of this value and `x`. */ def *(x: Float): Float = value * x /** Returns the product of this value and `x`. */ def *(x: Double): Double = value * x /** Returns the quotient of this value and `x`. */ def /(x: Byte): Long = value / x /** Returns the quotient of this value and `x`. */ def /(x: Short): Long = value / x /** Returns the quotient of this value and `x`. */ def /(x: Char): Long = value / x /** Returns the quotient of this value and `x`. */ def /(x: Int): Long = value / x /** Returns the quotient of this value and `x`. */ def /(x: Long): Long = value / x /** Returns the quotient of this value and `x`. */ def /(x: Float): Float = value / x /** Returns the quotient of this value and `x`. */ def /(x: Double): Double = value / x /** Returns the remainder of the division of this value by `x`. */ def %(x: Byte): Long = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Short): Long = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Char): Long = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Int): Long = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Long): Long = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Float): Float = value % x /** Returns the remainder of the division of this value by `x`. */ def %(x: Double): Double = value % x // Stuff from RichLong: /** * Returns a string representation of thisPosZLong
's underlyingLong
* as an unsigned integer in base 2. * ** The unsigned
* * @return the string representation of the unsignedlong
value is thisPosZLong
's underlyingLong
plus * 264 if the underlyingLong
is negative; otherwise, it is * equal to the underlyingLong
. This value is converted to a string of * ASCII digits in binary (base 2) with no extra leading *0
s. If the unsigned magnitude is zero, it is * represented by a single zero character'0'
* ('\u0030'
); otherwise, the first character of * the representation of the unsigned magnitude will not be the * zero character. The characters'0'
* ('\u0030'
) and'1'
* ('\u0031'
) are used as binary digits. *long
* value represented by thisPosZLong
's underlyingLong
in binary (base 2). */ def toBinaryString: String = java.lang.Long.toBinaryString(value) /** * Returns a string representation of thisPosZLong
's underlyingLong
* as an unsigned integer in base 16. * ** The unsigned
* *long
value is thisPosZLong
's underlyingLong
plus * 264 if the underlyingLong
is negative; otherwise, it is * equal to the underlyingLong
. This value is converted to a string of * ASCII digits in hexadecimal (base 16) with no extra * leading0
s. If the unsigned magnitude is zero, it * is represented by a single zero character'0'
* ('\u0030'
); otherwise, the first character of * the representation of the unsigned magnitude will not be the * zero character. The following characters are used as * hexadecimal digits: *** *0123456789abcdef
** These are the characters
* * @return the string representation of the unsigned'\u0030'
through *'\u0039'
and'\u0061'
through *'\u0066'
. If uppercase letters are desired, * thetoUpperCase
method may be called * on the result. *long
* value represented by thisPosZLong
's underlyingLong
in hexadecimal * (base 16). */ def toHexString: String = java.lang.Long.toHexString(value) /** * Returns a string representation of thisPosZLong
's underlyingLong
* as an unsigned integer in base 8. * ** The unsigned
* *long
value is thisPosZLong
's underlyingLong
plus * 264 if the underlyingLong
is negative; otherwise, it is * equal to the underlyingLong
. This value is converted to a string of * ASCII digits in octal (base 8) with no extra leading *0
s. ** If the unsigned magnitude is zero, it is represented by a * single zero character
* *'0'
* ('\u0030'
); otherwise, the first character of * the representation of the unsigned magnitude will not be the * zero character. The following characters are used as octal * digits: *** *01234567
** These are the characters
* * @return the string representation of the unsigned'\u0030'
through *'\u0037'
. *long
* value represented by thisPosZLong
's underlyingLong
in octal (base 8). */ def toOctalString: String = java.lang.Long.toOctalString(value) /** * Returnsthis
ifthis > that
orthat
otherwise. */ def max(that: PosZLong): PosZLong = if (math.max(value, that.value) == value) this else that /** * Returnsthis
ifthis < that
orthat
otherwise. */ def min(that: PosZLong): PosZLong = if (math.min(value, that.value) == value) this else that // adapted from RichInt: /** * Create aRange
from thisPosZLong
value * until the specifiedend
(exclusive) with step value 1. * * @param end The final bound of the range to make. * @return A [[scala.collection.immutable.NumericRange.Exclusive[Long]]] from `this` up to but * not including `end`. */ def until(end: Long): NumericRange.Exclusive[Long] = value.until(end) /** * Create aRange
from thisPosZLong
value * until the specifiedend
(exclusive) with the specifiedstep
value. * * @param end The final bound of the range to make. * @param end The final bound of the range to make. * @param step The number to increase by for each step of the range. * @return A [[scala.collection.immutable.NumericRange.Exclusive[Long]]] from `this` up to but * not including `end`. */ def until(end: Long, step: Long): NumericRange.Exclusive[Long] = value.until(end, step) /** * Create an inclusiveRange
from thisPosZLong
value * to the specifiedend
with step value 1. * * @param end The final bound of the range to make. * @return A [[scala.collection.immutable.NumericRange.Inclusive[Long]]] from `'''this'''` up to * and including `end`. */ def to(end: Long): NumericRange.Inclusive[Long] = value.to(end) /** * Create an inclusiveRange
from thisPosZLong
value * to the specifiedend
with the specifiedstep
value. * * @param end The final bound of the range to make. * @param step The number to increase by for each step of the range. * @return A [[scala.collection.immutable.NumericRange.Inclusive[Long]]] from `'''this'''` up to * and including `end`. */ def to(end: Long, step: Long): NumericRange.Inclusive[Long] = value.to(end, step) /** * Applies the passedLong => Long
function to the underlyingLong
* value, and if the result is positive, returns the result wrapped in aPosZLong
, * else throwsAssertionError
. * ** This method will inspect the result of applying the given function to this *
* *PosZLong
's underlyingLong
value and if the result * is non-negative, it will return aPosZLong
representing that value. * Otherwise, theLong
value returned by the given function is * not non-negative, this method will throwAssertionError
. ** This method differs from a vanilla
* * @param f theassert
orensuring
* call in that you get something you didn't already have if the assertion * succeeds: a type that promises anLong
is non-negative. * With this method, you are asserting that you are convinced the result of * the computation represented by applying the given function to thisPosZLong
's * value will not overflow. Instead of overflowing silently likeLong
, this * method will signal an overflow with a loudAssertionError
. *Long => Long
function to apply to thisPosZLong
's * underlyingLong
value. * @return the result of applying thisPosZLong
's underlyingLong
value to * to the passed function, wrapped in aPosZLong
if it is non-negative (else throwsAssertionError
). * @throws AssertionError if the result of applying thisPosZLong
's underlyingLong
value to * to the passed function is not positive. */ def ensuringValid(f: Long => Long): PosZLong = { val candidateResult: Long = f(value) if (PosZLongMacro.isValid(candidateResult)) new PosZLong(candidateResult) else throw new AssertionError(s"${candidateResult.toString()}, the result of applying the passed function to ${value.toString()}, was not a valid PosZLong") } } /** * The companion object forPosZLong
that offers * factory methods that producePosZLong
s, implicit * widening conversions fromPosZLong
to other * numeric types, and maximum and minimum constant values for *PosZLong
. */ object PosZLong { /** * The largest value representable as a non-negative *Long
, which isPosZLong(9223372036854775807)
. */ final val MaxValue: PosZLong = PosZLong.ensuringValid(Long.MaxValue) /** * The smallest value representable as a positive *Long
, which isPosZLong(0L)
. */ final val MinValue: PosZLong = PosZLong.ensuringValid(0L) // Can't use the macro here /** * A factory method that produces anOption[PosZLong]
given a *Long
value. * ** This method will inspect the passed
* *Long
value and if * it is a non-negativeLong
, it will return aPosZLong
representing that value, * wrapped in aSome
. Otherwise, the passedLong
* value is not non-negative, so this method will returnNone
. ** This factory method differs from the
* * @param value theapply
* factory method in thatapply
is implemented * via a macro that inspectsLong
literals at * compile time, whereasfrom
inspects *Long
values at run time. *Long
to inspect, and if non-negative, return * wrapped in aSome[PosZLong]
. * @return the specifiedLong
value wrapped in a *Some[PosZLong]
, if it is non-negative, else *None
. */ def from(value: Long): Option[PosZLong] = if (PosZLongMacro.isValid(value)) Some(new PosZLong(value)) else None /** * A factory/assertion method that produces anPosZLong
given a * validLong
value, or throwsAssertionError
, * if given an invalidLong
value. * * Note: you should use this method only when you are convinced that it will * always succeed, i.e., never throw an exception. It is good practice to * add a comment near the invocation of this method indicating ''why'' you think * it will always succeed to document your reasoning. If you are not sure an * `ensuringValid` call will always succeed, you should use one of the other * factory or validation methods provided on this object instead: `isValid`, * `tryingValid`, `passOrElse`, `goodOrElse`, or `rightOrElse`. * ** This method will inspect the passed
* *Long
value and if * it is a non-negativeLong
, it will return aPosZLong
representing that value. * Otherwise, the passedLong
value is not non-negative, so * this method will throwAssertionError
. ** This factory method differs from the
* * @param value theapply
* factory method in thatapply
is implemented * via a macro that inspectsLong
literals at * compile time, whereasfrom
inspects *Long
values at run time. * It differs from a vanillaassert
orensuring
* call in that you get something you didn't already have if the assertion * succeeds: a type that promises aLong
is positive. *Long
to inspect, and if non-negative, return * wrapped in aPosZLong
. * @return the specifiedLong
value wrapped in a *PosZLong
, if it is non-negative, else * throwsAssertionError
. * @throws AssertionError if the passed value is not non-negative */ def ensuringValid(value: Long): PosZLong = if (PosZLongMacro.isValid(value)) new PosZLong(value) else { throw new AssertionError(s"${value.toString()} was not a valid PosZLong") } /** * A factory/validation method that produces aPosZLong
, wrapped * in aSuccess
, given a validLong
value, or if the * givenLong
is invalid, anAssertionError
, wrapped * in aFailure
. * ** This method will inspect the passed
* *Long
value and if * it is a non-negativeLong
, it will return aPosZLong
* representing that value, wrapped in aSuccess
. * Otherwise, the passedLong
value is not non-negative, so this * method will return anAssertionError
, wrapped in aFailure
. ** This factory method differs from the
* * @param value theapply
factory method * in thatapply
is implemented via a macro that inspects *Long
literals at compile time, whereas this method inspects *Long
values at run time. *Long
to inspect, and if non-negative, return * wrapped in aSuccess(PosZLong)
. * @return the specifiedLong
value wrapped * in aSuccess(PosZLong)
, if it is non-negative, else aFailure(AssertionError)
. */ def tryingValid(value: Long): Try[PosZLong] = if (PosZLongMacro.isValid(value)) Success(new PosZLong(value)) else Failure(new AssertionError(s"${value.toString()} was not a valid PosZLong")) /** * A validation method that produces aPass
* given a validLong
value, or * an error value of typeE
produced by passing the * given invalidInt
value * to the given functionf
, wrapped in aFail
. * ** This method will inspect the passed
* *Long
value and if * it is a non-negativeLong
, it will return aPass
. * Otherwise, the passedLong
value is non-negative, so this * method will return a result of typeE
obtained by passing * the invalidLong
value to the given functionf
, * wrapped in a `Fail`. ** This factory method differs from the
* * @param value the `Long` to validate that it is non-negative. * @return a `Pass` if the specified `Long` value is non-negative, * else a `Fail` containing an error value produced by passing the * specified `Long` to the given function `f`. */ def passOrElse[E](value: Long)(f: Long => E): Validation[E] = if (PosZLongMacro.isValid(value)) Pass else Fail(f(value)) /** * A factory/validation method that produces aapply
factory method * in thatapply
is implemented via a macro that inspects *Long
literals at compile time, whereas this method inspects *Long
values at run time. *PosZLong
, wrapped * in aGood
, given a validLong
value, or if the * givenLong
is invalid, an error value of typeB
* produced by passing the given invalidLong
value * to the given functionf
, wrapped in aBad
. * ** This method will inspect the passed
* *Long
value and if * it is a non-negativeLong
, it will return aPosZLong
* representing that value, wrapped in aGood
. * Otherwise, the passedLong
value is not non-negative, so this * method will return a result of typeB
obtained by passing * the invalidLong
value to the given functionf
, * wrapped in a `Bad`. ** This factory method differs from the
* * @param value theapply
factory method * in thatapply
is implemented via a macro that inspects *Long
literals at compile time, whereas this method inspects *Long
values at run time. *Long
to inspect, and if non-negative, return * wrapped in aGood(PosZLong)
. * @return the specifiedLong
value wrapped * in aGood(PosZLong)
, if it is non-negative, else aBad(f(value))
. */ def goodOrElse[B](value: Long)(f: Long => B): PosZLong Or B = if (PosZLongMacro.isValid(value)) Good(PosZLong.ensuringValid(value)) else Bad(f(value)) /** * A factory/validation method that produces aPosZLong
, wrapped * in aRight
, given a validInt
value, or if the * givenInt
is invalid, an error value of typeL
* produced by passing the given invalidInt
value * to the given functionf
, wrapped in aLeft
. * ** This method will inspect the passed
* *Int
value and if * it is a non-negativeInt
, it will return aPosZLong
* representing that value, wrapped in aRight
. * Otherwise, the passedInt
value is not non-negative, so this * method will return a result of typeL
obtained by passing * the invalidInt
value to the given functionf
, * wrapped in a `Left`. ** This factory method differs from the
* * @param value theapply
factory method * in thatapply
is implemented via a macro that inspects *Int
literals at compile time, whereas this method inspects *Int
values at run time. *Int
to inspect, and if non-negative, return * wrapped in aRight(PosZLong)
. * @return the specifiedInt
value wrapped * in aRight(PosZLong)
, if it is non-negative, else aLeft(f(value))
. */ def rightOrElse[L](value: Long)(f: Long => L): Either[L, PosZLong] = if (PosZLongMacro.isValid(value)) Right(PosZLong.ensuringValid(value)) else Left(f(value)) /** * A predicate method that returns true if a given *Long
value is non-negative. * * @param value theLong
to inspect, and if non-negative, return true. * @return true if the specifiedLong
is non-negative, else false. */ def isValid(value: Long): Boolean = PosZLongMacro.isValid(value) /** * A factory method that produces aPosZLong
given a *Long
value and a defaultPosZLong
. * ** This method will inspect the passed
* *Long
value and if * it is a non-negativeLong
, it will return aPosZLong
representing that value. * Otherwise, the passedLong
value is not non-negative, so this * method will return the passeddefault
value. ** This factory method differs from the
* * @param value theapply
* factory method in thatapply
is implemented * via a macro that inspectsLong
literals at * compile time, whereasfrom
inspects *Long
values at run time. *Long
to inspect, and if non-negative, return. * @param default thePosZLong
to return if the passed *Long
value is not non-negative. * @return the specifiedLong
value wrapped in a *PosZLong
, if it is non-negative, else the *default
PosZLong
value. */ def fromOrElse(value: Long, default: => PosZLong): PosZLong = if (PosZLongMacro.isValid(value)) new PosZLong(value) else default import language.experimental.macros /** * A factory method, implemented via a macro, that produces a *PosZLong
if passed a validLong
* literal, otherwise a compile time error. * ** The macro that implements this method will inspect the * specified
* *Long
expression at compile time. If * the expression is a non-negativeLong
literal, * it will return aPosZLong
representing that value. * Otherwise, the passedLong
expression is either a literal * that is not non-negative, or is not a literal, so this method * will give a compiler error. ** This factory method differs from the
* * @param value thefrom
* factory method in that this method is implemented via a * macro that inspectsLong
literals at compile * time, whereasfrom
inspectsLong
* values at run time. *Long
literal expression to * inspect at compile time, and if non-negative, to return * wrapped in aPosZLong
at run time. * @return the specified, validLong
literal * value wrapped in aPosZLong
. (If the * specified expression is not a validLong
* literal, the invocation of this method will not * compile.) */ inline implicit def apply(value: => Long): PosZLong = ${ PosZLongMacro('{value}) } /** * Implicit widening conversion fromPosZLong
to *Long
. * * @param pos thePosZLong
to widen * @return theLong
value underlying the specified *PosZLong
. */ implicit def widenToLong(pos: PosZLong): Long = pos.value /** * Implicit widening conversion fromPosZLong
to *Float
. * * @param pos thePosZLong
to widen * @return theLong
value underlying the specified *PosZLong
, widened toFloat
. */ implicit def widenToFloat(pos: PosZLong): Float = pos.value /** * Implicit widening conversion fromPosZLong
to *Double
. * * @param pos thePosZLong
to widen * @return theLong
value underlying the specified *PosZLong
, widened toDouble
. */ implicit def widenToDouble(pos: PosZLong): Double = pos.value /** * Implicit widening conversion fromPosZLong
toPosZFloat
. * * @param pos thePosZLong
to widen * @return theLong
value underlying the specifiedPosZLong
, * widened toFloat
and wrapped in aPosZFloat
. */ implicit def widenToPosZFloat(pos: PosZLong): PosZFloat = PosZFloat.ensuringValid(pos.value) /** * Implicit widening conversion fromPosZLong
toPosZDouble
. * * @param pos thePosZLong
to widen * @return theLong
value underlying the specifiedPosZLong
, * widened toDouble
and wrapped in aPosZDouble
. */ implicit def widenToPosZDouble(pos: PosZLong): PosZDouble = PosZDouble.ensuringValid(pos.value) /** * Implicit Ordering instance. */ implicit val ordering: Ordering[PosZLong] = new Ordering[PosZLong] { def compare(x: PosZLong, y: PosZLong): Int = x.toLong.compare(y) } /** * The formerly implicitposZLongOrd
field has been deprecated and will be removed in a future version of ScalaTest. Please use theordering
field instead. */ @deprecated("The formerly implicit posZLongOrd field has been deprecated and will be removed in a future version of ScalaTest. Please use the ordering field instead.") val posZLongOrd: Ordering[PosZLong] = new Ordering[PosZLong] { def compare(x: PosZLong, y: PosZLong): Int = ordering.compare(x, y) } }