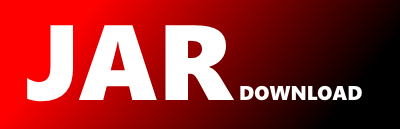
org.scalatest.matchers.dsl.MatcherFactory2.scala Maven / Gradle / Ivy
The newest version!
package org.scalatest.matchers.dsl
import org.scalatest.enablers._
import org.scalatest.matchers.MatchersHelper.andMatchersAndApply
import org.scalatest.matchers.MatchersHelper.orMatchersAndApply
import org.scalatest.matchers.dsl.MatcherWords
import scala.collection.GenTraversable
import scala.util.matching.Regex
import org.scalactic._
import TripleEqualsSupport.Spread
import TripleEqualsSupport.TripleEqualsInvocation
import org.scalatest.FailureMessages
import org.scalatest.Resources
import org.scalatest.matchers.Matcher
import org.scalatest.matchers.MatchResult
import org.scalatest.matchers.BeMatcher
import org.scalatest.matchers.BePropertyMatcher
import org.scalatest.matchers.HavePropertyMatcher
import org.scalatest.matchers.AMatcher
import org.scalatest.matchers.AnMatcher
import org.scalatest.matchers.MatchPatternMacro
import org.scalatest.matchers.TypeMatcherMacro
import org.scalatest.matchers.MatchPatternHelper
import org.scalatest.matchers.dsl.FullyMatchWord
import org.scalatest.matchers.dsl.StartWithWord
import org.scalatest.matchers.dsl.EndWithWord
import org.scalatest.matchers.dsl.IncludeWord
import org.scalatest.matchers.dsl.HaveWord
import org.scalatest.matchers.dsl.BeWord
import org.scalatest.matchers.dsl.NotWord
import org.scalatest.matchers.dsl.ContainWord
import org.scalatest.matchers.dsl.ResultOfLengthWordApplication
import org.scalatest.matchers.dsl.ResultOfSizeWordApplication
import org.scalatest.matchers.dsl.ResultOfMessageWordApplication
import org.scalatest.matchers.dsl.ResultOfLessThanComparison
import org.scalatest.matchers.dsl.ResultOfGreaterThanComparison
import org.scalatest.matchers.dsl.ResultOfLessThanOrEqualToComparison
import org.scalatest.matchers.dsl.ResultOfGreaterThanOrEqualToComparison
import org.scalatest.matchers.dsl.ResultOfAWordToSymbolApplication
import org.scalatest.matchers.dsl.ResultOfAWordToBePropertyMatcherApplication
import org.scalatest.matchers.dsl.ResultOfAWordToAMatcherApplication
import org.scalatest.matchers.dsl.ResultOfAnWordToSymbolApplication
import org.scalatest.matchers.dsl.ResultOfAnWordToBePropertyMatcherApplication
import org.scalatest.matchers.dsl.ResultOfAnWordToAnMatcherApplication
import org.scalatest.matchers.dsl.ResultOfTheSameInstanceAsApplication
import org.scalatest.matchers.dsl.ResultOfRegexWordApplication
import org.scalatest.matchers.dsl.ResultOfKeyWordApplication
import org.scalatest.matchers.dsl.ResultOfValueWordApplication
import org.scalatest.matchers.dsl.RegexWithGroups
import org.scalatest.matchers.dsl.ResultOfDefinedAt
import org.scalatest.matchers.dsl.ResultOfOneOfApplication
import org.scalatest.matchers.dsl.ResultOfOneElementOfApplication
import org.scalatest.matchers.dsl.ResultOfAtLeastOneOfApplication
import org.scalatest.matchers.dsl.ResultOfAtLeastOneElementOfApplication
import org.scalatest.matchers.dsl.ResultOfNoneOfApplication
import org.scalatest.matchers.dsl.ResultOfNoElementsOfApplication
import org.scalatest.matchers.dsl.ResultOfTheSameElementsAsApplication
import org.scalatest.matchers.dsl.ResultOfTheSameElementsInOrderAsApplication
import org.scalatest.matchers.dsl.ResultOfOnlyApplication
import org.scalatest.matchers.dsl.ResultOfAllOfApplication
import org.scalatest.matchers.dsl.ResultOfAllElementsOfApplication
import org.scalatest.matchers.dsl.ResultOfInOrderOnlyApplication
import org.scalatest.matchers.dsl.ResultOfInOrderApplication
import org.scalatest.matchers.dsl.ResultOfInOrderElementsOfApplication
import org.scalatest.matchers.dsl.ResultOfAtMostOneOfApplication
import org.scalatest.matchers.dsl.ResultOfAtMostOneElementOfApplication
import org.scalatest.matchers.dsl.SortedWord
import org.scalatest.matchers.dsl.ResultOfATypeInvocation
import org.scalatest.matchers.dsl.ResultOfAnTypeInvocation
import org.scalatest.matchers.dsl.ExistWord
import org.scalatest.matchers.dsl.ResultOfNotExist
import org.scalatest.matchers.dsl.ReadableWord
import org.scalatest.matchers.dsl.WritableWord
import org.scalatest.matchers.dsl.EmptyWord
import org.scalatest.matchers.dsl.DefinedWord
import scala.language.higherKinds
/**
* A matcher factory that can produce a matcher given two typeclass instances.
*
*
* In the type parameters for this class, "SC
" means superclass; "TC
"
* (in TC1
, TC2
, etc.) means typeclass.
* This class's matcher
factory method will produce a Matcher[T]
, where T
is a subtype of (or the same type
* as) SC
, given a typeclass instance for each TCn
* implicit parameter (for example, a TC1[T]
, TC2[T]
, etc.).
*
*
* @author Bill Venners
*/
// Add a TYPECLASSN for each N
abstract class MatcherFactory2[-SC, TC1[_], TC2[_]] { thisMatcherFactory =>
/**
* Factory method that will produce a Matcher[T]
, where T
is a subtype of (or the same type
* as) SC
, given a typeclass instance for each TCn
* implicit parameter (for example, a TC1[T]
, TC2[T]
, etc.).
*/
def matcher[T <: SC : TC1 : TC2]: Matcher[T]
/**
* Ands this matcher factory with the passed matcher.
*/
infix def and[U <: SC](rightMatcher: Matcher[U]): MatcherFactory2[U, TC1, TC2] =
new MatcherFactory2[U, TC1, TC2] {
def matcher[V <: U : TC1 : TC2]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
andMatchersAndApply(left, leftMatcher, rightMatcher)
}
override def toString: String = "(" + Prettifier.default(thisMatcherFactory) + ") and (" + Prettifier.default(rightMatcher) + ")"
}
}
override def toString: String = "(" + Prettifier.default(thisMatcherFactory) + ") and (" + Prettifier.default(rightMatcher) + ")"
}
/**
* Ors this matcher factory with the passed matcher.
*/
infix def or[U <: SC](rightMatcher: Matcher[U]): MatcherFactory2[U, TC1, TC2] =
new MatcherFactory2[U, TC1, TC2] {
def matcher[V <: U : TC1 : TC2]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
orMatchersAndApply(left, leftMatcher, rightMatcher)
}
override def toString: String = "(" + Prettifier.default(thisMatcherFactory) + ") or (" + Prettifier.default(rightMatcher) + ")"
}
}
override def toString: String = "(" + Prettifier.default(thisMatcherFactory) + ") or (" + Prettifier.default(rightMatcher) + ")"
}
/**
* Ands this matcher factory with the passed MatcherFactory1
that has the same final typeclass as this one.
*/
infix def and[U <: SC](rightMatcherFactory: MatcherFactory1[U, TC2]): MatcherFactory2[U, TC1, TC2] =
new MatcherFactory2[U, TC1, TC2] {
def matcher[V <: U : TC1 : TC2]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
andMatchersAndApply(left, leftMatcher, rightMatcher)
}
override def toString: String = "(" + Prettifier.default(thisMatcherFactory) + ") and (" + Prettifier.default(rightMatcherFactory) + ")"
}
}
override def toString: String = "(" + Prettifier.default(thisMatcherFactory) + ") and (" + Prettifier.default(rightMatcherFactory) + ")"
}
/**
* Ors this matcher factory with the passed MatcherFactory1
that has the same final typeclass as this one.
*/
infix def or[U <: SC](rightMatcherFactory: MatcherFactory1[U, TC2]): MatcherFactory2[U, TC1, TC2] =
new MatcherFactory2[U, TC1, TC2] {
def matcher[V <: U : TC1 : TC2]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
orMatchersAndApply(left, leftMatcher, rightMatcher)
}
override def toString: String = "(" + Prettifier.default(thisMatcherFactory) + ") or (" + Prettifier.default(rightMatcherFactory) + ")"
}
}
override def toString: String = "(" + Prettifier.default(thisMatcherFactory) + ") or (" + Prettifier.default(rightMatcherFactory) + ")"
}
/**
* Ands this matcher factory with the passed matcher factory.
*/
infix def and[U <: SC, TC3[_]](rightMatcherFactory: MatcherFactory1[U, TC3]): MatcherFactory3[U, TC1, TC2, TC3] =
new MatcherFactory3[U, TC1, TC2, TC3] {
def matcher[V <: U : TC1 : TC2 : TC3]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
andMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ors this matcher factory with the passed matcher factory.
*/
infix def or[U <: SC, TC3[_]](rightMatcherFactory: MatcherFactory1[U, TC3]): MatcherFactory3[U, TC1, TC2, TC3] =
new MatcherFactory3[U, TC1, TC2, TC3] {
def matcher[V <: U : TC1 : TC2 : TC3]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
orMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ands this matcher factory with the passed matcher factory.
*/
infix def and[U <: SC, TC3[_], TC4[_]](rightMatcherFactory: MatcherFactory2[U, TC3, TC4]): MatcherFactory4[U, TC1, TC2, TC3, TC4] =
new MatcherFactory4[U, TC1, TC2, TC3, TC4] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
andMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ors this matcher factory with the passed matcher factory.
*/
infix def or[U <: SC, TC3[_], TC4[_]](rightMatcherFactory: MatcherFactory2[U, TC3, TC4]): MatcherFactory4[U, TC1, TC2, TC3, TC4] =
new MatcherFactory4[U, TC1, TC2, TC3, TC4] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
orMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ands this matcher factory with the passed matcher factory.
*/
infix def and[U <: SC, TC3[_], TC4[_], TC5[_]](rightMatcherFactory: MatcherFactory3[U, TC3, TC4, TC5]): MatcherFactory5[U, TC1, TC2, TC3, TC4, TC5] =
new MatcherFactory5[U, TC1, TC2, TC3, TC4, TC5] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
andMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ors this matcher factory with the passed matcher factory.
*/
infix def or[U <: SC, TC3[_], TC4[_], TC5[_]](rightMatcherFactory: MatcherFactory3[U, TC3, TC4, TC5]): MatcherFactory5[U, TC1, TC2, TC3, TC4, TC5] =
new MatcherFactory5[U, TC1, TC2, TC3, TC4, TC5] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
orMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ands this matcher factory with the passed matcher factory.
*/
infix def and[U <: SC, TC3[_], TC4[_], TC5[_], TC6[_]](rightMatcherFactory: MatcherFactory4[U, TC3, TC4, TC5, TC6]): MatcherFactory6[U, TC1, TC2, TC3, TC4, TC5, TC6] =
new MatcherFactory6[U, TC1, TC2, TC3, TC4, TC5, TC6] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5 : TC6]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
andMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ors this matcher factory with the passed matcher factory.
*/
infix def or[U <: SC, TC3[_], TC4[_], TC5[_], TC6[_]](rightMatcherFactory: MatcherFactory4[U, TC3, TC4, TC5, TC6]): MatcherFactory6[U, TC1, TC2, TC3, TC4, TC5, TC6] =
new MatcherFactory6[U, TC1, TC2, TC3, TC4, TC5, TC6] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5 : TC6]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
orMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ands this matcher factory with the passed matcher factory.
*/
infix def and[U <: SC, TC3[_], TC4[_], TC5[_], TC6[_], TC7[_]](rightMatcherFactory: MatcherFactory5[U, TC3, TC4, TC5, TC6, TC7]): MatcherFactory7[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7] =
new MatcherFactory7[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5 : TC6 : TC7]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
andMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ors this matcher factory with the passed matcher factory.
*/
infix def or[U <: SC, TC3[_], TC4[_], TC5[_], TC6[_], TC7[_]](rightMatcherFactory: MatcherFactory5[U, TC3, TC4, TC5, TC6, TC7]): MatcherFactory7[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7] =
new MatcherFactory7[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5 : TC6 : TC7]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
orMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ands this matcher factory with the passed matcher factory.
*/
infix def and[U <: SC, TC3[_], TC4[_], TC5[_], TC6[_], TC7[_], TC8[_]](rightMatcherFactory: MatcherFactory6[U, TC3, TC4, TC5, TC6, TC7, TC8]): MatcherFactory8[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7, TC8] =
new MatcherFactory8[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7, TC8] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5 : TC6 : TC7 : TC8]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
andMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ors this matcher factory with the passed matcher factory.
*/
infix def or[U <: SC, TC3[_], TC4[_], TC5[_], TC6[_], TC7[_], TC8[_]](rightMatcherFactory: MatcherFactory6[U, TC3, TC4, TC5, TC6, TC7, TC8]): MatcherFactory8[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7, TC8] =
new MatcherFactory8[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7, TC8] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5 : TC6 : TC7 : TC8]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
orMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ands this matcher factory with the passed matcher factory.
*/
infix def and[U <: SC, TC3[_], TC4[_], TC5[_], TC6[_], TC7[_], TC8[_], TC9[_]](rightMatcherFactory: MatcherFactory7[U, TC3, TC4, TC5, TC6, TC7, TC8, TC9]): MatcherFactory9[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7, TC8, TC9] =
new MatcherFactory9[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7, TC8, TC9] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5 : TC6 : TC7 : TC8 : TC9]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
andMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* Ors this matcher factory with the passed matcher factory.
*/
infix def or[U <: SC, TC3[_], TC4[_], TC5[_], TC6[_], TC7[_], TC8[_], TC9[_]](rightMatcherFactory: MatcherFactory7[U, TC3, TC4, TC5, TC6, TC7, TC8, TC9]): MatcherFactory9[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7, TC8, TC9] =
new MatcherFactory9[U, TC1, TC2, TC3, TC4, TC5, TC6, TC7, TC8, TC9] {
def matcher[V <: U : TC1 : TC2 : TC3 : TC4 : TC5 : TC6 : TC7 : TC8 : TC9]: Matcher[V] = {
new Matcher[V] {
def apply(left: V): MatchResult = {
val leftMatcher = thisMatcherFactory.matcher
val rightMatcher = rightMatcherFactory.matcher
orMatchersAndApply(left, leftMatcher, rightMatcher)
}
}
}
}
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class AndHaveWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and have length (3 - 1)
* ^
*
*/
infix def length(expectedLength: Long): MatcherFactory3[SC, TC1, TC2, Length] = and(MatcherWords.have.length(expectedLength))
// These guys need to generate a MatcherFactory of N+1. And it needs N-1 TC's, with the last one being Length.
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and have size (3 - 1)
* ^
*
*/
infix def size(expectedSize: Long): MatcherFactory3[SC, TC1, TC2, Size] = and(MatcherWords.have.size(expectedSize))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and have message ("A message from Mars!")
* ^
*
*/
infix def message(expectedMessage: String): MatcherFactory3[SC, TC1, TC2, Messaging] = and(MatcherWords.have.message(expectedMessage))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and have size (3 - 1)
* ^
*
*/
infix def and(haveWord: HaveWord): AndHaveWord = new AndHaveWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class AndContainWord(prettifier: Prettifier, pos: source.Position) {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain (3 - 1)
* ^
*
*/
def apply(expectedElement: Any): MatcherFactory3[SC, TC1, TC2, Containing] = thisMatcherFactory.and(MatcherWords.contain(expectedElement))
// And some, the ones that would by themselves already generate a Matcher, just return a MatcherFactoryN where N is the same.
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain key ("one")
* ^
*
*/
infix def key(expectedKey: Any): MatcherFactory3[SC, TC1, TC2, KeyMapping] = thisMatcherFactory.and(MatcherWords.contain.key(expectedKey))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain value (1)
* ^
*
*/
infix def value(expectedValue: Any): MatcherFactory3[SC, TC1, TC2, ValueMapping] = thisMatcherFactory.and(MatcherWords.contain.value(expectedValue))
// And some, the ones that would by themselves already generate a Matcher, just return a MatcherFactoryN where N is the same.
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain theSameElementsAs List(1, 2, 3)
* ^
*
*/
infix def theSameElementsAs(right: GenTraversable[_]): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.contain.theSameElementsAs(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain theSameElementsInOrderAs List(1, 2, 3)
* ^
*
*/
infix def theSameElementsInOrderAs(right: GenTraversable[_]): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.and(MatcherWords.contain.theSameElementsInOrderAs(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain inOrderOnly (1, 2, 3)
* ^
*
*/
infix def inOrderOnly(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.and(MatcherWords.contain.inOrderOnly(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain allOf (1, 2, 3)
* ^
*
*/
infix def allOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.contain.allOf(firstEle, secondEle, remainingEles .toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain allElementsOf List(1, 2, 3)
* ^
*
*/
infix def allElementsOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.contain.allElementsOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain inOrder (1, 2, 3)
* ^
*
*/
infix def inOrder(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.and(MatcherWords.contain.inOrder(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain inOrderElementsOf List(1, 2, 3)
* ^
*
*/
infix def inOrderElementsOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.and(MatcherWords.contain.inOrderElementsOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain oneOf (1, 2, 3)
* ^
*
*/
infix def oneOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.and(MatcherWords.contain.oneOf(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain oneElementOf (1, 2, 3)
* ^
*
*/
infix def oneElementOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.and(MatcherWords.contain.oneElementOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain atLeastOneOf (1, 2, 3)
* ^
*
*/
infix def atLeastOneOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.contain.atLeastOneOf(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain atLeastOneElementOf (1, 2, 3)
* ^
*
*/
infix def atLeastOneElementOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.contain.atLeastOneElementOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain only (1, 2, 3)
* ^
*
*/
infix def only(right: Any*): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.contain.only(right.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain noneOf (1, 2, 3)
* ^
*
*/
infix def noneOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.and(MatcherWords.contain.noneOf(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain noElementsOf (1, 2, 3)
* ^
*
*/
infix def noElementsOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.and(MatcherWords.contain.noElementsOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain atMostOneOf (1, 2, 3)
* ^
*
*/
infix def atMostOneOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.contain.atMostOneOf(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain atMostOneOf (1, 2, 3)
* ^
*
*/
infix def atMostOneElementOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.contain.atMostOneElementOf(elements))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and contain key ("one")
* ^
*
*/
infix def and(containWord: ContainWord)(implicit prettifier: Prettifier, pos: source.Position): AndContainWord = new AndContainWord(prettifier, pos)
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class AndBeWord {
()
()
/**
* This method enables the following syntax given a MatcherFactory2
, where file
is a BePropertyMatcher
:
*
*
* aMatcherFactory and be a (file)
* ^
*
*/
infix def a[U](bePropertyMatcher: BePropertyMatcher[U]): MatcherFactory2[SC with AnyRef with U, TC1, TC2] = and(MatcherWords.be.a(bePropertyMatcher))
/**
* This method enables the following syntax given a MatcherFactory2
, where validNumber
is an AMatcher
:
*
*
* aMatcherFactory and be a (validNumber)
* ^
*
*/
infix def a[U](aMatcher: AMatcher[U]): MatcherFactory2[SC with U, TC1, TC2] = and(MatcherWords.be.a(aMatcher))
()
()
/**
* This method enables the following syntax given a MatcherFactory2
, where apple
is a BePropertyMatcher
:
*
*
* aMatcherFactory and be an (apple)
* ^
*
*/
infix def an[U](bePropertyMatcher: BePropertyMatcher[U]): MatcherFactory2[SC with AnyRef with U, TC1, TC2] = and(MatcherWords.be.an(bePropertyMatcher))
/**
* This method enables the following syntax given a MatcherFactory2
, where integerNumber
is an AnMatcher
:
*
*
* aMatcherFactory and be an (integerNumber)
* ^
*
*/
infix def an[U](anMatcher: AnMatcher[U]): MatcherFactory2[SC with U, TC1, TC2] = and(MatcherWords.be.an(anMatcher))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and be theSameInstanceAs (string)
* ^
*
*/
infix def theSameInstanceAs(anyRef: AnyRef): MatcherFactory2[SC with AnyRef, TC1, TC2] = and(MatcherWords.be.theSameInstanceAs(anyRef))
/**
* This method enables the following syntax, where fraction
refers to a PartialFunction
:
*
*
* aMatcherFactory and be definedAt (8)
* ^
*
*/
infix def definedAt[A, U <: PartialFunction[A, _]](right: A): MatcherFactory2[SC with U, TC1, TC2] = and(MatcherWords.be.definedAt(right))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and be a ('file)
* ^
*
*/
infix def and(beWord: BeWord): AndBeWord = new AndBeWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class AndFullyMatchWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and fullyMatch regex (decimal)
* ^
*
*/
infix def regex(regexString: String): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.fullyMatch.regex(regexString))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and fullyMatch regex (("a(b*)c" withGroup "bb"))
* ^
*
*/
infix def regex(regexWithGroups: RegexWithGroups): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.fullyMatch.regex(regexWithGroups))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and fullyMatch regex (decimalRegex)
* ^
*
*/
infix def regex(regex: Regex): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.fullyMatch.regex(regex))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and fullyMatch regex (decimalRegex)
* ^
*
*/
infix def and(fullyMatchWord: FullyMatchWord): AndFullyMatchWord = new AndFullyMatchWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class AndIncludeWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and include regex (decimal)
* ^
*
*/
infix def regex(regexString: String): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.include.regex(regexString))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and include regex ("a(b*)c" withGroup "bb")
* ^
*
*/
infix def regex(regexWithGroups: RegexWithGroups): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.include.regex(regexWithGroups))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and include regex (decimalRegex)
* ^
*
*/
infix def regex(regex: Regex): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.include.regex(regex))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and include regex ("wor.d")
* ^
*
*/
infix def and(includeWord: IncludeWord): AndIncludeWord = new AndIncludeWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class AndStartWithWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and startWith regex (decimal)
* ^
*
*/
infix def regex(regexString: String): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.startWith.regex(regexString))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and startWith regex ("a(b*)c" withGroup "bb")
* ^
*
*/
infix def regex(regexWithGroups: RegexWithGroups): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.startWith.regex(regexWithGroups))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and startWith regex (decimalRegex)
* ^
*
*/
infix def regex(regex: Regex): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.startWith.regex(regex))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and startWith regex ("1.7")
* ^
*
*/
infix def and(startWithWord: StartWithWord): AndStartWithWord = new AndStartWithWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class AndEndWithWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and endWith regex (decimal)
* ^
*
*/
infix def regex(regexString: String): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.endWith.regex(regexString))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and endWith regex ("a(b*)c" withGroup "bb")
* ^
*
*/
infix def regex(regexWithGroups: RegexWithGroups): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.endWith.regex(regexWithGroups))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and endWith regex (decimalRegex)
* ^
*
*/
infix def regex(regex: Regex): MatcherFactory2[SC with String, TC1, TC2] = and(MatcherWords.endWith.regex(regex))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and endWith regex (decimalRegex)
* ^
*
*/
infix def and(endWithWord: EndWithWord): AndEndWithWord = new AndEndWithWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class AndNotWord(prettifier: Prettifier, pos: source.Position) { thisAndNotWord =>
/**
* Get the MatcherFactory
instance, currently used by macro only.
*/
val owner = thisMatcherFactory
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not equal (3 - 1)
* ^
*
*/
infix def equal(any: Any): MatcherFactory3[SC, TC1, TC2, Equality] =
thisMatcherFactory.and(MatcherWords.not.apply(MatcherWords.equal(any)))
/**
* This method enables the following syntax, for the "primitive" numeric types:
*
*
* aMatcherFactory and not equal (17.0 +- 0.2)
* ^
*
*/
infix def equal[U](spread: Spread[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.and(MatcherWords.not.equal(spread))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not equal (null)
* ^
*
*/
infix def equal(o: Null): MatcherFactory2[SC, TC1, TC2] = {
thisMatcherFactory and {
new Matcher[SC] {
def apply(left: SC): MatchResult = {
MatchResult(
left != null,
Resources.rawEqualedNull,
Resources.rawDidNotEqualNull,
Resources.rawMidSentenceEqualedNull,
Resources.rawDidNotEqualNull,
Vector.empty,
Vector(left)
)
}
override def toString: String = "not equal null"
}
}
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not be (3 - 1)
* ^
*
*/
infix def be(any: Any): MatcherFactory2[SC, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.apply(MatcherWords.be(any)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not have length (3)
* ^
*
*/
infix def have(resultOfLengthWordApplication: ResultOfLengthWordApplication): MatcherFactory3[SC, TC1, TC2, Length] =
thisMatcherFactory.and(MatcherWords.not.apply(MatcherWords.have.length(resultOfLengthWordApplication.expectedLength)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not have size (3)
* ^
*
*/
infix def have(resultOfSizeWordApplication: ResultOfSizeWordApplication): MatcherFactory3[SC, TC1, TC2, Size] =
thisMatcherFactory.and(MatcherWords.not.apply(MatcherWords.have.size(resultOfSizeWordApplication.expectedSize)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not have message ("Message from Mars!")
* ^
*
*/
infix def have(resultOfMessageWordApplication: ResultOfMessageWordApplication): MatcherFactory3[SC, TC1, TC2, Messaging] =
thisMatcherFactory.and(MatcherWords.not.apply(MatcherWords.have.message(resultOfMessageWordApplication.expectedMessage)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not have (author ("Melville"))
* ^
*
*/
infix def have[U](firstPropertyMatcher: HavePropertyMatcher[U, _], propertyMatchers: HavePropertyMatcher[U, _]*): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.apply(MatcherWords.have(firstPropertyMatcher, propertyMatchers: _*)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not be < (6)
* ^
*
*/
infix def be[U](resultOfLessThanComparison: ResultOfLessThanComparison[U]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.be(resultOfLessThanComparison))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not be (null)
* ^
*
*/
infix def be(o: Null): MatcherFactory2[SC with AnyRef, TC1, TC2] = thisMatcherFactory.and(MatcherWords.not.be(o))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory (8) and not be > (6)
* ^
*
*/
infix def be[U](resultOfGreaterThanComparison: ResultOfGreaterThanComparison[U]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.be(resultOfGreaterThanComparison))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not be <= (2)
* ^
*
*/
infix def be[U](resultOfLessThanOrEqualToComparison: ResultOfLessThanOrEqualToComparison[U]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.be(resultOfLessThanOrEqualToComparison))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not be >= (6)
* ^
*
*/
infix def be[U](resultOfGreaterThanOrEqualToComparison: ResultOfGreaterThanOrEqualToComparison[U]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.be(resultOfGreaterThanOrEqualToComparison))
/**
*
* The deprecation period for the should be === syntax has expired, and the syntax may no longer be
* used. Please use should equal, should ===, shouldEqual,
* should be, or shouldBe instead.
*
*
*
* Note: usually syntax will be removed after its deprecation period. This was left in because otherwise the syntax could in some
* cases still compile, but silently wouldn't work.
*
*/
infix def be(tripleEqualsInvocation: TripleEqualsInvocation[_]): MatcherFactory2[SC, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.be(tripleEqualsInvocation)(pos))
()
()
/**
* This method enables the following syntax given a MatcherFactory2
, where odd
is a BeMatcher
:
*
*
* aMatcherFactory and not be (odd)
* ^
*
*/
infix def be[U](beMatcher: BeMatcher[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.and(MatcherWords.not.be(beMatcher))
/**
* This method enables the following syntax given a MatcherFactory2
, where directory
is a BePropertyMatcher
:
*
*
* aMatcherFactory and not be (directory)
* ^
*
*/
infix def be[U](bePropertyMatcher: BePropertyMatcher[U]): MatcherFactory2[SC with AnyRef with U, TC1, TC2] = thisMatcherFactory.and(MatcherWords.not.be(bePropertyMatcher))
()
()
/**
* This method enables the following syntax given a MatcherFactory2
, where validMarks
is an AMatcher
:
*
*
* aMatcherFactory and not be a (validMarks)
* ^
*
*/
infix def be[U](resultOfAWordApplication: ResultOfAWordToAMatcherApplication[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.and(MatcherWords.not.be(resultOfAWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
, where directory
is a BePropertyMatcher
:
*
*
* aMatcherFactory and not be a (directory)
* ^
*
*/
infix def be[U <: AnyRef](resultOfAWordApplication: ResultOfAWordToBePropertyMatcherApplication[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.and(MatcherWords.not.be(resultOfAWordApplication))
()
()
/**
* This method enables the following syntax given a MatcherFactory2
, where apple
is a BePropertyMatcher
:
*
*
* aMatcherFactory and not be an (apple)
* ^
*
*/
infix def be[SC <: AnyRef](resultOfAnWordApplication: ResultOfAnWordToBePropertyMatcherApplication[SC]) = thisMatcherFactory.and(MatcherWords.not.be(resultOfAnWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
, where invalidMarks
is a AnMatcher
:
*
*
* aMatcherFactory and not be an (invalidMarks)
* ^
*
*/
infix def be[U](resultOfAnWordApplication: ResultOfAnWordToAnMatcherApplication[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.and(MatcherWords.not.be(resultOfAnWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not be a [Book]
* ^
*
*/
inline infix def be(aType: ResultOfATypeInvocation[_]): MatcherFactory2[SC with AnyRef, TC1, TC2] =
${ MatcherFactory2.andNotATypeMatcherFactory2[SC, TC1, TC2]('{thisAndNotWord: MatcherFactory2[SC with AnyRef, TC1, TC2]#AndNotWord}, '{aType}) }
0
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not be an [Apple]
* ^
*
*/
inline infix def be(anType: ResultOfAnTypeInvocation[_]): MatcherFactory2[SC with AnyRef, TC1, TC2] =
${ MatcherFactory2.andNotAnTypeMatcherFactory2[SC, TC1, TC2]('{thisAndNotWord: MatcherFactory2[SC with AnyRef, TC1, TC2]#AndNotWord}, '{anType}) }
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not be theSameInstanceAs (otherString)
* ^
*
*/
infix def be(resultOfTheSameInstanceAsApplication: ResultOfTheSameInstanceAsApplication): MatcherFactory2[SC with AnyRef, TC1, TC2] = thisMatcherFactory.and(MatcherWords.not.be(resultOfTheSameInstanceAsApplication))
/**
* This method enables the following syntax, for the "primitive" numeric types:
*
*
* aMatcherFactory and not be (17.0 +- 0.2)
* ^
*
*/
infix def be[U](spread: Spread[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.and(MatcherWords.not.be(spread))
/**
* This method enables the following syntax, where fraction
is a PartialFunction
:
*
*
* aMatcherFactory and not be definedAt (8)
* ^
*
*/
infix def be[A, U <: PartialFunction[A, _]](resultOfDefinedAt: ResultOfDefinedAt[A]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.be(resultOfDefinedAt))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory and not be sorted
* ^
*
*/
infix def be(sortedWord: SortedWord) =
thisMatcherFactory.and(MatcherWords.not.be(sortedWord))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory and not be readable
* ^
*
*/
infix def be(readableWord: ReadableWord) =
thisMatcherFactory.and(MatcherWords.not.be(readableWord))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory and not be writable
* ^
*
*/
infix def be(writableWord: WritableWord) =
thisMatcherFactory.and(MatcherWords.not.be(writableWord))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory and not be empty
* ^
*
*/
infix def be(emptyWord: EmptyWord) =
thisMatcherFactory.and(MatcherWords.not.be(emptyWord))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory and not be defined
* ^
*
*/
infix def be(definedWord: DefinedWord) =
thisMatcherFactory.and(MatcherWords.not.be(definedWord))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not fullyMatch regex (decimal)
* ^
*
*/
infix def fullyMatch(resultOfRegexWordApplication: ResultOfRegexWordApplication): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.fullyMatch(resultOfRegexWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not include regex (decimal)
* ^
*
*/
infix def include(resultOfRegexWordApplication: ResultOfRegexWordApplication): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.include(resultOfRegexWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not include ("1.7")
* ^
*
*/
infix def include(expectedSubstring: String): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.include(expectedSubstring))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not startWith regex (decimal)
* ^
*
*/
infix def startWith(resultOfRegexWordApplication: ResultOfRegexWordApplication): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.startWith(resultOfRegexWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not startWith ("1.7")
* ^
*
*/
infix def startWith(expectedSubstring: String): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.startWith(expectedSubstring))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not endWith regex (decimal)
* ^
*
*/
infix def endWith(resultOfRegexWordApplication: ResultOfRegexWordApplication): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.endWith(resultOfRegexWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not endWith ("1.7")
* ^
*
*/
infix def endWith(expectedSubstring: String): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.and(MatcherWords.not.endWith(expectedSubstring))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain (3)
* ^
*
*/
infix def contain[U](expectedElement: U): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.and(MatcherWords.not.contain(expectedElement))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain key ("three")
* ^
*
*/
infix def contain(resultOfKeyWordApplication: ResultOfKeyWordApplication): MatcherFactory3[SC, TC1, TC2, KeyMapping] =
thisMatcherFactory.and(MatcherWords.not.contain(resultOfKeyWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain value (3)
* ^
*
*/
infix def contain(resultOfValueWordApplication: ResultOfValueWordApplication): MatcherFactory3[SC, TC1, TC2, ValueMapping] =
thisMatcherFactory.and(MatcherWords.not.contain(resultOfValueWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain oneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfOneOfApplication): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain oneElementOf (List(8, 1, 2))
* ^
*
*/
infix def contain(right: ResultOfOneElementOfApplication): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain atLeastOneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAtLeastOneOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain atLeastOneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAtLeastOneElementOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain noneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfNoneOfApplication): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain noElementsOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfNoElementsOfApplication): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain theSameElementsAs (List(8, 1, 2))
* ^
*
*/
infix def contain(right: ResultOfTheSameElementsAsApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain theSameElementsInOrderAs (List(8, 1, 2))
* ^
*
*/
infix def contain(right: ResultOfTheSameElementsInOrderAsApplication): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain only (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfOnlyApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain inOrderOnly (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfInOrderOnlyApplication): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain allOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAllOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain allElementsOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAllElementsOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain inOrder (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfInOrderApplication): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain inOrder (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfInOrderElementsOfApplication): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain atMostOneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAtMostOneOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain atMostOneElementOf (List(8, 1, 2))
* ^
*
*/
infix def contain(right: ResultOfAtMostOneElementOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.and(MatcherWords.not.contain(right))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory and not matchPattern { case Person("Bob", _) =>}
* ^
*
*/
inline infix def matchPattern(inline right: PartialFunction[Any, _]) =
${ MatcherFactory2.andNotMatchPattern('{thisAndNotWord: MatcherFactory2[SC with AnyRef, TC1, TC2]#AndNotWord}, '{right}) }
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory and not contain value (3)
* ^
*
*/
infix def and(notWord: NotWord)(implicit prettifier: Prettifier, pos: source.Position): AndNotWord = new AndNotWord(prettifier, pos)
/**
* This method enables the following syntax:
*
*
* aMatcherFactory and exist
* ^
*
*/
infix def and(existWord: ExistWord): MatcherFactory3[SC, TC1, TC2, Existence] =
thisMatcherFactory.and(MatcherWords.exist.matcherFactory)
/**
* This method enables the following syntax:
*
*
* aMatcherFactory and not (exist)
* ^
*
*/
infix def and(notExist: ResultOfNotExist): MatcherFactory3[SC, TC1, TC2, Existence] =
thisMatcherFactory.and(MatcherWords.not.exist)
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class OrHaveWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or have length (3 - 1)
* ^
*
*/
infix def length(expectedLength: Long): MatcherFactory3[SC, TC1, TC2, Length] = or(MatcherWords.have.length(expectedLength))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or have size (3 - 1)
* ^
*
*/
infix def size(expectedSize: Long): MatcherFactory3[SC, TC1, TC2, Size] = or(MatcherWords.have.size(expectedSize))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or have message ("Message from Mars!")
* ^
*
*/
infix def message(expectedMessage: String): MatcherFactory3[SC, TC1, TC2, Messaging] = or(MatcherWords.have.message(expectedMessage))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or have size (3 - 1)
* ^
*
*/
infix def or(haveWord: HaveWord): OrHaveWord = new OrHaveWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class OrContainWord(prettifier: Prettifier, pos: source.Position) {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain (3 - 1)
* ^
*
*/
def apply(expectedElement: Any): MatcherFactory3[SC, TC1, TC2, Containing] = thisMatcherFactory.or(MatcherWords.contain(expectedElement))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain key ("one")
* ^
*
*/
infix def key(expectedKey: Any): MatcherFactory3[SC, TC1, TC2, KeyMapping] = thisMatcherFactory.or(MatcherWords.contain.key(expectedKey))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain value (1)
* ^
*
*/
infix def value(expectedValue: Any): MatcherFactory3[SC, TC1, TC2, ValueMapping] = thisMatcherFactory.or(MatcherWords.contain.value(expectedValue))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain theSameElementsAs List(1, 2, 3)
* ^
*
*/
infix def theSameElementsAs(right: GenTraversable[_]): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.contain.theSameElementsAs(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain theSameElementsInOrderAs List(1, 2, 3)
* ^
*
*/
infix def theSameElementsInOrderAs(right: GenTraversable[_]): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.or(MatcherWords.contain.theSameElementsInOrderAs(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain inOrderOnly (1, 2, 3)
* ^
*
*/
infix def inOrderOnly(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.or(MatcherWords.contain.inOrderOnly(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain allOf (1, 2, 3)
* ^
*
*/
infix def allOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.contain.allOf(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain allElementsOf (1, 2, 3)
* ^
*
*/
infix def allElementsOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.contain.allElementsOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain inOrder (1, 2, 3)
* ^
*
*/
infix def inOrder(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.or(MatcherWords.contain.inOrder(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain inOrderElementsOf List(1, 2, 3)
* ^
*
*/
infix def inOrderElementsOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.or(MatcherWords.contain.inOrderElementsOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain oneOf (1, 2, 3)
* ^
*
*/
infix def oneOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.or(MatcherWords.contain.oneOf(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain oneElementOf (1, 2, 3)
* ^
*
*/
infix def oneElementOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.or(MatcherWords.contain.oneElementOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain atLeastOneOf (1, 2, 3)
* ^
*
*/
infix def atLeastOneOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.contain.atLeastOneOf(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain atLeastOneOf (1, 2, 3)
* ^
*
*/
infix def atLeastOneElementOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.contain.atLeastOneElementOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain only (1, 2, 3)
* ^
*
*/
infix def only(right: Any*): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.contain.only(right.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain noneOf (1, 2, 3)
* ^
*
*/
infix def noneOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.or(MatcherWords.contain.noneOf(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain noElementsOf (1, 2, 3)
* ^
*
*/
infix def noElementsOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.or(MatcherWords.contain.noElementsOf(elements))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain atMostOneOf (1, 2, 3)
* ^
*
*/
infix def atMostOneOf(firstEle: Any, secondEle: Any, remainingEles: Any*): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.contain.atMostOneOf(firstEle, secondEle, remainingEles.toList: _*)(prettifier, pos))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or contain atMostOneOf (1, 2, 3)
* ^
*
*/
infix def atMostOneElementOf(elements: GenTraversable[Any]): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.contain.atMostOneElementOf(elements))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* (aMatcherFactory or contain value (1))
* ^
*
*/
infix def or(containWord: ContainWord)(implicit prettifier: Prettifier, pos: source.Position): OrContainWord = new OrContainWord(prettifier, pos)
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class OrBeWord {
()
()
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or be a (directory)
* ^
*
*/
infix def a[U](bePropertyMatcher: BePropertyMatcher[U]): MatcherFactory2[SC with AnyRef with U, TC1, TC2] = or(MatcherWords.be.a(bePropertyMatcher))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or be a (validNumber)
* ^
*
*/
infix def a[U](aMatcher: AMatcher[U]): MatcherFactory2[SC with U, TC1, TC2] = or(MatcherWords.be.a(aMatcher))
()
()
/**
* This method enables the following syntax given a MatcherFactory2
, where apple
is a BePropertyMatcher
:
*
*
* aMatcherFactory or be an (apple)
* ^
*
*/
infix def an[U](bePropertyMatcher: BePropertyMatcher[U]): MatcherFactory2[SC with AnyRef with U, TC1, TC2] = or(MatcherWords.be.an(bePropertyMatcher))
/**
* This method enables the following syntax given a MatcherFactory2
, where integerNumber
is a AnMatcher
:
*
*
* aMatcherFactory or be an (integerNumber)
* ^
*
*/
infix def an[U](anMatcher: AnMatcher[U]): MatcherFactory2[SC with U, TC1, TC2] = or(MatcherWords.be.an(anMatcher))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or be theSameInstanceAs (otherString)
* ^
*
*/
infix def theSameInstanceAs(anyRef: AnyRef): MatcherFactory2[SC with AnyRef, TC1, TC2] = or(MatcherWords.be.theSameInstanceAs(anyRef))
/**
* This method enables the following syntax, where fraction
refers to a PartialFunction
:
*
*
* aMatcherFactory or be definedAt (8)
* ^
*
*/
infix def definedAt[A, U <: PartialFunction[A, _]](right: A): MatcherFactory2[SC with U, TC1, TC2] = or(MatcherWords.be.definedAt(right))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or be a ('directory)
* ^
*
*/
infix def or(beWord: BeWord): OrBeWord = new OrBeWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class OrFullyMatchWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or fullyMatch regex (decimal)
* ^
*
*/
infix def regex(regexString: String): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.fullyMatch.regex(regexString))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or fullyMatch regex ("a(b*)c" withGroup "bb")
* ^
*
*/
infix def regex(regexWithGroups: RegexWithGroups): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.fullyMatch.regex(regexWithGroups))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or fullyMatch regex (decimal)
* ^
*
*/
infix def regex(regex: Regex): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.fullyMatch.regex(regex))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or fullyMatch regex (decimal)
* ^
*
*/
infix def or(fullyMatchWord: FullyMatchWord): OrFullyMatchWord = new OrFullyMatchWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class OrIncludeWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or include regex (decimal)
* ^
*
*/
infix def regex(regexString: String): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.include.regex(regexString))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or include regex ("a(b*)c" withGroup "bb")
* ^
*
*/
infix def regex(regexWithGroups: RegexWithGroups): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.include.regex(regexWithGroups))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or include regex (decimal)
* ^
*
*/
infix def regex(regex: Regex): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.include.regex(regex))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or include regex ("1.7")
* ^
*
*/
infix def or(includeWord: IncludeWord): OrIncludeWord = new OrIncludeWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class OrStartWithWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or startWith regex (decimal)
* ^
*
*/
infix def regex(regexString: String): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.startWith.regex(regexString))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or startWith regex ("a(b*)c" withGroup "bb")
* ^
*
*/
infix def regex(regexWithGroups: RegexWithGroups): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.startWith.regex(regexWithGroups))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or startWith regex (decimal)
* ^
*
*/
infix def regex(regex: Regex): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.startWith.regex(regex))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or startWith regex ("1.7")
* ^
*
*/
infix def or(startWithWord: StartWithWord): OrStartWithWord = new OrStartWithWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class OrEndWithWord {
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or endWith regex (decimal)
* ^
*
*/
infix def regex(regexString: String): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.endWith.regex(regexString))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or endWith regex ("d(e*)f" withGroup "ee")
* ^
*
*/
infix def regex(regexWithGroups: RegexWithGroups): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.endWith.regex(regexWithGroups))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or endWith regex (decimal)
* ^
*
*/
infix def regex(regex: Regex): MatcherFactory2[SC with String, TC1, TC2] = or(MatcherWords.endWith.regex(regex))
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or endWith regex ("7b")
* ^
*
*/
infix def or(endWithWord: EndWithWord): OrEndWithWord = new OrEndWithWord
/**
* This class is part of the ScalaTest matchers DSL. Please see the documentation for Matchers
for an overview of
* the matchers DSL.
*
* @author Bill Venners
*/
final class OrNotWord(prettifier: Prettifier, pos: source.Position) { thisOrNotWord =>
/**
* Get the MatcherFactory
instance, currently used by macro.
*/
val owner = thisMatcherFactory
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not equal (3 - 1)
* ^
*
*/
infix def equal(any: Any): MatcherFactory3[SC, TC1, TC2, Equality] =
thisMatcherFactory.or(MatcherWords.not.apply(MatcherWords.equal(any)))
/**
* This method enables the following syntax for the "primitive" numeric types:
*
*
* aMatcherFactory or not equal (17.0 +- 0.2)
* ^
*
*/
infix def equal[U](spread: Spread[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.equal(spread))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not equal (null)
* ^
*
*/
infix def equal(o: Null): MatcherFactory2[SC, TC1, TC2] = {
thisMatcherFactory or {
new Matcher[SC] {
def apply(left: SC): MatchResult = {
MatchResult(
left != null,
Resources.rawEqualedNull,
Resources.rawDidNotEqualNull,
Resources.rawMidSentenceEqualedNull,
Resources.rawDidNotEqualNull,
Vector.empty,
Vector(left)
)
}
override def toString: String = "not equal null"
}
}
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not be (2)
* ^
*
*/
infix def be(any: Any): MatcherFactory2[SC, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.apply(MatcherWords.be(any)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not have length (3)
* ^
*
*/
infix def have(resultOfLengthWordApplication: ResultOfLengthWordApplication): MatcherFactory3[SC, TC1, TC2, Length] =
thisMatcherFactory.or(MatcherWords.not.apply(MatcherWords.have.length(resultOfLengthWordApplication.expectedLength)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not have size (3)
* ^
*
*/
infix def have(resultOfSizeWordApplication: ResultOfSizeWordApplication): MatcherFactory3[SC, TC1, TC2, Size] =
thisMatcherFactory.or(MatcherWords.not.apply(MatcherWords.have.size(resultOfSizeWordApplication.expectedSize)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not have message ("Message from Mars!")
* ^
*
*/
infix def have(resultOfMessageWordApplication: ResultOfMessageWordApplication): MatcherFactory3[SC, TC1, TC2, Messaging] =
thisMatcherFactory.or(MatcherWords.not.apply(MatcherWords.have.message(resultOfMessageWordApplication.expectedMessage)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not have (author ("Melville"))
* ^
*
*/
infix def have[U](firstPropertyMatcher: HavePropertyMatcher[U, _], propertyMatchers: HavePropertyMatcher[U, _]*): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.apply(MatcherWords.have(firstPropertyMatcher, propertyMatchers: _*)))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not be (null)
* ^
*
*/
infix def be(o: Null): MatcherFactory2[SC with AnyRef, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.be(o))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not be < (8)
* ^
*
*/
infix def be[U](resultOfLessThanComparison: ResultOfLessThanComparison[U]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.be(resultOfLessThanComparison))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not be > (6)
* ^
*
*/
infix def be[U](resultOfGreaterThanComparison: ResultOfGreaterThanComparison[U]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.be(resultOfGreaterThanComparison))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not be <= (2)
* ^
*
*/
infix def be[U](resultOfLessThanOrEqualToComparison: ResultOfLessThanOrEqualToComparison[U]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.be(resultOfLessThanOrEqualToComparison))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not be >= (6)
* ^
*
*/
infix def be[U](resultOfGreaterThanOrEqualToComparison: ResultOfGreaterThanOrEqualToComparison[U]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.be(resultOfGreaterThanOrEqualToComparison))
/**
*
* The deprecation period for the "be ===" syntax has expired, and the syntax
* will now throw NotAllowedException
. Please use should equal, should ===, shouldEqual,
* should be, or shouldBe instead.
*
*
*
* Note: usually syntax will be removed after its deprecation period. This was left in because otherwise the syntax could in some
* cases still compile, but silently wouldn't work.
*
*/
infix def be(tripleEqualsInvocation: TripleEqualsInvocation[_]): MatcherFactory2[SC, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.be(tripleEqualsInvocation)(pos))
()
()
/**
* This method enables the following syntax given a MatcherFactory2
, where odd
is a BeMatcher
:
*
*
* aMatcherFactory or not be (odd)
* ^
*
*/
infix def be[U](beMatcher: BeMatcher[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.be(beMatcher))
/**
* This method enables the following syntax given a MatcherFactory2
, where file
is a BePropertyMatcher
:
*
*
* aMatcherFactory or not be (file)
* ^
*
*/
infix def be[U](bePropertyMatcher: BePropertyMatcher[U]): MatcherFactory2[SC with AnyRef with U, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.be(bePropertyMatcher))
()
()
/**
* This method enables the following syntax given a MatcherFactory2
, where validMarks
is an AMatcher
:
*
*
* aMatcherFactory or not be a (validMarks)
* ^
*
*/
infix def be[U](resultOfAWordApplication: ResultOfAWordToAMatcherApplication[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.be(resultOfAWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
, where file
is a BePropertyMatcher
:
*
*
* aMatcherFactory or not be a (file)
* ^
*
*/
infix def be[U <: AnyRef](resultOfAWordApplication: ResultOfAWordToBePropertyMatcherApplication[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.be(resultOfAWordApplication))
()
()
/**
* This method enables the following syntax given a MatcherFactory2
, where apple
is a BePropertyMatcher
:
*
*
* aMatcherFactory or not be an (apple)
* ^
*
*/
infix def be[U <: AnyRef](resultOfAnWordApplication: ResultOfAnWordToBePropertyMatcherApplication[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.be(resultOfAnWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
, where invalidMarks
is an AnMatcher
:
*
*
* aMatcherFactory and not be an (invalidMarks)
* ^
*
*/
infix def be[U](resultOfAnWordApplication: ResultOfAnWordToAnMatcherApplication[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.be(resultOfAnWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not be a [Book]
* ^
*
*/
inline infix def be(aType: ResultOfATypeInvocation[_]): MatcherFactory2[SC with AnyRef, TC1, TC2] =
${ MatcherFactory2.orNotATypeMatcherFactory2[SC, TC1, TC2]('{thisOrNotWord: MatcherFactory2[SC with AnyRef, TC1, TC2]#OrNotWord}, '{aType}) }
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not be an [Apple]
* ^
*
*/
inline infix def be(anType: ResultOfAnTypeInvocation[_]): MatcherFactory2[SC with AnyRef, TC1, TC2] =
${ MatcherFactory2.orNotAnTypeMatcherFactory2[SC, TC1, TC2]('{thisOrNotWord: MatcherFactory2[SC with AnyRef, TC1, TC2]#OrNotWord}, '{anType}) }
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not be theSameInstanceAs (string)
* ^
*
*/
infix def be(resultOfTheSameInstanceAsApplication: ResultOfTheSameInstanceAsApplication): MatcherFactory2[SC with AnyRef, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.be(resultOfTheSameInstanceAsApplication))
/**
* This method enables the following syntax for the "primitive" numeric types:
*
*
* aMatcherFactory or not be (17.0 +- 0.2)
* ^
*
*/
infix def be[U](spread: Spread[U]): MatcherFactory2[SC with U, TC1, TC2] = thisMatcherFactory.or(MatcherWords.not.be(spread))
/**
* This method enables the following syntax, where fraction
is a PartialFunction
:
*
*
* aMatcherFactory or not be definedAt (8)
* ^
*
*/
infix def be[A, U <: PartialFunction[A, _]](resultOfDefinedAt: ResultOfDefinedAt[A]): MatcherFactory2[SC with U, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.be(resultOfDefinedAt))
/**
* This method enables the following syntax, where fraction
is a PartialFunction
:
*
*
* aMatcherFactory or not be sorted
* ^
*
*/
infix def be(sortedWord: SortedWord) =
thisMatcherFactory.or(MatcherWords.not.be(sortedWord))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory or not be readable
* ^
*
*/
infix def be(readableWord: ReadableWord) =
thisMatcherFactory.or(MatcherWords.not.be(readableWord))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory or not be writable
* ^
*
*/
infix def be(writableWord: WritableWord) =
thisMatcherFactory.or(MatcherWords.not.be(writableWord))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory or not be empty
* ^
*
*/
infix def be(emptyWord: EmptyWord) =
thisMatcherFactory.or(MatcherWords.not.be(emptyWord))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory or not be defined
* ^
*
*/
infix def be(definedWord: DefinedWord) =
thisMatcherFactory.or(MatcherWords.not.be(definedWord))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not fullyMatch regex (decimal)
* ^
*
*/
infix def fullyMatch(resultOfRegexWordApplication: ResultOfRegexWordApplication): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.fullyMatch(resultOfRegexWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not include regex (decimal)
* ^
*
*/
infix def include(resultOfRegexWordApplication: ResultOfRegexWordApplication): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.include(resultOfRegexWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not include ("1.7")
* ^
*
*/
infix def include(expectedSubstring: String): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.include(expectedSubstring))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not startWith regex (decimal)
* ^
*
*/
infix def startWith(resultOfRegexWordApplication: ResultOfRegexWordApplication): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.startWith(resultOfRegexWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not startWith ("1.7")
* ^
*
*/
infix def startWith(expectedSubstring: String): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.startWith(expectedSubstring))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not endWith regex (decimal)
* ^
*
*/
infix def endWith(resultOfRegexWordApplication: ResultOfRegexWordApplication): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.endWith(resultOfRegexWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not endWith ("1.7")
* ^
*
*/
infix def endWith(expectedSubstring: String): MatcherFactory2[SC with String, TC1, TC2] =
thisMatcherFactory.or(MatcherWords.not.endWith(expectedSubstring))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain (3)
* ^
*
*/
infix def contain[U](expectedElement: U): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.or(MatcherWords.not.contain(expectedElement))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain key ("three")
* ^
*
*/
infix def contain(resultOfKeyWordApplication: ResultOfKeyWordApplication): MatcherFactory3[SC, TC1, TC2, KeyMapping] =
thisMatcherFactory.or(MatcherWords.not.contain(resultOfKeyWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain value (3)
* ^
*
*/
infix def contain(resultOfValueWordApplication: ResultOfValueWordApplication): MatcherFactory3[SC, TC1, TC2, ValueMapping] =
thisMatcherFactory.or(MatcherWords.not.contain(resultOfValueWordApplication))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain oneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfOneOfApplication): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain oneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfOneElementOfApplication): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain atLeastOneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAtLeastOneOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain atLeastOneElementOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAtLeastOneElementOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain noneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfNoneOfApplication): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain noElementsOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfNoElementsOfApplication): MatcherFactory3[SC, TC1, TC2, Containing] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain theSameElementsAs (List(8, 1, 2))
* ^
*
*/
infix def contain(right: ResultOfTheSameElementsAsApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain theSameElementsInOrderAs (List(8, 1, 2))
* ^
*
*/
infix def contain(right: ResultOfTheSameElementsInOrderAsApplication): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain only (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfOnlyApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain inOrderOnly (8, 1, 2))
* ^
*
*/
infix def contain(right: ResultOfInOrderOnlyApplication): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain allOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAllOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain allOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAllElementsOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain inOrder (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfInOrderApplication): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain inOrderElementsOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfInOrderElementsOfApplication): MatcherFactory3[SC, TC1, TC2, Sequencing] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain atMostOneOf (8, 1, 2)
* ^
*
*/
infix def contain(right: ResultOfAtMostOneOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain atMostOneElementOf (List(8, 1, 2))
* ^
*
*/
infix def contain(right: ResultOfAtMostOneElementOfApplication): MatcherFactory3[SC, TC1, TC2, Aggregating] =
thisMatcherFactory.or(MatcherWords.not.contain(right))
/**
* This method enables the following syntax:
*
*
* aMatcherFactory or not matchPattern { case Person("Bob", _) =>}
* ^
*
*/
inline infix def matchPattern(inline right: PartialFunction[Any, _]) =
${ MatcherFactory2.orNotMatchPattern('{thisOrNotWord: MatcherFactory2[SC with AnyRef, TC1, TC2]#OrNotWord}, '{right}) }
}
/**
* This method enables the following syntax given a MatcherFactory2
:
*
*
* aMatcherFactory or not contain value (3)
* ^
*
*/
infix def or(notWord: NotWord)(implicit prettifier: Prettifier, pos: source.Position): OrNotWord = new OrNotWord(prettifier, pos)
/**
* This method enables the following syntax:
*
*
* aMatcherFactory or exist
* ^
*
*/
infix def or(existWord: ExistWord): MatcherFactory3[SC, TC1, TC2, Existence] =
thisMatcherFactory.or(MatcherWords.exist.matcherFactory)
/**
* This method enables the following syntax:
*
*
* aMatcherFactory or not (exist)
* ^
*
*/
infix def or(notExist: ResultOfNotExist): MatcherFactory3[SC, TC1, TC2, Existence] =
thisMatcherFactory.or(MatcherWords.not.exist)
}
/**
* Companion object containing an implicit method that converts a MatcherFactory2
to a Matcher
.
*
* @author Bill Venners
*/
object MatcherFactory2 {
import scala.language.implicitConversions
import scala.quoted._
/**
* Converts a MatcherFactory2
to a Matcher
.
*
* @param matcherFactory a MatcherFactory2 to convert
* @return a Matcher produced by the passed MatcherFactory2
*/
implicit def produceMatcher[SC, TC1[_], TC2[_], T <: SC : TC1 : TC2](matcherFactory: MatcherFactory2[SC, TC1, TC2]): Matcher[T] =
matcherFactory.matcher
/**
* This method is called by macro that supports 'and not a [Type]' syntax.
*/
def andNotATypeMatcherFactory2[SC: Type, TC1[_], TC2[_]](self: Expr[MatcherFactory2[SC & AnyRef, TC1, TC2]#AndNotWord], aType: Expr[ResultOfATypeInvocation[_]])(using Quotes, Type[TC1], Type[TC2]): Expr[MatcherFactory2[SC with AnyRef, TC1, TC2]] = {
val rhs = TypeMatcherMacro.notATypeMatcher(aType)
'{ ($self).owner.and($rhs) }
}
/**
* This method is called by macro that supports 'or not a [Type]' syntax.
*/
def orNotATypeMatcherFactory2[SC: Type, TC1[_], TC2[_]](self: Expr[MatcherFactory2[SC & AnyRef, TC1, TC2]#OrNotWord], aType: Expr[ResultOfATypeInvocation[_]])(using Quotes, Type[TC1], Type[TC2]): Expr[MatcherFactory2[SC with AnyRef, TC1, TC2]] = {
val rhs = TypeMatcherMacro.notATypeMatcher(aType)
'{ ($self).owner.or($rhs) }
}
/**
* This method is called by macro that supports 'and not a [Type]' syntax.
*/
def andNotAnTypeMatcherFactory2[SC: Type, TC1[_], TC2[_]](self: Expr[MatcherFactory2[SC & AnyRef, TC1, TC2]#AndNotWord], anType: Expr[ResultOfAnTypeInvocation[_]])(using Quotes, Type[TC1], Type[TC2]): Expr[MatcherFactory2[SC with AnyRef, TC1, TC2]] = {
val rhs = TypeMatcherMacro.notAnTypeMatcher(anType)
'{ ($self).owner.and($rhs) }
}
/**
* This method is called by macro that supports 'or not a [Type]' syntax.
*/
def orNotAnTypeMatcherFactory2[SC: Type, TC1[_], TC2[_]](self: Expr[MatcherFactory2[SC & AnyRef, TC1, TC2]#OrNotWord], anType: Expr[ResultOfAnTypeInvocation[_]])(using Quotes, Type[TC1], Type[TC2]): Expr[MatcherFactory2[SC with AnyRef, TC1, TC2]] = {
val rhs = TypeMatcherMacro.notAnTypeMatcher(anType)
'{ ($self).owner.or($rhs) }
}
def andNotMatchPattern[SC: Type, TC1[_], TC2[_]](self: Expr[MatcherFactory2[SC & AnyRef, TC1, TC2]#AndNotWord], right: Expr[PartialFunction[Any, _]])(using Quotes, Type[TC1], Type[TC2]): Expr[MatcherFactory2[SC with AnyRef, TC1, TC2]] = {
org.scalatest.matchers.MatchPatternMacro.checkCaseDefinitions(right)
val notMatcher = '{ MatchPatternHelper.notMatchPatternMatcher($right) }
'{ ($self).owner.and($notMatcher) }
}
def orNotMatchPattern[SC: Type, TC1[_], TC2[_]](self: Expr[MatcherFactory2[SC & AnyRef, TC1, TC2]#OrNotWord], right: Expr[PartialFunction[Any, _]])(using Quotes, Type[TC1], Type[TC2]): Expr[MatcherFactory2[SC with AnyRef, TC1, TC2]] = {
org.scalatest.matchers.MatchPatternMacro.checkCaseDefinitions(right)
val notMatcher = '{ MatchPatternHelper.notMatchPatternMatcher($right) }
'{ ($self).owner.or($notMatcher) }
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy