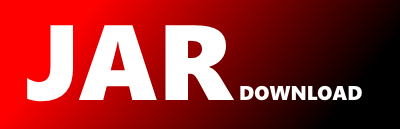
org.scalactic.Resources.scala Maven / Gradle / Ivy
The newest version!
package org.scalactic
private[scalactic] object Resources {
def formatString(rawString: String, args: Array[Any]): String = {
args.zipWithIndex.foldLeft(rawString) { case (result, (arg, idx)) =>
result.replaceAllLiterally(s"{$idx}", arg.toString())
}
}
final val rawBigProblems = "An exception or error caused a run to abort."
final val bigProblems = rawBigProblems
final val rawBigProblemsWithMessage = "An exception or error caused a run to abort: {0}"
def bigProblemsWithMessage(param0: Any): String =
rawBigProblemsWithMessage.replaceAllLiterally("{0}", param0.toString())
final val rawDidNotEqual = "{0} did not equal {1}"
def didNotEqual(param0: Any, param1: Any): String =
rawDidNotEqual.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawEqualed = "{0} equaled {1}"
def equaled(param0: Any, param1: Any): String =
rawEqualed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasPlusOrMinus = "{0} was {1} plus or minus {2}"
def wasPlusOrMinus(param0: Any, param1: Any, param2: Any): String =
rawWasPlusOrMinus.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawWasNotPlusOrMinus = "{0} was not {1} plus or minus {2}"
def wasNotPlusOrMinus(param0: Any, param1: Any, param2: Any): String =
rawWasNotPlusOrMinus.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawExpressionWasFalse = "Expression was false"
final val expressionWasFalse = rawExpressionWasFalse
final val rawExpressionWasTrue = "Expression was true"
final val expressionWasTrue = rawExpressionWasTrue
final val rawWasNull = "{0} was null"
def wasNull(param0: Any): String =
rawWasNull.replaceAllLiterally("{0}", param0.toString())
final val rawWereNull = "{0} were null"
def wereNull(param0: Any): String =
rawWereNull.replaceAllLiterally("{0}", param0.toString())
final val rawComma = ",\u0020"
final val comma = rawComma
final val rawAnd = "{0} and {1}"
def and(param0: Any, param1: Any): String =
rawAnd.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasTrue = "{0} was true"
def wasTrue(param0: Any): String =
rawWasTrue.replaceAllLiterally("{0}", param0.toString())
final val rawWasFalse = "{0} was false"
def wasFalse(param0: Any): String =
rawWasFalse.replaceAllLiterally("{0}", param0.toString())
final val rawWasLessThan = "{0} was less than {1}"
def wasLessThan(param0: Any, param1: Any): String =
rawWasLessThan.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotLessThan = "{0} was not less than {1}"
def wasNotLessThan(param0: Any, param1: Any): String =
rawWasNotLessThan.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasGreaterThan = "{0} was greater than {1}"
def wasGreaterThan(param0: Any, param1: Any): String =
rawWasGreaterThan.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotGreaterThan = "{0} was not greater than {1}"
def wasNotGreaterThan(param0: Any, param1: Any): String =
rawWasNotGreaterThan.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasLessThanOrEqualTo = "{0} was less than or equal to {1}"
def wasLessThanOrEqualTo(param0: Any, param1: Any): String =
rawWasLessThanOrEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotLessThanOrEqualTo = "{0} was not less than or equal to {1}"
def wasNotLessThanOrEqualTo(param0: Any, param1: Any): String =
rawWasNotLessThanOrEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasGreaterThanOrEqualTo = "{0} was greater than or equal to {1}"
def wasGreaterThanOrEqualTo(param0: Any, param1: Any): String =
rawWasGreaterThanOrEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotGreaterThanOrEqualTo = "{0} was not greater than or equal to {1}"
def wasNotGreaterThanOrEqualTo(param0: Any, param1: Any): String =
rawWasNotGreaterThanOrEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotStartWith = "{0} did not start with {1}"
def didNotStartWith(param0: Any, param1: Any): String =
rawDidNotStartWith.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawStartedWith = "{0} started with {1}"
def startedWith(param0: Any, param1: Any): String =
rawStartedWith.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotEndWith = "{0} did not end with {1}"
def didNotEndWith(param0: Any, param1: Any): String =
rawDidNotEndWith.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawEndedWith = "{0} ended with {1}"
def endedWith(param0: Any, param1: Any): String =
rawEndedWith.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContain = "{0} did not contain {1}"
def didNotContain(param0: Any, param1: Any): String =
rawDidNotContain.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContained = "{0} contained {1}"
def contained(param0: Any, param1: Any): String =
rawContained.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainKey = "{0} did not contain key {1}"
def didNotContainKey(param0: Any, param1: Any): String =
rawDidNotContainKey.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedKey = "{0} contained key {1}"
def containedKey(param0: Any, param1: Any): String =
rawContainedKey.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasTheSameInstanceAs = "{0} was the same instance as {1}"
def wasTheSameInstanceAs(param0: Any, param1: Any): String =
rawWasTheSameInstanceAs.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotTheSameInstanceAs = "{0} was not the same instance as {1}"
def wasNotTheSameInstanceAs(param0: Any, param1: Any): String =
rawWasNotTheSameInstanceAs.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotEmpty = "{0} was not empty"
def wasNotEmpty(param0: Any): String =
rawWasNotEmpty.replaceAllLiterally("{0}", param0.toString())
final val rawWasEmpty = "{0} was empty"
def wasEmpty(param0: Any): String =
rawWasEmpty.replaceAllLiterally("{0}", param0.toString())
final val rawWasNotInstanceOf = "{0} was not instance of {1}"
def wasNotInstanceOf(param0: Any, param1: Any): String =
rawWasNotInstanceOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasInstanceOf = "{0} was instance of {1}"
def wasInstanceOf(param0: Any, param1: Any): String =
rawWasInstanceOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHadLengthInsteadOfExpectedLength = "{0} had length {1} instead of expected length {2}"
def hadLengthInsteadOfExpectedLength(param0: Any, param1: Any, param2: Any): String =
rawHadLengthInsteadOfExpectedLength.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawHadLength = "{0} had length {1}"
def hadLength(param0: Any, param1: Any): String =
rawHadLength.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHadSizeInsteadOfExpectedSize = "{0} had size {1} instead of expected size {2}"
def hadSizeInsteadOfExpectedSize(param0: Any, param1: Any, param2: Any): String =
rawHadSizeInsteadOfExpectedSize.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawHadSize = "{0} had size {1}"
def hadSize(param0: Any, param1: Any): String =
rawHadSize.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawCommaBut = "{0}, but {1}"
def commaBut(param0: Any, param1: Any): String =
rawCommaBut.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawCommaAnd = "{0}, and {1}"
def commaAnd(param0: Any, param1: Any): String =
rawCommaAnd.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawVariableWasValue = "{0} was {1}"
def variableWasValue(param0: Any, param1: Any): String =
rawVariableWasValue.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawNotValidPosInt = "PosInt.apply can only be invoked on a positive (n > 0) integer literal, like PosInt(42)."
final val notValidPosInt = rawNotValidPosInt
final val rawNotLiteralPosInt = "PosInt.apply can only be invoked on an integer literal, like PosInt(42). Please use PosInt.from instead."
final val notLiteralPosInt = rawNotLiteralPosInt
final val rawNotValidPosLong = "PosLong.apply can only be invoked on a positive (n > 0L) long literal, like PosLong(42L)."
final val notValidPosLong = rawNotValidPosLong
final val rawNotLiteralPosLong = "PosLong.apply can only be invoked on an integer literal, like PosLong(42L). Please use PosLong.from instead."
final val notLiteralPosLong = rawNotLiteralPosLong
final val rawNotValidPosFloat = "PosFloat.apply can only be invoked on a positive (n > 0.0F && !n.isNaN) floating point literal, like PosFloat(42.0F)."
final val notValidPosFloat = rawNotValidPosFloat
final val rawNotLiteralPosFloat = "PosFloat.apply can only be invoked on a floating point literal, like PosFloat(42.0F). Please use PosFloat.from instead."
final val notLiteralPosFloat = rawNotLiteralPosFloat
final val rawNotValidPosDouble = "PosDouble.apply can only be invoked on a positive (n > 0.0 && !n.isNaN) floating point literal, like PosDouble(42.0)."
final val notValidPosDouble = rawNotValidPosDouble
final val rawNotLiteralPosDouble = "PosDouble.apply can only be invoked on a floating point literal, like PosDouble(42.0). Please use PosDouble.from instead."
final val notLiteralPosDouble = rawNotLiteralPosDouble
final val rawNotValidPosZInt = "PosZInt.apply can only be invoked on a non-negative (n >= 0) integer literal, like PosZInt(42)."
final val notValidPosZInt = rawNotValidPosZInt
final val rawNotLiteralPosZInt = "PosZInt.apply can only be invoked on an integer literal, like PosZInt(42). Please use PosZInt.from instead."
final val notLiteralPosZInt = rawNotLiteralPosZInt
final val rawNotValidPosZLong = "PosZLong.apply can only be invoked on a non-negative (n >= 0L) integer literal, like PosZLong(42L)."
final val notValidPosZLong = rawNotValidPosZLong
final val rawNotLiteralPosZLong = "PosZLong.apply can only be invoked on an long literal, like PosZLong(42L). Please use PosZLong.from instead."
final val notLiteralPosZLong = rawNotLiteralPosZLong
final val rawNotValidPosZFloat = "PosZFloat.apply can only be invoked on a non-negative (n >= 0.0F && !n.isNaN) floating point literal, like PosZFloat(42.0F)."
final val notValidPosZFloat = rawNotValidPosZFloat
final val rawNotLiteralPosZFloat = "PosZFloat.apply can only be invoked on a floating point literal, like PosZFloat(42.0F). Please use PosZFloat.from instead."
final val notLiteralPosZFloat = rawNotLiteralPosZFloat
final val rawNotValidPosZDouble = "PosZDouble.apply can only be invoked on a non-negative (n >= 0.0 && !n.isNaN) floating point literal, like PosZDouble(42.0)."
final val notValidPosZDouble = rawNotValidPosZDouble
final val rawNotLiteralPosZDouble = "PosZDouble.apply can only be invoked on a floating point literal, like PosZDouble(42.0). Please use PosZDouble.from instead."
final val notLiteralPosZDouble = rawNotLiteralPosZDouble
final val rawPleaseDefineScalacticFillFilePathnameEnvVar = "Please set the environment variable SCALACTIC_FILL_FILE_PATHNAMES to yes at compile time to enable this feature."
final val pleaseDefineScalacticFillFilePathnameEnvVar = rawPleaseDefineScalacticFillFilePathnameEnvVar
final val rawNotValidNonZeroInt = "NonZeroInt.apply can only be invoked on a non-zero (n != 0) integer literal, like NonZeroInt(42)."
final val notValidNonZeroInt = rawNotValidNonZeroInt
final val rawNotLiteralNonZeroInt = "NonZeroInt.apply can only be invoked on an integer literal, like NonZeroInt(42). Please use NonZeroInt.from instead."
final val notLiteralNonZeroInt = rawNotLiteralNonZeroInt
final val rawNotValidNonZeroLong = "NonZeroLong.apply can only be invoked on a non-zero (n != 0L) long literal, like NonZeroLong(42L)."
final val notValidNonZeroLong = rawNotValidNonZeroLong
final val rawNotLiteralNonZeroLong = "NonZeroLong.apply can only be invoked on an long literal, like NonZeroLong(42L). Please use NonZeroLong.from instead."
final val notLiteralNonZeroLong = rawNotLiteralNonZeroLong
final val rawNotValidNonZeroFloat = "NonZeroFloat.apply can only be invoked on a non-zero (n != 0.0F && !i.isNaN) floating point literal, like NonZeroFloat(1.1F)."
final val notValidNonZeroFloat = rawNotValidNonZeroFloat
final val rawNotLiteralNonZeroFloat = "NonZeroFloat.apply can only be invoked on a floating point literal, like NonZeroFloat(1.1F). Please use NonZeroFloat.from instead."
final val notLiteralNonZeroFloat = rawNotLiteralNonZeroFloat
final val rawNotValidNonZeroDouble = "NonZeroDouble.apply can only be invoked on a non-zero (n != 0.0 && !i.isNaN) double literal, like NonZeroDouble(42.0)."
final val notValidNonZeroDouble = rawNotValidNonZeroDouble
final val rawNotLiteralNonZeroDouble = "ZonZeroDouble.apply can only be invoked on a non-zero literal, like NonZeroDouble(42.0). Please use NonZeroDouble.from instead."
final val notLiteralNonZeroDouble = rawNotLiteralNonZeroDouble
final val rawNotValidNegInt = "NegInt.apply can only be invoked on a negative (n < 0) integer literal, like NegInt(-42)."
final val notValidNegInt = rawNotValidNegInt
final val rawNotLiteralNegInt = "NegInt.apply can only be invoked on an integer literal, like NegInt(-42). Please use NegInt.from instead."
final val notLiteralNegInt = rawNotLiteralNegInt
final val rawNotValidNegLong = "NegLong.apply can only be invoked on a negative (n < 0L) long literal, like NegLong(-42L)."
final val notValidNegLong = rawNotValidNegLong
final val rawNotLiteralNegLong = "NegLong.apply can only be invoked on a long literal, like NegLong(-42L). Please use NegLong.from instead."
final val notLiteralNegLong = rawNotLiteralNegLong
final val rawNotValidNegFloat = "NegFloat.apply can only be invoked on a negative (n < 0.0F && !n.isNaN) floating point literal, like NegFloat(-42.0F)."
final val notValidNegFloat = rawNotValidNegFloat
final val rawNotLiteralNegFloat = "NegFloat.apply can only be invoked on a floating point literal, like NegFloat(-42.0F). Please use NegFloat.from instead."
final val notLiteralNegFloat = rawNotLiteralNegFloat
final val rawNotValidNegDouble = "NegDouble.apply can only be invoked on a negative (n < 0.0 && !n.isNaN) floating point literal, like NegDouble(-42.0)."
final val notValidNegDouble = rawNotValidNegDouble
final val rawNotLiteralNegDouble = "NegDouble.apply can only be invoked on a floating point literal, like NegDouble(-42.0). Please use NegDouble.from instead."
final val notLiteralNegDouble = rawNotLiteralNegDouble
final val rawNotValidNegZInt = "NegZInt.apply can only be invoked on a non-positive (n <= 0) integer literal, like NegZInt(42)."
final val notValidNegZInt = rawNotValidNegZInt
final val rawNotLiteralNegZInt = "NegZInt.apply can only be invoked on an integer literal, like NegZInt(-42). Please use NegZInt.from instead."
final val notLiteralNegZInt = rawNotLiteralNegZInt
final val rawNotValidNegZLong = "NegZLong.apply can only be invoked on a non-positive (n <= 0L) integer literal, like NegZLong(-42L)."
final val notValidNegZLong = rawNotValidNegZLong
final val rawNotLiteralNegZLong = "NegZLong.apply can only be invoked on an integer literal, like NegZLong(-42L). Please use NegZLong.from instead."
final val notLiteralNegZLong = rawNotLiteralNegZLong
final val rawNotValidNegZFloat = "NegZFloat.apply can only be invoked on a non-positive (n <= 0.0F && !n.isNaN) floating point literal, like NegZFloat(-42.0F)."
final val notValidNegZFloat = rawNotValidNegZFloat
final val rawNotLiteralNegZFloat = "NegZFloat.apply can only be invoked on a floating point literal, like NegZFloat(-42.0F). Please use NegZFloat.from instead."
final val notLiteralNegZFloat = rawNotLiteralNegZFloat
final val rawNotValidNegZDouble = "NegZDouble.apply can only be invoked on a non-positive (n <= 0.0 && !n.isNaN) floating point literal, like NegZDouble(-42.0)."
final val notValidNegZDouble = rawNotValidNegZDouble
final val rawNotLiteralNegZDouble = "NegZDouble.apply can only be invoked on a floating point literal, like NegZDouble(-42.0). Please use NegZDouble.from instead."
final val notLiteralNegZDouble = rawNotLiteralNegZDouble
final val rawNotValidPosFiniteFloat = "PosFiniteFloat.apply can only be invoked on a finite positive (n > 0.0F && n != Float.PositiveInfinity && !n.isNaN) floating point literal, like PosFiniteFloat(42.0F)."
final val notValidPosFiniteFloat = rawNotValidPosFiniteFloat
final val rawNotLiteralPosFiniteFloat = "PosFinietFloat.apply can only be invoked on a floating point literal, like PosFiniteFloat(42.0F). Please use PosFiniteFloat.from instead."
final val notLiteralPosFiniteFloat = rawNotLiteralPosFiniteFloat
final val rawNotValidPosFiniteDouble = "PosFiniteDouble.apply can only be invoked on a finite positive (n > 0.0 && n != Double.PositiveInfinity && !n.isNaN) floating point literal, like PosFiniteDouble(42.0)."
final val notValidPosFiniteDouble = rawNotValidPosFiniteDouble
final val rawNotLiteralPosFiniteDouble = "PosFiniteDouble.apply can only be invoked on a floating point literal, like PosFiniteDouble(42.0). Please use PosFiniteDouble.from instead."
final val notLiteralPosFiniteDouble = rawNotLiteralPosFiniteDouble
final val rawNotValidPosZFiniteFloat = "PosZFiniteFloat.apply can only be invoked on a finite non-negative (n >= 0.0F && n != Float.PositiveInfinity && !n.isNaN) floating point literal, like PosZFiniteFloat(42.0F)."
final val notValidPosZFiniteFloat = rawNotValidPosZFiniteFloat
final val rawNotLiteralPosZFiniteFloat = "PosZFiniteFloat.apply can only be invoked on a floating point literal, like PosZFiniteFloat(42.0F). Please use PosZFiniteFloat.from instead."
final val notLiteralPosZFiniteFloat = rawNotLiteralPosZFiniteFloat
final val rawNotValidPosZFiniteDouble = "PosZFiniteDouble.apply can only be invoked on a finite non-negative (n >= 0.0 && n != Double.PositiveInfinity && !n.isNaN) floating point literal, like PosZFiniteDouble(42.0)."
final val notValidPosZFiniteDouble = rawNotValidPosZFiniteDouble
final val rawNotLiteralPosZFiniteDouble = "PosZFiniteDouble.apply can only be invoked on a floating point literal, like PosZFiniteDouble(42.0). Please use PosZFiniteDouble.from instead."
final val notLiteralPosZFiniteDouble = rawNotLiteralPosZFiniteDouble
final val rawNotValidNegFiniteFloat = "NegFiniteFloat.apply can only be invoked on a finite negative (n < 0.0F && n != Float.NegativeInfinity && !n.isNaN) floating point literal, like NegFiniteFloat(-42.0F)."
final val notValidNegFiniteFloat = rawNotValidNegFiniteFloat
final val rawNotLiteralNegFiniteFloat = "NegFiniteFloat.apply can only be invoked on a floating point literal, like NegFiniteFloat(-42.0F). Please use NegFiniteFloat.from instead."
final val notLiteralNegFiniteFloat = rawNotLiteralNegFiniteFloat
final val rawNotValidNegFiniteDouble = "NegFiniteDouble.apply can only be invoked on a finite negative (n < 0.0 && n != Double.NegativeInfinity && !n.isNaN) floating point literal, like NegFiniteDouble(-42.0)."
final val notValidNegFiniteDouble = rawNotValidNegFiniteDouble
final val rawNotLiteralNegFiniteDouble = "NegFiniteDouble.apply can only be invoked on a floating point literal, like NegFiniteDouble(-42.0). Please use NegFiniteDouble.from instead."
final val notLiteralNegFiniteDouble = rawNotLiteralNegFiniteDouble
final val rawNotValidNegZFiniteFloat = "NegZFiniteFloat.apply can only be invoked on a finite non-positive (n <= 0.0F && n != Float.NegativeInfinity && !n.isNaN) floating point literal, like NegZFiniteFloat(-42.0F)."
final val notValidNegZFiniteFloat = rawNotValidNegZFiniteFloat
final val rawNotLiteralNegZFiniteFloat = "NegZFiniteFloat.apply can only be invoked on a floating point literal, like NegZFiniteFloat(-42.0F). Please use NegZFiniteFloat.from instead."
final val notLiteralNegZFiniteFloat = rawNotLiteralNegZFiniteFloat
final val rawNotValidNegZFiniteDouble = "NegZFiniteDouble.apply can only be invoked on a finite non-positive (n <= 0.0 && n != Double.NegativeInfinity && !n.isNaN) floating point literal, like NegZFiniteDouble(-42.0)."
final val notValidNegZFiniteDouble = rawNotValidNegZFiniteDouble
final val rawNotLiteralNegZFiniteDouble = "NegZFiniteDouble.apply can only be invoked on a floating point literal, like NegZFiniteDouble(-42.0). Please use NegZFiniteDouble.from instead."
final val notLiteralNegZFiniteDouble = rawNotLiteralNegZFiniteDouble
final val rawNotValidNonZeroFiniteFloat = "NonZeroFiniteFloat.apply can only be invoked on a finite non-zero (n != 0.0F && n != Float.PositiveInfinity && n != Float.NegativeInfinity && !n.isNaN) floating point literal, like NonZeroFiniteFloat(1.1F)."
final val notValidNonZeroFiniteFloat = rawNotValidNonZeroFiniteFloat
final val rawNotLiteralNonZeroFiniteFloat = "NonZeroFiniteFloat.apply can only be invoked on a floating point literal, like NonZeroFloat(1.1F). Please use NonZeroFiniteFloat.from instead."
final val notLiteralNonZeroFiniteFloat = rawNotLiteralNonZeroFiniteFloat
final val rawNotValidNonZeroFiniteDouble = "NonZeroFiniteDouble.apply can only be invoked on a finite non-zero (n != 0.0 && n != Double.PositiveInfinity && n != Double.NegativeInfinity && !n.isNaN) double literal, like NonZeroFiniteDouble(42.0)."
final val notValidNonZeroFiniteDouble = rawNotValidNonZeroFiniteDouble
final val rawNotLiteralNonZeroFiniteDouble = "ZonZeroFiniteDouble.apply can only be invoked on a finite non-zero literal, like NonZeroFiniteDouble(42.0). Please use NonZeroFiniteDouble.from instead."
final val notLiteralNonZeroFiniteDouble = rawNotLiteralNonZeroFiniteDouble
final val rawNotValidFiniteFloat = "PosFiniteFloat.apply can only be invoked on a finite (n != Float.PositiveInfinity && n != Float.NegativeInfinity) floating point literal, like FiniteFloat(42.0F)."
final val notValidFiniteFloat = rawNotValidFiniteFloat
final val rawNotLiteralFiniteFloat = "PosFiniteFloat.apply can only be invoked on a floating point literal, like FiniteFloat(42.0F). Please use FiniteFloat.from instead."
final val notLiteralFiniteFloat = rawNotLiteralFiniteFloat
final val rawNotValidFiniteDouble = "PosFiniteDouble.apply can only be invoked on a finite (n != Double.PositiveInfinity && n != Double.NegativeInfinity) floating point literal, like FiniteDouble(42.0)."
final val notValidFiniteDouble = rawNotValidFiniteDouble
final val rawNotLiteralFiniteDouble = "PosFiniteDouble.apply can only be invoked on a floating point literal, like FiniteDouble(42.0). Please use FiniteDouble.from instead."
final val notLiteralFiniteDouble = rawNotLiteralFiniteDouble
final val rawNotValidNumericChar = "NumericChar.apply can only be invoked on Char literals that are numbers, like '8'."
final val notValidNumericChar = rawNotValidNumericChar
final val rawNotLiteralNumericChar = "NumericChar.apply can only be invoked on Char literals that are numbers, like '8'. Please use NumericChar.from instead."
final val notLiteralNumericChar = rawNotLiteralNumericChar
final val rawInvalidSize = "Invalid size: {0}"
def invalidSize(param0: Any): String =
rawInvalidSize.replaceAllLiterally("{0}", param0.toString())
def bigProblems(ex: Throwable): String = {
val message = if (ex.getMessage == null) "" else ex.getMessage.trim
if (message.length > 0) Resources.bigProblemsWithMessage(message) else Resources.bigProblems
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy