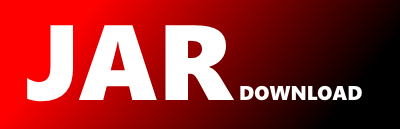
org.scalatest.Resources.scala Maven / Gradle / Ivy
The newest version!
package org.scalatest
private[scalatest] object Resources {
def formatString(rawString: String, args: Array[Any]): String = {
args.zipWithIndex.foldLeft(rawString) { case (result, (arg, idx)) =>
result.replaceAllLiterally(s"{$idx}", arg.toString())
}
}
final val rawFactExceptionWasThrown = "Exception {0} was thrown"
def factExceptionWasThrown(param0: Any): String =
rawFactExceptionWasThrown.replaceAllLiterally("{0}", param0.toString())
final val rawFactNoExceptionWasThrown = "No exception was thrown"
final val factNoExceptionWasThrown = rawFactNoExceptionWasThrown
final val rawMidSentenceFactExceptionWasThrown = "exception {0} was thrown"
def midSentenceFactExceptionWasThrown(param0: Any): String =
rawMidSentenceFactExceptionWasThrown.replaceAllLiterally("{0}", param0.toString())
final val rawMidSentenceFactNoExceptionWasThrown = "no exception was thrown"
final val midSentenceFactNoExceptionWasThrown = rawMidSentenceFactNoExceptionWasThrown
final val rawExceptionExpected = "Expected exception {0} to be thrown, but no exception was thrown"
def exceptionExpected(param0: Any): String =
rawExceptionExpected.replaceAllLiterally("{0}", param0.toString())
final val rawExpectedExceptionWasThrown = "Did not expect exception {0} to be thrown, but it was thrown"
def expectedExceptionWasThrown(param0: Any): String =
rawExpectedExceptionWasThrown.replaceAllLiterally("{0}", param0.toString())
final val rawMidSentenceExceptionExpected = "expected exception {0} to be thrown, but no exception was thrown"
def midSentenceExceptionExpected(param0: Any): String =
rawMidSentenceExceptionExpected.replaceAllLiterally("{0}", param0.toString())
final val rawMidSentenceExpectedExceptionWasThrown = "did not expect exception {0} to be thrown, but it was thrown"
def midSentenceExpectedExceptionWasThrown(param0: Any): String =
rawMidSentenceExpectedExceptionWasThrown.replaceAllLiterally("{0}", param0.toString())
final val rawNoExceptionWasThrown = "No exception was thrown."
final val noExceptionWasThrown = rawNoExceptionWasThrown
final val rawResultWas = "No exception was thrown. Instead, result was: {0}"
def resultWas(param0: Any): String =
rawResultWas.replaceAllLiterally("{0}", param0.toString())
final val rawExceptionThrown = "{0} was thrown."
def exceptionThrown(param0: Any): String =
rawExceptionThrown.replaceAllLiterally("{0}", param0.toString())
final val rawDidNotEqual = "{0} did not equal {1}"
def didNotEqual(param0: Any, param1: Any): String =
rawDidNotEqual.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWrongException = "Expected exception {0} to be thrown, but {1} was thrown"
def wrongException(param0: Any, param1: Any): String =
rawWrongException.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawMidSentenceWrongException = "expected exception {0} to be thrown, but {1} was thrown"
def midSentenceWrongException(param0: Any, param1: Any): String =
rawMidSentenceWrongException.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawAnException = "Expected no exception to be thrown, but {0} was thrown."
def anException(param0: Any): String =
rawAnException.replaceAllLiterally("{0}", param0.toString())
final val rawExceptionNotExpected = "An unexpected {0} was thrown."
def exceptionNotExpected(param0: Any): String =
rawExceptionNotExpected.replaceAllLiterally("{0}", param0.toString())
final val rawExpectedButGot = "Expected {0}, but got {1}"
def expectedButGot(param0: Any, param1: Any): String =
rawExpectedButGot.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawExpectedAndGot = "Expected {0}, and got {1}"
def expectedAndGot(param0: Any, param1: Any): String =
rawExpectedAndGot.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawMidSentenceExpectedButGot = "expected {0}, but got {1}"
def midSentenceExpectedButGot(param0: Any, param1: Any): String =
rawMidSentenceExpectedButGot.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawMidSentenceExpectedAndGot = "expected {0}, and got {1}"
def midSentenceExpectedAndGot(param0: Any, param1: Any): String =
rawMidSentenceExpectedAndGot.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawConditionFalse = "A boolean condition should have been true."
final val conditionFalse = rawConditionFalse
final val rawRefNotNull = "A reference should have been null."
final val refNotNull = rawRefNotNull
final val rawRefNull = "A reference should have been non-null."
final val refNull = rawRefNull
final val rawFloatInfinite = "A float value was infinite. Expected: {0} Actual: {1}. Delta: {2}."
def floatInfinite(param0: Any, param1: Any, param2: Any): String =
rawFloatInfinite.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawFloatNaN = "A float value was NaN. Expected: {0} Actual: {1}. Delta: {2}."
def floatNaN(param0: Any, param1: Any, param2: Any): String =
rawFloatNaN.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawDoubleInfinite = "A double value was infinite. Expected: {0} Actual: {1}. Delta: {2}."
def doubleInfinite(param0: Any, param1: Any, param2: Any): String =
rawDoubleInfinite.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawDoubleNaN = "A double value was NaN. Expected: {0} Actual: {1}. Delta: {2}."
def doubleNaN(param0: Any, param1: Any, param2: Any): String =
rawDoubleNaN.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawTestEvent = "Test Event: {0}: {1}"
def testEvent(param0: Any, param1: Any): String =
rawTestEvent.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawExpressionFailed = "{0} failed"
def expressionFailed(param0: Any): String =
rawExpressionFailed.replaceAllLiterally("{0}", param0.toString())
final val rawTestFailed = "TEST FAILED - {0}"
def testFailed(param0: Any): String =
rawTestFailed.replaceAllLiterally("{0}", param0.toString())
final val rawTestStarting = "Test Starting - {0}"
def testStarting(param0: Any): String =
rawTestStarting.replaceAllLiterally("{0}", param0.toString())
final val rawTestSucceeded = "Test Succeeded - {0}"
def testSucceeded(param0: Any): String =
rawTestSucceeded.replaceAllLiterally("{0}", param0.toString())
final val rawTestIgnored = "Test Ignored - {0}"
def testIgnored(param0: Any): String =
rawTestIgnored.replaceAllLiterally("{0}", param0.toString())
final val rawTestPending = "Test Pending - {0}"
def testPending(param0: Any): String =
rawTestPending.replaceAllLiterally("{0}", param0.toString())
final val rawTestCanceled = "Test Canceled - {0}"
def testCanceled(param0: Any): String =
rawTestCanceled.replaceAllLiterally("{0}", param0.toString())
final val rawSuiteStarting = "Suite Starting - {0}"
def suiteStarting(param0: Any): String =
rawSuiteStarting.replaceAllLiterally("{0}", param0.toString())
final val rawSuiteCompleted = "Suite Completed - {0}"
def suiteCompleted(param0: Any): String =
rawSuiteCompleted.replaceAllLiterally("{0}", param0.toString())
final val rawSuiteAborted = "SUITE ABORTED - {0}"
def suiteAborted(param0: Any): String =
rawSuiteAborted.replaceAllLiterally("{0}", param0.toString())
final val rawRunAborted = "*** RUN ABORTED ***"
final val runAborted = rawRunAborted
final val rawInfoProvided = "Info Provided - {0}"
def infoProvided(param0: Any): String =
rawInfoProvided.replaceAllLiterally("{0}", param0.toString())
final val rawAlertProvided = "Alert Provided - {0}"
def alertProvided(param0: Any): String =
rawAlertProvided.replaceAllLiterally("{0}", param0.toString())
final val rawNoteProvided = "Note Provided - {0}"
def noteProvided(param0: Any): String =
rawNoteProvided.replaceAllLiterally("{0}", param0.toString())
final val rawMarkupProvided = "Markup Provided - {0}"
def markupProvided(param0: Any): String =
rawMarkupProvided.replaceAllLiterally("{0}", param0.toString())
final val rawScopeOpened = "Scope Opened - {0}"
def scopeOpened(param0: Any): String =
rawScopeOpened.replaceAllLiterally("{0}", param0.toString())
final val rawScopeClosed = "Scope Closed - {0}"
def scopeClosed(param0: Any): String =
rawScopeClosed.replaceAllLiterally("{0}", param0.toString())
final val rawScopePending = "Scope Pending - {0}"
def scopePending(param0: Any): String =
rawScopePending.replaceAllLiterally("{0}", param0.toString())
final val rawPayloadToString = "payload.get.toString: {0} "
def payloadToString(param0: Any): String =
rawPayloadToString.replaceAllLiterally("{0}", param0.toString())
final val rawNoNameSpecified = "(No name specified)"
final val noNameSpecified = rawNoNameSpecified
final val rawRunStarting = "Run starting. Expected test count is: {0}"
def runStarting(param0: Any): String =
rawRunStarting.replaceAllLiterally("{0}", param0.toString())
final val rawRerunStarting = "Rerun starting. Expected test count is: {0}"
def rerunStarting(param0: Any): String =
rawRerunStarting.replaceAllLiterally("{0}", param0.toString())
final val rawRerunCompleted = "Rerun completed. Total number of tests run was: {0}"
def rerunCompleted(param0: Any): String =
rawRerunCompleted.replaceAllLiterally("{0}", param0.toString())
final val rawRerunStopped = "Rerun stopped. Total number of tests run was: {0}"
def rerunStopped(param0: Any): String =
rawRerunStopped.replaceAllLiterally("{0}", param0.toString())
final val rawFriendlyFailure = "Invalid option given to Runner.\njava org.suiterunner.Runner [option1 [option2..]] [suite1 [suite2...]]\n Valid options are:\n -g display graphical user interface\n -o print results to standard output\n -e print results to standard error\n -f print results to file\n -r pass test events to reporter"
final val friendlyFailure = rawFriendlyFailure
final val rawShowStackTraceOption = "Show Stack Traces"
final val showStackTraceOption = rawShowStackTraceOption
final val rawSuitebeforeclass = "Suite class names must appear after reporters."
final val suitebeforeclass = rawSuitebeforeclass
final val rawReportTestsStarting = "Report Tests Starting"
final val reportTestsStarting = rawReportTestsStarting
final val rawReportTestsSucceeded = "Report Test Success"
final val reportTestsSucceeded = rawReportTestsSucceeded
final val rawReportTestsFailed = "Report Test Failed"
final val reportTestsFailed = rawReportTestsFailed
final val rawReportAlerts = "Report Alerts"
final val reportAlerts = rawReportAlerts
final val rawReportInfo = "Report Miscellaneous Information Messages"
final val reportInfo = rawReportInfo
final val rawReportStackTraces = "Include Stack Traces in Reports"
final val reportStackTraces = rawReportStackTraces
final val rawReportRunStarting = "Report Run Starting"
final val reportRunStarting = rawReportRunStarting
final val rawReportRunCompleted = "Report Run Completed"
final val reportRunCompleted = rawReportRunCompleted
final val rawReportSummary = "Show A Summary of Results"
final val reportSummary = rawReportSummary
final val rawProbarg = "Problem arg: {0}"
def probarg(param0: Any): String =
rawProbarg.replaceAllLiterally("{0}", param0.toString())
final val rawErrBuildingDispatchReporter = "Error preparing reporters."
final val errBuildingDispatchReporter = rawErrBuildingDispatchReporter
final val rawMissingFileName = "A -f option must be followed by an output file name."
final val missingFileName = rawMissingFileName
final val rawMissingReporterClassName = "A -r option must be followed by a Reporter class name."
final val missingReporterClassName = rawMissingReporterClassName
final val rawErrParsingArgs = "Error parsing command line arguments."
final val errParsingArgs = rawErrParsingArgs
final val rawInvalidConfigOption = "Invalid configuration option: {0}"
def invalidConfigOption(param0: Any): String =
rawInvalidConfigOption.replaceAllLiterally("{0}", param0.toString())
final val rawCantOpenFile = "Unable to create a PrintReporter that prints reports to a file."
final val cantOpenFile = rawCantOpenFile
final val rawReporterThrew = "Reporter completed abruptly with an exception after receiving event: {0}."
def reporterThrew(param0: Any): String =
rawReporterThrew.replaceAllLiterally("{0}", param0.toString())
final val rawReporterDisposeThrew = "Reporter completed abruptly with an exception on invocation of the dispose method."
final val reporterDisposeThrew = rawReporterDisposeThrew
final val rawSlowpokeDetectorEventNotFound = "The \"Slowpoke\" detector received a test-finished event for which it had not seen a matching test-starting event: suiteName = {0}, suiteId = {1}, testName = {2}."
def slowpokeDetectorEventNotFound(param0: Any, param1: Any, param2: Any): String =
rawSlowpokeDetectorEventNotFound.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawSuiteExecutionStarting = "The run method of a nested suite is about to be invoked."
final val suiteExecutionStarting = rawSuiteExecutionStarting
final val rawExecuteException = "Exception encountered when invoking run on a nested suite."
final val executeException = rawExecuteException
final val rawExecuteExceptionWithMessage = "Exception encountered when invoking run on a nested suite - {0}"
def executeExceptionWithMessage(param0: Any): String =
rawExecuteExceptionWithMessage.replaceAllLiterally("{0}", param0.toString())
final val rawRunOnSuiteException = "Exception encountered when invoking run on a suite."
final val runOnSuiteException = rawRunOnSuiteException
final val rawRunOnSuiteExceptionWithMessage = "Exception encountered when invoking run on a suite - {0}"
def runOnSuiteExceptionWithMessage(param0: Any): String =
rawRunOnSuiteExceptionWithMessage.replaceAllLiterally("{0}", param0.toString())
final val rawSuiteCompletedNormally = "The run method of a nested suite returned normally."
final val suiteCompletedNormally = rawSuiteCompletedNormally
final val rawNotOneOfTheChosenStyles = "{0} is not one of the chosen styles, which are: {1}. For information on chosen styles, see the Scaladoc documentation for org.scalatest.tools.Runner."
def notOneOfTheChosenStyles(param0: Any, param1: Any): String =
rawNotOneOfTheChosenStyles.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawNotTheChosenStyle = "{0} is not the chosen style, which is: {1}. For information on chosen styles, see the Scaladoc documentation for org.scalatest.tools.Runner."
def notTheChosenStyle(param0: Any, param1: Any): String =
rawNotTheChosenStyle.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawRerun = "Rerun"
final val Rerun = rawRerun
final val rawExecuteStopping = "The run method of a Suite is returning because a stop was requested."
final val executeStopping = rawExecuteStopping
final val rawIllegalReporterArg = "An illegal reporter argument was specified on the command line: \"{0}\"."
def illegalReporterArg(param0: Any): String =
rawIllegalReporterArg.replaceAllLiterally("{0}", param0.toString())
final val rawCantLoadReporterClass = "Couldn't load a Reporter class: \"{0}\"."
def cantLoadReporterClass(param0: Any): String =
rawCantLoadReporterClass.replaceAllLiterally("{0}", param0.toString())
final val rawCantInstantiateReporter = "Couldn't instantiate a Reporter class: \"{0}\". Is the class public with a public no-arg constructor?"
def cantInstantiateReporter(param0: Any): String =
rawCantInstantiateReporter.replaceAllLiterally("{0}", param0.toString())
final val rawOverwriteExistingFile = "The file \"{0}\" already exists in this directory. Replace it?"
def overwriteExistingFile(param0: Any): String =
rawOverwriteExistingFile.replaceAllLiterally("{0}", param0.toString())
final val rawCannotLoadSuite = "Unable to load a Suite class. This could be due to an error in your runpath. Missing class: {0}"
def cannotLoadSuite(param0: Any): String =
rawCannotLoadSuite.replaceAllLiterally("{0}", param0.toString())
final val rawCannotLoadDiscoveredSuite = "Unable to load a Suite class {0} that was discovered in the runpath: {1}"
def cannotLoadDiscoveredSuite(param0: Any, param1: Any): String =
rawCannotLoadDiscoveredSuite.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawNonSuite = "One or more requested classes are not Suites: "
final val nonSuite = rawNonSuite
final val rawCannotInstantiateSuite = "Unable to instantiate a Suite class. Is each Suite class you specified public, with a public no-arg constuctor? Suite class name: {0}"
def cannotInstantiateSuite(param0: Any): String =
rawCannotInstantiateSuite.replaceAllLiterally("{0}", param0.toString())
final val rawCannotLoadClass = "A needed class was not found. This could be due to an error in your runpath. Missing class: {0}"
def cannotLoadClass(param0: Any): String =
rawCannotLoadClass.replaceAllLiterally("{0}", param0.toString())
final val rawBigProblems = "An exception or error caused a run to abort. "
final val bigProblems = rawBigProblems
final val rawBigProblemsWithMessage = "An exception or error caused a run to abort: {0} "
def bigProblemsWithMessage(param0: Any): String =
rawBigProblemsWithMessage.replaceAllLiterally("{0}", param0.toString())
final val rawBigProblemsMaybeCustomReporter = "An exception or error caused a run to abort. This may have been caused by a problematic custom reporter."
final val bigProblemsMaybeCustomReporter = rawBigProblemsMaybeCustomReporter
final val rawCannotFindMethod = "The Suite to rerun does not contain the method to rerun. Method name: {0}"
def cannotFindMethod(param0: Any): String =
rawCannotFindMethod.replaceAllLiterally("{0}", param0.toString())
final val rawSecurityWhenRerunning = "A SecurityException was thrown when attempting a rerun: {0}"
def securityWhenRerunning(param0: Any): String =
rawSecurityWhenRerunning.replaceAllLiterally("{0}", param0.toString())
final val rawOverwriteDialogTitle = "Save"
final val overwriteDialogTitle = rawOverwriteDialogTitle
final val rawOpenPrefs = "Open Recipe"
final val openPrefs = rawOpenPrefs
final val rawSavePrefs = "Save Recipe"
final val savePrefs = rawSavePrefs
final val rawRunsFailures = "Runs and Failures"
final val runsFailures = rawRunsFailures
final val rawAllEvents = "All Events"
final val allEvents = rawAllEvents
final val rawNeedFileNameTitle = "Edit Reporter Configuration"
final val needFileNameTitle = rawNeedFileNameTitle
final val rawNeedFileNameMessage = "A file name is required to create a File Reporter. Please supply a valid file name."
final val needFileNameMessage = rawNeedFileNameMessage
final val rawNeedClassNameTitle = "Edit Reporter Configuration"
final val needClassNameTitle = rawNeedClassNameTitle
final val rawNeedClassNameMessage = "A Reporter class name is required to create a Custom Reporter. Please supply a fully qualified name of a class that implements org.suiterunner.Reporter."
final val needClassNameMessage = rawNeedClassNameMessage
final val rawNoSuitesFoundText = "No Suites found in the runpath"
final val NoSuitesFoundText = rawNoSuitesFoundText
final val rawCantInvokeExceptionText = "Can't invoke method"
final val cantInvokeExceptionText = rawCantInvokeExceptionText
final val rawMultipleTestsFailed = "*** {0} TESTS FAILED ***"
def multipleTestsFailed(param0: Any): String =
rawMultipleTestsFailed.replaceAllLiterally("{0}", param0.toString())
final val rawOneTestFailed = "*** 1 TEST FAILED ***"
final val oneTestFailed = rawOneTestFailed
final val rawOneSuiteAborted = "*** 1 SUITE ABORTED ***"
final val oneSuiteAborted = rawOneSuiteAborted
final val rawMultipleSuitesAborted = "*** {0} SUITES ABORTED ***"
def multipleSuitesAborted(param0: Any): String =
rawMultipleSuitesAborted.replaceAllLiterally("{0}", param0.toString())
final val rawAllTestsPassed = "All tests passed."
final val allTestsPassed = rawAllTestsPassed
final val rawNoTestsWereExecuted = "No tests were executed."
final val noTestsWereExecuted = rawNoTestsWereExecuted
final val rawEventsLabel = "Events:"
final val eventsLabel = rawEventsLabel
final val rawDetailsLabel = "Details:"
final val detailsLabel = rawDetailsLabel
final val rawTestsRun = "Tests Run:"
final val testsRun = rawTestsRun
final val rawTestsFailed = "Failed:"
final val testsFailed = rawTestsFailed
final val rawTestsExpected = "Expected:"
final val testsExpected = rawTestsExpected
final val rawTestsIgnored = "Ignored:"
final val testsIgnored = rawTestsIgnored
final val rawTestsPending = "Pending:"
final val testsPending = rawTestsPending
final val rawTestsCanceled = "Canceled:"
final val testsCanceled = rawTestsCanceled
final val rawScalaTestTitle = "ScalaTest"
final val ScalaTestTitle = rawScalaTestTitle
final val rawScalaTestMenu = "ScalaTest"
final val ScalaTestMenu = rawScalaTestMenu
final val rawRun = "Run"
final val Run = rawRun
final val rawStop = "Stop"
final val Stop = rawStop
final val rawExit = "Exit"
final val Exit = rawExit
final val rawAbout = "About..."
final val About = rawAbout
final val rawAboutBoxTitle = "About ScalaTest"
final val AboutBoxTitle = rawAboutBoxTitle
final val rawAppName = "ScalaTest"
final val AppName = rawAppName
final val rawAppCopyright = "Copyright (C) 2001-2014 Artima, Inc. All rights reserved."
final val AppCopyright = rawAppCopyright
final val rawAppURL = "www.scalatest.org"
final val AppURL = rawAppURL
final val rawReason = "A tool for testing Scala and Java software"
final val Reason = rawReason
final val rawTrademarks = "ScalaTest is a trademark of Artima, Inc."
final val Trademarks = rawTrademarks
final val rawArtimaInc = "Artima, Inc."
final val ArtimaInc = rawArtimaInc
final val rawMoreInfo = "For more information, visit:"
final val MoreInfo = rawMoreInfo
final val rawViewMenu = "View"
final val ViewMenu = rawViewMenu
final val rawJavaSuiteRunnerFile = "srj"
final val JavaSuiteRunnerFile = rawJavaSuiteRunnerFile
final val rawJavaSuiteRunnerFileDescription = "Recipe Files (*.srj)"
final val JavaSuiteRunnerFileDescription = rawJavaSuiteRunnerFileDescription
final val rawDefaultConfiguration = "default"
final val defaultConfiguration = rawDefaultConfiguration
final val rawReporterTypeLabel = "Reporter Type:"
final val reporterTypeLabel = rawReporterTypeLabel
final val rawGraphicReporterType = "Graphic Reporter"
final val graphicReporterType = rawGraphicReporterType
final val rawCustomReporterType = "Custom Reporter"
final val customReporterType = rawCustomReporterType
final val rawStdoutReporterType = "Standard Output Reporter"
final val stdoutReporterType = rawStdoutReporterType
final val rawStderrReporterType = "Standard Error Reporter"
final val stderrReporterType = rawStderrReporterType
final val rawFileReporterType = "File Reporter"
final val fileReporterType = rawFileReporterType
final val rawReporterConfigLabel = "Reporter Configuration: {0}"
def reporterConfigLabel(param0: Any): String =
rawReporterConfigLabel.replaceAllLiterally("{0}", param0.toString())
final val rawUnusedField = "Field for Custom and File Reporters:"
final val unusedField = rawUnusedField
final val rawCouldntRun = "Couldn't Run"
final val couldntRun = rawCouldntRun
final val rawCouldntRerun = "Couldn't Rerun"
final val couldntRerun = rawCouldntRerun
final val rawMENU_PRESENT_DISCOVERY_STARTING = "Discovery Starting Reports"
final val MENU_PRESENT_DISCOVERY_STARTING = rawMENU_PRESENT_DISCOVERY_STARTING
final val rawMENU_PRESENT_DISCOVERY_COMPLETED = "Discovery Completed Reports"
final val MENU_PRESENT_DISCOVERY_COMPLETED = rawMENU_PRESENT_DISCOVERY_COMPLETED
final val rawMENU_PRESENT_RUN_STARTING = "Run Starting Reports"
final val MENU_PRESENT_RUN_STARTING = rawMENU_PRESENT_RUN_STARTING
final val rawMENU_PRESENT_TEST_STARTING = "Test Starting Reports"
final val MENU_PRESENT_TEST_STARTING = rawMENU_PRESENT_TEST_STARTING
final val rawMENU_PRESENT_TEST_FAILED = "Test Failed Reports"
final val MENU_PRESENT_TEST_FAILED = rawMENU_PRESENT_TEST_FAILED
final val rawMENU_PRESENT_TEST_SUCCEEDED = "Test Succeeded Reports"
final val MENU_PRESENT_TEST_SUCCEEDED = rawMENU_PRESENT_TEST_SUCCEEDED
final val rawMENU_PRESENT_TEST_IGNORED = "Test Ignored Reports"
final val MENU_PRESENT_TEST_IGNORED = rawMENU_PRESENT_TEST_IGNORED
final val rawMENU_PRESENT_TEST_PENDING = "Test Pending Reports"
final val MENU_PRESENT_TEST_PENDING = rawMENU_PRESENT_TEST_PENDING
final val rawMENU_PRESENT_TEST_CANCELED = "Test Canceled Reports"
final val MENU_PRESENT_TEST_CANCELED = rawMENU_PRESENT_TEST_CANCELED
final val rawMENU_PRESENT_SUITE_STARTING = "Suite Starting reports"
final val MENU_PRESENT_SUITE_STARTING = rawMENU_PRESENT_SUITE_STARTING
final val rawMENU_PRESENT_SUITE_ABORTED = "Suite Aborted Reports"
final val MENU_PRESENT_SUITE_ABORTED = rawMENU_PRESENT_SUITE_ABORTED
final val rawMENU_PRESENT_SUITE_COMPLETED = "Suite Completed Reports"
final val MENU_PRESENT_SUITE_COMPLETED = rawMENU_PRESENT_SUITE_COMPLETED
final val rawMENU_PRESENT_INFO_PROVIDED = "Information Provided Reports"
final val MENU_PRESENT_INFO_PROVIDED = rawMENU_PRESENT_INFO_PROVIDED
final val rawMENU_PRESENT_ALERT_PROVIDED = "Alert Reports"
final val MENU_PRESENT_ALERT_PROVIDED = rawMENU_PRESENT_ALERT_PROVIDED
final val rawMENU_PRESENT_NOTE_PROVIDED = "Note Reports"
final val MENU_PRESENT_NOTE_PROVIDED = rawMENU_PRESENT_NOTE_PROVIDED
final val rawMENU_PRESENT_SCOPE_OPENED = "Scope Opened Reports"
final val MENU_PRESENT_SCOPE_OPENED = rawMENU_PRESENT_SCOPE_OPENED
final val rawMENU_PRESENT_SCOPE_CLOSED = "Scope Closed Reports"
final val MENU_PRESENT_SCOPE_CLOSED = rawMENU_PRESENT_SCOPE_CLOSED
final val rawMENU_PRESENT_SCOPE_PENDING = "Scope Pending Reports"
final val MENU_PRESENT_SCOPE_PENDING = rawMENU_PRESENT_SCOPE_PENDING
final val rawMENU_PRESENT_MARKUP_PROVIDED = "Markup Provided Reports"
final val MENU_PRESENT_MARKUP_PROVIDED = rawMENU_PRESENT_MARKUP_PROVIDED
final val rawMENU_PRESENT_RUN_STOPPED = "Run Stopped Reports"
final val MENU_PRESENT_RUN_STOPPED = rawMENU_PRESENT_RUN_STOPPED
final val rawMENU_PRESENT_RUN_ABORTED = "Run Aborted Reports"
final val MENU_PRESENT_RUN_ABORTED = rawMENU_PRESENT_RUN_ABORTED
final val rawMENU_PRESENT_RUN_COMPLETED = "Run Completed Reports"
final val MENU_PRESENT_RUN_COMPLETED = rawMENU_PRESENT_RUN_COMPLETED
final val rawRUN_STARTING = "Run Starting"
final val RUN_STARTING = rawRUN_STARTING
final val rawTEST_STARTING = "Test Starting"
final val TEST_STARTING = rawTEST_STARTING
final val rawTEST_FAILED = "Test Failed"
final val TEST_FAILED = rawTEST_FAILED
final val rawTEST_SUCCEEDED = "Test Succeeded"
final val TEST_SUCCEEDED = rawTEST_SUCCEEDED
final val rawTEST_IGNORED = "Test Ignored"
final val TEST_IGNORED = rawTEST_IGNORED
final val rawTEST_PENDING = "Test Pending"
final val TEST_PENDING = rawTEST_PENDING
final val rawTEST_CANCELED = "Test Canceled"
final val TEST_CANCELED = rawTEST_CANCELED
final val rawSUITE_STARTING = "Suite Starting"
final val SUITE_STARTING = rawSUITE_STARTING
final val rawSUITE_ABORTED = "Suite Aborted"
final val SUITE_ABORTED = rawSUITE_ABORTED
final val rawSUITE_COMPLETED = "Suite Completed"
final val SUITE_COMPLETED = rawSUITE_COMPLETED
final val rawINFO_PROVIDED = "Info Provided"
final val INFO_PROVIDED = rawINFO_PROVIDED
final val rawALERT_PROVIDED = "Alert Provided"
final val ALERT_PROVIDED = rawALERT_PROVIDED
final val rawNOTE_PROVIDED = "Note Provided"
final val NOTE_PROVIDED = rawNOTE_PROVIDED
final val rawSCOPE_OPENED = "Scope Opened"
final val SCOPE_OPENED = rawSCOPE_OPENED
final val rawSCOPE_CLOSED = "Scope Closed"
final val SCOPE_CLOSED = rawSCOPE_CLOSED
final val rawSCOPE_PENDING = "Scope Pending"
final val SCOPE_PENDING = rawSCOPE_PENDING
final val rawMARKUP_PROVIDED = "Markup Provided"
final val MARKUP_PROVIDED = rawMARKUP_PROVIDED
final val rawRUN_STOPPED = "Run Stopped"
final val RUN_STOPPED = rawRUN_STOPPED
final val rawRUN_ABORTED = "Run Aborted"
final val RUN_ABORTED = rawRUN_ABORTED
final val rawRUN_COMPLETED = "Run Completed"
final val RUN_COMPLETED = rawRUN_COMPLETED
final val rawDISCOVERY_STARTING = "Discovery Starting"
final val DISCOVERY_STARTING = rawDISCOVERY_STARTING
final val rawDISCOVERY_COMPLETED = "Discovery Completed"
final val DISCOVERY_COMPLETED = rawDISCOVERY_COMPLETED
final val rawRERUN_DISCOVERY_STARTING = "Rerun Discovery Starting"
final val RERUN_DISCOVERY_STARTING = rawRERUN_DISCOVERY_STARTING
final val rawRERUN_DISCOVERY_COMPLETED = "Rerun Discovery Completed"
final val RERUN_DISCOVERY_COMPLETED = rawRERUN_DISCOVERY_COMPLETED
final val rawRERUN_RUN_STARTING = "Rerun Starting"
final val RERUN_RUN_STARTING = rawRERUN_RUN_STARTING
final val rawRERUN_TEST_STARTING = "Rerun Test Starting"
final val RERUN_TEST_STARTING = rawRERUN_TEST_STARTING
final val rawRERUN_TEST_FAILED = "Rerun Test Failed"
final val RERUN_TEST_FAILED = rawRERUN_TEST_FAILED
final val rawRERUN_TEST_SUCCEEDED = "Rerun Test Succeeded"
final val RERUN_TEST_SUCCEEDED = rawRERUN_TEST_SUCCEEDED
final val rawRERUN_TEST_IGNORED = "Rerun Test Ignored"
final val RERUN_TEST_IGNORED = rawRERUN_TEST_IGNORED
final val rawRERUN_TEST_PENDING = "Rerun Test Pending"
final val RERUN_TEST_PENDING = rawRERUN_TEST_PENDING
final val rawRERUN_TEST_CANCELED = "Rerun Test Canceled"
final val RERUN_TEST_CANCELED = rawRERUN_TEST_CANCELED
final val rawRERUN_SUITE_STARTING = "Rerun Suite Starting"
final val RERUN_SUITE_STARTING = rawRERUN_SUITE_STARTING
final val rawRERUN_SUITE_ABORTED = "Rerun Suite Aborted"
final val RERUN_SUITE_ABORTED = rawRERUN_SUITE_ABORTED
final val rawRERUN_SUITE_COMPLETED = "Rerun Suite Completed"
final val RERUN_SUITE_COMPLETED = rawRERUN_SUITE_COMPLETED
final val rawRERUN_INFO_PROVIDED = "Rerun Info Provided"
final val RERUN_INFO_PROVIDED = rawRERUN_INFO_PROVIDED
final val rawRERUN_ALERT_PROVIDED = "Rerun Alert Provided"
final val RERUN_ALERT_PROVIDED = rawRERUN_ALERT_PROVIDED
final val rawRERUN_NOTE_PROVIDED = "Rerun Note Provided"
final val RERUN_NOTE_PROVIDED = rawRERUN_NOTE_PROVIDED
final val rawRERUN_MARKUP_PROVIDED = "Rerun Markup Provided"
final val RERUN_MARKUP_PROVIDED = rawRERUN_MARKUP_PROVIDED
final val rawRERUN_RUN_STOPPED = "Rerun Stopped"
final val RERUN_RUN_STOPPED = rawRERUN_RUN_STOPPED
final val rawRERUN_RUN_ABORTED = "Rerun Aborted"
final val RERUN_RUN_ABORTED = rawRERUN_RUN_ABORTED
final val rawRERUN_RUN_COMPLETED = "Rerun Completed"
final val RERUN_RUN_COMPLETED = rawRERUN_RUN_COMPLETED
final val rawRERUN_SCOPE_OPENED = "Rerun Scope Opened"
final val RERUN_SCOPE_OPENED = rawRERUN_SCOPE_OPENED
final val rawRERUN_SCOPE_CLOSED = "Rerun Scope Closed"
final val RERUN_SCOPE_CLOSED = rawRERUN_SCOPE_CLOSED
final val rawRERUN_SCOPE_PENDING = "Rerun Scope Pending"
final val RERUN_SCOPE_PENDING = rawRERUN_SCOPE_PENDING
final val rawDetailsEvent = "Event"
final val DetailsEvent = rawDetailsEvent
final val rawDetailsSuiteId = "Suite ID"
final val DetailsSuiteId = rawDetailsSuiteId
final val rawDetailsName = "Name"
final val DetailsName = rawDetailsName
final val rawDetailsMessage = "Message"
final val DetailsMessage = rawDetailsMessage
final val rawLineNumber = "Line"
final val LineNumber = rawLineNumber
final val rawDetailsDate = "Date"
final val DetailsDate = rawDetailsDate
final val rawDetailsThread = "Thread"
final val DetailsThread = rawDetailsThread
final val rawDetailsThrowable = "Exception"
final val DetailsThrowable = rawDetailsThrowable
final val rawDetailsCause = "Cause"
final val DetailsCause = rawDetailsCause
final val rawNone = "None"
final val None = rawNone
final val rawDetailsDuration = "Duration"
final val DetailsDuration = rawDetailsDuration
final val rawDetailsSummary = "Summary"
final val DetailsSummary = rawDetailsSummary
final val rawShould = "should {0}"
def should(param0: Any): String =
rawShould.replaceAllLiterally("{0}", param0.toString())
final val rawItShould = "it should {0}"
def itShould(param0: Any): String =
rawItShould.replaceAllLiterally("{0}", param0.toString())
final val rawPrefixSuffix = "{0} {1}"
def prefixSuffix(param0: Any, param1: Any): String =
rawPrefixSuffix.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawPrefixShouldSuffix = "{0} should {1}"
def prefixShouldSuffix(param0: Any, param1: Any): String =
rawPrefixShouldSuffix.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawTestSucceededIconChar = "-"
final val testSucceededIconChar = rawTestSucceededIconChar
final val rawTestFailedIconChar = "-"
final val testFailedIconChar = rawTestFailedIconChar
final val rawIconPlusShortName = "{0} {1}"
def iconPlusShortName(param0: Any, param1: Any): String =
rawIconPlusShortName.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawIconPlusShortNameAndNote = "{0} {1} {2}"
def iconPlusShortNameAndNote(param0: Any, param1: Any, param2: Any): String =
rawIconPlusShortNameAndNote.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawInfoProvidedIconChar = "+"
final val infoProvidedIconChar = rawInfoProvidedIconChar
final val rawMarkupProvidedIconChar = "+"
final val markupProvidedIconChar = rawMarkupProvidedIconChar
final val rawFailedNote = "*** FAILED ***"
final val failedNote = rawFailedNote
final val rawAbortedNote = "*** ABORTED ***"
final val abortedNote = rawAbortedNote
final val rawSpecTextAndNote = "{0} {1}"
def specTextAndNote(param0: Any, param1: Any): String =
rawSpecTextAndNote.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawIgnoredNote = "!!! IGNORED !!!"
final val ignoredNote = rawIgnoredNote
final val rawPendingNote = "(pending)"
final val pendingNote = rawPendingNote
final val rawCanceledNote = "!!! CANCELED !!!"
final val canceledNote = rawCanceledNote
final val rawInfoProvidedNote = ""
final val infoProvidedNote = rawInfoProvidedNote
final val rawAlertProvidedNote = ""
final val alertProvidedNote = rawAlertProvidedNote
final val rawNoteProvidedNote = ""
final val noteProvidedNote = rawNoteProvidedNote
final val rawScopeOpenedNote = ""
final val scopeOpenedNote = rawScopeOpenedNote
final val rawScopeClosedNote = ""
final val scopeClosedNote = rawScopeClosedNote
final val rawGivenMessage = "Given {0}"
def givenMessage(param0: Any): String =
rawGivenMessage.replaceAllLiterally("{0}", param0.toString())
final val rawWhenMessage = "When {0}"
def whenMessage(param0: Any): String =
rawWhenMessage.replaceAllLiterally("{0}", param0.toString())
final val rawThenMessage = "Then {0}"
def thenMessage(param0: Any): String =
rawThenMessage.replaceAllLiterally("{0}", param0.toString())
final val rawAndMessage = "And {0}"
def andMessage(param0: Any): String =
rawAndMessage.replaceAllLiterally("{0}", param0.toString())
final val rawScenario = "Scenario: {0}"
def scenario(param0: Any): String =
rawScenario.replaceAllLiterally("{0}", param0.toString())
final val rawCommaBut = "{0}, but {1}"
def commaBut(param0: Any, param1: Any): String =
rawCommaBut.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawCommaAnd = "{0}, and {1}"
def commaAnd(param0: Any, param1: Any): String =
rawCommaAnd.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawCommaDoubleAmpersand = "({0}) && ({1})"
def commaDoubleAmpersand(param0: Any, param1: Any): String =
rawCommaDoubleAmpersand.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawCommaDoublePipe = "({0}) || ({1})"
def commaDoublePipe(param0: Any, param1: Any): String =
rawCommaDoublePipe.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawUnaryBang = "!({0})"
def unaryBang(param0: Any): String =
rawUnaryBang.replaceAllLiterally("{0}", param0.toString())
final val rawEqualed = "{0} equaled {1}"
def equaled(param0: Any, param1: Any): String =
rawEqualed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWas = "{0} was {1}"
def was(param0: Any, param1: Any): String =
rawWas.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNot = "{0} was not {1}"
def wasNot(param0: Any, param1: Any): String =
rawWasNot.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasA = "{0} was a {1}"
def wasA(param0: Any, param1: Any): String =
rawWasA.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotA = "{0} was not a {1}"
def wasNotA(param0: Any, param1: Any): String =
rawWasNotA.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasAn = "{0} was an {1}"
def wasAn(param0: Any, param1: Any): String =
rawWasAn.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotAn = "{0} was not an {1}"
def wasNotAn(param0: Any, param1: Any): String =
rawWasNotAn.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasDefinedAt = "{0} was defined at {1}"
def wasDefinedAt(param0: Any, param1: Any): String =
rawWasDefinedAt.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotDefinedAt = "{0} was not defined at {1}"
def wasNotDefinedAt(param0: Any, param1: Any): String =
rawWasNotDefinedAt.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawEqualedPlusOrMinus = "{0} equaled {1} plus or minus {2}"
def equaledPlusOrMinus(param0: Any, param1: Any, param2: Any): String =
rawEqualedPlusOrMinus.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawDidNotEqualPlusOrMinus = "{0} did not equal {1} plus or minus {2}"
def didNotEqualPlusOrMinus(param0: Any, param1: Any, param2: Any): String =
rawDidNotEqualPlusOrMinus.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawWasPlusOrMinus = "{0} was {1} plus or minus {2}"
def wasPlusOrMinus(param0: Any, param1: Any, param2: Any): String =
rawWasPlusOrMinus.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawWasNotPlusOrMinus = "{0} was not {1} plus or minus {2}"
def wasNotPlusOrMinus(param0: Any, param1: Any, param2: Any): String =
rawWasNotPlusOrMinus.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawWasLessThan = "{0} was less than {1}"
def wasLessThan(param0: Any, param1: Any): String =
rawWasLessThan.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotLessThan = "{0} was not less than {1}"
def wasNotLessThan(param0: Any, param1: Any): String =
rawWasNotLessThan.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasGreaterThan = "{0} was greater than {1}"
def wasGreaterThan(param0: Any, param1: Any): String =
rawWasGreaterThan.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotGreaterThan = "{0} was not greater than {1}"
def wasNotGreaterThan(param0: Any, param1: Any): String =
rawWasNotGreaterThan.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasLessThanOrEqualTo = "{0} was less than or equal to {1}"
def wasLessThanOrEqualTo(param0: Any, param1: Any): String =
rawWasLessThanOrEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotLessThanOrEqualTo = "{0} was not less than or equal to {1}"
def wasNotLessThanOrEqualTo(param0: Any, param1: Any): String =
rawWasNotLessThanOrEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasGreaterThanOrEqualTo = "{0} was greater than or equal to {1}"
def wasGreaterThanOrEqualTo(param0: Any, param1: Any): String =
rawWasGreaterThanOrEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotGreaterThanOrEqualTo = "{0} was not greater than or equal to {1}"
def wasNotGreaterThanOrEqualTo(param0: Any, param1: Any): String =
rawWasNotGreaterThanOrEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasSameInstanceAs = "{0} was the same instance as {1}"
def wasSameInstanceAs(param0: Any, param1: Any): String =
rawWasSameInstanceAs.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotSameInstanceAs = "{0} was not the same instance as {1}"
def wasNotSameInstanceAs(param0: Any, param1: Any): String =
rawWasNotSameInstanceAs.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawBooleanExpressionWas = "the boolean expression was {0}"
def booleanExpressionWas(param0: Any): String =
rawBooleanExpressionWas.replaceAllLiterally("{0}", param0.toString())
final val rawBooleanExpressionWasNot = "the boolean expression was not {0}"
def booleanExpressionWasNot(param0: Any): String =
rawBooleanExpressionWasNot.replaceAllLiterally("{0}", param0.toString())
final val rawWasAnInstanceOf = "{0} was an instance of {1}"
def wasAnInstanceOf(param0: Any, param1: Any): String =
rawWasAnInstanceOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotAnInstanceOf = "{0} was not an instance of {1}, but an instance of {2}"
def wasNotAnInstanceOf(param0: Any, param1: Any, param2: Any): String =
rawWasNotAnInstanceOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawWasEmpty = "{0} was empty"
def wasEmpty(param0: Any): String =
rawWasEmpty.replaceAllLiterally("{0}", param0.toString())
final val rawWasNotEmpty = "{0} was not empty"
def wasNotEmpty(param0: Any): String =
rawWasNotEmpty.replaceAllLiterally("{0}", param0.toString())
final val rawWasNull = "The reference was null"
final val wasNull = rawWasNull
final val rawMidSentenceWasNull = "the reference was null"
final val midSentenceWasNull = rawMidSentenceWasNull
final val rawWasNotNull = "{0} was not null"
def wasNotNull(param0: Any): String =
rawWasNotNull.replaceAllLiterally("{0}", param0.toString())
final val rawEqualedNull = "The reference equaled null"
final val equaledNull = rawEqualedNull
final val rawMidSentenceEqualedNull = "the reference equaled null"
final val midSentenceEqualedNull = rawMidSentenceEqualedNull
final val rawDidNotEqualNull = "{0} did not equal null"
def didNotEqualNull(param0: Any): String =
rawDidNotEqualNull.replaceAllLiterally("{0}", param0.toString())
final val rawWasNone = "{0} was None"
def wasNone(param0: Any): String =
rawWasNone.replaceAllLiterally("{0}", param0.toString())
final val rawWasNotNone = "{0} was not None"
def wasNotNone(param0: Any): String =
rawWasNotNone.replaceAllLiterally("{0}", param0.toString())
final val rawWasNil = "{0} was Nil"
def wasNil(param0: Any): String =
rawWasNil.replaceAllLiterally("{0}", param0.toString())
final val rawWasNotNil = "{0} was not Nil"
def wasNotNil(param0: Any): String =
rawWasNotNil.replaceAllLiterally("{0}", param0.toString())
final val rawWasSome = "{0} was equal to Some({1})"
def wasSome(param0: Any, param1: Any): String =
rawWasSome.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotSome = "{0} was not equal to Some({1})"
def wasNotSome(param0: Any, param1: Any): String =
rawWasNotSome.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHasNeitherAOrAnMethod = "{0} has neither a {1} nor an {2} method"
def hasNeitherAOrAnMethod(param0: Any, param1: Any, param2: Any): String =
rawHasNeitherAOrAnMethod.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawHasNeitherAnOrAnMethod = "{0} has neither an {1} nor an {2} method"
def hasNeitherAnOrAnMethod(param0: Any, param1: Any, param2: Any): String =
rawHasNeitherAnOrAnMethod.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawHasBothAAndAnMethod = "{0} has both a {1} and an {2} method"
def hasBothAAndAnMethod(param0: Any, param1: Any, param2: Any): String =
rawHasBothAAndAnMethod.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawHasBothAnAndAnMethod = "{0} has both an {1} and an {2} method"
def hasBothAnAndAnMethod(param0: Any, param1: Any, param2: Any): String =
rawHasBothAnAndAnMethod.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawDidNotEndWith = "{0} did not end with substring {1}"
def didNotEndWith(param0: Any, param1: Any): String =
rawDidNotEndWith.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawEndedWith = "{0} ended with substring {1}"
def endedWith(param0: Any, param1: Any): String =
rawEndedWith.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotStartWith = "{0} did not start with substring {1}"
def didNotStartWith(param0: Any, param1: Any): String =
rawDidNotStartWith.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawStartedWith = "{0} started with substring {1}"
def startedWith(param0: Any, param1: Any): String =
rawStartedWith.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotStartWithRegex = "{0} did not start with a substring that matched the regular expression {1}"
def didNotStartWithRegex(param0: Any, param1: Any): String =
rawDidNotStartWithRegex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawStartedWithRegex = "{0} started with a substring that matched the regular expression {1}"
def startedWithRegex(param0: Any, param1: Any): String =
rawStartedWithRegex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawStartedWithRegexButNotGroup = "{0} started with a substring that matched the regular expression {1}, but {2} did not match group {3}"
def startedWithRegexButNotGroup(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawStartedWithRegexButNotGroup.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawStartedWithRegexButNotGroupAtIndex = "{0} started with a substring that matched the regular expression {1}, but {2} did not match group {3} at index {4}"
def startedWithRegexButNotGroupAtIndex(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any): String =
rawStartedWithRegexButNotGroupAtIndex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString())
final val rawStartedWithRegexAndGroup = "{0} started with a substring that matched the regular expression {1} and group {2}"
def startedWithRegexAndGroup(param0: Any, param1: Any, param2: Any): String =
rawStartedWithRegexAndGroup.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawDidNotEndWithRegex = "{0} did not end with a substring that matched the regular expression {1}"
def didNotEndWithRegex(param0: Any, param1: Any): String =
rawDidNotEndWithRegex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawEndedWithRegex = "{0} ended with a substring that matched the regular expression {1}"
def endedWithRegex(param0: Any, param1: Any): String =
rawEndedWithRegex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawEndedWithRegexButNotGroup = "{0} ended with a substring that matched the regular expression {1}, but {2} did not match group {3}"
def endedWithRegexButNotGroup(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawEndedWithRegexButNotGroup.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawEndedWithRegexButNotGroupAtIndex = "{0} ended with a substring that matched the regular expression {1}, but {2} did not match group {3} at index {4}"
def endedWithRegexButNotGroupAtIndex(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any): String =
rawEndedWithRegexButNotGroupAtIndex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString())
final val rawEndedWithRegexAndGroup = "{0} ended with a substring that matched the regular expression {1} and group {2}"
def endedWithRegexAndGroup(param0: Any, param1: Any, param2: Any): String =
rawEndedWithRegexAndGroup.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawDidNotContainNull = "{0} did not contain null"
def didNotContainNull(param0: Any): String =
rawDidNotContainNull.replaceAllLiterally("{0}", param0.toString())
final val rawContainedNull = "{0} contained null"
def containedNull(param0: Any): String =
rawContainedNull.replaceAllLiterally("{0}", param0.toString())
final val rawDidNotContainKey = "{0} did not contain key {1}"
def didNotContainKey(param0: Any, param1: Any): String =
rawDidNotContainKey.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedKey = "{0} contained key {1}"
def containedKey(param0: Any, param1: Any): String =
rawContainedKey.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainValue = "{0} did not contain value {1}"
def didNotContainValue(param0: Any, param1: Any): String =
rawDidNotContainValue.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedValue = "{0} contained value {1}"
def containedValue(param0: Any, param1: Any): String =
rawContainedValue.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHadSizeInsteadOfExpectedSize = "{0} had size {1} instead of expected size {2}"
def hadSizeInsteadOfExpectedSize(param0: Any, param1: Any, param2: Any): String =
rawHadSizeInsteadOfExpectedSize.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawHadSize = "{0} had size {1}"
def hadSize(param0: Any, param1: Any): String =
rawHadSize.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHadMessageInsteadOfExpectedMessage = "{0} had message {1} instead of expected message {2}"
def hadMessageInsteadOfExpectedMessage(param0: Any, param1: Any, param2: Any): String =
rawHadMessageInsteadOfExpectedMessage.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawHadExpectedMessage = "{0} had message {1}"
def hadExpectedMessage(param0: Any, param1: Any): String =
rawHadExpectedMessage.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainExpectedElement = "{0} did not contain element {1}"
def didNotContainExpectedElement(param0: Any, param1: Any): String =
rawDidNotContainExpectedElement.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedExpectedElement = "{0} contained element {1}"
def containedExpectedElement(param0: Any, param1: Any): String =
rawContainedExpectedElement.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotIncludeSubstring = "{0} did not include substring {1}"
def didNotIncludeSubstring(param0: Any, param1: Any): String =
rawDidNotIncludeSubstring.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawIncludedSubstring = "{0} included substring {1}"
def includedSubstring(param0: Any, param1: Any): String =
rawIncludedSubstring.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotIncludeRegex = "{0} did not include substring that matched regex {1}"
def didNotIncludeRegex(param0: Any, param1: Any): String =
rawDidNotIncludeRegex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawIncludedRegex = "{0} included substring that matched regex {1}"
def includedRegex(param0: Any, param1: Any): String =
rawIncludedRegex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawIncludedRegexButNotGroup = "{0} included substring that matched regex {1}, but {2} did not match group {3}"
def includedRegexButNotGroup(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawIncludedRegexButNotGroup.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawIncludedRegexButNotGroupAtIndex = "{0} included substring that matched regex {1}, but {2} did not match group {3} at index {4}"
def includedRegexButNotGroupAtIndex(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any): String =
rawIncludedRegexButNotGroupAtIndex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString())
final val rawIncludedRegexAndGroup = "{0} included substring that matched regex {1} and group {2}"
def includedRegexAndGroup(param0: Any, param1: Any, param2: Any): String =
rawIncludedRegexAndGroup.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawHadLengthInsteadOfExpectedLength = "{0} had length {1} instead of expected length {2}"
def hadLengthInsteadOfExpectedLength(param0: Any, param1: Any, param2: Any): String =
rawHadLengthInsteadOfExpectedLength.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawHadLength = "{0} had length {1}"
def hadLength(param0: Any, param1: Any): String =
rawHadLength.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotFullyMatchRegex = "{0} did not fully match the regular expression {1}"
def didNotFullyMatchRegex(param0: Any, param1: Any): String =
rawDidNotFullyMatchRegex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawFullyMatchedRegex = "{0} fully matched the regular expression {1}"
def fullyMatchedRegex(param0: Any, param1: Any): String =
rawFullyMatchedRegex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawFullyMatchedRegexButNotGroup = "{0} fully matched the regular expression {1}, but {2} did not match group {3}"
def fullyMatchedRegexButNotGroup(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawFullyMatchedRegexButNotGroup.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawFullyMatchedRegexButNotGroupAtIndex = "{0} fully matched the regular expression {1}, but {2} did not match group {3} at index {4}"
def fullyMatchedRegexButNotGroupAtIndex(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any): String =
rawFullyMatchedRegexButNotGroupAtIndex.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString())
final val rawFullyMatchedRegexAndGroup = "{0} fully matched the regular expression {1} and group {2}"
def fullyMatchedRegexAndGroup(param0: Any, param1: Any, param2: Any): String =
rawFullyMatchedRegexAndGroup.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawMatchResultedInFalse = "The value {0} matched a case in the specified partial function, but the result was false"
def matchResultedInFalse(param0: Any): String =
rawMatchResultedInFalse.replaceAllLiterally("{0}", param0.toString())
final val rawDidNotMatch = "The value {0} did not match any case in the specified partial function"
def didNotMatch(param0: Any): String =
rawDidNotMatch.replaceAllLiterally("{0}", param0.toString())
final val rawMatchResultedInTrue = "The value {0} matched a case in the specified partial function, and the result was true"
def matchResultedInTrue(param0: Any): String =
rawMatchResultedInTrue.replaceAllLiterally("{0}", param0.toString())
final val rawNoLengthStructure = "have length ({0}) used with an object that had no public field or method named 'length' or 'getLength'"
def noLengthStructure(param0: Any): String =
rawNoLengthStructure.replaceAllLiterally("{0}", param0.toString())
final val rawNoSizeStructure = "have size ({0}) used with an object that had no public field or method named 'size' or 'getSize'"
def noSizeStructure(param0: Any): String =
rawNoSizeStructure.replaceAllLiterally("{0}", param0.toString())
final val rawSizeAndGetSize = "have size ({0}) used with an object that has multiple fields and/or methods named 'size' and 'getSize'"
def sizeAndGetSize(param0: Any): String =
rawSizeAndGetSize.replaceAllLiterally("{0}", param0.toString())
final val rawNegativeOrZeroRange = "Range ({0}) passed to +- was zero or negative. Must be a positive non-zero number."
def negativeOrZeroRange(param0: Any): String =
rawNegativeOrZeroRange.replaceAllLiterally("{0}", param0.toString())
final val rawDidNotContainSameElements = "{0} did not contain the same elements as {1}"
def didNotContainSameElements(param0: Any, param1: Any): String =
rawDidNotContainSameElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedSameElements = "{0} contained the same elements as {1}"
def containedSameElements(param0: Any, param1: Any): String =
rawContainedSameElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainSameElementsInOrder = "{0} did not contain the same elements in the same (iterated) order as {1}"
def didNotContainSameElementsInOrder(param0: Any, param1: Any): String =
rawDidNotContainSameElementsInOrder.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedSameElementsInOrder = "{0} contained the same elements in the same (iterated) order as {1}"
def containedSameElementsInOrder(param0: Any, param1: Any): String =
rawContainedSameElementsInOrder.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainAllOfElements = "{0} did not contain all of ({1})"
def didNotContainAllOfElements(param0: Any, param1: Any): String =
rawDidNotContainAllOfElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedAllOfElements = "{0} contained all of ({1})"
def containedAllOfElements(param0: Any, param1: Any): String =
rawContainedAllOfElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawAllOfDuplicate = "allOf must not contain any duplicated values"
final val allOfDuplicate = rawAllOfDuplicate
final val rawDidNotContainAllElementsOf = "{0} did not contain all elements of {1}"
def didNotContainAllElementsOf(param0: Any, param1: Any): String =
rawDidNotContainAllElementsOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedAllElementsOf = "{0} contained all elements of {1}"
def containedAllElementsOf(param0: Any, param1: Any): String =
rawContainedAllElementsOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainAllOfElementsInOrder = "{0} did not contain all of ({1}) in order"
def didNotContainAllOfElementsInOrder(param0: Any, param1: Any): String =
rawDidNotContainAllOfElementsInOrder.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedAllOfElementsInOrder = "{0} contained all of ({1}) in order"
def containedAllOfElementsInOrder(param0: Any, param1: Any): String =
rawContainedAllOfElementsInOrder.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainAllElementsOfInOrder = "{0} did not contain all elements of {1} in order"
def didNotContainAllElementsOfInOrder(param0: Any, param1: Any): String =
rawDidNotContainAllElementsOfInOrder.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedAllElementsOfInOrder = "{0} contained all elements of {1} in order"
def containedAllElementsOfInOrder(param0: Any, param1: Any): String =
rawContainedAllElementsOfInOrder.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawInOrderDuplicate = "inOrder must not contain any duplicated values"
final val inOrderDuplicate = rawInOrderDuplicate
final val rawDidNotContainOneOfElements = "{0} did not contain one (and only one) of ({1})"
def didNotContainOneOfElements(param0: Any, param1: Any): String =
rawDidNotContainOneOfElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedOneOfElements = "{0} contained one (and only one) of ({1})"
def containedOneOfElements(param0: Any, param1: Any): String =
rawContainedOneOfElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainOneElementOf = "{0} did not contain one (and only one) element of {1}"
def didNotContainOneElementOf(param0: Any, param1: Any): String =
rawDidNotContainOneElementOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedOneElementOf = "{0} contained one (and only one) element of {1}"
def containedOneElementOf(param0: Any, param1: Any): String =
rawContainedOneElementOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainAtLeastOneOf = "{0} did not contain at least one of ({1})"
def didNotContainAtLeastOneOf(param0: Any, param1: Any): String =
rawDidNotContainAtLeastOneOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedAtLeastOneOf = "{0} contained at least one of ({1})"
def containedAtLeastOneOf(param0: Any, param1: Any): String =
rawContainedAtLeastOneOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawAtLeastOneOfDuplicate = "atLeastOneOf must not contain any duplicated values"
final val atLeastOneOfDuplicate = rawAtLeastOneOfDuplicate
final val rawDidNotContainAtLeastOneElementOf = "{0} did not contain at least one element of {1}"
def didNotContainAtLeastOneElementOf(param0: Any, param1: Any): String =
rawDidNotContainAtLeastOneElementOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedAtLeastOneElementOf = "{0} contained at least one element of {1}"
def containedAtLeastOneElementOf(param0: Any, param1: Any): String =
rawContainedAtLeastOneElementOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawOneOfDuplicate = "oneOf must not contain any duplicated values"
final val oneOfDuplicate = rawOneOfDuplicate
final val rawDidNotContainOnlyElements = "{0} did not contain only ({1})"
def didNotContainOnlyElements(param0: Any, param1: Any): String =
rawDidNotContainOnlyElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedOnlyElements = "{0} contained only ({1})"
def containedOnlyElements(param0: Any, param1: Any): String =
rawContainedOnlyElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotContainOnlyElementsWithFriendlyReminder = "{0} did not contain only ({1}), did you forget to say : _*"
def didNotContainOnlyElementsWithFriendlyReminder(param0: Any, param1: Any): String =
rawDidNotContainOnlyElementsWithFriendlyReminder.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedOnlyElementsWithFriendlyReminder = "{0} contained only ({1}), did you forget to say : _*"
def containedOnlyElementsWithFriendlyReminder(param0: Any, param1: Any): String =
rawContainedOnlyElementsWithFriendlyReminder.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawOnlyDuplicate = "only must not contain any duplicated values"
final val onlyDuplicate = rawOnlyDuplicate
final val rawOnlyEmpty = "only must be given at least one element"
final val onlyEmpty = rawOnlyEmpty
final val rawDidNotContainInOrderOnlyElements = "{0} did not contain only ({1}) in order"
def didNotContainInOrderOnlyElements(param0: Any, param1: Any): String =
rawDidNotContainInOrderOnlyElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedInOrderOnlyElements = "{0} contained only ({1}) in order"
def containedInOrderOnlyElements(param0: Any, param1: Any): String =
rawContainedInOrderOnlyElements.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawInOrderOnlyDuplicate = "inOrderOnly must not contain any duplicated values"
final val inOrderOnlyDuplicate = rawInOrderOnlyDuplicate
final val rawAtMostOneOfDuplicate = "atMostOneOf must not contain any duplicated values"
final val atMostOneOfDuplicate = rawAtMostOneOfDuplicate
final val rawDidNotContainAtMostOneOf = "{0} did not contain at most one of ({1})"
def didNotContainAtMostOneOf(param0: Any, param1: Any): String =
rawDidNotContainAtMostOneOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedAtMostOneOf = "{0} contained at most one of ({1})"
def containedAtMostOneOf(param0: Any, param1: Any): String =
rawContainedAtMostOneOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawAtMostOneElementOfDuplicate = "atMostOneElementOf must not contain any duplicated values"
final val atMostOneElementOfDuplicate = rawAtMostOneElementOfDuplicate
final val rawDidNotContainAtMostOneElementOf = "{0} did not contain at most one element of {1}"
def didNotContainAtMostOneElementOf(param0: Any, param1: Any): String =
rawDidNotContainAtMostOneElementOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedAtMostOneElementOf = "{0} contained at most one element of {1}"
def containedAtMostOneElementOf(param0: Any, param1: Any): String =
rawContainedAtMostOneElementOf.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawNoneOfDuplicate = "noneOf must not contain any duplicated values"
final val noneOfDuplicate = rawNoneOfDuplicate
final val rawDidNotContainA = "{0} did not contain a {1}"
def didNotContainA(param0: Any, param1: Any): String =
rawDidNotContainA.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedA = "{0} contained a {1}: {2}"
def containedA(param0: Any, param1: Any, param2: Any): String =
rawContainedA.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawDidNotContainAn = "{0} did not contain an {1}"
def didNotContainAn(param0: Any, param1: Any): String =
rawDidNotContainAn.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawContainedAn = "{0} contained an {1}: {2}"
def containedAn(param0: Any, param1: Any, param2: Any): String =
rawContainedAn.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawWasNotSorted = "{0} was not sorted"
def wasNotSorted(param0: Any): String =
rawWasNotSorted.replaceAllLiterally("{0}", param0.toString())
final val rawWasSorted = "{0} was sorted"
def wasSorted(param0: Any): String =
rawWasSorted.replaceAllLiterally("{0}", param0.toString())
final val rawWasNotDefined = "{0} was not defined"
def wasNotDefined(param0: Any): String =
rawWasNotDefined.replaceAllLiterally("{0}", param0.toString())
final val rawWasDefined = "{0} was defined"
def wasDefined(param0: Any): String =
rawWasDefined.replaceAllLiterally("{0}", param0.toString())
final val rawDoesNotExist = "{0} does not exist"
def doesNotExist(param0: Any): String =
rawDoesNotExist.replaceAllLiterally("{0}", param0.toString())
final val rawExists = "{0} exists"
def exists(param0: Any): String =
rawExists.replaceAllLiterally("{0}", param0.toString())
final val rawWasNotReadable = "{0} was not readable"
def wasNotReadable(param0: Any): String =
rawWasNotReadable.replaceAllLiterally("{0}", param0.toString())
final val rawWasReadable = "{0} was readable"
def wasReadable(param0: Any): String =
rawWasReadable.replaceAllLiterally("{0}", param0.toString())
final val rawWasNotWritable = "{0} was not writable"
def wasNotWritable(param0: Any): String =
rawWasNotWritable.replaceAllLiterally("{0}", param0.toString())
final val rawWasWritable = "{0} was writable"
def wasWritable(param0: Any): String =
rawWasWritable.replaceAllLiterally("{0}", param0.toString())
final val rawDidNotMatchTheGivenPattern = "{0} did not match the given pattern"
def didNotMatchTheGivenPattern(param0: Any): String =
rawDidNotMatchTheGivenPattern.replaceAllLiterally("{0}", param0.toString())
final val rawMatchedTheGivenPattern = "{0} matched the given pattern"
def matchedTheGivenPattern(param0: Any): String =
rawMatchedTheGivenPattern.replaceAllLiterally("{0}", param0.toString())
final val rawDuplicateTestName = "Duplicate test name: {0}"
def duplicateTestName(param0: Any): String =
rawDuplicateTestName.replaceAllLiterally("{0}", param0.toString())
final val rawCantNestFeatureClauses = "Feature clauses cannot be nested."
final val cantNestFeatureClauses = rawCantNestFeatureClauses
final val rawItCannotAppearInsideAnotherIt = "An it clause may not appear inside another it clause."
final val itCannotAppearInsideAnotherIt = rawItCannotAppearInsideAnotherIt
final val rawItCannotAppearInsideAnotherItOrThey = "An it clause may not appear inside another it or they clause."
final val itCannotAppearInsideAnotherItOrThey = rawItCannotAppearInsideAnotherItOrThey
final val rawTheyCannotAppearInsideAnotherItOrThey = "A they clause may not appear inside another it or they clause."
final val theyCannotAppearInsideAnotherItOrThey = rawTheyCannotAppearInsideAnotherItOrThey
final val rawDescribeCannotAppearInsideAnIt = "A describe clause may not appear inside an it clause."
final val describeCannotAppearInsideAnIt = rawDescribeCannotAppearInsideAnIt
final val rawIgnoreCannotAppearInsideAnIt = "An ignore clause may not appear inside an it clause."
final val ignoreCannotAppearInsideAnIt = rawIgnoreCannotAppearInsideAnIt
final val rawIgnoreCannotAppearInsideAnItOrAThey = "An ignore clause may not appear inside an it or a they clause."
final val ignoreCannotAppearInsideAnItOrAThey = rawIgnoreCannotAppearInsideAnItOrAThey
final val rawScenarioCannotAppearInsideAnotherScenario = "A scenario clause may not appear inside another scenario clause."
final val scenarioCannotAppearInsideAnotherScenario = rawScenarioCannotAppearInsideAnotherScenario
final val rawFeatureCannotAppearInsideAScenario = "A feature clause may not appear inside a scenario clause."
final val featureCannotAppearInsideAScenario = rawFeatureCannotAppearInsideAScenario
final val rawIgnoreCannotAppearInsideAScenario = "An ignore clause may not appear inside a scenario clause."
final val ignoreCannotAppearInsideAScenario = rawIgnoreCannotAppearInsideAScenario
final val rawTestCannotAppearInsideAnotherTest = "A test clause may not appear inside another test clause."
final val testCannotAppearInsideAnotherTest = rawTestCannotAppearInsideAnotherTest
final val rawPropertyCannotAppearInsideAnotherProperty = "A property clause may not appear inside another property clause."
final val propertyCannotAppearInsideAnotherProperty = rawPropertyCannotAppearInsideAnotherProperty
final val rawIgnoreCannotAppearInsideATest = "An ignore clause may not appear inside a test clause."
final val ignoreCannotAppearInsideATest = rawIgnoreCannotAppearInsideATest
final val rawIgnoreCannotAppearInsideAProperty = "An ignore clause may not appear inside a property clause."
final val ignoreCannotAppearInsideAProperty = rawIgnoreCannotAppearInsideAProperty
final val rawShouldCannotAppearInsideAnIn = "a \"should\" clause may not appear inside an \"in\" clause"
final val shouldCannotAppearInsideAnIn = rawShouldCannotAppearInsideAnIn
final val rawMustCannotAppearInsideAnIn = "a \"must\" clause may not appear inside an \"in\" clause"
final val mustCannotAppearInsideAnIn = rawMustCannotAppearInsideAnIn
final val rawWhenCannotAppearInsideAnIn = "a \"when\" clause may not appear inside an \"in\" clause"
final val whenCannotAppearInsideAnIn = rawWhenCannotAppearInsideAnIn
final val rawThatCannotAppearInsideAnIn = "a \"that\" clause may not appear inside an \"in\" clause"
final val thatCannotAppearInsideAnIn = rawThatCannotAppearInsideAnIn
final val rawWhichCannotAppearInsideAnIn = "a \"which\" clause may not appear inside an \"in\" clause"
final val whichCannotAppearInsideAnIn = rawWhichCannotAppearInsideAnIn
final val rawCanCannotAppearInsideAnIn = "a \"can\" clause may not appear inside an \"in\" clause"
final val canCannotAppearInsideAnIn = rawCanCannotAppearInsideAnIn
final val rawBehaviorOfCannotAppearInsideAnIn = "a \"behavior of\" clause may not appear inside an \"in\" clause"
final val behaviorOfCannotAppearInsideAnIn = rawBehaviorOfCannotAppearInsideAnIn
final val rawDashCannotAppearInsideAnIn = "a \"-\" clause may not appear inside an \"in\" clause"
final val dashCannotAppearInsideAnIn = rawDashCannotAppearInsideAnIn
final val rawInCannotAppearInsideAnotherIn = "An in clause may not appear inside another in clause."
final val inCannotAppearInsideAnotherIn = rawInCannotAppearInsideAnotherIn
final val rawInCannotAppearInsideAnotherInOrIs = "An in clause may not appear inside another in or is clause."
final val inCannotAppearInsideAnotherInOrIs = rawInCannotAppearInsideAnotherInOrIs
final val rawIsCannotAppearInsideAnotherInOrIs = "An is clause may not appear inside another in or is clause."
final val isCannotAppearInsideAnotherInOrIs = rawIsCannotAppearInsideAnotherInOrIs
final val rawIgnoreCannotAppearInsideAnIn = "An ignore clause may not appear inside an in clause."
final val ignoreCannotAppearInsideAnIn = rawIgnoreCannotAppearInsideAnIn
final val rawIgnoreCannotAppearInsideAnInOrAnIs = "An ignore clause may not appear inside an in or an is clause."
final val ignoreCannotAppearInsideAnInOrAnIs = rawIgnoreCannotAppearInsideAnInOrAnIs
final val rawRegistrationAlreadyClosed = "Test Registration is already closed."
final val registrationAlreadyClosed = rawRegistrationAlreadyClosed
final val rawItMustAppearAfterTopLevelSubject = "An it clause must only appear after a top level subject clause."
final val itMustAppearAfterTopLevelSubject = rawItMustAppearAfterTopLevelSubject
final val rawTheyMustAppearAfterTopLevelSubject = "A they clause must only appear after a top level subject clause."
final val theyMustAppearAfterTopLevelSubject = rawTheyMustAppearAfterTopLevelSubject
final val rawAllPropertiesHadExpectedValues = "All properties had their expected values, respectively, on object {0}"
def allPropertiesHadExpectedValues(param0: Any): String =
rawAllPropertiesHadExpectedValues.replaceAllLiterally("{0}", param0.toString())
final val rawMidSentenceAllPropertiesHadExpectedValues = "all properties had their expected values, respectively, on object {0}"
def midSentenceAllPropertiesHadExpectedValues(param0: Any): String =
rawMidSentenceAllPropertiesHadExpectedValues.replaceAllLiterally("{0}", param0.toString())
final val rawPropertyHadExpectedValue = "The {0} property had its expected value {1}, on object {2}"
def propertyHadExpectedValue(param0: Any, param1: Any, param2: Any): String =
rawPropertyHadExpectedValue.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawMidSentencePropertyHadExpectedValue = "the {0} property had its expected value {1}, on object {2}"
def midSentencePropertyHadExpectedValue(param0: Any, param1: Any, param2: Any): String =
rawMidSentencePropertyHadExpectedValue.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawPropertyDidNotHaveExpectedValue = "The {0} property had value {2}, instead of its expected value {1}, on object {3}"
def propertyDidNotHaveExpectedValue(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawPropertyDidNotHaveExpectedValue.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawMidSentencePropertyDidNotHaveExpectedValue = "the {0} property had value {2}, instead of its expected value {1}, on object {3}"
def midSentencePropertyDidNotHaveExpectedValue(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawMidSentencePropertyDidNotHaveExpectedValue.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawPropertyNotFound = "have {0} ({1}) used with an object that had no public field or method named {0} or {2}"
def propertyNotFound(param0: Any, param1: Any, param2: Any): String =
rawPropertyNotFound.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawPropertyCheckSucceeded = "Property check succeeded"
final val propertyCheckSucceeded = rawPropertyCheckSucceeded
final val rawLengthPropertyNotAnInteger = "The length property was none of Byte, Short, Int, or Long."
final val lengthPropertyNotAnInteger = rawLengthPropertyNotAnInteger
final val rawSizePropertyNotAnInteger = "The size property was none of Byte, Short, Int, or Long."
final val sizePropertyNotAnInteger = rawSizePropertyNotAnInteger
final val rawWasEqualTo = "{0} was equal to {1}"
def wasEqualTo(param0: Any, param1: Any): String =
rawWasEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawWasNotEqualTo = "{0} was not equal to {1}"
def wasNotEqualTo(param0: Any, param1: Any): String =
rawWasNotEqualTo.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawPrintedReportPlusLineNumber = "{0} ({1})"
def printedReportPlusLineNumber(param0: Any, param1: Any): String =
rawPrintedReportPlusLineNumber.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawPrintedReportPlusPath = "{0}\n** {1} **"
def printedReportPlusPath(param0: Any, param1: Any): String =
rawPrintedReportPlusPath.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawPropertyFailed = "Falsified after {0} successful property evaluations."
def propertyFailed(param0: Any): String =
rawPropertyFailed.replaceAllLiterally("{0}", param0.toString())
final val rawPropertyExhausted = "Gave up after {0} successful property evaluations. {1} evaluations were discarded."
def propertyExhausted(param0: Any, param1: Any): String =
rawPropertyExhausted.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawUndecoratedPropertyCheckFailureMessage = "Property check failed."
final val undecoratedPropertyCheckFailureMessage = rawUndecoratedPropertyCheckFailureMessage
final val rawPropertyException = "{0} was thrown during property evaluation."
def propertyException(param0: Any): String =
rawPropertyException.replaceAllLiterally("{0}", param0.toString())
final val rawGeneratorException = "{0} was thrown during argument generation."
def generatorException(param0: Any): String =
rawGeneratorException.replaceAllLiterally("{0}", param0.toString())
final val rawThrownExceptionsMessage = "Message: {0}"
def thrownExceptionsMessage(param0: Any): String =
rawThrownExceptionsMessage.replaceAllLiterally("{0}", param0.toString())
final val rawThrownExceptionsLocation = "Location: ({0})"
def thrownExceptionsLocation(param0: Any): String =
rawThrownExceptionsLocation.replaceAllLiterally("{0}", param0.toString())
final val rawPropCheckExhausted = "Gave up after {0} successful property evaluations. {1} evaluations were discarded."
def propCheckExhausted(param0: Any, param1: Any): String =
rawPropCheckExhausted.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawPropCheckExhaustedAfterOne = "Gave up after 1 successful property evaluation. {0} evaluations were discarded."
def propCheckExhaustedAfterOne(param0: Any): String =
rawPropCheckExhaustedAfterOne.replaceAllLiterally("{0}", param0.toString())
final val rawOccurredAtRow = "Occurred at table row {0} (zero based, not counting headings), which had values ("
def occurredAtRow(param0: Any): String =
rawOccurredAtRow.replaceAllLiterally("{0}", param0.toString())
final val rawOccurredOnValues = "Occurred when passed generated values ("
final val occurredOnValues = rawOccurredOnValues
final val rawPropCheckLabel = "Label of failing property:"
final val propCheckLabel = rawPropCheckLabel
final val rawPropCheckLabels = "Labels of failing property:"
final val propCheckLabels = rawPropCheckLabels
final val rawSuiteAndTestNamesFormattedForDisplay = "{0}, {1}"
def suiteAndTestNamesFormattedForDisplay(param0: Any, param1: Any): String =
rawSuiteAndTestNamesFormattedForDisplay.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawInitSeed = "Init Seed: {0}"
def initSeed(param0: Any): String =
rawInitSeed.replaceAllLiterally("{0}", param0.toString())
final val rawNotLoneElement = "Expected {0} to contain exactly 1 element, but it has size {1}"
def notLoneElement(param0: Any, param1: Any): String =
rawNotLoneElement.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawTestSummary = "Tests: succeeded {0}, failed {1}, canceled {2}, ignored {3}, pending {4}"
def testSummary(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any): String =
rawTestSummary.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString())
final val rawSuiteSummary = "Suites: completed {0}, aborted {1}"
def suiteSummary(param0: Any, param1: Any): String =
rawSuiteSummary.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawSuiteScopeSummary = "Suites: completed {0}, aborted {1} Scopes: pending {2}"
def suiteScopeSummary(param0: Any, param1: Any, param2: Any): String =
rawSuiteScopeSummary.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawRunCompletedIn = "Run completed in {0}."
def runCompletedIn(param0: Any): String =
rawRunCompletedIn.replaceAllLiterally("{0}", param0.toString())
final val rawRunCompleted = "Run completed."
final val runCompleted = rawRunCompleted
final val rawRunAbortedIn = "Run aborted after {0}."
def runAbortedIn(param0: Any): String =
rawRunAbortedIn.replaceAllLiterally("{0}", param0.toString())
final val rawRunStoppedIn = "Run stopped after {0}."
def runStoppedIn(param0: Any): String =
rawRunStoppedIn.replaceAllLiterally("{0}", param0.toString())
final val rawRunStopped = "Run aborted."
final val runStopped = rawRunStopped
final val rawTotalNumberOfTestsRun = "Total number of tests run: {0}"
def totalNumberOfTestsRun(param0: Any): String =
rawTotalNumberOfTestsRun.replaceAllLiterally("{0}", param0.toString())
final val rawOneMillisecond = "1 millisecond"
final val oneMillisecond = rawOneMillisecond
final val rawMilliseconds = "{0} milliseconds"
def milliseconds(param0: Any): String =
rawMilliseconds.replaceAllLiterally("{0}", param0.toString())
final val rawOneSecond = "1 second"
final val oneSecond = rawOneSecond
final val rawOneSecondOneMillisecond = "1 second, 1 millisecond"
final val oneSecondOneMillisecond = rawOneSecondOneMillisecond
final val rawOneSecondMilliseconds = "1 second, {0} milliseconds"
def oneSecondMilliseconds(param0: Any): String =
rawOneSecondMilliseconds.replaceAllLiterally("{0}", param0.toString())
final val rawSeconds = "{0} seconds"
def seconds(param0: Any): String =
rawSeconds.replaceAllLiterally("{0}", param0.toString())
final val rawSecondsMilliseconds = "{0} seconds, {1} milliseconds"
def secondsMilliseconds(param0: Any, param1: Any): String =
rawSecondsMilliseconds.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawOneMinute = "1 minute"
final val oneMinute = rawOneMinute
final val rawOneMinuteOneSecond = "1 minute, 1 second"
final val oneMinuteOneSecond = rawOneMinuteOneSecond
final val rawOneMinuteSeconds = "1 minute, {0} seconds"
def oneMinuteSeconds(param0: Any): String =
rawOneMinuteSeconds.replaceAllLiterally("{0}", param0.toString())
final val rawMinutes = "{0} minutes"
def minutes(param0: Any): String =
rawMinutes.replaceAllLiterally("{0}", param0.toString())
final val rawMinutesOneSecond = "{0} minutes, 1 second"
def minutesOneSecond(param0: Any): String =
rawMinutesOneSecond.replaceAllLiterally("{0}", param0.toString())
final val rawMinutesSeconds = "{0} minutes, {1} seconds"
def minutesSeconds(param0: Any, param1: Any): String =
rawMinutesSeconds.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawOneHour = "1 hour"
final val oneHour = rawOneHour
final val rawOneHourOneSecond = "1 hour, 1 second"
final val oneHourOneSecond = rawOneHourOneSecond
final val rawOneHourSeconds = "1 hour, {0} seconds"
def oneHourSeconds(param0: Any): String =
rawOneHourSeconds.replaceAllLiterally("{0}", param0.toString())
final val rawOneHourOneMinute = "1 hour, 1 minute"
final val oneHourOneMinute = rawOneHourOneMinute
final val rawOneHourOneMinuteOneSecond = "1 hour, 1 minute, 1 second"
final val oneHourOneMinuteOneSecond = rawOneHourOneMinuteOneSecond
final val rawOneHourOneMinuteSeconds = "1 hour, 1 minute, {0} seconds"
def oneHourOneMinuteSeconds(param0: Any): String =
rawOneHourOneMinuteSeconds.replaceAllLiterally("{0}", param0.toString())
final val rawOneHourMinutes = "1 hour, {0} minutes"
def oneHourMinutes(param0: Any): String =
rawOneHourMinutes.replaceAllLiterally("{0}", param0.toString())
final val rawOneHourMinutesOneSecond = "1 hour, {0} minutes, 1 second"
def oneHourMinutesOneSecond(param0: Any): String =
rawOneHourMinutesOneSecond.replaceAllLiterally("{0}", param0.toString())
final val rawOneHourMinutesSeconds = "1 hour, {0} minutes, {1} seconds"
def oneHourMinutesSeconds(param0: Any, param1: Any): String =
rawOneHourMinutesSeconds.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHours = "{0} hours"
def hours(param0: Any): String =
rawHours.replaceAllLiterally("{0}", param0.toString())
final val rawHoursOneSecond = "{0} hours, 1 second"
def hoursOneSecond(param0: Any): String =
rawHoursOneSecond.replaceAllLiterally("{0}", param0.toString())
final val rawHoursSeconds = "{0} hours, {1} seconds"
def hoursSeconds(param0: Any, param1: Any): String =
rawHoursSeconds.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHoursOneMinute = "{0} hours, 1 minute"
def hoursOneMinute(param0: Any): String =
rawHoursOneMinute.replaceAllLiterally("{0}", param0.toString())
final val rawHoursOneMinuteOneSecond = "{0} hours, 1 minute, 1 second"
def hoursOneMinuteOneSecond(param0: Any): String =
rawHoursOneMinuteOneSecond.replaceAllLiterally("{0}", param0.toString())
final val rawHoursOneMinuteSeconds = "{0} hours, 1 minute, {1} seconds"
def hoursOneMinuteSeconds(param0: Any, param1: Any): String =
rawHoursOneMinuteSeconds.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHoursMinutes = "{0} hours, {1} minutes"
def hoursMinutes(param0: Any, param1: Any): String =
rawHoursMinutes.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHoursMinutesOneSecond = "{0} hours, {1} minutes, 1 second"
def hoursMinutesOneSecond(param0: Any, param1: Any): String =
rawHoursMinutesOneSecond.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawHoursMinutesSeconds = "{0} hours, {1} minutes, {2} seconds"
def hoursMinutesSeconds(param0: Any, param1: Any, param2: Any): String =
rawHoursMinutesSeconds.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawWithDuration = "{0} ({1})"
def withDuration(param0: Any, param1: Any): String =
rawWithDuration.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawFeature = "Feature: {0}"
def feature(param0: Any): String =
rawFeature.replaceAllLiterally("{0}", param0.toString())
final val rawNeedFixtureInTestName = "No test found with the name {0}, because in an org.scalatest.fixture.Suite test names end in either \"(Fixture)\" or \"(Fixture, Informer)\""
def needFixtureInTestName(param0: Any): String =
rawNeedFixtureInTestName.replaceAllLiterally("{0}", param0.toString())
final val rawTestNotFound = "Test not found: {0}"
def testNotFound(param0: Any): String =
rawTestNotFound.replaceAllLiterally("{0}", param0.toString())
final val rawPendingUntilFixed = "A block of code that was marked pendingUntilFixed did not throw an exception. Remove \"pendingUntilFixed\" and the curly braces to eliminate this failure."
final val pendingUntilFixed = rawPendingUntilFixed
final val rawDashXDeprecated = "-x has been deprecated and will select an XML reporter in a future version of ScalaTest. Please use -l instead of -x to specify tagsToExclude."
final val dashXDeprecated = rawDashXDeprecated
final val rawThreadCalledAfterConductingHasCompleted = "Cannot invoke the thread method on Conductor after its multi-threaded test has completed."
final val threadCalledAfterConductingHasCompleted = rawThreadCalledAfterConductingHasCompleted
final val rawCannotInvokeWhenFinishedAfterConduct = "Cannot invoke whenFinished after conduct (which is called by whenFinished) has been invoked."
final val cannotInvokeWhenFinishedAfterConduct = rawCannotInvokeWhenFinishedAfterConduct
final val rawCantRegisterThreadsWithSameName = "Cannot register two threads with the same name. Duplicate name: {0}."
def cantRegisterThreadsWithSameName(param0: Any): String =
rawCantRegisterThreadsWithSameName.replaceAllLiterally("{0}", param0.toString())
final val rawCannotCallConductTwice = "A Conductor's conduct method can only be invoked once."
final val cannotCallConductTwice = rawCannotCallConductTwice
final val rawCannotWaitForBeatZero = "A Conductor starts at beat zero, so you can't wait for beat zero."
final val cannotWaitForBeatZero = rawCannotWaitForBeatZero
final val rawCannotWaitForNegativeBeat = "A Conductor starts at beat zero, so you can only wait for a beat greater than zero."
final val cannotWaitForNegativeBeat = rawCannotWaitForNegativeBeat
final val rawCannotPassNonPositiveClockPeriod = "The clockPeriod passed to conduct must be greater than zero. Value passed was: {0}."
def cannotPassNonPositiveClockPeriod(param0: Any): String =
rawCannotPassNonPositiveClockPeriod.replaceAllLiterally("{0}", param0.toString())
final val rawCannotPassNonPositiveTimeout = "The timeout passed to conduct must be greater than zero. Value passed was: {0}."
def cannotPassNonPositiveTimeout(param0: Any): String =
rawCannotPassNonPositiveTimeout.replaceAllLiterally("{0}", param0.toString())
final val rawWhenFinishedCanOnlyBeCalledByMainThread = "whenFinished can only be called by the thread that created Conductor."
final val whenFinishedCanOnlyBeCalledByMainThread = rawWhenFinishedCanOnlyBeCalledByMainThread
final val rawSuspectedDeadlock = "Test aborted because of suspected deadlock. No progress has been made (the beat did not advance) for {0} clock periods ({1})."
def suspectedDeadlock(param0: Any, param1: Any): String =
rawSuspectedDeadlock.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawTestTimedOut = "Test timed out because threads existed that were runnable while no progress was made (the beat did not advance) for {0}."
def testTimedOut(param0: Any): String =
rawTestTimedOut.replaceAllLiterally("{0}", param0.toString())
final val rawSuspectedDeadlockDEPRECATED = "Test aborted because of suspected deadlock. No progress has been made (the beat did not advance) for {0} clock periods ({1} ms)."
def suspectedDeadlockDEPRECATED(param0: Any, param1: Any): String =
rawSuspectedDeadlockDEPRECATED.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawTestTimedOutDEPRECATED = "Test timed out because threads existed that were runnable while no progress was made (the beat did not advance) for {0} seconds."
def testTimedOutDEPRECATED(param0: Any): String =
rawTestTimedOutDEPRECATED.replaceAllLiterally("{0}", param0.toString())
final val rawConcurrentInformerMod = "Two threads have apparently attempted to run a suite at the same time. This has resulted in both threads attempting to concurrently change the current Informer. Suite class name: {0}"
def concurrentInformerMod(param0: Any): String =
rawConcurrentInformerMod.replaceAllLiterally("{0}", param0.toString())
final val rawConcurrentNotifierMod = "Two threads have apparently attempted to run a suite at the same time. This has resulted in both threads attempting to concurrently change the current Notifier. Suite class name: {0}"
def concurrentNotifierMod(param0: Any): String =
rawConcurrentNotifierMod.replaceAllLiterally("{0}", param0.toString())
final val rawConcurrentAlerterMod = "Two threads have apparently attempted to run a suite at the same time. This has resulted in both threads attempting to concurrently change the current Alerter. Suite class name: {0}"
def concurrentAlerterMod(param0: Any): String =
rawConcurrentAlerterMod.replaceAllLiterally("{0}", param0.toString())
final val rawConcurrentDocumenterMod = "Two threads have apparently attempted to run a suite at the same time. This has resulted in both threads attempting to concurrently change the current Documenter. Suite class name: {0}"
def concurrentDocumenterMod(param0: Any): String =
rawConcurrentDocumenterMod.replaceAllLiterally("{0}", param0.toString())
final val rawCantCallInfoNow = "\"Sorry, you can only call the info method during the registration and running phases (i.e., when constructing or executing this {0}).\""
def cantCallInfoNow(param0: Any): String =
rawCantCallInfoNow.replaceAllLiterally("{0}", param0.toString())
final val rawCantCallMarkupNow = "\"Sorry, you can only call the markup method during the registration and running phases (i.e., when constructing or executing this {0}).\""
def cantCallMarkupNow(param0: Any): String =
rawCantCallMarkupNow.replaceAllLiterally("{0}", param0.toString())
final val rawConcurrentFunSuiteMod = "Two threads attempted to modify FunSuite's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"testsFor\" or \"test\" methods on the object before the first thread completed its construction."
final val concurrentFunSuiteMod = rawConcurrentFunSuiteMod
final val rawConcurrentPropSpecMod = "Two threads attempted to modify PropSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"testsFor\" or \"test\" methods on the object before the first thread completed its construction."
final val concurrentPropSpecMod = rawConcurrentPropSpecMod
final val rawConcurrentFixtureFunSuiteMod = "Two threads attempted to modify FixtureFunSuite's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"testsFor\" or \"test\" methods on the object before the first thread completed its construction."
final val concurrentFixtureFunSuiteMod = rawConcurrentFixtureFunSuiteMod
final val rawConcurrentFixturePropSpecMod = "Two threads attempted to modify FixturePropSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"testsFor\" or \"test\" methods on the object before the first thread completed its construction."
final val concurrentFixturePropSpecMod = rawConcurrentFixturePropSpecMod
final val rawConcurrentSpecMod = "Two threads attempted to modify Spec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"describe\" or \"it\" methods on the object before the first thread completed its construction."
final val concurrentSpecMod = rawConcurrentSpecMod
final val rawConcurrentFreeSpecMod = "Two threads attempted to modify FreeSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"describe\" or \"it\" methods on the object before the first thread completed its construction."
final val concurrentFreeSpecMod = rawConcurrentFreeSpecMod
final val rawConcurrentFixtureSpecMod = "Two threads attempted to modify FixtureSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"describe\" or \"it\" methods on the object before the first thread completed its construction."
final val concurrentFixtureSpecMod = rawConcurrentFixtureSpecMod
final val rawConcurrentFlatSpecMod = "Two threads attempted to modify FlatSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to register tests (such as with \"it should\") on the object before the first thread completed its construction."
final val concurrentFlatSpecMod = rawConcurrentFlatSpecMod
final val rawConcurrentFixtureFlatSpecMod = "Two threads attempted to modify FixtureFlatSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to register tests (such as with \"it should\") on the object before the first thread completed its construction."
final val concurrentFixtureFlatSpecMod = rawConcurrentFixtureFlatSpecMod
final val rawConcurrentWordSpecMod = "Two threads attempted to modify WordSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to register tests (such as with \"do something\" in) on the object before the first thread completed its construction."
final val concurrentWordSpecMod = rawConcurrentWordSpecMod
final val rawConcurrentFixtureWordSpecMod = "Two threads attempted to modify FixtureWordSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to register tests (such as with \"do something\" in) on the object before the first thread completed its construction."
final val concurrentFixtureWordSpecMod = rawConcurrentFixtureWordSpecMod
final val rawConcurrentFixtureFreeSpecMod = "Two threads attempted to modify FixtureFreeSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to register tests (such as with \"do something\" in) on the object before the first thread completed its construction."
final val concurrentFixtureFreeSpecMod = rawConcurrentFixtureFreeSpecMod
final val rawConcurrentFeatureSpecMod = "Two threads attempted to modify FeatureSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"feature\" or \"scenario\" methods on the object before the first thread completed its construction."
final val concurrentFeatureSpecMod = rawConcurrentFeatureSpecMod
final val rawConcurrentFixtureFeatureSpecMod = "Two threads attempted to modify FixtureFeatureSpec's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"feature\" or \"scenario\" methods on the object before the first thread completed its construction."
final val concurrentFixtureFeatureSpecMod = rawConcurrentFixtureFeatureSpecMod
final val rawConcurrentDocSpecMod = "Two threads attempted to modify Doc's internal data, which should only be modified by the thread that constructs the object. This likely means that a subclass has allowed the this reference to escape during construction, and some other thread attempted to invoke the \"body\" method on the object before the first thread completed its construction."
final val concurrentDocSpecMod = rawConcurrentDocSpecMod
final val rawTryNotAFailure = "The Try on which failure was invoked was not a Failure; it was {0}."
def tryNotAFailure(param0: Any): String =
rawTryNotAFailure.replaceAllLiterally("{0}", param0.toString())
final val rawTryNotASuccess = "The Try on which success was invoked was not a Success; it was {0}."
def tryNotASuccess(param0: Any): String =
rawTryNotASuccess.replaceAllLiterally("{0}", param0.toString())
final val rawOptionValueNotDefined = "The Option on which value was invoked was not defined."
final val optionValueNotDefined = rawOptionValueNotDefined
final val rawEitherLeftValueNotDefined = "The Either on which left.value was invoked was not defined as a Left; it was {0}."
def eitherLeftValueNotDefined(param0: Any): String =
rawEitherLeftValueNotDefined.replaceAllLiterally("{0}", param0.toString())
final val rawEitherRightValueNotDefined = "The Either on which right.value was invoked was not defined as a Right; it was {0}. "
def eitherRightValueNotDefined(param0: Any): String =
rawEitherRightValueNotDefined.replaceAllLiterally("{0}", param0.toString())
final val rawEitherValueNotDefined = "The Either on which value was invoked was not defined as a Right; it was {0}. "
def eitherValueNotDefined(param0: Any): String =
rawEitherValueNotDefined.replaceAllLiterally("{0}", param0.toString())
final val rawPartialFunctionValueNotDefined = "The PartialFunction on which valueAt( {0} ) was invoked was not defined."
def partialFunctionValueNotDefined(param0: Any): String =
rawPartialFunctionValueNotDefined.replaceAllLiterally("{0}", param0.toString())
final val rawInsidePartialFunctionNotDefined = "The partial function passed as the second parameter to inside was not defined at the value passed as the first parameter to inside, which was: {0}"
def insidePartialFunctionNotDefined(param0: Any): String =
rawInsidePartialFunctionNotDefined.replaceAllLiterally("{0}", param0.toString())
final val rawInsidePartialFunctionAppendSomeMsg = "{0},\n{1}inside {2}"
def insidePartialFunctionAppendSomeMsg(param0: Any, param1: Any, param2: Any): String =
rawInsidePartialFunctionAppendSomeMsg.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawInsidePartialFunctionAppendNone = "An exception was thrown,\n{0}inside {1}"
def insidePartialFunctionAppendNone(param0: Any, param1: Any): String =
rawInsidePartialFunctionAppendNone.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotEventuallySucceed = "The code passed to eventually never returned normally. Attempted {0} times over {1}."
def didNotEventuallySucceed(param0: Any, param1: Any): String =
rawDidNotEventuallySucceed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotEventuallySucceedBecause = "The code passed to eventually never returned normally. Attempted {0} times over {1}. Last failure message: {2}."
def didNotEventuallySucceedBecause(param0: Any, param1: Any, param2: Any): String =
rawDidNotEventuallySucceedBecause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawDidNotUltimatelySucceed = "The future-producing code passed to ultimately never yielded a successful future. Attempted {0} times over {1}."
def didNotUltimatelySucceed(param0: Any, param1: Any): String =
rawDidNotUltimatelySucceed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDidNotUltimatelySucceedBecause = "The future-producing code passed to ultimately never yielded a successful future. Attempted {0} times over {1}. Last failure message: {2}."
def didNotUltimatelySucceedBecause(param0: Any, param1: Any, param2: Any): String =
rawDidNotUltimatelySucceedBecause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawWasNeverReady = "A timeout occurred waiting for a future to complete. Waited {0}."
def wasNeverReady(param0: Any): String =
rawWasNeverReady.replaceAllLiterally("{0}", param0.toString())
final val rawAwaitMustBeCalledOnCreatingThread = "The await method on Waiter may only be called from the same thread that constructed the Waiter."
final val awaitMustBeCalledOnCreatingThread = rawAwaitMustBeCalledOnCreatingThread
final val rawAwaitTimedOut = "The await method on Waiter timed out."
final val awaitTimedOut = rawAwaitTimedOut
final val rawFutureReturnedAnException = "The future returned an exception of type: {0}."
def futureReturnedAnException(param0: Any): String =
rawFutureReturnedAnException.replaceAllLiterally("{0}", param0.toString())
final val rawFutureReturnedAnExceptionWithMessage = "The future returned an exception of type: {0}, with message: {1}."
def futureReturnedAnExceptionWithMessage(param0: Any, param1: Any): String =
rawFutureReturnedAnExceptionWithMessage.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawFutureWasCanceled = "The future was canceled."
final val futureWasCanceled = rawFutureWasCanceled
final val rawFutureExpired = "The future expired. Queried {0} times, sleeping {1} milliseconds between each query."
def futureExpired(param0: Any, param1: Any): String =
rawFutureExpired.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawTimeoutFailedAfter = "The code passed to failAfter did not complete within {0}."
def timeoutFailedAfter(param0: Any): String =
rawTimeoutFailedAfter.replaceAllLiterally("{0}", param0.toString())
final val rawTimeoutFailingAfter = "The code passed to failingAfter did not complete within {0}."
def timeoutFailingAfter(param0: Any): String =
rawTimeoutFailingAfter.replaceAllLiterally("{0}", param0.toString())
final val rawTimeoutCanceledAfter = "The code passed to cancelAfter did not complete within {0}."
def timeoutCanceledAfter(param0: Any): String =
rawTimeoutCanceledAfter.replaceAllLiterally("{0}", param0.toString())
final val rawTimeoutCancelingAfter = "The code passed to cancelingAfter did not complete within {0}."
def timeoutCancelingAfter(param0: Any): String =
rawTimeoutCancelingAfter.replaceAllLiterally("{0}", param0.toString())
final val rawTestTimeLimitExceeded = "The test did not complete within the specified {0} time limit."
def testTimeLimitExceeded(param0: Any): String =
rawTestTimeLimitExceeded.replaceAllLiterally("{0}", param0.toString())
final val rawSingularNanosecondUnits = "{0} nanosecond"
def singularNanosecondUnits(param0: Any): String =
rawSingularNanosecondUnits.replaceAllLiterally("{0}", param0.toString())
final val rawPluralNanosecondUnits = "{0} nanoseconds"
def pluralNanosecondUnits(param0: Any): String =
rawPluralNanosecondUnits.replaceAllLiterally("{0}", param0.toString())
final val rawSingularMicrosecondUnits = "{0} microsecond"
def singularMicrosecondUnits(param0: Any): String =
rawSingularMicrosecondUnits.replaceAllLiterally("{0}", param0.toString())
final val rawPluralMicrosecondUnits = "{0} microseconds"
def pluralMicrosecondUnits(param0: Any): String =
rawPluralMicrosecondUnits.replaceAllLiterally("{0}", param0.toString())
final val rawSingularMillisecondUnits = "{0} millisecond"
def singularMillisecondUnits(param0: Any): String =
rawSingularMillisecondUnits.replaceAllLiterally("{0}", param0.toString())
final val rawPluralMillisecondUnits = "{0} milliseconds"
def pluralMillisecondUnits(param0: Any): String =
rawPluralMillisecondUnits.replaceAllLiterally("{0}", param0.toString())
final val rawSingularSecondUnits = "{0} second"
def singularSecondUnits(param0: Any): String =
rawSingularSecondUnits.replaceAllLiterally("{0}", param0.toString())
final val rawPluralSecondUnits = "{0} seconds"
def pluralSecondUnits(param0: Any): String =
rawPluralSecondUnits.replaceAllLiterally("{0}", param0.toString())
final val rawSingularMinuteUnits = "{0} minute"
def singularMinuteUnits(param0: Any): String =
rawSingularMinuteUnits.replaceAllLiterally("{0}", param0.toString())
final val rawPluralMinuteUnits = "{0} minutes"
def pluralMinuteUnits(param0: Any): String =
rawPluralMinuteUnits.replaceAllLiterally("{0}", param0.toString())
final val rawSingularHourUnits = "{0} hour"
def singularHourUnits(param0: Any): String =
rawSingularHourUnits.replaceAllLiterally("{0}", param0.toString())
final val rawPluralHourUnits = "{0} hours"
def pluralHourUnits(param0: Any): String =
rawPluralHourUnits.replaceAllLiterally("{0}", param0.toString())
final val rawSingularDayUnits = "{0} day"
def singularDayUnits(param0: Any): String =
rawSingularDayUnits.replaceAllLiterally("{0}", param0.toString())
final val rawPluralDayUnits = "{0} days"
def pluralDayUnits(param0: Any): String =
rawPluralDayUnits.replaceAllLiterally("{0}", param0.toString())
final val rawLeftAndRight = "{0} and {1}"
def leftAndRight(param0: Any, param1: Any): String =
rawLeftAndRight.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawLeftCommaAndRight = "{0}, and {1}"
def leftCommaAndRight(param0: Any, param1: Any): String =
rawLeftCommaAndRight.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawConfigMapEntryNotFound = "The config map did not contain required entry with key \"{0}\"."
def configMapEntryNotFound(param0: Any): String =
rawConfigMapEntryNotFound.replaceAllLiterally("{0}", param0.toString())
final val rawConfigMapEntryHadUnexpectedType = "The config map entry with key \"{0}\" had an unexpected (runtime) type: {1}. The expected (runtime) type was: {2}. The value was: {3}."
def configMapEntryHadUnexpectedType(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawConfigMapEntryHadUnexpectedType.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawForAssertionsMoreThanZero = "{0} argument must be more than 0"
def forAssertionsMoreThanZero(param0: Any): String =
rawForAssertionsMoreThanZero.replaceAllLiterally("{0}", param0.toString())
final val rawForAssertionsMoreThanEqualZero = "{0} argument must be more than or equal 0"
def forAssertionsMoreThanEqualZero(param0: Any): String =
rawForAssertionsMoreThanEqualZero.replaceAllLiterally("{0}", param0.toString())
final val rawForAssertionsMoreThan = "{0} argument must be more than {1} argument"
def forAssertionsMoreThan(param0: Any, param1: Any): String =
rawForAssertionsMoreThan.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawForAssertionsGenTraversableMessageWithStackDepth = "at index {0}, {1} ({2})"
def forAssertionsGenTraversableMessageWithStackDepth(param0: Any, param1: Any, param2: Any): String =
rawForAssertionsGenTraversableMessageWithStackDepth.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawForAssertionsGenTraversableMessageWithoutStackDepth = "at index {0}, {1}"
def forAssertionsGenTraversableMessageWithoutStackDepth(param0: Any, param1: Any): String =
rawForAssertionsGenTraversableMessageWithoutStackDepth.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawForAssertionsGenMapMessageWithStackDepth = "at key {0}, {1} ({2})"
def forAssertionsGenMapMessageWithStackDepth(param0: Any, param1: Any, param2: Any): String =
rawForAssertionsGenMapMessageWithStackDepth.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawForAssertionsGenMapMessageWithoutStackDepth = "at key {0}, {1}"
def forAssertionsGenMapMessageWithoutStackDepth(param0: Any, param1: Any): String =
rawForAssertionsGenMapMessageWithoutStackDepth.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawForAssertionsNoElement = "no element"
final val forAssertionsNoElement = rawForAssertionsNoElement
final val rawForAssertionsElement = "{0} element"
def forAssertionsElement(param0: Any): String =
rawForAssertionsElement.replaceAllLiterally("{0}", param0.toString())
final val rawForAssertionsElements = "{0} elements"
def forAssertionsElements(param0: Any): String =
rawForAssertionsElements.replaceAllLiterally("{0}", param0.toString())
final val rawForAssertionsIndexLabel = "index {0}"
def forAssertionsIndexLabel(param0: Any): String =
rawForAssertionsIndexLabel.replaceAllLiterally("{0}", param0.toString())
final val rawForAssertionsIndexAndLabel = "index {0} and {1}"
def forAssertionsIndexAndLabel(param0: Any, param1: Any): String =
rawForAssertionsIndexAndLabel.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawForAssertionsKeyLabel = "key {0}"
def forAssertionsKeyLabel(param0: Any): String =
rawForAssertionsKeyLabel.replaceAllLiterally("{0}", param0.toString())
final val rawForAssertionsKeyAndLabel = "key {0} and {1}"
def forAssertionsKeyAndLabel(param0: Any, param1: Any): String =
rawForAssertionsKeyAndLabel.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawForAllFailed = "forAll failed, because: \n{0} \nin {1}"
def forAllFailed(param0: Any, param1: Any): String =
rawForAllFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawAllShorthandFailed = "'all' inspection failed, because: \n{0} \nin {1}"
def allShorthandFailed(param0: Any, param1: Any): String =
rawAllShorthandFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawForAtLeastFailedNoElement = "forAtLeast({0}) failed, because no element satisfied the assertion block: \n{1} \nin {2}"
def forAtLeastFailedNoElement(param0: Any, param1: Any, param2: Any): String =
rawForAtLeastFailedNoElement.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawForAtLeastFailed = "forAtLeast({0}) failed, because only {1} satisfied the assertion block: \n{2} \nin {3}"
def forAtLeastFailed(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawForAtLeastFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawAtLeastShorthandFailedNoElement = "'atLeast({0})' inspection failed, because no element satisfied the assertion block: \n{1} \nin {2}"
def atLeastShorthandFailedNoElement(param0: Any, param1: Any, param2: Any): String =
rawAtLeastShorthandFailedNoElement.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawAtLeastShorthandFailed = "'atLeast({0})' inspection failed, because only {1} satisfied the assertion block: \n{2} \nin {3}"
def atLeastShorthandFailed(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawAtLeastShorthandFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawForAtMostFailed = "forAtMost({0}) failed, because {1} elements satisfied the assertion block at {2} in {3}"
def forAtMostFailed(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawForAtMostFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawAtMostShorthandFailed = "'atMost({0})' inspection failed, because {1} elements satisfied the assertion block at {2} in {3}"
def atMostShorthandFailed(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawAtMostShorthandFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawForExactlyFailedNoElement = "forExactly({0}) failed, because no element satisfied the assertion block: \n{1} \nin {2}"
def forExactlyFailedNoElement(param0: Any, param1: Any, param2: Any): String =
rawForExactlyFailedNoElement.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawForExactlyFailedLess = "forExactly({0}) failed, because only {1} satisfied the assertion block at {2}: \n{3} \nin {4}"
def forExactlyFailedLess(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any): String =
rawForExactlyFailedLess.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString())
final val rawForExactlyFailedMore = "forExactly({0}) failed, because {1} satisfied the assertion block at {2} in {3}"
def forExactlyFailedMore(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawForExactlyFailedMore.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawExactlyShorthandFailedNoElement = "'exactly({0})' inspection failed, because no element satisfied the assertion block: \n{1} \nin {2}"
def exactlyShorthandFailedNoElement(param0: Any, param1: Any, param2: Any): String =
rawExactlyShorthandFailedNoElement.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawExactlyShorthandFailedLess = "'exactly({0})' inspection failed, because only {1} satisfied the assertion block at {2}: \n{3} \nin {4}"
def exactlyShorthandFailedLess(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any): String =
rawExactlyShorthandFailedLess.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString())
final val rawExactlyShorthandFailedMore = "'exactly({0})' inspection failed, because {1} satisfied the assertion block at {2} in {3}"
def exactlyShorthandFailedMore(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawExactlyShorthandFailedMore.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawForNoFailed = "forNo failed, because 1 element satisfied the assertion block at {0} in {1}"
def forNoFailed(param0: Any, param1: Any): String =
rawForNoFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawNoShorthandFailed = "'no' inspection failed, because 1 element satisfied the assertion block at {0} in {1}"
def noShorthandFailed(param0: Any, param1: Any): String =
rawNoShorthandFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawForBetweenFailedNoElement = "forBetween({0}, {1}) failed, because no element satisfied the assertion block: \n{2} \nin {3}"
def forBetweenFailedNoElement(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawForBetweenFailedNoElement.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawForBetweenFailedLess = "forBetween({0}, {1}) failed, because only {2} satisfied the assertion block at {3}: \n{4} \nin {5}"
def forBetweenFailedLess(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any, param5: Any): String =
rawForBetweenFailedLess.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString()).replaceAllLiterally("{5}", param5.toString())
final val rawForBetweenFailedMore = "forBetween({0}, {1}) failed, because {2} satisfied the assertion block at {3} in {4}"
def forBetweenFailedMore(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any): String =
rawForBetweenFailedMore.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString())
final val rawBetweenShorthandFailedNoElement = "'between({0}, {1})' inspection failed, because no element satisfied the assertion block: \n{2} \nin {3}"
def betweenShorthandFailedNoElement(param0: Any, param1: Any, param2: Any, param3: Any): String =
rawBetweenShorthandFailedNoElement.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString())
final val rawBetweenShorthandFailedLess = "'between({0}, {1})' inspection failed, because only {2} satisfied the assertion block at {3}: \n{4} \nin {5}"
def betweenShorthandFailedLess(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any, param5: Any): String =
rawBetweenShorthandFailedLess.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString()).replaceAllLiterally("{5}", param5.toString())
final val rawBetweenShorthandFailedMore = "'between({0}, {1})' inspection failed, because {2} satisfied the assertion block at {3} in {4}"
def betweenShorthandFailedMore(param0: Any, param1: Any, param2: Any, param3: Any, param4: Any): String =
rawBetweenShorthandFailedMore.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString()).replaceAllLiterally("{3}", param3.toString()).replaceAllLiterally("{4}", param4.toString())
final val rawForEveryFailed = "forEvery failed, because: \n{0} \nin {1}"
def forEveryFailed(param0: Any, param1: Any): String =
rawForEveryFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawEveryShorthandFailed = "'every' inspection failed, because: \n{0} \nin {1}"
def everyShorthandFailed(param0: Any, param1: Any): String =
rawEveryShorthandFailed.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawDiscoveryStarting = "Discovery starting."
final val discoveryStarting = rawDiscoveryStarting
final val rawDiscoveryCompleted = "Discovery completed."
final val discoveryCompleted = rawDiscoveryCompleted
final val rawDiscoveryCompletedIn = "Discovery completed in {0}."
def discoveryCompletedIn(param0: Any): String =
rawDiscoveryCompletedIn.replaceAllLiterally("{0}", param0.toString())
final val rawDoingDiscovery = "Discovering Suites to run..."
final val doingDiscovery = rawDoingDiscovery
final val rawAtCheckpointAt = "(in Checkpoint) at"
final val atCheckpointAt = rawAtCheckpointAt
final val rawSlowpokeDetected = "Test still running after {0}: suite name: {1}, test name: {2}."
def slowpokeDetected(param0: Any, param1: Any, param2: Any): String =
rawSlowpokeDetected.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawAlertFormattedText = "*** {0}"
def alertFormattedText(param0: Any): String =
rawAlertFormattedText.replaceAllLiterally("{0}", param0.toString())
final val rawNoteFormattedText = "*** {0}"
def noteFormattedText(param0: Any): String =
rawNoteFormattedText.replaceAllLiterally("{0}", param0.toString())
final val rawTestFlickered = "Test canceled because flickered: initially failed, but succeeded on retry"
final val testFlickered = rawTestFlickered
final val rawCannotRerun = "Test in memory file is not rerunnable: rerun event = {0}, suiteId = {1}, testName = {2}."
def cannotRerun(param0: Any, param1: Any, param2: Any): String =
rawCannotRerun.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawTestCannotBeNestedInsideAnotherTest = "Test cannot be nested inside another test."
final val testCannotBeNestedInsideAnotherTest = rawTestCannotBeNestedInsideAnotherTest
final val rawNonEmptyMatchPatternCase = "No code is allowed to the right of rocket symbols (=>) in a partial function passed to matchPattern, because matchPattern is intended only for ensuring that an expression matches a pattern. If you want to make further assertions after a successful pattern match, use org.scalatest.Inside instead."
final val nonEmptyMatchPatternCase = rawNonEmptyMatchPatternCase
final val rawExpectedTypeErrorButGotNone = "Expected a type error, but got none for code: {0}"
def expectedTypeErrorButGotNone(param0: Any): String =
rawExpectedTypeErrorButGotNone.replaceAllLiterally("{0}", param0.toString())
final val rawGotTypeErrorAsExpected = "Got a type error as expected for code: {0}"
def gotTypeErrorAsExpected(param0: Any): String =
rawGotTypeErrorAsExpected.replaceAllLiterally("{0}", param0.toString())
final val rawExpectedCompileErrorButGotNone = "Expected a compiler error, but got none for code: {0}"
def expectedCompileErrorButGotNone(param0: Any): String =
rawExpectedCompileErrorButGotNone.replaceAllLiterally("{0}", param0.toString())
final val rawDidNotCompile = "{0} did not compile"
def didNotCompile(param0: Any): String =
rawDidNotCompile.replaceAllLiterally("{0}", param0.toString())
final val rawCompiledSuccessfully = "{0} compiled successfully"
def compiledSuccessfully(param0: Any): String =
rawCompiledSuccessfully.replaceAllLiterally("{0}", param0.toString())
final val rawExpectedTypeErrorButGotParseError = "Expected a type error, but got the following parse error: \"{0}\", for code: {1}"
def expectedTypeErrorButGotParseError(param0: Any, param1: Any): String =
rawExpectedTypeErrorButGotParseError.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawExpectedNoErrorButGotTypeError = "Expected no compiler error, but got the following type error: \"{0}\", for code: {1}"
def expectedNoErrorButGotTypeError(param0: Any, param1: Any): String =
rawExpectedNoErrorButGotTypeError.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawExpectedNoErrorButGotParseError = "Expected no compiler error, but got the following parse error: \"{0}\", for code: {1}"
def expectedNoErrorButGotParseError(param0: Any, param1: Any): String =
rawExpectedNoErrorButGotParseError.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawAnExpressionOfTypeNullIsIneligibleForImplicitConversion = "an expression of type Null is ineligible for implicit conversion"
final val anExpressionOfTypeNullIsIneligibleForImplicitConversion = rawAnExpressionOfTypeNullIsIneligibleForImplicitConversion
final val rawBeTripleEqualsNotAllowed = "The deprecation cycle for 'be ===' has finished and the syntax is no longer supported. Please use equal, be, or === syntax instead."
final val beTripleEqualsNotAllowed = rawBeTripleEqualsNotAllowed
final val rawAssertionShouldBePutInsideItOrTheyClauseNotDescribeClause = "Assertion should be put inside it or they clause, not describe clause."
final val assertionShouldBePutInsideItOrTheyClauseNotDescribeClause = rawAssertionShouldBePutInsideItOrTheyClauseNotDescribeClause
final val rawExceptionWasThrownInDescribeClause = "{0} was thrown inside describe({1}), construction cannot continue: {2}"
def exceptionWasThrownInDescribeClause(param0: Any, param1: Any, param2: Any): String =
rawExceptionWasThrownInDescribeClause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawAssertionShouldBePutInsideInClauseNotDashClause = "Assertion should be put inside in clause, not - clause."
final val assertionShouldBePutInsideInClauseNotDashClause = rawAssertionShouldBePutInsideInClauseNotDashClause
final val rawExceptionWasThrownInDashClause = "{0} was thrown inside {1} -, construction cannot continue: {2}"
def exceptionWasThrownInDashClause(param0: Any, param1: Any, param2: Any): String =
rawExceptionWasThrownInDashClause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawAssertionShouldBePutInsideScenarioClauseNotFeatureClause = "Assertion should be put inside scenario clause, not feature clause."
final val assertionShouldBePutInsideScenarioClauseNotFeatureClause = rawAssertionShouldBePutInsideScenarioClauseNotFeatureClause
final val rawExceptionWasThrownInFeatureClause = "{0} was thrown scenario({1}) -, construction cannot continue: {2}"
def exceptionWasThrownInFeatureClause(param0: Any, param1: Any, param2: Any): String =
rawExceptionWasThrownInFeatureClause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawAssertionShouldBePutInsideItOrTheyClauseNotShouldMustWhenThatWhichOrCanClause = "Assertion should be put inside it or they clause, not should, must, when, that, which or can clause."
final val assertionShouldBePutInsideItOrTheyClauseNotShouldMustWhenThatWhichOrCanClause = rawAssertionShouldBePutInsideItOrTheyClauseNotShouldMustWhenThatWhichOrCanClause
final val rawExceptionWasThrownInShouldClause = "{0} was thrown inside {1} should, construction cannot continue: {2}"
def exceptionWasThrownInShouldClause(param0: Any, param1: Any, param2: Any): String =
rawExceptionWasThrownInShouldClause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawExceptionWasThrownInMustClause = "{0} was thrown inside {1} must, construction cannot continue: {2}"
def exceptionWasThrownInMustClause(param0: Any, param1: Any, param2: Any): String =
rawExceptionWasThrownInMustClause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawExceptionWasThrownInWhenClause = "{0} was thrown inside {1} when, construction cannot continue: {2}"
def exceptionWasThrownInWhenClause(param0: Any, param1: Any, param2: Any): String =
rawExceptionWasThrownInWhenClause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawExceptionWasThrownInThatClause = "{0} was thrown inside {1} that, construction cannot continue: {2}"
def exceptionWasThrownInThatClause(param0: Any, param1: Any, param2: Any): String =
rawExceptionWasThrownInThatClause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawExceptionWasThrownInWhichClause = "{0} was thrown inside {1} which, construction cannot continue: {2}"
def exceptionWasThrownInWhichClause(param0: Any, param1: Any, param2: Any): String =
rawExceptionWasThrownInWhichClause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawExceptionWasThrownInCanClause = "{0} was thrown inside {1} can, construction cannot continue: {2}"
def exceptionWasThrownInCanClause(param0: Any, param1: Any, param2: Any): String =
rawExceptionWasThrownInCanClause.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString()).replaceAllLiterally("{2}", param2.toString())
final val rawAssertionShouldBePutInsideDefNotObject = "Assertion should be put inside def, not object."
final val assertionShouldBePutInsideDefNotObject = rawAssertionShouldBePutInsideDefNotObject
final val rawExceptionWasThrownInObject = "{0} was thrown inside object `{1}`, construction cannot continue."
def exceptionWasThrownInObject(param0: Any, param1: Any): String =
rawExceptionWasThrownInObject.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawTableDrivenForEveryFailed = "tableDrivenForEveryFailed: {0}"
def tableDrivenForEveryFailed(param0: Any): String =
rawTableDrivenForEveryFailed.replaceAllLiterally("{0}", param0.toString())
final val rawTableDrivenExistsFailed = "tableDrivenExistsFailed: {0}"
def tableDrivenExistsFailed(param0: Any): String =
rawTableDrivenExistsFailed.replaceAllLiterally("{0}", param0.toString())
final val rawWithFixtureNotAllowedInAsyncFixtures = "withFixture is not allowed in AsyncFixtures"
final val withFixtureNotAllowedInAsyncFixtures = rawWithFixtureNotAllowedInAsyncFixtures
final val rawLeftParensCommaBut = "({0}), but {1}"
def leftParensCommaBut(param0: Any, param1: Any): String =
rawLeftParensCommaBut.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawRightParensCommaBut = "{0}, but ({1})"
def rightParensCommaBut(param0: Any, param1: Any): String =
rawRightParensCommaBut.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawBothParensCommaBut = "({0}), but ({1})"
def bothParensCommaBut(param0: Any, param1: Any): String =
rawBothParensCommaBut.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawLeftParensCommaAnd = "({0}), and {1}"
def leftParensCommaAnd(param0: Any, param1: Any): String =
rawLeftParensCommaAnd.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawRightParensCommaAnd = "{0}, and ({1})"
def rightParensCommaAnd(param0: Any, param1: Any): String =
rawRightParensCommaAnd.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawBothParensCommaAnd = "({0}), and ({1})"
def bothParensCommaAnd(param0: Any, param1: Any): String =
rawBothParensCommaAnd.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawAssertionWasTrue = "Assertion was true"
final val assertionWasTrue = rawAssertionWasTrue
final val rawFromEqualToToHavingLengthsBetween = "The from and to values passed to havingLengthsBetween must not be equal, but were both {0}. Please use the havingLength method instead."
def fromEqualToToHavingLengthsBetween(param0: Any): String =
rawFromEqualToToHavingLengthsBetween.replaceAllLiterally("{0}", param0.toString())
final val rawFromGreaterThanToHavingLengthsBetween = "The from value passed to havingLengthsBetween, which was {0}, must be less than the to value, which was {1}. Please swap the order of the arguments."
def fromGreaterThanToHavingLengthsBetween(param0: Any, param1: Any): String =
rawFromGreaterThanToHavingLengthsBetween.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawFromEqualToToHavingSizesBetween = "The from and to values passed to havingSizesBetween must not be equal, but were both {0}. Please use the havingLength method instead."
def fromEqualToToHavingSizesBetween(param0: Any): String =
rawFromEqualToToHavingSizesBetween.replaceAllLiterally("{0}", param0.toString())
final val rawFromGreaterThanToHavingSizesBetween = "The from value passed to havingSizesBetween, which was {0}, must be less than the to value, which was {1}. Please swap the order of the arguments."
def fromGreaterThanToHavingSizesBetween(param0: Any, param1: Any): String =
rawFromGreaterThanToHavingSizesBetween.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawAnalysis = "Analysis:"
final val analysis = rawAnalysis
final val rawDeprecatedChosenStyleWarning = "Warning: The chosen style has been deprecated and will be removed in future version of ScalaTest, because each style trait will be available as its own module."
final val deprecatedChosenStyleWarning = rawDeprecatedChosenStyleWarning
final val rawFlexmarkClassNotFound = "Flexmark Pegdown adapter class not found, please add flexmark-all (https://mvnrepository.com/artifact/com.vladsch.flexmark/flexmark/0.62.2) to your test classpath."
final val flexmarkClassNotFound = rawFlexmarkClassNotFound
final val rawUnableToSerializePayload = "Warning: Unable to serialize payload of type {0} for {1}, wrapping it as NotSerializableWrapperException."
def unableToSerializePayload(param0: Any, param1: Any): String =
rawUnableToSerializePayload.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawUnableToSerializeThrowable = "Warning: Unable to serialize throwable of type {0} for {1}, setting it as NotSerializableWrapperException."
def unableToSerializeThrowable(param0: Any, param1: Any): String =
rawUnableToSerializeThrowable.replaceAllLiterally("{0}", param0.toString()).replaceAllLiterally("{1}", param1.toString())
final val rawUnableToReadSerializedEvent = "Warning: Unable to read from client, please check on client for further details of the problem."
final val unableToReadSerializedEvent = rawUnableToReadSerializedEvent
final val rawUnableToContinueRun = "Fatal: Existing as unable to continue running tests, after 3 failing attempts to read event from server socket. "
final val unableToContinueRun = rawUnableToContinueRun
def bigProblems(ex: Throwable): String = {
val message = if (ex.getMessage == null) "" else ex.getMessage.trim
if (message.length > 0) Resources.bigProblemsWithMessage(message) else Resources.bigProblems
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy