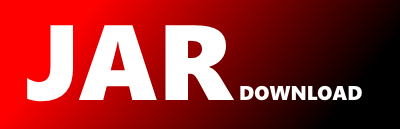
org.scalautils.Uniformity.scala Maven / Gradle / Ivy
Show all versions of scalatest_2.11.0-M5 Show documentation
/* * Copyright 2001-2013 Artima, Inc. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package org.scalautils /** * Defines a custom way to normalize instances of a type that can also handle normalization of that type when passed as
A so that it can be safely passed toAny
. * **
* * @tparam A the type whose uniformity is being defined */ trait Uniformity[A] extends Normalization[A] { thisUniformity => // TODO: Add an example of Array[String] isInstanceOfA here and in NormalizedEquality /* TODO: Need to fix this scaladoc! * Indicates whether the passed object is an instance of typeA
. * ** This method is invoked by the
areEqual
method of subclassNormalizedEquality
to determine whether or not *b
can be cast tonormalized
. * To implement this method, simply callb.isInstanceOf[A]
for the actualA
type. * For example, if you are defining aNormalizedEquality[String]
, yourisInstanceOf
* method should look like: * * ** def isInstanceOfA(b: Any) = b.isInstanceOf[String] ** ** If you are defining a
* *NormalizedEquality[xml.Node]
yourisInstanceOf
method * should look like: ** def isInstanceOfA(b: Any) = b.isInstanceOf[xml.Node] ** * @param b the object to inspect to determine whether it is an instance ofA
* @return true if the passed object is an instance of
A
*/ /** * Returns either the result of passing this object tonormalized
, if appropriate, or the same object. */ def normalizedOrSame(b: Any): Any /** * Indicates whether thisUniformity
'snormalized
method can handle the passed object, if cast to the appropriate type. * ** If this method returns true for a particular passed object, it means that if the object is passed * to
* *normalizedOrSame
, that method will return the result of passing it tonormalized
. * It does not mean that the object will necessarily be modified when passed tonormalizedOrSame
ornormalized
. * For example, thelowerCased
field ofStringNormalizations
is aUniformity[String]
* that normalizes strings by forcing all characters to lower case: ** scala> import org.scalautils._ * import org.scalautils._ * * scala> import StringNormalizations._ * import StringNormalizations._ * * scala> lowerCased * res0: org.scalautils.Uniformity[String] = org.scalautils.StringNormalizations$$anon$1@236db810 * * scala> lowerCased.normalized("HALLOOO!") * res1: String = hallooo! ** ** Now consider two strings held from variables of type
* *AnyRef
: ** scala> val yell: AnyRef = "HOWDY" * yell: AnyRef = HOWDY * * scala> val whisper: AnyRef = "howdy" * whisper: AnyRef = howdy ** ** As you would expect, when
* *yell
is passed tonormalizedCanHandle
, it returns true, and when *yell
is passed tonormalizedOrSame
, it returns a lower-cased (normal) form: ** scala> lowerCased.normalizedCanHandle(yell) * res2: Boolean = true * * scala> lowerCased.normalizedOrSame(yell) * res3: Any = howdy ** ** A similar thing happens, however, when
* *whisper
is passed tonormalizedCanHandle
andnormalizedOrSame
, * even though in this case the string is already in normal form according to thelowerCased
Uniformity
: ** scala> lowerCased.normalizedCanHandle(whisper) * res4: Boolean = true * * scala> lowerCased.normalizedOrSame(whisper) * res5: Any = howdy ** ** This illustrates that
* *normalizedCanHandle
does not indicate that the passed object is not in normalized form already, just that * it can be be handled by thenormalized
method. This further means that thenormalized
method itself * simply ensures that objects are returned in normal form. It need not necessarily change them: if a passed object is already in * normal form,normalized
can (and usually should) return the exact same object. That is in fact what happened when we normalized *whisper
. Sincewhisper
's value of"hello"
was already in normal form (all lower-cased),normalized
( * invoked by thenormalizedOrSame
method) returned the exact same object passed: ** scala> val whisperNormed = res5.asInstanceOf[AnyRef] * whisperNormed: AnyRef = howdy * * scala> whisperNormed eq whisper * res8: Boolean = true **/ def normalizedCanHandle(b: Any): Boolean /** * Returns a newUniformity
that combines this and the passedUniformity
. * ** The
* *normalized
,normalizeCanHandle
, andnormalizedOrSame
methods * of theUniformity
's returned by this method return a result * obtained by passing it first to thisNormalization
's implementation of the method, * then passing that result to the otherNormalization
'snormalized
the method, respectively. * Essentially, the body of the composednormalized
method is: ** uniformityPassedToAnd.normalized(uniformityOnWhichAndWasInvoked.normalized(a)) ** ** The body of the composed
* *normalizeCanHandle
method is: ** uniformityPassedToAnd.normalizeCanHandle(uniformityOnWhichAndWasInvoked.normalizeCanHandle(a)) ** ** And the body of the composed
* *normalizedOrSame
method is: ** uniformityPassedToAnd.normalizedOrSame(uniformityOnWhichAndWasInvoked.normalizedOrSame(a)) ** ** If the passed object is already in normal form, this method may return the same instance passed. *
* * @param other aUniformity
to 'and' with this one * @return aUniformity
representing the composition of this and the passedUniformity
*/ final def and(other: Uniformity[A]): Uniformity[A] = new Uniformity[A] { // Note in Scaladoc what order, and recommend people don't do side effects anyway. // By order, I mean left's normalized gets called first then right's normalized gets called on that result, for "left and right" def normalized(a: A): A = other.normalized(thisUniformity.normalized(a)) def normalizedCanHandle(b: Any): Boolean = other.normalizedCanHandle(b) || thisUniformity.normalizedCanHandle(b) def normalizedOrSame(b: Any): Any = other.normalizedOrSame(thisUniformity.normalizedOrSame(b)) } }