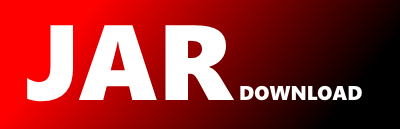
org.scalautils.LegacyTripleEquals.scala Maven / Gradle / Ivy
Show all versions of scalautils_2.11 Show documentation
/* * Copyright 2001-2013 Artima, Inc. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package org.scalautils import TripleEqualsSupport._ /** * Provides
Equalizer. * * *===
and!==
operators that returnBoolean
, delegate the equality determination * to anEquality
type class, and require no relationship between the types of the two values compared. * ** *
* Recommended Usage: * Trait LegacyTripleEquals
is useful (in test, not production, code) when you need to determine equality for a type of object differently than its *equals
method: either you can't change theequals
method, or theequals
method is sensible generally, but * you are in a special situation where you need something else. If you also want a compile-time type check, however, you should use one * ofLegacyTripleEquals
sibling traits: *ConversionCheckedLegacyTripleEquals
orTypeCheckedLegacyTripleEquals
. ** * Note: This trait is extended by
* *org.scalatest.Assertions
, because it provides the same kind of===
operator that was * historically provided byAssertions
. * The purpose of this trait is to maintain compatibility with existing ScalaTest code that uses the original===
operator. After * ScalaTest no longer supports Scala 2.9, the “legacy” triple equals traits will be deprecated and eventually removed. Good error messages will * be obtained for both==
and===
through assert macros. In the transition phase, you can in production code use regular triple equals traits, * whose===
operators returnBoolean
, and in test code use "legacy" triple equals traits, whose===
* operators returnOption[String]
. * ** This trait will override or hide implicit methods defined by its sibling traits, *
* *ConversionCheckedLegacyTripleEquals
orTypeCheckedLegacyTripleEquals
, * and can therefore be used to temporarily turn off type checking in a limited scope. * Because the methods inLegacyTripleEquals
(and its siblings) override all the methods defined in * supertypeTripleEqualsSupport
, you can achieve the same * kind of nested tuning of equality constraints whether you mix in traits, import from companion objects, or use some combination of both. ** In short, you should be able to select a primary constraint level via either a mixin or import, then change that in nested scopes * however you want, again either through a mixin or import, without getting any implicit conversion ambiguity. The innermost constraint level in scope * will always be in force. *
* * @author Bill Venners */ @deprecated("org.scalautils.LegacyTripleEquals has been deprecated and will be removed in a future version of ScalaTest. If you need this, please copy the source code into your own trait instead.") trait LegacyTripleEquals extends TripleEqualsSupport { import scala.language.implicitConversions implicit override def unconstrainedEquality[A, B](implicit equalityOfA: Equality[A]): Constraint[A, B] = new EqualityConstraint[A, B](equalityOfA) override def convertToEqualizer[T](left: T): Equalizer[T] = new Equalizer(left) override def convertToCheckingEqualizer[T](left: T): CheckingEqualizer[T] = new CheckingEqualizer(left) implicit override def convertToLegacyEqualizer[T](left: T): LegacyEqualizer[T] = new LegacyEqualizer(left) override def convertToLegacyCheckingEqualizer[T](left: T): LegacyCheckingEqualizer[T] = new LegacyCheckingEqualizer(left) override def lowPriorityTypeCheckedConstraint[A, B](implicit equivalenceOfB: Equivalence[B], ev: A <:< B): Constraint[A, B] = new AToBEquivalenceConstraint[A, B](equivalenceOfB, ev) override def convertEquivalenceToAToBConstraint[A, B](equivalenceOfB: Equivalence[B])(implicit ev: A <:< B): Constraint[A, B] = new AToBEquivalenceConstraint[A, B](equivalenceOfB, ev) override def typeCheckedConstraint[A, B](implicit equivalenceOfA: Equivalence[A], ev: B <:< A): Constraint[A, B] = new BToAEquivalenceConstraint[A, B](equivalenceOfA, ev) override def convertEquivalenceToBToAConstraint[A, B](equivalenceOfA: Equivalence[A])(implicit ev: B <:< A): Constraint[A, B] = new BToAEquivalenceConstraint[A, B](equivalenceOfA, ev) override def lowPriorityConversionCheckedConstraint[A, B](implicit equivalenceOfB: Equivalence[B], cnv: A => B): Constraint[A, B] = new AToBEquivalenceConstraint[A, B](equivalenceOfB, cnv) override def convertEquivalenceToAToBConversionConstraint[A, B](equivalenceOfB: Equivalence[B])(implicit ev: A => B): Constraint[A, B] = new AToBEquivalenceConstraint[A, B](equivalenceOfB, ev) override def conversionCheckedConstraint[A, B](implicit equivalenceOfA: Equivalence[A], cnv: B => A): Constraint[A, B] = new BToAEquivalenceConstraint[A, B](equivalenceOfA, cnv) override def convertEquivalenceToBToAConversionConstraint[A, B](equivalenceOfA: Equivalence[A])(implicit ev: B => A): Constraint[A, B] = new BToAEquivalenceConstraint[A, B](equivalenceOfA, ev) /** * Implicit conversion from
Any
toEqualizer
, used to enable * assertions with===
comparisons. * ** For more information * on this mechanism, see the documentation for
* Because trait
* *Suite
mixes inAssertions
, this implicit conversion will always be * available by default in ScalaTestSuite
s. This is the only implicit conversion that is in scope by default in every * ScalaTestSuite
. Other implicit conversions offered by ScalaTest, such as those that support the matchers DSL * orinvokePrivate
, must be explicitly invited into your test code, either by mixing in a trait or importing the * members of its companion object. The reason ScalaTest requires you to invite in implicit conversions (with the exception of the * implicit conversion for===
operator) is because if one of ScalaTest's implicit conversions clashes with an * implicit conversion used in the code you are trying to test, your program won't compile. Thus there is a chance that if you * are ever trying to use a library or test some code that also offers an implicit conversion involving a===
operator, * you could run into the problem of a compiler error due to an ambiguous implicit conversion. If that happens, you can turn off * the implicit conversion offered by thisconvertToEqualizer
method simply by overriding the method in your *Suite
subclass, but not marking it as implicit: ** // In your Suite subclass * override def convertToEqualizer(left: Any) = new Equalizer(left) ** * @param left the object whose type to convert toEqualizer
. * @throws NullPointerException ifleft
isnull
. */ // implicit override def convertToEqualizer[T](left: T): Equalizer[T] = new Equalizer(left) } /** * Companion object to traitLegacyTripleEquals
that facilitates the importing ofLegacyTripleEquals
members as * an alternative to mixing it in. One use case is to importLegacyTripleEquals
members so you can use * them in the Scala interpreter: * ** $ scala -classpath scalatest.jar * Welcome to Scala version 2.10.0 * Type in expressions to have them evaluated. * Type :help for more information. * * scala> import org.scalautils._ * import org.scalautils._ * * scala> import LegacyTripleEquals._ * import LegacyTripleEquals._ * * scala> 1 + 1 === 2 * res0: Option[String] = None **/ object LegacyTripleEquals extends LegacyTripleEquals