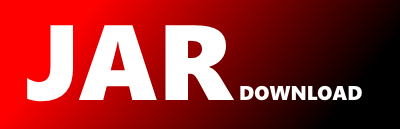
org.scalautils.EqualityConstraint.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scalautils_2.9.1 Show documentation
Show all versions of scalautils_2.9.1 Show documentation
ScalaUtils is an open-source library for Scala projects that
contains portions of the ScalaTest project that may make sense
to use in production.
The newest version!
/*
* Copyright 2001-2013 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalautils
import annotation.implicitNotFound
// I'm unaware of any reason this needs to be sealed, but can start out that way and loosen it if someone
// wants to create some other kind of constraint.
/**
* Abstract class used to enforce type constraints for equality checks.
*/
@implicitNotFound(msg = "types ${A} and ${B} do not adhere to the equality constraint selected for the === and !== operators; the missing implicit parameter is of type org.scalautils.EqualityConstraint[${A},${B}]")
sealed abstract class EqualityConstraint[A, B] {
/**
* Indicates whether the objects passed as a
and b
are equal.
*
* @param a a left-hand-side object being compared with another (right-hand-side one) for equality (e.g., a == b
)
* @param b a right-hand-side object being compared with another (left-hand-side one) for equality (e.g., a == b
)
*/
def areEqual(a: A, b: B): Boolean
}
/**
* An implementation of EqualityConstraint
for two types A
and B
that requires an Equality[A]
to
* which its areEqual
method can delegate an equality comparison.
*
* @param equalityofA an Equality
type class for A
*/
final class BasicEqualityConstraint[A, B](equalityOfA: Equality[A]) extends EqualityConstraint[A, B] {
/**
* Indicates whether the objects passed as a
and b
are equal by returning the
* result of invoking areEqual(a, b)
on the passed equalityOfA
object.
*
* @param a a left-hand-side object being compared with another (right-hand-side one) for equality (e.g., a == b
)
* @param b a right-hand-side object being compared with another (left-hand-side one) for equality (e.g., a == b
)
*/
def areEqual(a: A, b: B): Boolean = equalityOfA.areEqual(a, b)
}
/**
* An implementation of EqualityConstraint
for two types A
and B
that requires an Equality[A]
* and a conversion function from B
to A
.
*
* @param equalityofA an Equality
type class for A
*/
final class BToAEqualityConstraint[A, B](equalityOfA: Equality[A], cnv: B => A) extends EqualityConstraint[A, B] {
/**
* Indicates whether the objects passed as a
and b
are equal by returning the
* result of invoking areEqual(a, cnv(b))
on the passed equalityOfA
object.
*
*
* In other words, the b
object of type B
is first converted to an A
via the passed conversion
* function, cnv
, then compared for equality with the a
object.
*
*
* @param a a left-hand-side object being compared with another (right-hand-side one) for equality (e.g., a == b
)
* @param b a right-hand-side object being compared with another (left-hand-side one) for equality (e.g., a == b
)
*/
override def areEqual(a: A, b: B): Boolean = equalityOfA.areEqual(a, cnv(b))
}
/**
* An implementation of EqualityConstraint
for two types A
and B
that requires an Equality[B]
* and a conversion function from A
to B
.
*
* @param equalityofB an Equality
type class for B
*/
final class AToBEqualityConstraint[A, B](equalityOfB: Equality[B], cnv: A => B) extends EqualityConstraint[A, B] {
/**
* Indicates whether the objects passed as a
and b
are equal by return the
* result of invoking areEqual(cnv(a), b)
on the passed equalityOfB
object.
*
*
* In other words, the a
object of type A
is first converted to a B
via the passed conversion
* function, cnv
, then compared for equality with the b
object.
*
*
* @param a a left-hand-side object being compared with another (right-hand-side one) for equality (e.g., a == b
)
* @param b a right-hand-side object being compared with another (left-hand-side one) for equality (e.g., a == b
)
*/
override def areEqual(a: A, b: B): Boolean = equalityOfB.areEqual(cnv(a), b)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy