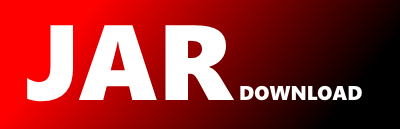
org.schwering.irc.lib.util.IRCCommand Maven / Gradle / Ivy
Show all versions of irclib Show documentation
/**
* IRClib - A Java Internet Relay Chat library
* Copyright (C) 2006-2015 Christoph Schwering
* and/or other contributors as indicated by the @author tags.
*
* This library and the accompanying materials are made available under the
* terms of the
* - GNU Lesser General Public License,
* - Apache License, Version 2.0 and
* - Eclipse Public License v1.0.
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY.
*/
package org.schwering.irc.lib.util;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* An enum of IRC commands. Adapted from http://en.wikipedia.org/wiki/List_of_Internet_Relay_Chat_commands
* Hopefully, the list is correct and complete.
*
* @author Peter Palaga
*/
public enum IRCCommand {
/**
* {@code ADMIN}
*
* Syntax:
*
*
* ADMIN [<servers>]
*
*
* Instructs the server to return information about the administrator of the
* server specified by <server>, or the current server if target is
* omitted.[1]
*/
ADMIN,
/**
* Syntax:
*
*
* AWAY [<message>]
*
*
* Provides the server with a message to automatically send in reply to a
* PRIVMSG directed at the user, but not to a channel they are on.[2] If
* <message> is omitted, the away status is removed.
*
* Defined in RFC 1459
*/
AWAY,
/**
* Syntax:
*
*
* CNOTICE <nickname> <channel> :<message>
*
*
* Sends a channel NOTICE message to <nickname> on <channel>
* that bypasses flood protection limits. The target nickname must be in the
* same channel as the client issuing the command, and the client must be a
* channel operator.
*
* Normally an IRC server will limit the number of different targets a
* client can send messages to within a certain time frame to prevent
* spammers or bots from mass-messaging users on the network, however this
* command can be used by channel operators to bypass that limit in their
* channel. For example, it is often used by help operators that may be
* communicating with a large number of users in a help channel at one time.
*
* This command is not formally defined in an RFC, but is in use by some IRC
* networks. Support is indicated in a RPL_ISUPPORT reply (numeric 005) with
* the CNOTICE keyword
*/
CNOTICE,
/**
* Syntax:
*
*
* CPRIVMSG <nickname> <channel> :<message>
*
*
* Sends a private message to <nickname> on <channel> that
* bypasses flood protection limits. The target nickname must be in the same
* channel as the client issuing the command, and the client must be a
* channel operator.
*
* Normally an IRC server will limit the number of different targets a
* client can send messages to within a certain time frame to prevent
* spammers or bots from mass-messaging users on the network, however this
* command can be used by channel operators to bypass that limit in their
* channel. For example, it is often used by help operators that may be
* communicating with a large number of users in a help channel at one time.
*
* This command is not formally defined in an RFC, but is in use by some IRC
* networks. Support is indicated in a RPL_ISUPPORT reply (numeric 005) with
* the CPRIVMSG keyword
*/
CPRIVMSG,
/**
* Syntax:
*
*
* CONNECT <target server> [<port> [<remote server>]] (RFC 1459)
*
*
*
* CONNECT <target server> <port> [<remote server>] (RFC 2812)
*
*
* Instructs the server <remote server> (or the current server, if
* <remote server> is omitted) to connect to <target server> on
* port <port>.[3][4] This command should only be available to IRC
* Operators.
*
* Defined in RFC 1459; the <port> parameter became mandatory in RFC
* 2812
*/
CONNECT,
/**
* Syntax:
*
*
* DIE
*
*
* Instructs the server to shut down.[5]
*
* Defined in RFC 2812
*/
DIE,
/**
* Syntax:
*
* :<source> ENCAP <destination> <subcommand>
* <parameters> This command is for use by servers to encapsulate
* commands so that they will propagate across hub servers not yet updated
* to support them, and indicates the subcommand and its parameters should
* be passed unaltered to the destination, where it will be unencapsulated
* and parsed. This facilitates implementation of new features without a
* need to restart all servers before they are usable across the network.[6]
*/
ENCAP,
/**
* Syntax:
*
*
* ERROR <error message>
*
*
* This command is for use by servers to report errors to other servers. It
* is also used before terminating client connections.[7]
*
* Defined in RFC 1459
*/
ERROR,
/**
* Syntax:
*
*
* HELP
*
*
* Requests the server help file.
*
* This command is not formally defined in an RFC, but is in use by most
* major IRC daemons.
*/
HELP,
/**
* Syntax:
*
*
* INFO [<target>]
*
*
* Returns information about the <target> server, or the current
* server if <target> is omitted.[8] Information returned includes the
* server's version, when it was compiled, the patch level, when it was
* started, and any other information which may be considered to be
* relevant.
*
* Defined in RFC 1459
*/
INFO,
/**
* Syntax:
*
*
* INVITE <nickname> <channel>
*
*
* Invites <nickname> to the channel <channel>.[9]
* <channel> does not have to exist, but if it does, only members of
* the channel are allowed to invite other clients. If the channel mode i is
* set, only channel operators may invite other clients.
*
* Defined in RFC 1459
*/
INVITE,
/**
* Syntax:
*
*
* ISON <nicknames>
*
*
* Queries the server to see if the clients in the space-separated list
* <nicknames> are currently on the network.[10] The server returns
* only the nicknames that are on the network in a space-separated list. If
* none of the clients are on the network the server returns an empty list.
*
* Defined in RFC 1459
*/
ISON,
/**
* Syntax:
*
*
* JOIN <channels> [<keys>]
*
*
* Makes the client join the channels in the comma-separated list
* <channels>, specifying the passwords, if needed, in the
* comma-separated list <keys>.[11] If the channel(s) do not exist
* then they will be created.
*
* Defined in RFC 1459
*/
JOIN,
/**
* Syntax:
*
*
* KICK <channel> <client> [<message>]
*
*
* Forcibly removes <client> from <channel>.[12] This command
* may only be issued by channel operators.
*
* Defined in RFC 1459
*/
KICK,
/**
* Syntax:
*
*
* KILL <client> <comment>
*
*
* Forcibly removes <client> from the network.[13] This command may
* only be issued by IRC operators.
*
* Defined in RFC 1459
*/
KILL,
/**
* Syntax:
*
*
* KNOCK <channel> [<message>]
*
*
* Sends a NOTICE to an invitation-only <channel> with an optional
* <message>, requesting an invite.
*
* This command is not formally defined by an RFC, but is supported by most
* major IRC daemons. Support is indicated in a RPL_ISUPPORT reply (numeric
* 005) with the KNOCK keyword.
*/
KNOCK,
/**
* Syntax:
*
*
* LINKS [<remote server> [<server mask>]]
*
*
* Lists all server links matching <server mask>, if given, on
* <remote server>, or the current server if omitted.[14]
*
* Defined in RFC 1459
*/
LINKS,
/**
* Syntax:
*
*
* LIST [<channels> [<server>]]
*
*
* Lists all channels on the server.[15] If the comma-separated list
* <channels> is given, it will return the channel topics. If
* <server> is given, the command will be forwarded to <server>
* for evaluation.
*
* Defined in RFC 1459
*/
LIST,
/**
* Syntax:
*
*
* LUSERS [<mask> [<server>]]
*
*
* Returns statistics about the size of the network.[16] If called with no
* arguments, the statistics will reflect the entire network. If
* <mask> is given, it will return only statistics reflecting the
* masked subset of the network. If <target> is given, the command
* will be forwarded to <server> for evaluation.
*
* Defined in RFC 2812
*/
LUSERS,
/**
* Syntax:
*
*
* MODE <nickname> <flags> (user)
*
*
*
* MODE <channel> <flags> [<args>]
*
*
* The MODE command is dual-purpose. It can be used to set both user and
* channel modes.[17]
*
* Defined in RFC 1459
*/
MODE,
/**
* Syntax:
*
*
* MOTD [<server>]
*
*
* Returns the message of the day on <server> or the current server if
* it is omitted.[18]
*
* Defined in RFC 2812
*/
MOTD,
/**
* Syntax:
*
*
* NAMES [<channels>] (RFC 1459)
*
*
*
* NAMES [<channels> [<server>]] (RFC 2812)
*
*
* Returns a list of who is on the comma-separated list of <channels>,
* by channel name.[19] If <channels> is omitted, all users are shown,
* grouped by channel name with all users who are not on a channel being
* shown as part of channel "*". If <server> is specified, the command
* is sent to <server> for evaluation.[20]
*
* Defined in RFC 1459; the optional <server> parameter was added in
* RFC 2812
*/
NAMES,
/**
* Syntax:
*
*
* PROTOCTL NAMESX
*
*
* Instructs the server to send names in an RPL_NAMES reply prefixed with
* their respective channel status. For example:
*
* With NAMESX
*
* :irc.server.net 353 Phyre = #SomeChannel :@+WiZ Without NAMESX
*
* :irc.server.net 353 Phyre = #SomeChannel :WiZ This command can ONLY be
* used if the NAMESX keyword is returned in an RPL_ISUPPORT (numeric 005)
* reply. It may also be combined with the UHNAMES command.
*
* This command is not formally defined in an RFC, but is recognized by most
* major IRC daemons.
*/
NAMESX,
/**
* Syntax:
*
*
* NICK <nickname> [<hopcount>] (RFC 1459)
*
*
*
* NICK <nickname> (RFC 2812)
*
*
* Allows a client to change their IRC nickname. Hopcount is for use between
* servers to specify how far away a nickname is from its home
* server.[21][22]
*
* Defined in RFC 1459; the optional <hopcount> parameter was removed
* in RFC 2812
*/
NICK,
/**
* Syntax:
*
*
* NOTICE <msgtarget> <message>
*
*
* This command works similarly to PRIVMSG, except automatic replies must
* never be sent in reply to NOTICE messages.[23]
*
* Defined in RFC 1459
*/
NOTICE,
/**
* Syntax:
*
*
* OPER <username> <password>
*
*
* Authenticates a user as an IRC operator on that server/network.[24]
*
* Defined in RFC 1459
*/
OPER,
/**
* Syntax:
*
*
* PART <channels> [<message>]
*
*
* Causes a user to leave the channels in the comma-separated list
* <channels>.[25]
*
* Defined in RFC 1459
*/
PART,
/**
* Syntax:
*
*
* PASS <password>
*
*
* Sets a connection password.[26] This command must be sent before the
* NICK/USER registration combination.
*
* Defined in RFC 1459
*/
PASS,
/**
* Syntax:
*
*
* PING <server1> [<server2>]
*
*
* Tests the presence of a connection.[27] A PING message results in a PONG
* reply. If <server2> is specified, the message gets passed on to it.
*
* Defined in RFC 1459
*/
PING,
/**
* Syntax:
*
*
* PONG <server1> [<server2>]
*
*
* This command is a reply to the PING command and works in much the same
* way.[28]
*
* Defined in RFC 1459
*/
PONG,
/**
* Syntax:
*
*
* PRIVMSG <msgtarget> <message>
*
*
* Sends <message> to <msgtarget>, which is usually a user or
* channel.[29]
*
* Defined in RFC 1459
*/
PRIVMSG,
/**
* Syntax:
*
*
* QUIT [<message>]
*
*
* Disconnects the user from the server.[30]
*
* Defined in RFC 1459
*/
QUIT,
/**
* Syntax:
*
*
* REHASH
*
*
* Causes the server to re-read and re-process its configuration
* file(s).[31] This command can only be sent by IRC Operators.
*
* Defined in RFC 1459
*/
REHASH,
/**
* Syntax:
*
*
* RESTART
*
*
* Restarts a server.[32] It may only be sent by IRC Operators.
*
* Defined in RFC 1459
*/
RESTART,
/**
* Syntax:
*
*
* RULES
*
*
* Requests the server rules.
*
* This command is not formally defined in an RFC, but is used by
* most[which?] major IRC daemons.
*/
RULES,
/**
* Syntax:
*
*
* SERVER <servername> <hopcount> <info>
*
*
* The server message is used to tell a server that the other end of a new
* connection is a server.[33] This message is also used to pass server data
* over whole net. <hopcount> details how many hops (server
* connections) away <servername> is. <info> contains addition
* human-readable information about the server.
*
* Defined in RFC 1459
*/
SERVER,
/**
* Syntax:
*
*
* SERVICE <nickname> <reserved> <distribution> <type> <reserved> <info>
*
*
* Registers a new service on the network.[34]
*
* Defined in RFC 2812
*/
SERVICE,
/**
* Syntax:
*
*
* SERVLIST [<mask> [<type>]]
*
*
* Lists the services currently on the network.[35]
*
* Defined in RFC 2812
*/
SERVLIST,
/**
* Syntax:
*
*
* SQUERY <servicename> <text>
*
*
* Identical to PRIVMSG except the recipient must be a service.[36]
*
* Defined in RFC 2812
*/
SQUERY,
/**
* Syntax:
*
*
* SQUIT <server> <comment>
*
*
* Causes <server> to quit the network.[37]
*
* Defined in RFC 1459
*/
SQUIT,
/**
* Syntax:
*
*
* SETNAME <new real name>
*
*
* Allows a client to change the "real name" specified when registering a
* connection.
*
* This command is not formally defined by an RFC, but is in use by some IRC
* daemons. Support is indicated in a RPL_ISUPPORT reply (numeric 005) with
* the SETNAME keyword
*/
SETNAME,
/**
* Syntax:
*
*
* SILENCE [+/-<hostmask>]
*
*
* Adds or removes a host mask to a server-side ignore list that prevents
* matching users from sending the client messages. More than one mask may
* be specified in a space-separated list, each item prefixed with a "+" or
* "-" to designate whether it is being added or removed. Sending the
* command with no parameters returns the entries in the client's ignore
* list.
*
* This command is not formally defined in an RFC, but is supported by
* most[which?] major IRC daemons. Support is indicated in a RPL_ISUPPORT
* reply (numeric 005) with the SILENCE keyword and the maximum number of
* entries a client may have in its ignore list. For example:
*
* :irc.server.net 005 WiZ WALLCHOPS WATCH=128 SILENCE=15 MODES=12
* CHANTYPES=#
*/
SILENCE,
/**
* Syntax:
*
*
* STATS <query> [<server>]
*
*
* Returns statistics about the current server, or <server> if it's
* specified.[38]
*
* Defined in RFC 1459
*/
STATS,
/**
* Syntax:
*
*
* SUMMON <user> [<server>] (RFC 1459)
*
*
*
* SUMMON <user> [<server> [<channel>]] (RFC 2812)
*
*
* Gives users who are on the same host as <server> a message asking
* them to join IRC.[39][40]
*
* Defined in RFC 1459; the optional <channel> parameter was added in
* RFC 2812
*/
SUMMON,
/**
* Syntax:
*
*
* TIME [<server>]
*
*
* Returns the local time on the current server, or <server> if
* specified.[41]
*
* Defined in RFC 1459
*/
TIME,
/**
* Syntax:
*
*
* TOPIC <channel> [<topic>]
*
*
* Allows the client to query or set the channel topic on
* <channel>.[42] If <topic> is given, it sets the channel topic
* to <topic>. If channel mode +t is set, only a channel operator may
* set the topic.
*
* Defined in RFC 1459
*/
TOPIC,
/**
* Syntax:
*
*
* TRACE [<target>]
*
*
* Trace a path across the IRC network to a specific server or client, in a
* similar method to traceroute.[43]
*
* Defined in RFC 1459
*/
TRACE,
/**
* Syntax:
*
*
* PROTOCTL UHNAMES
*
*
* Instructs the server to send names in an RPL_NAMES reply in the long
* format:
*
* With UHNAMES
*
* :irc.server.net 353 Phyre = #SomeChannel :WiZ!user@somehost Without
* UHNAMES
*
* :irc.server.net 353 Phyre = #SomeChannel :WiZ This command can ONLY be
* used if the UHNAMES keyword is returned in an RPL_ISUPPORT (numeric 005)
* reply. It may also be combined with the NAMESX command.
*
* This command is not formally defined in an RFC, but is recognized by most
* major IRC daemons.
*/
UHNAMES,
/**
* Syntax:
*
*
* USER <username> <hostname> <servername> <realname> (RFC 1459)
*
*
*
* USER <user> <mode> <unused> <realname> (RFC 2812)
*
*
* This command is used at the beginning of a connection to specify the
* username, hostname, real name and initial user modes of the connecting
* client.[44][45] <realname> may contain spaces, and thus must be
* prefixed with a colon.
*
* Defined in RFC 1459, modified in RFC 2812
*/
USER,
/**
* Syntatx:
*
*
* USERHOST <nickname> [<nickname> <nickname> ...]
*
*
* Returns a list of information about the nicknames specified.[46]
*
* Defined in RFC 1459
*/
USERHOST,
/**
* Syntax:
*
*
* USERIP <nickname>
*
*
* Requests the direct IP address of the user with the specified nickname.
*
* This command is often used to obtain the IP of an abusive user to more
* effectively perform a ban. It is unclear what, if any, privileges are
* required to execute this command on a server.
*
* This command is not formally defined by an RFC, but is in use by some IRC
* daemons. Support is indicated in a RPL_ISUPPORT reply (numeric 005) with
* the USERIP keyword.
*/
USERIP,
/**
* Syntax:
*
*
* USERS [<server>]
*
*
* Returns a list of users and information about those users in a format
* similar to the UNIX commands who, rusers and finger.[47]
*
* Defined in RFC 1459
*/
USERS,
/**
* Syntax:
*
*
* VERSION [<server>]
*
*
* Returns the version of <server>, or the current server if
* omitted.[48]
*
* Defined in RFC 1459
*/
VERSION,
/**
* Syntax:
*
*
* WALLOPS <message>
*
*
* Sends <message> to all operators connected to the server (RFC
* 1459), or all users with user mode 'w' set (RFC 2812).[49][50]
*
* Defined in RFC 1459
*/
WALLOPS,
/**
* Syntax:
*
*
* WATCH [+/-<nicknames>]
*
*
* Adds or removes a user to a client's server-side friends list. More than
* one nickname may be specified in a space-separated list, each item
* prefixed with a "+" or "-" to designate whether it is being added or
* removed. Sending the command with no parameters returns the entries in
* the client's friends list.
*
* This command is not formally defined in an RFC, but is supported by
* most[which?] major IRC daemons. Support is indicated in a RPL_ISUPPORT
* reply (numeric 005) with the WATCH keyword and the maximum number of
* entries a client may have in its friends list. For example:
*
* :irc.server.net 005 WiZ WALLCHOPS WATCH=128 SILENCE=15 MODES=12
* CHANTYPES=#
*/
WATCH,
/**
* Syntax:
*
*
* WHO [<name> ["o"]]
*
*
* Returns a list of users who match <name>.[51] If the flag "o" is
* given, the server will only return information about IRC Operators.
*
* Defined in RFC 1459
*/
WHO,
/**
* Syntax:
*
*
* WHOIS [<server>] <nicknames>
*
*
* Returns information about the comma-separated list of nicknames masks
* <nicknames>.[52] If <server> is given, the command is
* forwarded to it for processing.
*
* Defined in RFC 1459
*/
WHOIS,
/**
* Syntax:
*
*
* WHOWAS <nickname> [<count> [<server>]]
*
*
* Used to return information about a nickname that is no longer in use (due
* to client disconnection, or nickname changes).[53] If given, the server
* will return information from the last <count> times the nickname
* has been used. If <server> is given, the command is forwarded to it
* for processing. In RFC 2812, <nickname> can be a comma-separated
* list of nicknames.[54]
*
* Defined in RFC 1459
*/
WHOWAS;
private static final Map FAST_LOOKUP;
static {
Map fastLookUp = new HashMap(64);
IRCCommand[] directives = values();
for (IRCCommand directive : directives) {
fastLookUp.put(directive.name(), directive);
}
FAST_LOOKUP = Collections.unmodifiableMap(fastLookUp);
}
/**
* A {@link HashMap}-backed and {@code null}-tolerant alternative to
* {@link #valueOf(String)}. The lookup is case-sensitive.
*
* @param command
* the command as a {@link String}
* @return the {@link IRCCommand} that corresponds to the given string
* {@code command} or {@code null} if no such command exists
*/
public static IRCCommand fastValueOf(String command) {
return FAST_LOOKUP.get(command);
}
}