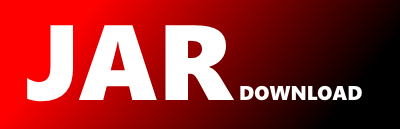
salvo.jesus.graph.AbstractPathImpl Maven / Gradle / Ivy
Show all versions of OpenJGraph Show documentation
package salvo.jesus.graph;
import salvo.jesus.graph.listener.*;
import java.util.*;
/**
* AbstractPathImpl is a partial implementation and common base
* for PathImpl, SimplePathImpl, and CyclePathImpl.
*
* @author John V. Sichi
* @version $Id: AbstractPathImpl.java,v 1.1 2002/03/12 07:48:34 perfecthash Exp $
*/
abstract class AbstractPathImpl extends GraphImpl implements Path
{
private AbstractPathListener listener;
protected void setListener(AbstractPathListener listener)
{
this.listener = listener;
}
/**
* @see Path#getFirstVertex
*/
public Vertex getFirstVertex() {
return listener.getFirstVertex();
}
/**
* @see Path#getLastVertex
*/
public Vertex getLastVertex() {
return listener.getLastVertex();
}
/**
* Adds a Vertex into the Path.
*
* This will call Graph.add( Vertex ) only if
* the Vertex is not yet part of the Graph.
* Note that this will also automatically add an Edge from the
* last Vertex that was added to the this Vertex
* being added.
*
* If adding this new Edge instance is not desired and you want
* to add an existing Edge instance instead ( i.e.: from
* an Edge in a Graph ), then you should call
* addEdge( Edge ) instead.
*
* @param newvertex Vertex to be added to the Path
* @throws IllegalPathException
*/
public void add( Vertex newVertex ) throws Exception {
if (getLastVertex() == null) {
super.add(newVertex);
} else {
addEdge(getLastVertex(),newVertex);
}
}
/**
* Removes the last Vertex that was added in the Path.
*/
public void remove() throws Exception {
Vertex v = listener.getLastVertex();
if (v == null) {
throw new NoSuchElementException();
}
remove(v);
}
/**
* Returns a String representation of the Path.
*/
public String toString() {
Iterator iterator = traverse(getFirstVertex()).iterator();
StringBuffer out = new StringBuffer();
String arrow = "->";
while( iterator.hasNext()) {
out.append( iterator.next().toString() );
if( iterator.hasNext()) {
out.append( arrow );
}
}
return out.toString();
}
}
// End AbstractPathImpl.java