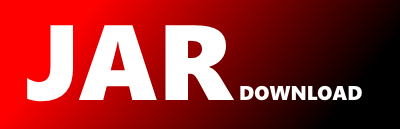
org.lsmp.djepExamples.MatrixSpeed Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jep Show documentation
Show all versions of jep Show documentation
JEP is a Java library for parsing and evaluating mathematical expressions.
The newest version!
/* @author rich
* Created on 26-Feb-2004
*/
package org.lsmp.djepExamples;
import org.nfunk.jep.*;
import org.lsmp.djep.matrixJep.*;
import org.lsmp.djep.vectorJep.*;
/**
* Compares the speed of matrix operations
* using both VectorJep or MatrixJep.
*/
public class MatrixSpeed {
static MatrixJep mj;
static VectorJep vj;
static int num_itts = 100000; // for normal use
// static int num_itts = 1000; // for use with profiler
public static void main(String args[]) {
long t1 = System.currentTimeMillis();
initVec();
long t2 = System.currentTimeMillis();
System.out.println("Vec initialise "+(t2-t1));
initMat();
long t3 = System.currentTimeMillis();
System.out.println("Mat initialise "+(t3-t2));
doBoth("y=[[1,2,3],[3,4,5],[6,7,8]]","y*y");
doBoth("y=[[1,2,3],[3,4,5],[6,7,8]]","y+y");
doBoth("y=[[1,2,3],[3,4,5],[6,7,8]]","y-y");
doBoth("y=[[1,2,3],[3,4,5],[6,7,8]]","y*y+y");
doBoth("y=[1,2,3]","y+y");
doBoth("y=[1,2,3]","y . y");
doBoth("y=[1,2,3]","y^^y");
}
public static void doBoth(String eqn1,String eqn2)
{
System.out.println("Testing speed for <"+eqn1+"> and <"+eqn2+">");
doVec(eqn1,eqn2);
doMat(eqn1,eqn2);
}
static void initVec()
{
vj = new VectorJep();
vj.addStandardConstants();
vj.addStandardFunctions();
vj.addComplex();
vj.setAllowUndeclared(true);
vj.setImplicitMul(true);
vj.setAllowAssignment(true);
}
static void doVec(String eqn1,String eqn2)
{
// System.out.println("vec init"+(t4-t3));
try
{
Node node1 = vj.parse(eqn1);
vj.evaluate(node1);
Node node = vj.parse(eqn2);
long t1 = System.currentTimeMillis();
// System.out.println("vec parse"+(t1-t4));
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy