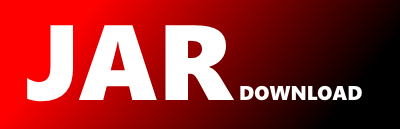
com.carrotsearch.junitbenchmarks.XMLConsumer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of junit-benchmarks Show documentation
Show all versions of junit-benchmarks Show documentation
A framework for writing performance micro-benchmarks using JUnit4 annotations, forked from https://github.com/carrotsearch/junit-benchmarks.
package com.carrotsearch.junitbenchmarks;
import java.io.*;
import java.text.NumberFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
/**
* {@link IResultsConsumer} that writes XML files for each benchmark.
*/
public final class XMLConsumer extends AutocloseConsumer implements Closeable
{
/**
* Timestamp format.
*/
public static final String TIMESTAMP_FORMAT = "yyyy-MM-dd'T'HH:mm:ss.SSSZ";
/**
* Output XML writer.
*/
private Writer writer;
/**
* Internal buffer for appends.
*/
private final StringBuilder b = new StringBuilder();
/**
* Number format.
*/
private final NumberFormat nf;
/**
* Instantiate from global options.
*/
public XMLConsumer() throws IOException
{
this(getDefaultOutputFile());
}
/*
*
*/
public XMLConsumer(File fileName) throws IOException
{
writer = new OutputStreamWriter(new FileOutputStream(fileName), "UTF-8");
addAutoclose(this);
writer.write("\n\n");
nf = NumberFormat.getInstance(Locale.ENGLISH);
nf.setMaximumFractionDigits(3);
nf.setGroupingUsed(false);
}
/**
* Accept a single benchmark result.
*/
public void accept(Result result) throws IOException
{
// We emit XML by hand. If anything more difficult comes up, we can switch
// to SimpleXML or some other XML binding solution.
b.setLength(0);
b.append("\t \n\n");
writer.write(b.toString());
writer.flush();
}
/**
* Close the output XML stream.
*/
public void close()
{
try
{
if (this.writer != null)
{
writer.write(" ");
writer.close();
writer = null;
removeAutoclose(this);
}
}
catch (IOException e)
{
// Ignore.
}
}
/**
* Returns the default output file.
*/
private static File getDefaultOutputFile()
{
final String xmlPath = System.getProperty(BenchmarkOptionsSystemProperties.XML_FILE_PROPERTY);
if (xmlPath != null && !xmlPath.trim().equals(""))
{
return new File(xmlPath);
}
throw new IllegalArgumentException("Missing global property: "
+ BenchmarkOptionsSystemProperties.XML_FILE_PROPERTY);
}
/**
* Unique timestamp for this XML consumer.
*/
private static String tstamp()
{
SimpleDateFormat sdf = new SimpleDateFormat(TIMESTAMP_FORMAT);
return sdf.format(new Date());
}
/**
* Append an attribute to XML.
*/
private void attribute(StringBuilder b, String attrName, String value)
{
b.append(' ');
b.append(attrName);
b.append("=\"");
b.append(Escape.xmlAttrEscape(value));
b.append('"');
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy