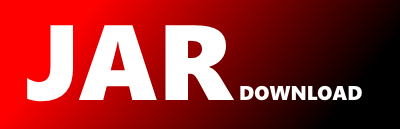
org.scijava.display.DisplayService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scijava-common Show documentation
Show all versions of scijava-common Show documentation
SciJava Common is a shared library for SciJava software. It provides a plugin framework, with an extensible mechanism for service discovery, backed by its own annotation processor, so that plugins can be loaded dynamically. It is used by both ImageJ and SCIFIO.
/*
* #%L
* SciJava Common shared library for SciJava software.
* %%
* Copyright (C) 2009 - 2016 Board of Regents of the University of
* Wisconsin-Madison, Broad Institute of MIT and Harvard, and Max Planck
* Institute of Molecular Cell Biology and Genetics.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
* #L%
*/
package org.scijava.display;
import java.util.List;
import org.scijava.display.event.DisplayCreatedEvent;
import org.scijava.display.event.DisplayDeletedEvent;
import org.scijava.event.EventService;
import org.scijava.object.ObjectService;
import org.scijava.plugin.PluginInfo;
import org.scijava.plugin.PluginService;
import org.scijava.service.SciJavaService;
/**
* Interface for service that tracks available {@link Display}s.
*
* @author Barry DeZonia
* @author Curtis Rueden
* @author Grant Harris
*/
public interface DisplayService extends SciJavaService {
EventService getEventService();
ObjectService getObjectService();
PluginService getPluginService();
/** Gets the currently active display (of any Display type). */
Display> getActiveDisplay();
/** Gets the most recently active display (of the specified Display type). */
> D getActiveDisplay(Class displayClass);
/**
* Set the active display.
*
* @param display
*/
void setActiveDisplay(Display> display);
/** Gets the list of known display plugins. */
List>> getDisplayPlugins();
/**
* Gets the display plugin of the given class, or null if none.
*/
> PluginInfo> getDisplayPlugin(
Class pluginClass);
/**
* Gets the display plugin of the given class name, or null if none.
*
* @throws ClassCastException if the plugin found is not a display plugin.
*/
PluginInfo> getDisplayPlugin(String className);
/**
* Gets the list of display plugins of the given type (e.g.,
* {@code ImageDisplay.class}).
*/
> List> getDisplayPluginsOfType(
Class type);
/** Gets a list of all available displays. */
List> getDisplays();
/**
* Gets a list of all available displays of the given type (e.g.,
* {@code ImageDisplay.class}).
*/
> List getDisplaysOfType(Class type);
/** Gets a display by its name. */
Display> getDisplay(String name);
/** Gets a list of displays currently visualizing the given object. */
List> getDisplays(Object o);
/**
* Checks whether the given name is already taken by an existing display.
*
* @param name The name to check.
* @return true if the name is available, false if already taken.
*/
boolean isUniqueName(String name);
/**
* Creates a display for the given object, publishing a
* {@link DisplayCreatedEvent} to notify interested parties. In particular:
*
* - Visible UIs will respond to this event by showing the display.
* - The {@link ObjectService} will add the new display to its index, until
* a corresponding {@link DisplayDeletedEvent} is later published.
*
*
* To create a {@link Display} without publishing an event, see
* {@link #createDisplayQuietly}.
*
*
* @param o The object for which a display should be created. The object is
* then added to the display.
* @return Newly created {@code Display>} containing the given object. The
* Display is typed with ? rather than T matching the Object because
* it is possible for the Display to be a collection of some other
* sort of object than the one being added. For example, ImageDisplay
* is a {@code Display} with the DataView wrapping a
* Dataset, yet the ImageDisplay supports adding Datasets directly,
* taking care of wrapping them in a DataView as needed.
*/
Display> createDisplay(Object o);
/**
* Creates a display for the given object, publishing a
* {@link DisplayCreatedEvent} to notify interested parties. In particular:
*
* - Visible UIs will respond to this event by showing the display.
* - The {@link ObjectService} will add the new display to its index, until
* a corresponding {@link DisplayDeletedEvent} is later published.
*
*
* To create a {@link Display} without publishing an event, see
* {@link #createDisplayQuietly}.
*
*
* @param name The name to be assigned to the display.
* @param o The object for which a display should be created. The object is
* then added to the display.
* @return Newly created {@code Display>} containing the given object. The
* Display is typed with ? rather than T matching the Object because
* it is possible for the Display to be a collection of some other
* sort of object than the one being added. For example, ImageDisplay
* is a {@code Display} with the DataView wrapping a
* Dataset, yet the ImageDisplay supports adding Datasets directly,
* taking care of wrapping them in a DataView as needed.
*/
Display> createDisplay(String name, Object o);
/**
* Creates a display for the given object, without publishing a
* {@link DisplayCreatedEvent}. Hence, the display will not be automatically
* shown or tracked.
*
* @param o The object for which a display should be created. The object is
* then added to the display.
* @return Newly created {@code Display>} containing the given object. The
* Display is typed with ? rather than T matching the Object because
* it is possible for the Display to be a collection of some other
* sort of object than the one being added. For example, ImageDisplay
* is a {@code Display} with the DataView wrapping a
* Dataset, yet the ImageDisplay supports adding Datasets directly,
* taking care of wrapping them in a DataView as needed.
*/
Display> createDisplayQuietly(Object o);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy