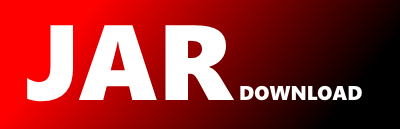
org.scijava.ops.engine.eval.OpEvaluator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scijava-ops-engine Show documentation
Show all versions of scijava-ops-engine Show documentation
Java implementation of the SciJava Ops matching engine.
The newest version!
/*
* #%L
* Java implementation of the SciJava Ops matching engine.
* %%
* Copyright (C) 2016 - 2024 SciJava developers.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
* #L%
*/
package org.scijava.ops.engine.eval;
import java.util.Arrays;
import java.util.Collections;
import java.util.Deque;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.scijava.function.Functions;
import org.scijava.ops.api.OpEnvironment;
import org.scijava.ops.engine.util.FunctionUtils;
import org.scijava.ops.spi.Op;
import org.scijava.parsington.Operator;
import org.scijava.parsington.Operators;
import org.scijava.parsington.Variable;
import org.scijava.parsington.eval.DefaultStackEvaluator;
import org.scijava.parsington.eval.Evaluator;
import org.scijava.types.Nil;
/**
* A Parsington {@link Evaluator} using available Ops.
*
* @author Curtis Rueden
*/
public class OpEvaluator extends DefaultStackEvaluator {
private final OpEnvironment ops;
/** Map of Parsington {@link Operator}s to Ops operation names. */
private final HashMap opMap;
public OpEvaluator(final OpEnvironment ops) {
this.ops = ops;
opMap = new HashMap<>();
// Map each standard Parsington operator to its associated op name.
// TODO: Consider creating a plugin extension point for defining these.
// -- dot --
// opMap.put(Operators.DOT, "dot");
// -- groups --
// opMap.put(Operators.PARENS, "parens");
// opMap.put(Operators.BRACKETS, "brackets");
// opMap.put(Operators.BRACES, "braces");
// -- transpose, power --
// opMap.put(Operators.TRANSPOSE, "transpose");
// opMap.put(Operators.DOT_TRANSPOSE, "dotTranspose");
opMap.put(Operators.POW, "math.pow");
// opMap.put(Operators.DOT_POW, "dotPow");
// -- unary --
opMap.put(Operators.POS, "identity");
opMap.put(Operators.NEG, "math.negate");
// opMap.put(Operators.COMPLEMENT, "complement");
// opMap.put(Operators.NOT, "not");
// -- multiplicative --
opMap.put(Operators.MUL, "math.multiply");
opMap.put(Operators.DIV, "math.divide");
opMap.put(Operators.MOD, "math.remainder");
// opMap.put(Operators.RIGHT_DIV, "rightDiv");
// opMap.put(Operators.DOT_DIV, "dotDiv");
// opMap.put(Operators.DOT_RIGHT_DIV, "dotRightDiv");
// -- additive --
opMap.put(Operators.ADD, "math.add");
opMap.put(Operators.SUB, "math.subtract");
// -- shift --
opMap.put(Operators.LEFT_SHIFT, "math.leftShift");
opMap.put(Operators.RIGHT_SHIFT, "math.rightShift");
opMap.put(Operators.UNSIGNED_RIGHT_SHIFT, "math.unsignedLeftShift");
// -- colon --
// opMap.put(Operators.COLON, "colon");
// -- relational --
opMap.put(Operators.LESS_THAN, "math.lessThan");
opMap.put(Operators.GREATER_THAN, "math.greaterThan");
opMap.put(Operators.LESS_THAN_OR_EQUAL, "math.lessThanOrEqual");
opMap.put(Operators.GREATER_THAN_OR_EQUAL, "math.greaterThanOrEqual");
// opMap.put(Operators.INSTANCEOF, "instanceof");
// -- equality --
opMap.put(Operators.EQUAL, "math.equal");
opMap.put(Operators.NOT_EQUAL, "math.notEqual");
// -- bitwise --
opMap.put(Operators.BITWISE_AND, "math.and");
opMap.put(Operators.BITWISE_OR, "math.or");
// -- logical --
opMap.put(Operators.LOGICAL_AND, "logic.and");
opMap.put(Operators.LOGICAL_OR, "logic.or");
}
// -- OpEvaluator methods --
/**
* Executes the given {@link Operator operation} with the specified argument
* list.
*/
public Object execute(final Operator op, final Object... args) {
return execute(getOpName(op), args);
}
/** Executes the given op with the specified argument list. */
public Object execute(final String opName, final Object... args) {
// Unwrap the arguments.
final Object[] argValues = new Object[args.length];
for (int i = 0; i < args.length; i++) {
argValues[i] = value(args[i]);
}
// generate Nils from types
Nil>[] inTypes = Arrays.stream(args).map((obj) -> type(value(obj)))
.toArray(Nil[]::new);
Nil
© 2015 - 2025 Weber Informatics LLC | Privacy Policy