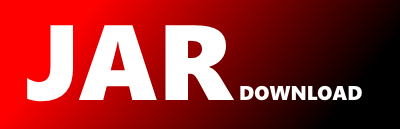
org.scijava.ops.engine.util.Infos Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scijava-ops-engine Show documentation
Show all versions of scijava-ops-engine Show documentation
Java implementation of the SciJava Ops matching engine.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy