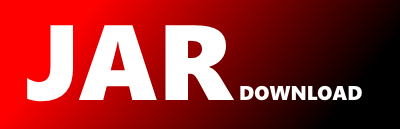
scommons.websql.encoding.TupleDecoders.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scommons-websql-encoding_sjs0.6_2.13 Show documentation
Show all versions of scommons-websql-encoding_sjs0.6_2.13 Show documentation
Encoders/Decoders for WebSQL/SQLite DB types
The newest version!
// DO NOT EDIT: generated by TupleEncodingSpec.scala
package scommons.websql.encoding
trait TupleDecoders extends BaseEncodingDsl {
implicit def tuple2Decoder[T1, T2](
implicit d1: Decoder[T1], d2: Decoder[T2]
): Decoder[(T1, T2)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r))
}
implicit def tuple3Decoder[T1, T2, T3](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3]
): Decoder[(T1, T2, T3)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r))
}
implicit def tuple4Decoder[T1, T2, T3, T4](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4]
): Decoder[(T1, T2, T3, T4)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r))
}
implicit def tuple5Decoder[T1, T2, T3, T4, T5](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5]
): Decoder[(T1, T2, T3, T4, T5)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r))
}
implicit def tuple6Decoder[T1, T2, T3, T4, T5, T6](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6]
): Decoder[(T1, T2, T3, T4, T5, T6)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r))
}
implicit def tuple7Decoder[T1, T2, T3, T4, T5, T6, T7](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7]
): Decoder[(T1, T2, T3, T4, T5, T6, T7)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r))
}
implicit def tuple8Decoder[T1, T2, T3, T4, T5, T6, T7, T8](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r))
}
implicit def tuple9Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r))
}
implicit def tuple10Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r))
}
implicit def tuple11Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r))
}
implicit def tuple12Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r))
}
implicit def tuple13Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r))
}
implicit def tuple14Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13], d14: Decoder[T14]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r),
d14(r.index, r))
}
implicit def tuple15Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13], d14: Decoder[T14], d15: Decoder[T15]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r),
d14(r.index, r),
d15(r.index, r))
}
implicit def tuple16Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13], d14: Decoder[T14], d15: Decoder[T15], d16: Decoder[T16]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r),
d14(r.index, r),
d15(r.index, r),
d16(r.index, r))
}
implicit def tuple17Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13], d14: Decoder[T14], d15: Decoder[T15], d16: Decoder[T16], d17: Decoder[T17]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r),
d14(r.index, r),
d15(r.index, r),
d16(r.index, r),
d17(r.index, r))
}
implicit def tuple18Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13], d14: Decoder[T14], d15: Decoder[T15], d16: Decoder[T16], d17: Decoder[T17], d18: Decoder[T18]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r),
d14(r.index, r),
d15(r.index, r),
d16(r.index, r),
d17(r.index, r),
d18(r.index, r))
}
implicit def tuple19Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13], d14: Decoder[T14], d15: Decoder[T15], d16: Decoder[T16], d17: Decoder[T17], d18: Decoder[T18], d19: Decoder[T19]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r),
d14(r.index, r),
d15(r.index, r),
d16(r.index, r),
d17(r.index, r),
d18(r.index, r),
d19(r.index, r))
}
implicit def tuple20Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13], d14: Decoder[T14], d15: Decoder[T15], d16: Decoder[T16], d17: Decoder[T17], d18: Decoder[T18], d19: Decoder[T19], d20: Decoder[T20]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r),
d14(r.index, r),
d15(r.index, r),
d16(r.index, r),
d17(r.index, r),
d18(r.index, r),
d19(r.index, r),
d20(r.index, r))
}
implicit def tuple21Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13], d14: Decoder[T14], d15: Decoder[T15], d16: Decoder[T16], d17: Decoder[T17], d18: Decoder[T18], d19: Decoder[T19], d20: Decoder[T20], d21: Decoder[T21]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r),
d14(r.index, r),
d15(r.index, r),
d16(r.index, r),
d17(r.index, r),
d18(r.index, r),
d19(r.index, r),
d20(r.index, r),
d21(r.index, r))
}
implicit def tuple22Decoder[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22](
implicit d1: Decoder[T1], d2: Decoder[T2], d3: Decoder[T3], d4: Decoder[T4], d5: Decoder[T5], d6: Decoder[T6], d7: Decoder[T7], d8: Decoder[T8], d9: Decoder[T9], d10: Decoder[T10], d11: Decoder[T11], d12: Decoder[T12], d13: Decoder[T13], d14: Decoder[T14], d15: Decoder[T15], d16: Decoder[T16], d17: Decoder[T17], d18: Decoder[T18], d19: Decoder[T19], d20: Decoder[T20], d21: Decoder[T21], d22: Decoder[T22]
): Decoder[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22)] =
WebSqlDecoder { (_: Index, r: ResultRow) =>
(d1(r.index, r),
d2(r.index, r),
d3(r.index, r),
d4(r.index, r),
d5(r.index, r),
d6(r.index, r),
d7(r.index, r),
d8(r.index, r),
d9(r.index, r),
d10(r.index, r),
d11(r.index, r),
d12(r.index, r),
d13(r.index, r),
d14(r.index, r),
d15(r.index, r),
d16(r.index, r),
d17(r.index, r),
d18(r.index, r),
d19(r.index, r),
d20(r.index, r),
d21(r.index, r),
d22(r.index, r))
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy