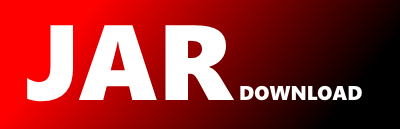
scray.service.qmodel.thriftjava.ScrayTColumnInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scray-client-jdbc Show documentation
Show all versions of scray-client-jdbc Show documentation
scray java code, which can be used from java and scala
/**
* generated by Scrooge ${project.version}
*/
package scray.service.qmodel.thriftjava;
import com.twitter.scrooge.Option;
import com.twitter.scrooge.Utilities;
import com.twitter.scrooge.ThriftStruct;
import com.twitter.scrooge.ThriftStructCodec;
import com.twitter.scrooge.ThriftStructCodec3;
import org.apache.thrift.protocol.*;
import java.nio.ByteBuffer;
import java.net.InetSocketAddress;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.HashMap;
import java.util.Set;
import java.util.HashSet;
/**
* Column identifier
*/
@javax.annotation.Generated(value = "com.twitter.scrooge.Compiler", date = "2017-04-25T16:52:50.412+0200")
public class ScrayTColumnInfo implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("ScrayTColumnInfo");
private static final TField NameField = new TField("name", TType.STRING, (short) 1);
final String name;
private static final TField ColumnTField = new TField("columnT", TType.STRING, (short) 2);
final Option columnT;
private static final TField TableIdField = new TField("tableId", TType.STRUCT, (short) 3);
final Option tableId;
public static class Builder {
private String _name = null;
private Boolean _got_name = false;
public Builder name(String value) {
this._name = value;
this._got_name = true;
return this;
}
public Builder unsetName() {
this._name = null;
this._got_name = false;
return this;
}
private String _columnT = null;
private Boolean _got_columnT = false;
public Builder columnT(String value) {
this._columnT = value;
this._got_columnT = true;
return this;
}
public Builder unsetColumnT() {
this._columnT = null;
this._got_columnT = false;
return this;
}
private ScrayTTableInfo _tableId = null;
private Boolean _got_tableId = false;
public Builder tableId(ScrayTTableInfo value) {
this._tableId = value;
this._got_tableId = true;
return this;
}
public Builder unsetTableId() {
this._tableId = null;
this._got_tableId = false;
return this;
}
public ScrayTColumnInfo build() {
return new ScrayTColumnInfo(
this._name,
Option.make(this._got_columnT, this._columnT),
Option.make(this._got_tableId, this._tableId) );
}
}
public Builder copy() {
Builder builder = new Builder();
builder.name(this.name);
if (this.columnT.isDefined()) builder.columnT(this.columnT.get());
if (this.tableId.isDefined()) builder.tableId(this.tableId.get());
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public ScrayTColumnInfo decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
String name = null;
String columnT = null;
ScrayTTableInfo tableId = null;
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case 1: /* name */
switch (_field.type) {
case TType.STRING:
String name_item;
name_item = _iprot.readString();
name = name_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.name(name);
break;
case 2: /* columnT */
switch (_field.type) {
case TType.STRING:
String columnT_item;
columnT_item = _iprot.readString();
columnT = columnT_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.columnT(columnT);
break;
case 3: /* tableId */
switch (_field.type) {
case TType.STRUCT:
ScrayTTableInfo tableId_item;
tableId_item = ScrayTTableInfo.decode(_iprot);
tableId = tableId_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.tableId(tableId);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(ScrayTColumnInfo struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static ScrayTColumnInfo decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(ScrayTColumnInfo struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public ScrayTColumnInfo(
String name,
Option columnT,
Option tableId
) {
this.name = name;
this.columnT = columnT;
this.tableId = tableId;
}
public ScrayTColumnInfo(
String name
) {
this.name = name;
this.columnT = Option.none();
this.tableId = Option.none();
}
public String getName() {
return this.name;
}
public boolean isSetName() {
return this.name != null;
}
public String getColumnT() {
return this.columnT.get();
}
public boolean isSetColumnT() {
return this.columnT.isDefined();
}
public ScrayTTableInfo getTableId() {
return this.tableId.get();
}
public boolean isSetTableId() {
return this.tableId.isDefined();
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
_oprot.writeFieldBegin(NameField);
String name_item = name;
_oprot.writeString(name_item);
_oprot.writeFieldEnd();
if (columnT.isDefined()) { _oprot.writeFieldBegin(ColumnTField);
String columnT_item = columnT.get();
_oprot.writeString(columnT_item);
_oprot.writeFieldEnd();
}
if (tableId.isDefined()) { _oprot.writeFieldBegin(TableIdField);
ScrayTTableInfo tableId_item = tableId.get();
tableId_item.write(_oprot);
_oprot.writeFieldEnd();
}
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof ScrayTColumnInfo)) return false;
ScrayTColumnInfo that = (ScrayTColumnInfo) other;
return
this.name.equals(that.name) &&
this.columnT.equals(that.columnT) &&
this.tableId.equals(that.tableId);
}
@Override
public String toString() {
return "ScrayTColumnInfo(" + this.name + "," + this.columnT + "," + this.tableId + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.name == null ? 0 : this.name.hashCode());
hash = hash * (this.columnT.isDefined() ? 0 : this.columnT.get().hashCode());
hash = hash * (this.tableId.isDefined() ? 0 : this.tableId.get().hashCode());
return hash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy