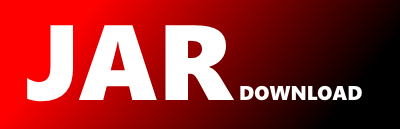
scray.service.qmodel.thriftjava.ScrayTQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scray-client-jdbc Show documentation
Show all versions of scray-client-jdbc Show documentation
scray java code, which can be used from java and scala
/**
* generated by Scrooge ${project.version}
*/
package scray.service.qmodel.thriftjava;
import com.twitter.scrooge.Option;
import com.twitter.scrooge.Utilities;
import com.twitter.scrooge.ThriftStruct;
import com.twitter.scrooge.ThriftStructCodec;
import com.twitter.scrooge.ThriftStructCodec3;
import org.apache.thrift.protocol.*;
import java.nio.ByteBuffer;
import java.net.InetSocketAddress;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.HashMap;
import java.util.Set;
import java.util.HashSet;
/**
* Main query type
*/
@javax.annotation.Generated(value = "com.twitter.scrooge.Compiler", date = "2017-04-25T16:52:50.412+0200")
public class ScrayTQuery implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("ScrayTQuery");
private static final TField QueryInfoField = new TField("queryInfo", TType.STRUCT, (short) 1);
final ScrayTQueryInfo queryInfo;
private static final TField ValuesField = new TField("values", TType.MAP, (short) 2);
final Map values;
private static final TField QueryExpressionField = new TField("queryExpression", TType.STRING, (short) 3);
final String queryExpression;
public static class Builder {
private ScrayTQueryInfo _queryInfo = null;
private Boolean _got_queryInfo = false;
public Builder queryInfo(ScrayTQueryInfo value) {
this._queryInfo = value;
this._got_queryInfo = true;
return this;
}
public Builder unsetQueryInfo() {
this._queryInfo = null;
this._got_queryInfo = false;
return this;
}
private Map _values = Utilities.makeMap();
private Boolean _got_values = false;
public Builder values(Map value) {
this._values = value;
this._got_values = true;
return this;
}
public Builder unsetValues() {
this._values = Utilities.makeMap();
this._got_values = false;
return this;
}
private String _queryExpression = null;
private Boolean _got_queryExpression = false;
public Builder queryExpression(String value) {
this._queryExpression = value;
this._got_queryExpression = true;
return this;
}
public Builder unsetQueryExpression() {
this._queryExpression = null;
this._got_queryExpression = false;
return this;
}
public ScrayTQuery build() {
return new ScrayTQuery(
this._queryInfo,
this._values,
this._queryExpression );
}
}
public Builder copy() {
Builder builder = new Builder();
builder.queryInfo(this.queryInfo);
builder.values(this.values);
builder.queryExpression(this.queryExpression);
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public ScrayTQuery decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
ScrayTQueryInfo queryInfo = null;
Map values = Utilities.makeMap();
String queryExpression = null;
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case 1: /* queryInfo */
switch (_field.type) {
case TType.STRUCT:
ScrayTQueryInfo queryInfo_item;
queryInfo_item = ScrayTQueryInfo.decode(_iprot);
queryInfo = queryInfo_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.queryInfo(queryInfo);
break;
case 2: /* values */
switch (_field.type) {
case TType.MAP:
Map values_item;
TMap _map_values_item = _iprot.readMapBegin();
values_item = new HashMap();
int _i_values_item = 0;
String values_item_key;
ByteBuffer values_item_value;
while (_i_values_item < _map_values_item.size) {
values_item_key = _iprot.readString();
values_item_value = _iprot.readBinary();
values_item.put(values_item_key, values_item_value);
_i_values_item += 1;
}
_iprot.readMapEnd();
values = values_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.values(values);
break;
case 3: /* queryExpression */
switch (_field.type) {
case TType.STRING:
String queryExpression_item;
queryExpression_item = _iprot.readString();
queryExpression = queryExpression_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.queryExpression(queryExpression);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(ScrayTQuery struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static ScrayTQuery decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(ScrayTQuery struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public ScrayTQuery(
ScrayTQueryInfo queryInfo,
Map values,
String queryExpression
) {
this.queryInfo = queryInfo;
this.values = values;
this.queryExpression = queryExpression;
}
public ScrayTQueryInfo getQueryInfo() {
return this.queryInfo;
}
public boolean isSetQueryInfo() {
return this.queryInfo != null;
}
public Map getValues() {
return this.values;
}
public boolean isSetValues() {
return this.values != null;
}
public String getQueryExpression() {
return this.queryExpression;
}
public boolean isSetQueryExpression() {
return this.queryExpression != null;
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
_oprot.writeFieldBegin(QueryInfoField);
ScrayTQueryInfo queryInfo_item = queryInfo;
queryInfo_item.write(_oprot);
_oprot.writeFieldEnd();
_oprot.writeFieldBegin(ValuesField);
Map values_item = values;
_oprot.writeMapBegin(new TMap(TType.STRING, TType.STRING, values_item.size()));
for (Map.Entry _values_item_entry : values_item.entrySet()) {
String values_item_key = _values_item_entry.getKey();
_oprot.writeString(values_item_key);
ByteBuffer values_item_value = _values_item_entry.getValue();
_oprot.writeBinary(values_item_value);
}
_oprot.writeMapEnd();
_oprot.writeFieldEnd();
_oprot.writeFieldBegin(QueryExpressionField);
String queryExpression_item = queryExpression;
_oprot.writeString(queryExpression_item);
_oprot.writeFieldEnd();
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof ScrayTQuery)) return false;
ScrayTQuery that = (ScrayTQuery) other;
return
this.queryInfo.equals(that.queryInfo) &&
this.values.equals(that.values) &&
this.queryExpression.equals(that.queryExpression);
}
@Override
public String toString() {
return "ScrayTQuery(" + this.queryInfo + "," + this.values + "," + this.queryExpression + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.queryInfo == null ? 0 : this.queryInfo.hashCode());
hash = hash * (this.values == null ? 0 : this.values.hashCode());
hash = hash * (this.queryExpression == null ? 0 : this.queryExpression.hashCode());
return hash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy