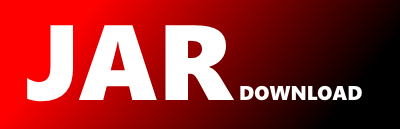
scray.service.qmodel.thriftjava.ScrayTQueryInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scray-client-jdbc Show documentation
Show all versions of scray-client-jdbc Show documentation
scray java code, which can be used from java and scala
/**
* generated by Scrooge ${project.version}
*/
package scray.service.qmodel.thriftjava;
import com.twitter.scrooge.Option;
import com.twitter.scrooge.Utilities;
import com.twitter.scrooge.ThriftStruct;
import com.twitter.scrooge.ThriftStructCodec;
import com.twitter.scrooge.ThriftStructCodec3;
import org.apache.thrift.protocol.*;
import java.nio.ByteBuffer;
import java.net.InetSocketAddress;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.HashMap;
import java.util.Set;
import java.util.HashSet;
/**
* Query identifier
*/
@javax.annotation.Generated(value = "com.twitter.scrooge.Compiler", date = "2017-04-25T16:52:50.412+0200")
public class ScrayTQueryInfo implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("ScrayTQueryInfo");
private static final TField QueryIdField = new TField("queryId", TType.STRUCT, (short) 1);
final Option queryId;
private static final TField QuerySpaceField = new TField("querySpace", TType.STRING, (short) 2);
final String querySpace;
private static final TField TableInfoField = new TField("tableInfo", TType.STRUCT, (short) 3);
final ScrayTTableInfo tableInfo;
private static final TField ColumnsField = new TField("columns", TType.LIST, (short) 4);
final List columns;
private static final TField PagesizeField = new TField("pagesize", TType.I32, (short) 5);
final Option pagesize;
private static final TField ExpiresField = new TField("expires", TType.I64, (short) 6);
final Option expires;
public static class Builder {
private ScrayUUID _queryId = null;
private Boolean _got_queryId = false;
public Builder queryId(ScrayUUID value) {
this._queryId = value;
this._got_queryId = true;
return this;
}
public Builder unsetQueryId() {
this._queryId = null;
this._got_queryId = false;
return this;
}
private String _querySpace = null;
private Boolean _got_querySpace = false;
public Builder querySpace(String value) {
this._querySpace = value;
this._got_querySpace = true;
return this;
}
public Builder unsetQuerySpace() {
this._querySpace = null;
this._got_querySpace = false;
return this;
}
private ScrayTTableInfo _tableInfo = null;
private Boolean _got_tableInfo = false;
public Builder tableInfo(ScrayTTableInfo value) {
this._tableInfo = value;
this._got_tableInfo = true;
return this;
}
public Builder unsetTableInfo() {
this._tableInfo = null;
this._got_tableInfo = false;
return this;
}
private List _columns = Utilities.makeList();
private Boolean _got_columns = false;
public Builder columns(List value) {
this._columns = value;
this._got_columns = true;
return this;
}
public Builder unsetColumns() {
this._columns = Utilities.makeList();
this._got_columns = false;
return this;
}
private int _pagesize = 0;
private Boolean _got_pagesize = false;
public Builder pagesize(int value) {
this._pagesize = value;
this._got_pagesize = true;
return this;
}
public Builder unsetPagesize() {
this._pagesize = 0;
this._got_pagesize = false;
return this;
}
private long _expires = 0L;
private Boolean _got_expires = false;
public Builder expires(long value) {
this._expires = value;
this._got_expires = true;
return this;
}
public Builder unsetExpires() {
this._expires = 0L;
this._got_expires = false;
return this;
}
public ScrayTQueryInfo build() {
return new ScrayTQueryInfo(
Option.make(this._got_queryId, this._queryId),
this._querySpace,
this._tableInfo,
this._columns,
Option.make(this._got_pagesize, this._pagesize),
Option.make(this._got_expires, this._expires) );
}
}
public Builder copy() {
Builder builder = new Builder();
if (this.queryId.isDefined()) builder.queryId(this.queryId.get());
builder.querySpace(this.querySpace);
builder.tableInfo(this.tableInfo);
builder.columns(this.columns);
if (this.pagesize.isDefined()) builder.pagesize(this.pagesize.get());
if (this.expires.isDefined()) builder.expires(this.expires.get());
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public ScrayTQueryInfo decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
ScrayUUID queryId = null;
String querySpace = null;
ScrayTTableInfo tableInfo = null;
List columns = Utilities.makeList();
int pagesize = 0;
long expires = 0L;
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case 1: /* queryId */
switch (_field.type) {
case TType.STRUCT:
ScrayUUID queryId_item;
queryId_item = ScrayUUID.decode(_iprot);
queryId = queryId_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.queryId(queryId);
break;
case 2: /* querySpace */
switch (_field.type) {
case TType.STRING:
String querySpace_item;
querySpace_item = _iprot.readString();
querySpace = querySpace_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.querySpace(querySpace);
break;
case 3: /* tableInfo */
switch (_field.type) {
case TType.STRUCT:
ScrayTTableInfo tableInfo_item;
tableInfo_item = ScrayTTableInfo.decode(_iprot);
tableInfo = tableInfo_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.tableInfo(tableInfo);
break;
case 4: /* columns */
switch (_field.type) {
case TType.LIST:
List columns_item;
TList _list_columns_item = _iprot.readListBegin();
columns_item = new ArrayList();
int _i_columns_item = 0;
ScrayTColumnInfo columns_item_element;
while (_i_columns_item < _list_columns_item.size) {
columns_item_element = ScrayTColumnInfo.decode(_iprot);
columns_item.add(columns_item_element);
_i_columns_item += 1;
}
_iprot.readListEnd();
columns = columns_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.columns(columns);
break;
case 5: /* pagesize */
switch (_field.type) {
case TType.I32:
Integer pagesize_item;
pagesize_item = _iprot.readI32();
pagesize = pagesize_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.pagesize(pagesize);
break;
case 6: /* expires */
switch (_field.type) {
case TType.I64:
Long expires_item;
expires_item = _iprot.readI64();
expires = expires_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.expires(expires);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(ScrayTQueryInfo struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static ScrayTQueryInfo decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(ScrayTQueryInfo struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public ScrayTQueryInfo(
Option queryId,
String querySpace,
ScrayTTableInfo tableInfo,
List columns,
Option pagesize,
Option expires
) {
this.queryId = queryId;
this.querySpace = querySpace;
this.tableInfo = tableInfo;
this.columns = columns;
this.pagesize = pagesize;
this.expires = expires;
}
public ScrayTQueryInfo(
String querySpace,
ScrayTTableInfo tableInfo,
List columns
) {
this.queryId = Option.none();
this.querySpace = querySpace;
this.tableInfo = tableInfo;
this.columns = columns;
this.pagesize = Option.none();
this.expires = Option.none();
}
public ScrayUUID getQueryId() {
return this.queryId.get();
}
public boolean isSetQueryId() {
return this.queryId.isDefined();
}
public String getQuerySpace() {
return this.querySpace;
}
public boolean isSetQuerySpace() {
return this.querySpace != null;
}
public ScrayTTableInfo getTableInfo() {
return this.tableInfo;
}
public boolean isSetTableInfo() {
return this.tableInfo != null;
}
public List getColumns() {
return this.columns;
}
public boolean isSetColumns() {
return this.columns != null;
}
public int getPagesize() {
return this.pagesize.get();
}
public boolean isSetPagesize() {
return this.pagesize.isDefined();
}
public long getExpires() {
return this.expires.get();
}
public boolean isSetExpires() {
return this.expires.isDefined();
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
if (queryId.isDefined()) { _oprot.writeFieldBegin(QueryIdField);
ScrayUUID queryId_item = queryId.get();
queryId_item.write(_oprot);
_oprot.writeFieldEnd();
}
_oprot.writeFieldBegin(QuerySpaceField);
String querySpace_item = querySpace;
_oprot.writeString(querySpace_item);
_oprot.writeFieldEnd();
_oprot.writeFieldBegin(TableInfoField);
ScrayTTableInfo tableInfo_item = tableInfo;
tableInfo_item.write(_oprot);
_oprot.writeFieldEnd();
_oprot.writeFieldBegin(ColumnsField);
List columns_item = columns;
_oprot.writeListBegin(new TList(TType.STRUCT, columns_item.size()));
for (ScrayTColumnInfo columns_item_element : columns_item) {
columns_item_element.write(_oprot);
}
_oprot.writeListEnd();
_oprot.writeFieldEnd();
if (pagesize.isDefined()) { _oprot.writeFieldBegin(PagesizeField);
Integer pagesize_item = pagesize.get();
_oprot.writeI32(pagesize_item);
_oprot.writeFieldEnd();
}
if (expires.isDefined()) { _oprot.writeFieldBegin(ExpiresField);
Long expires_item = expires.get();
_oprot.writeI64(expires_item);
_oprot.writeFieldEnd();
}
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof ScrayTQueryInfo)) return false;
ScrayTQueryInfo that = (ScrayTQueryInfo) other;
return
this.queryId.equals(that.queryId) &&
this.querySpace.equals(that.querySpace) &&
this.tableInfo.equals(that.tableInfo) &&
this.columns.equals(that.columns) &&
this.pagesize.equals(that.pagesize)
&&
this.expires.equals(that.expires)
;
}
@Override
public String toString() {
return "ScrayTQueryInfo(" + this.queryId + "," + this.querySpace + "," + this.tableInfo + "," + this.columns + "," + this.pagesize + "," + this.expires + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.queryId.isDefined() ? 0 : this.queryId.get().hashCode());
hash = hash * (this.querySpace == null ? 0 : this.querySpace.hashCode());
hash = hash * (this.tableInfo == null ? 0 : this.tableInfo.hashCode());
hash = hash * (this.columns == null ? 0 : this.columns.hashCode());
hash = hash * (this.pagesize.isDefined() ? 0 : new Integer(this.pagesize.get()).hashCode());
hash = hash * (this.expires.isDefined() ? 0 : new Long(this.expires.get()).hashCode());
return hash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy