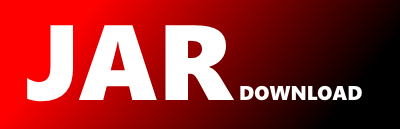
scray.service.qmodel.thriftjava.ScrayTTableInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scray-client-jdbc Show documentation
Show all versions of scray-client-jdbc Show documentation
scray java code, which can be used from java and scala
/**
* generated by Scrooge ${project.version}
*/
package scray.service.qmodel.thriftjava;
import com.twitter.scrooge.Option;
import com.twitter.scrooge.Utilities;
import com.twitter.scrooge.ThriftStruct;
import com.twitter.scrooge.ThriftStructCodec;
import com.twitter.scrooge.ThriftStructCodec3;
import org.apache.thrift.protocol.*;
import java.nio.ByteBuffer;
import java.net.InetSocketAddress;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.HashMap;
import java.util.Set;
import java.util.HashSet;
/**
* Table identifier
*/
@javax.annotation.Generated(value = "com.twitter.scrooge.Compiler", date = "2017-04-25T16:52:50.412+0200")
public class ScrayTTableInfo implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("ScrayTTableInfo");
private static final TField DbSystemField = new TField("dbSystem", TType.STRING, (short) 1);
final String dbSystem;
private static final TField DbIdField = new TField("dbId", TType.STRING, (short) 2);
final String dbId;
private static final TField TableIdField = new TField("tableId", TType.STRING, (short) 3);
final String tableId;
private static final TField KeyTField = new TField("keyT", TType.STRING, (short) 4);
final Option keyT;
public static class Builder {
private String _dbSystem = null;
private Boolean _got_dbSystem = false;
public Builder dbSystem(String value) {
this._dbSystem = value;
this._got_dbSystem = true;
return this;
}
public Builder unsetDbSystem() {
this._dbSystem = null;
this._got_dbSystem = false;
return this;
}
private String _dbId = null;
private Boolean _got_dbId = false;
public Builder dbId(String value) {
this._dbId = value;
this._got_dbId = true;
return this;
}
public Builder unsetDbId() {
this._dbId = null;
this._got_dbId = false;
return this;
}
private String _tableId = null;
private Boolean _got_tableId = false;
public Builder tableId(String value) {
this._tableId = value;
this._got_tableId = true;
return this;
}
public Builder unsetTableId() {
this._tableId = null;
this._got_tableId = false;
return this;
}
private String _keyT = null;
private Boolean _got_keyT = false;
public Builder keyT(String value) {
this._keyT = value;
this._got_keyT = true;
return this;
}
public Builder unsetKeyT() {
this._keyT = null;
this._got_keyT = false;
return this;
}
public ScrayTTableInfo build() {
return new ScrayTTableInfo(
this._dbSystem,
this._dbId,
this._tableId,
Option.make(this._got_keyT, this._keyT) );
}
}
public Builder copy() {
Builder builder = new Builder();
builder.dbSystem(this.dbSystem);
builder.dbId(this.dbId);
builder.tableId(this.tableId);
if (this.keyT.isDefined()) builder.keyT(this.keyT.get());
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public ScrayTTableInfo decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
String dbSystem = null;
String dbId = null;
String tableId = null;
String keyT = null;
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case 1: /* dbSystem */
switch (_field.type) {
case TType.STRING:
String dbSystem_item;
dbSystem_item = _iprot.readString();
dbSystem = dbSystem_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.dbSystem(dbSystem);
break;
case 2: /* dbId */
switch (_field.type) {
case TType.STRING:
String dbId_item;
dbId_item = _iprot.readString();
dbId = dbId_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.dbId(dbId);
break;
case 3: /* tableId */
switch (_field.type) {
case TType.STRING:
String tableId_item;
tableId_item = _iprot.readString();
tableId = tableId_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.tableId(tableId);
break;
case 4: /* keyT */
switch (_field.type) {
case TType.STRING:
String keyT_item;
keyT_item = _iprot.readString();
keyT = keyT_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.keyT(keyT);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(ScrayTTableInfo struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static ScrayTTableInfo decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(ScrayTTableInfo struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public ScrayTTableInfo(
String dbSystem,
String dbId,
String tableId,
Option keyT
) {
this.dbSystem = dbSystem;
this.dbId = dbId;
this.tableId = tableId;
this.keyT = keyT;
}
public ScrayTTableInfo(
String dbSystem,
String dbId,
String tableId
) {
this.dbSystem = dbSystem;
this.dbId = dbId;
this.tableId = tableId;
this.keyT = Option.none();
}
public String getDbSystem() {
return this.dbSystem;
}
public boolean isSetDbSystem() {
return this.dbSystem != null;
}
public String getDbId() {
return this.dbId;
}
public boolean isSetDbId() {
return this.dbId != null;
}
public String getTableId() {
return this.tableId;
}
public boolean isSetTableId() {
return this.tableId != null;
}
public String getKeyT() {
return this.keyT.get();
}
public boolean isSetKeyT() {
return this.keyT.isDefined();
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
_oprot.writeFieldBegin(DbSystemField);
String dbSystem_item = dbSystem;
_oprot.writeString(dbSystem_item);
_oprot.writeFieldEnd();
_oprot.writeFieldBegin(DbIdField);
String dbId_item = dbId;
_oprot.writeString(dbId_item);
_oprot.writeFieldEnd();
_oprot.writeFieldBegin(TableIdField);
String tableId_item = tableId;
_oprot.writeString(tableId_item);
_oprot.writeFieldEnd();
if (keyT.isDefined()) { _oprot.writeFieldBegin(KeyTField);
String keyT_item = keyT.get();
_oprot.writeString(keyT_item);
_oprot.writeFieldEnd();
}
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof ScrayTTableInfo)) return false;
ScrayTTableInfo that = (ScrayTTableInfo) other;
return
this.dbSystem.equals(that.dbSystem) &&
this.dbId.equals(that.dbId) &&
this.tableId.equals(that.tableId) &&
this.keyT.equals(that.keyT);
}
@Override
public String toString() {
return "ScrayTTableInfo(" + this.dbSystem + "," + this.dbId + "," + this.tableId + "," + this.keyT + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.dbSystem == null ? 0 : this.dbSystem.hashCode());
hash = hash * (this.dbId == null ? 0 : this.dbId.hashCode());
hash = hash * (this.tableId == null ? 0 : this.tableId.hashCode());
hash = hash * (this.keyT.isDefined() ? 0 : this.keyT.get().hashCode());
return hash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy