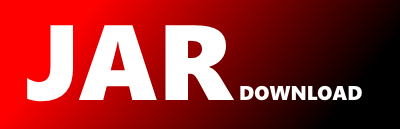
scray.service.qservice.thriftjava.ScrayMetaTService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scray-client-jdbc Show documentation
Show all versions of scray-client-jdbc Show documentation
scray java code, which can be used from java and scala
/**
* generated by Scrooge ${project.version}
*/
package scray.service.qservice.thriftjava;
import com.twitter.scrooge.Option;
import com.twitter.scrooge.ThriftStruct;
import com.twitter.scrooge.ThriftStructCodec;
import com.twitter.scrooge.ThriftStructCodec3;
import com.twitter.scrooge.Utilities;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.HashMap;
import java.util.Set;
import java.util.HashSet;
import org.apache.thrift.protocol.*;
import org.apache.thrift.TApplicationException;
import com.twitter.util.Future;
import com.twitter.util.FutureEventListener;
import com.twitter.finagle.SourcedException;
import com.twitter.finagle.stats.NullStatsReceiver;
import com.twitter.finagle.stats.StatsReceiver;
import com.twitter.finagle.thrift.ThriftClientRequest;
import java.util.Arrays;
import org.apache.thrift.TException;
import com.twitter.finagle.Service;
import com.twitter.finagle.stats.Counter;
import com.twitter.util.Function;
import com.twitter.util.Function2;
import org.apache.thrift.transport.TMemoryBuffer;
import org.apache.thrift.transport.TMemoryInputTransport;
import org.apache.thrift.transport.TTransport;
/**
* Scray meta service
* The service is provided by scray seed nodes
*/
@javax.annotation.Generated(value = "com.twitter.scrooge.Compiler", date = "2017-04-25T16:52:50.412+0200")
public class ScrayMetaTService {
public interface Iface {
/**
* Fetch a list of service endpoints.
* Each endpoint provides ScrayStatelessTService and ScrayStatefulTService alternatives.
* Queries can address different endpoints for load distribution.
*/
public List getServiceEndpoints();
/**
* Add new service endpoint.
* The endpoint will be removed after a default expiration period.
*/
public ScrayTServiceEndpoint addServiceEndpoint(ScrayTServiceEndpoint endpoint);
/**
* Restore the default expiration period of an endpoint.
*/
public Void refreshServiceEndpoint(scray.service.qmodel.thriftjava.ScrayUUID endpointID);
/**
* Return vital sign
*/
public Boolean ping();
/**
* Shutdown the server
*/
public Void shutdown(com.twitter.scrooge.Option waitNanos);
}
public interface FutureIface {
/**
* Fetch a list of service endpoints.
* Each endpoint provides ScrayStatelessTService and ScrayStatefulTService alternatives.
* Queries can address different endpoints for load distribution.
*/
public Future> getServiceEndpoints();
/**
* Add new service endpoint.
* The endpoint will be removed after a default expiration period.
*/
public Future addServiceEndpoint(ScrayTServiceEndpoint endpoint);
/**
* Restore the default expiration period of an endpoint.
*/
public Future refreshServiceEndpoint(scray.service.qmodel.thriftjava.ScrayUUID endpointID);
/**
* Return vital sign
*/
public Future ping();
/**
* Shutdown the server
*/
public Future shutdown(com.twitter.scrooge.Option waitNanos);
}
static class getServiceEndpoints$args implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("getServiceEndpoints_args");
public static class Builder {
public getServiceEndpoints$args build() {
return new getServiceEndpoints$args(
);
}
}
public Builder copy() {
Builder builder = new Builder();
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public getServiceEndpoints$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(getServiceEndpoints$args struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static getServiceEndpoints$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(getServiceEndpoints$args struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public getServiceEndpoints$args(
) {
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
return this == other;
}
@Override
public String toString() {
return "getServiceEndpoints$args()";
}
@Override
public int hashCode() {
return super.hashCode();
}
}
static class getServiceEndpoints$result implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("getServiceEndpoints_result");
private static final TField SuccessField = new TField("success", TType.LIST, (short) 0);
final Option> success;
public static class Builder {
private List _success = Utilities.makeList();
private Boolean _got_success = false;
public Builder success(List value) {
this._success = value;
this._got_success = true;
return this;
}
public Builder unsetSuccess() {
this._success = Utilities.makeList();
this._got_success = false;
return this;
}
public getServiceEndpoints$result build() {
return new getServiceEndpoints$result(
Option.make(this._got_success, this._success) );
}
}
public Builder copy() {
Builder builder = new Builder();
if (this.success.isDefined()) builder.success(this.success.get());
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public getServiceEndpoints$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
List success = Utilities.makeList();
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case 0: /* success */
switch (_field.type) {
case TType.LIST:
List success_item;
TList _list_success_item = _iprot.readListBegin();
success_item = new ArrayList();
int _i_success_item = 0;
ScrayTServiceEndpoint success_item_element;
while (_i_success_item < _list_success_item.size) {
success_item_element = ScrayTServiceEndpoint.decode(_iprot);
success_item.add(success_item_element);
_i_success_item += 1;
}
_iprot.readListEnd();
success = success_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.success(success);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(getServiceEndpoints$result struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static getServiceEndpoints$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(getServiceEndpoints$result struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public getServiceEndpoints$result(
Option> success
) {
this.success = success;
}
public List getSuccess() {
return this.success.get();
}
public boolean isSetSuccess() {
return this.success.isDefined();
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
if (success.isDefined()) { _oprot.writeFieldBegin(SuccessField);
List success_item = success.get();
_oprot.writeListBegin(new TList(TType.STRUCT, success_item.size()));
for (ScrayTServiceEndpoint success_item_element : success_item) {
success_item_element.write(_oprot);
}
_oprot.writeListEnd();
_oprot.writeFieldEnd();
}
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof getServiceEndpoints$result)) return false;
getServiceEndpoints$result that = (getServiceEndpoints$result) other;
return
this.success.equals(that.success);
}
@Override
public String toString() {
return "getServiceEndpoints$result(" + this.success + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.success.isDefined() ? 0 : this.success.get().hashCode());
return hash;
}
}
static class addServiceEndpoint$args implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("addServiceEndpoint_args");
private static final TField EndpointField = new TField("endpoint", TType.STRUCT, (short) -1);
final ScrayTServiceEndpoint endpoint;
public static class Builder {
private ScrayTServiceEndpoint _endpoint = null;
private Boolean _got_endpoint = false;
public Builder endpoint(ScrayTServiceEndpoint value) {
this._endpoint = value;
this._got_endpoint = true;
return this;
}
public Builder unsetEndpoint() {
this._endpoint = null;
this._got_endpoint = false;
return this;
}
public addServiceEndpoint$args build() {
return new addServiceEndpoint$args(
this._endpoint );
}
}
public Builder copy() {
Builder builder = new Builder();
builder.endpoint(this.endpoint);
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public addServiceEndpoint$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
ScrayTServiceEndpoint endpoint = null;
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case -1: /* endpoint */
switch (_field.type) {
case TType.STRUCT:
ScrayTServiceEndpoint endpoint_item;
endpoint_item = ScrayTServiceEndpoint.decode(_iprot);
endpoint = endpoint_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.endpoint(endpoint);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(addServiceEndpoint$args struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static addServiceEndpoint$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(addServiceEndpoint$args struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public addServiceEndpoint$args(
ScrayTServiceEndpoint endpoint
) {
this.endpoint = endpoint;
}
public ScrayTServiceEndpoint getEndpoint() {
return this.endpoint;
}
public boolean isSetEndpoint() {
return this.endpoint != null;
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
_oprot.writeFieldBegin(EndpointField);
ScrayTServiceEndpoint endpoint_item = endpoint;
endpoint_item.write(_oprot);
_oprot.writeFieldEnd();
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof addServiceEndpoint$args)) return false;
addServiceEndpoint$args that = (addServiceEndpoint$args) other;
return
this.endpoint.equals(that.endpoint);
}
@Override
public String toString() {
return "addServiceEndpoint$args(" + this.endpoint + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.endpoint == null ? 0 : this.endpoint.hashCode());
return hash;
}
}
static class addServiceEndpoint$result implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("addServiceEndpoint_result");
private static final TField SuccessField = new TField("success", TType.STRUCT, (short) 0);
final Option success;
public static class Builder {
private ScrayTServiceEndpoint _success = null;
private Boolean _got_success = false;
public Builder success(ScrayTServiceEndpoint value) {
this._success = value;
this._got_success = true;
return this;
}
public Builder unsetSuccess() {
this._success = null;
this._got_success = false;
return this;
}
public addServiceEndpoint$result build() {
return new addServiceEndpoint$result(
Option.make(this._got_success, this._success) );
}
}
public Builder copy() {
Builder builder = new Builder();
if (this.success.isDefined()) builder.success(this.success.get());
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public addServiceEndpoint$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
ScrayTServiceEndpoint success = null;
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case 0: /* success */
switch (_field.type) {
case TType.STRUCT:
ScrayTServiceEndpoint success_item;
success_item = ScrayTServiceEndpoint.decode(_iprot);
success = success_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.success(success);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(addServiceEndpoint$result struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static addServiceEndpoint$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(addServiceEndpoint$result struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public addServiceEndpoint$result(
Option success
) {
this.success = success;
}
public ScrayTServiceEndpoint getSuccess() {
return this.success.get();
}
public boolean isSetSuccess() {
return this.success.isDefined();
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
if (success.isDefined()) { _oprot.writeFieldBegin(SuccessField);
ScrayTServiceEndpoint success_item = success.get();
success_item.write(_oprot);
_oprot.writeFieldEnd();
}
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof addServiceEndpoint$result)) return false;
addServiceEndpoint$result that = (addServiceEndpoint$result) other;
return
this.success.equals(that.success);
}
@Override
public String toString() {
return "addServiceEndpoint$result(" + this.success + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.success.isDefined() ? 0 : this.success.get().hashCode());
return hash;
}
}
static class refreshServiceEndpoint$args implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("refreshServiceEndpoint_args");
private static final TField EndpointIDField = new TField("endpointID", TType.STRUCT, (short) -1);
final scray.service.qmodel.thriftjava.ScrayUUID endpointID;
public static class Builder {
private scray.service.qmodel.thriftjava.ScrayUUID _endpointID = null;
private Boolean _got_endpointID = false;
public Builder endpointID(scray.service.qmodel.thriftjava.ScrayUUID value) {
this._endpointID = value;
this._got_endpointID = true;
return this;
}
public Builder unsetEndpointID() {
this._endpointID = null;
this._got_endpointID = false;
return this;
}
public refreshServiceEndpoint$args build() {
return new refreshServiceEndpoint$args(
this._endpointID );
}
}
public Builder copy() {
Builder builder = new Builder();
builder.endpointID(this.endpointID);
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public refreshServiceEndpoint$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
scray.service.qmodel.thriftjava.ScrayUUID endpointID = null;
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case -1: /* endpointID */
switch (_field.type) {
case TType.STRUCT:
scray.service.qmodel.thriftjava.ScrayUUID endpointID_item;
endpointID_item = scray.service.qmodel.thriftjava.ScrayUUID.decode(_iprot);
endpointID = endpointID_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.endpointID(endpointID);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(refreshServiceEndpoint$args struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static refreshServiceEndpoint$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(refreshServiceEndpoint$args struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public refreshServiceEndpoint$args(
scray.service.qmodel.thriftjava.ScrayUUID endpointID
) {
this.endpointID = endpointID;
}
public scray.service.qmodel.thriftjava.ScrayUUID getEndpointID() {
return this.endpointID;
}
public boolean isSetEndpointID() {
return this.endpointID != null;
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
_oprot.writeFieldBegin(EndpointIDField);
scray.service.qmodel.thriftjava.ScrayUUID endpointID_item = endpointID;
endpointID_item.write(_oprot);
_oprot.writeFieldEnd();
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof refreshServiceEndpoint$args)) return false;
refreshServiceEndpoint$args that = (refreshServiceEndpoint$args) other;
return
this.endpointID.equals(that.endpointID);
}
@Override
public String toString() {
return "refreshServiceEndpoint$args(" + this.endpointID + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.endpointID == null ? 0 : this.endpointID.hashCode());
return hash;
}
}
static class refreshServiceEndpoint$result implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("refreshServiceEndpoint_result");
public static class Builder {
public refreshServiceEndpoint$result build() {
return new refreshServiceEndpoint$result(
);
}
}
public Builder copy() {
Builder builder = new Builder();
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public refreshServiceEndpoint$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(refreshServiceEndpoint$result struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static refreshServiceEndpoint$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(refreshServiceEndpoint$result struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public refreshServiceEndpoint$result(
) {
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
return this == other;
}
@Override
public String toString() {
return "refreshServiceEndpoint$result()";
}
@Override
public int hashCode() {
return super.hashCode();
}
}
static class ping$args implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("ping_args");
public static class Builder {
public ping$args build() {
return new ping$args(
);
}
}
public Builder copy() {
Builder builder = new Builder();
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public ping$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(ping$args struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static ping$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(ping$args struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public ping$args(
) {
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
return this == other;
}
@Override
public String toString() {
return "ping$args()";
}
@Override
public int hashCode() {
return super.hashCode();
}
}
static class ping$result implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("ping_result");
private static final TField SuccessField = new TField("success", TType.BOOL, (short) 0);
final Option success;
public static class Builder {
private boolean _success = false;
private Boolean _got_success = false;
public Builder success(boolean value) {
this._success = value;
this._got_success = true;
return this;
}
public Builder unsetSuccess() {
this._success = false;
this._got_success = false;
return this;
}
public ping$result build() {
return new ping$result(
Option.make(this._got_success, this._success) );
}
}
public Builder copy() {
Builder builder = new Builder();
if (this.success.isDefined()) builder.success(this.success.get());
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public ping$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
boolean success = false;
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case 0: /* success */
switch (_field.type) {
case TType.BOOL:
Boolean success_item;
success_item = _iprot.readBool();
success = success_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.success(success);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(ping$result struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static ping$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(ping$result struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public ping$result(
Option success
) {
this.success = success;
}
public boolean getSuccess() {
return this.success.get();
}
public boolean isSetSuccess() {
return this.success.isDefined();
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
if (success.isDefined()) { _oprot.writeFieldBegin(SuccessField);
Boolean success_item = success.get();
_oprot.writeBool(success_item);
_oprot.writeFieldEnd();
}
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof ping$result)) return false;
ping$result that = (ping$result) other;
return
this.success.equals(that.success)
;
}
@Override
public String toString() {
return "ping$result(" + this.success + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.success.isDefined() ? 0 : new Boolean(this.success.get()).hashCode());
return hash;
}
}
static class shutdown$args implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("shutdown_args");
private static final TField WaitNanosField = new TField("waitNanos", TType.I64, (short) -1);
final Option waitNanos;
public static class Builder {
private long _waitNanos = 0L;
private Boolean _got_waitNanos = false;
public Builder waitNanos(long value) {
this._waitNanos = value;
this._got_waitNanos = true;
return this;
}
public Builder unsetWaitNanos() {
this._waitNanos = 0L;
this._got_waitNanos = false;
return this;
}
public shutdown$args build() {
return new shutdown$args(
Option.make(this._got_waitNanos, this._waitNanos) );
}
}
public Builder copy() {
Builder builder = new Builder();
if (this.waitNanos.isDefined()) builder.waitNanos(this.waitNanos.get());
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public shutdown$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
long waitNanos = 0L;
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
case -1: /* waitNanos */
switch (_field.type) {
case TType.I64:
Long waitNanos_item;
waitNanos_item = _iprot.readI64();
waitNanos = waitNanos_item;
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
builder.waitNanos(waitNanos);
break;
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(shutdown$args struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static shutdown$args decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(shutdown$args struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public shutdown$args(
Option waitNanos
) {
this.waitNanos = waitNanos;
}
public long getWaitNanos() {
return this.waitNanos.get();
}
public boolean isSetWaitNanos() {
return this.waitNanos.isDefined();
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
if (waitNanos.isDefined()) { _oprot.writeFieldBegin(WaitNanosField);
Long waitNanos_item = waitNanos.get();
_oprot.writeI64(waitNanos_item);
_oprot.writeFieldEnd();
}
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
if (!(other instanceof shutdown$args)) return false;
shutdown$args that = (shutdown$args) other;
return
this.waitNanos.equals(that.waitNanos)
;
}
@Override
public String toString() {
return "shutdown$args(" + this.waitNanos + ")";
}
@Override
public int hashCode() {
int hash = 1;
hash = hash * (this.waitNanos.isDefined() ? 0 : new Long(this.waitNanos.get()).hashCode());
return hash;
}
}
static class shutdown$result implements ThriftStruct {
private static final TStruct STRUCT = new TStruct("shutdown_result");
public static class Builder {
public shutdown$result build() {
return new shutdown$result(
);
}
}
public Builder copy() {
Builder builder = new Builder();
return builder;
}
public static ThriftStructCodec CODEC = new ThriftStructCodec3() {
@Override
public shutdown$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
Builder builder = new Builder();
Boolean _done = false;
_iprot.readStructBegin();
while (!_done) {
TField _field = _iprot.readFieldBegin();
if (_field.type == TType.STOP) {
_done = true;
} else {
switch (_field.id) {
default:
TProtocolUtil.skip(_iprot, _field.type);
}
_iprot.readFieldEnd();
}
}
_iprot.readStructEnd();
try {
return builder.build();
} catch (IllegalStateException stateEx) {
throw new TProtocolException(stateEx.getMessage());
}
}
@Override
public void encode(shutdown$result struct, TProtocol oprot) throws org.apache.thrift.TException {
struct.write(oprot);
}
};
public static shutdown$result decode(TProtocol _iprot) throws org.apache.thrift.TException {
return CODEC.decode(_iprot);
}
public static void encode(shutdown$result struct, TProtocol oprot) throws org.apache.thrift.TException {
CODEC.encode(struct, oprot);
}
public shutdown$result(
) {
}
public void write(TProtocol _oprot) throws org.apache.thrift.TException {
validate();
_oprot.writeStructBegin(STRUCT);
_oprot.writeFieldStop();
_oprot.writeStructEnd();
}
private void validate() throws org.apache.thrift.protocol.TProtocolException {
}
@Override
public boolean equals(Object other) {
return this == other;
}
@Override
public String toString() {
return "shutdown$result()";
}
@Override
public int hashCode() {
return super.hashCode();
}
}
/**
* Scray meta service
* The service is provided by scray seed nodes
*/
public static class FinagledClient implements FutureIface {
private com.twitter.finagle.Service service;
private String serviceName;
private TProtocolFactory protocolFactory /* new TBinaryProtocol.Factory */;
private StatsReceiver scopedStats;
public FinagledClient(
com.twitter.finagle.Service service,
TProtocolFactory protocolFactory /* new TBinaryProtocol.Factory */,
String serviceName,
StatsReceiver stats
) {
this.service = service;
this.serviceName = serviceName;
this.protocolFactory = protocolFactory;
if (serviceName != "") {
this.scopedStats = stats.scope(serviceName);
} else {
this.scopedStats = stats;
}
}
// ----- boilerplate that should eventually be moved into finagle:
protected ThriftClientRequest encodeRequest(String name, ThriftStruct args) {
TMemoryBuffer buf = new TMemoryBuffer(512);
TProtocol oprot = protocolFactory.getProtocol(buf);
try {
oprot.writeMessageBegin(new TMessage(name, TMessageType.CALL, 0));
args.write(oprot);
oprot.writeMessageEnd();
} catch (TException e) {
// not real.
}
byte[] bytes = Arrays.copyOfRange(buf.getArray(), 0, buf.length());
return new ThriftClientRequest(bytes, false);
}
protected T decodeResponse(byte[] resBytes, ThriftStructCodec codec) throws TException {
TProtocol iprot = protocolFactory.getProtocol(new TMemoryInputTransport(resBytes));
TMessage msg = iprot.readMessageBegin();
try {
if (msg.type == TMessageType.EXCEPTION) {
TException exception = TApplicationException.read(iprot);
if (exception instanceof SourcedException) {
if (this.serviceName != "") ((SourcedException) exception).serviceName_$eq(this.serviceName);
}
throw exception;
} else {
return codec.decode(iprot);
}
} finally {
iprot.readMessageEnd();
}
}
protected Exception missingResult(String name) {
return new TApplicationException(
TApplicationException.MISSING_RESULT,
"`" + name + "` failed: unknown result"
);
}
protected class __Stats {
public Counter requestsCounter, successCounter, failuresCounter;
public StatsReceiver failuresScope;
public __Stats(String name) {
StatsReceiver scope = FinagledClient.this.scopedStats.scope(name);
this.requestsCounter = scope.counter0("requests");
this.successCounter = scope.counter0("success");
this.failuresCounter = scope.counter0("failures");
this.failuresScope = scope.scope("failures");
}
}
// ----- end boilerplate.
private __Stats ___stats_getServiceEndpoints;
private __Stats __stats_getServiceEndpoints() {
if (___stats_getServiceEndpoints == null) {
___stats_getServiceEndpoints = new __Stats("getServiceEndpoints");
}
return ___stats_getServiceEndpoints;
}
/**
* Fetch a list of service endpoints.
* Each endpoint provides ScrayStatelessTService and ScrayStatefulTService alternatives.
* Queries can address different endpoints for load distribution.
*/
public Future> getServiceEndpoints() {
__stats_getServiceEndpoints().requestsCounter.incr();
Future> rv = this.service.apply(encodeRequest("getServiceEndpoints", new getServiceEndpoints$args())).flatMap(new Function>>() {
public Future> apply(byte[] in) {
try {
getServiceEndpoints$result result = decodeResponse(in, getServiceEndpoints$result.CODEC);
if (result.success.isDefined()) return Future.value(result.success.get());
return Future.exception(missingResult("getServiceEndpoints"));
} catch (TException e) {
return Future.exception(e);
}
}
}).rescue(new Function>>() {
public Future> apply(Throwable t) {
if (t instanceof SourcedException) {
((SourcedException) t).serviceName_$eq(FinagledClient.this.serviceName);
}
return Future.exception(t);
}
});
rv.addEventListener(new FutureEventListener>() {
public void onSuccess(List result) {
__stats_getServiceEndpoints().successCounter.incr();
}
public void onFailure(Throwable t) {
__stats_getServiceEndpoints().failuresCounter.incr();
__stats_getServiceEndpoints().failuresScope.counter0(t.getClass().getName()).incr();
}
});
return rv;
}
private __Stats ___stats_addServiceEndpoint;
private __Stats __stats_addServiceEndpoint() {
if (___stats_addServiceEndpoint == null) {
___stats_addServiceEndpoint = new __Stats("addServiceEndpoint");
}
return ___stats_addServiceEndpoint;
}
/**
* Add new service endpoint.
* The endpoint will be removed after a default expiration period.
*/
public Future addServiceEndpoint(ScrayTServiceEndpoint endpoint) {
__stats_addServiceEndpoint().requestsCounter.incr();
Future rv = this.service.apply(encodeRequest("addServiceEndpoint", new addServiceEndpoint$args(endpoint))).flatMap(new Function>() {
public Future apply(byte[] in) {
try {
addServiceEndpoint$result result = decodeResponse(in, addServiceEndpoint$result.CODEC);
if (result.success.isDefined()) return Future.value(result.success.get());
return Future.exception(missingResult("addServiceEndpoint"));
} catch (TException e) {
return Future.exception(e);
}
}
}).rescue(new Function>() {
public Future apply(Throwable t) {
if (t instanceof SourcedException) {
((SourcedException) t).serviceName_$eq(FinagledClient.this.serviceName);
}
return Future.exception(t);
}
});
rv.addEventListener(new FutureEventListener() {
public void onSuccess(ScrayTServiceEndpoint result) {
__stats_addServiceEndpoint().successCounter.incr();
}
public void onFailure(Throwable t) {
__stats_addServiceEndpoint().failuresCounter.incr();
__stats_addServiceEndpoint().failuresScope.counter0(t.getClass().getName()).incr();
}
});
return rv;
}
private __Stats ___stats_refreshServiceEndpoint;
private __Stats __stats_refreshServiceEndpoint() {
if (___stats_refreshServiceEndpoint == null) {
___stats_refreshServiceEndpoint = new __Stats("refreshServiceEndpoint");
}
return ___stats_refreshServiceEndpoint;
}
/**
* Restore the default expiration period of an endpoint.
*/
public Future refreshServiceEndpoint(scray.service.qmodel.thriftjava.ScrayUUID endpointID) {
__stats_refreshServiceEndpoint().requestsCounter.incr();
Future rv = this.service.apply(encodeRequest("refreshServiceEndpoint", new refreshServiceEndpoint$args(endpointID))).flatMap(new Function>() {
public Future apply(byte[] in) {
try {
refreshServiceEndpoint$result result = decodeResponse(in, refreshServiceEndpoint$result.CODEC);
return Future.value(null);
} catch (TException e) {
return Future.exception(e);
}
}
}).rescue(new Function>() {
public Future apply(Throwable t) {
if (t instanceof SourcedException) {
((SourcedException) t).serviceName_$eq(FinagledClient.this.serviceName);
}
return Future.exception(t);
}
});
rv.addEventListener(new FutureEventListener() {
public void onSuccess(Void result) {
__stats_refreshServiceEndpoint().successCounter.incr();
}
public void onFailure(Throwable t) {
__stats_refreshServiceEndpoint().failuresCounter.incr();
__stats_refreshServiceEndpoint().failuresScope.counter0(t.getClass().getName()).incr();
}
});
return rv;
}
private __Stats ___stats_ping;
private __Stats __stats_ping() {
if (___stats_ping == null) {
___stats_ping = new __Stats("ping");
}
return ___stats_ping;
}
/**
* Return vital sign
*/
public Future ping() {
__stats_ping().requestsCounter.incr();
Future rv = this.service.apply(encodeRequest("ping", new ping$args())).flatMap(new Function>() {
public Future apply(byte[] in) {
try {
ping$result result = decodeResponse(in, ping$result.CODEC);
if (result.success.isDefined()) return Future.value(result.success.get());
return Future.exception(missingResult("ping"));
} catch (TException e) {
return Future.exception(e);
}
}
}).rescue(new Function>() {
public Future apply(Throwable t) {
if (t instanceof SourcedException) {
((SourcedException) t).serviceName_$eq(FinagledClient.this.serviceName);
}
return Future.exception(t);
}
});
rv.addEventListener(new FutureEventListener() {
public void onSuccess(Boolean result) {
__stats_ping().successCounter.incr();
}
public void onFailure(Throwable t) {
__stats_ping().failuresCounter.incr();
__stats_ping().failuresScope.counter0(t.getClass().getName()).incr();
}
});
return rv;
}
private __Stats ___stats_shutdown;
private __Stats __stats_shutdown() {
if (___stats_shutdown == null) {
___stats_shutdown = new __Stats("shutdown");
}
return ___stats_shutdown;
}
/**
* Shutdown the server
*/
public Future shutdown(com.twitter.scrooge.Option waitNanos) {
__stats_shutdown().requestsCounter.incr();
Future rv = this.service.apply(encodeRequest("shutdown", new shutdown$args(waitNanos))).flatMap(new Function>() {
public Future apply(byte[] in) {
try {
shutdown$result result = decodeResponse(in, shutdown$result.CODEC);
return Future.value(null);
} catch (TException e) {
return Future.exception(e);
}
}
}).rescue(new Function>() {
public Future apply(Throwable t) {
if (t instanceof SourcedException) {
((SourcedException) t).serviceName_$eq(FinagledClient.this.serviceName);
}
return Future.exception(t);
}
});
rv.addEventListener(new FutureEventListener() {
public void onSuccess(Void result) {
__stats_shutdown().successCounter.incr();
}
public void onFailure(Throwable t) {
__stats_shutdown().failuresCounter.incr();
__stats_shutdown().failuresScope.counter0(t.getClass().getName()).incr();
}
});
return rv;
}
}
/**
* Scray meta service
* The service is provided by scray seed nodes
*/
public static class FinagledService extends Service {
final private FutureIface iface;
final private TProtocolFactory protocolFactory;
public FinagledService(final FutureIface iface, final TProtocolFactory protocolFactory) {
this.iface = iface;
this.protocolFactory = protocolFactory;
addFunction("getServiceEndpoints", new Function2>() {
public Future apply(TProtocol iprot, final Integer seqid) {
try {
getServiceEndpoints$args args = getServiceEndpoints$args.decode(iprot);
iprot.readMessageEnd();
Future> result;
try {
result = iface.getServiceEndpoints();
} catch (Throwable t) {
result = Future.exception(t);
}
return result.flatMap(new Function, Future>() {
public Future apply(List value){
return reply("getServiceEndpoints", seqid, new getServiceEndpoints$result.Builder().success(value).build());
}
}).rescue(new Function>() {
public Future apply(Throwable t) {
return Future.exception(t);
}
});
} catch (TProtocolException e) {
try {
iprot.readMessageEnd();
return exception("getServiceEndpoints", seqid, TApplicationException.PROTOCOL_ERROR, e.getMessage());
} catch (Exception unrecoverable) {
return Future.exception(unrecoverable);
}
} catch (Throwable t) {
return Future.exception(t);
}
}
});
addFunction("addServiceEndpoint", new Function2>() {
public Future apply(TProtocol iprot, final Integer seqid) {
try {
addServiceEndpoint$args args = addServiceEndpoint$args.decode(iprot);
iprot.readMessageEnd();
Future result;
try {
result = iface.addServiceEndpoint(args.endpoint);
} catch (Throwable t) {
result = Future.exception(t);
}
return result.flatMap(new Function>() {
public Future apply(ScrayTServiceEndpoint value){
return reply("addServiceEndpoint", seqid, new addServiceEndpoint$result.Builder().success(value).build());
}
}).rescue(new Function>() {
public Future apply(Throwable t) {
return Future.exception(t);
}
});
} catch (TProtocolException e) {
try {
iprot.readMessageEnd();
return exception("addServiceEndpoint", seqid, TApplicationException.PROTOCOL_ERROR, e.getMessage());
} catch (Exception unrecoverable) {
return Future.exception(unrecoverable);
}
} catch (Throwable t) {
return Future.exception(t);
}
}
});
addFunction("refreshServiceEndpoint", new Function2>() {
public Future apply(TProtocol iprot, final Integer seqid) {
try {
refreshServiceEndpoint$args args = refreshServiceEndpoint$args.decode(iprot);
iprot.readMessageEnd();
Future result;
try {
result = iface.refreshServiceEndpoint(args.endpointID);
} catch (Throwable t) {
result = Future.exception(t);
}
return result.flatMap(new Function>() {
public Future apply(Void value){
return reply("refreshServiceEndpoint", seqid, new refreshServiceEndpoint$result.Builder().build());
}
}).rescue(new Function>() {
public Future apply(Throwable t) {
return Future.exception(t);
}
});
} catch (TProtocolException e) {
try {
iprot.readMessageEnd();
return exception("refreshServiceEndpoint", seqid, TApplicationException.PROTOCOL_ERROR, e.getMessage());
} catch (Exception unrecoverable) {
return Future.exception(unrecoverable);
}
} catch (Throwable t) {
return Future.exception(t);
}
}
});
addFunction("ping", new Function2>() {
public Future apply(TProtocol iprot, final Integer seqid) {
try {
ping$args args = ping$args.decode(iprot);
iprot.readMessageEnd();
Future result;
try {
result = iface.ping();
} catch (Throwable t) {
result = Future.exception(t);
}
return result.flatMap(new Function>() {
public Future apply(Boolean value){
return reply("ping", seqid, new ping$result.Builder().success(value).build());
}
}).rescue(new Function>() {
public Future apply(Throwable t) {
return Future.exception(t);
}
});
} catch (TProtocolException e) {
try {
iprot.readMessageEnd();
return exception("ping", seqid, TApplicationException.PROTOCOL_ERROR, e.getMessage());
} catch (Exception unrecoverable) {
return Future.exception(unrecoverable);
}
} catch (Throwable t) {
return Future.exception(t);
}
}
});
addFunction("shutdown", new Function2>() {
public Future apply(TProtocol iprot, final Integer seqid) {
try {
shutdown$args args = shutdown$args.decode(iprot);
iprot.readMessageEnd();
Future result;
try {
result = iface.shutdown(args.waitNanos);
} catch (Throwable t) {
result = Future.exception(t);
}
return result.flatMap(new Function>() {
public Future apply(Void value){
return reply("shutdown", seqid, new shutdown$result.Builder().build());
}
}).rescue(new Function>() {
public Future apply(Throwable t) {
return Future.exception(t);
}
});
} catch (TProtocolException e) {
try {
iprot.readMessageEnd();
return exception("shutdown", seqid, TApplicationException.PROTOCOL_ERROR, e.getMessage());
} catch (Exception unrecoverable) {
return Future.exception(unrecoverable);
}
} catch (Throwable t) {
return Future.exception(t);
}
}
});
}
// ----- boilerplate that should eventually be moved into finagle:
protected Map>> functionMap =
new HashMap>>();
protected void addFunction(String name, Function2> fn) {
functionMap.put(name, fn);
}
protected Function2> getFunction(String name) {
return functionMap.get(name);
}
protected Future exception(String name, int seqid, int code, String message) {
try {
TApplicationException x = new TApplicationException(code, message);
TMemoryBuffer memoryBuffer = new TMemoryBuffer(512);
TProtocol oprot = protocolFactory.getProtocol(memoryBuffer);
oprot.writeMessageBegin(new TMessage(name, TMessageType.EXCEPTION, seqid));
x.write(oprot);
oprot.writeMessageEnd();
oprot.getTransport().flush();
return Future.value(Arrays.copyOfRange(memoryBuffer.getArray(), 0, memoryBuffer.length()));
} catch (Exception e) {
return Future.exception(e);
}
}
protected Future reply(String name, int seqid, ThriftStruct result) {
try {
TMemoryBuffer memoryBuffer = new TMemoryBuffer(512);
TProtocol oprot = protocolFactory.getProtocol(memoryBuffer);
oprot.writeMessageBegin(new TMessage(name, TMessageType.REPLY, seqid));
result.write(oprot);
oprot.writeMessageEnd();
return Future.value(Arrays.copyOfRange(memoryBuffer.getArray(), 0, memoryBuffer.length()));
} catch (Exception e) {
return Future.exception(e);
}
}
public final Future apply(byte[] request) {
TTransport inputTransport = new TMemoryInputTransport(request);
TProtocol iprot = protocolFactory.getProtocol(inputTransport);
try {
TMessage msg = iprot.readMessageBegin();
Function2> f = functionMap.get(msg.name);
if (f != null) {
return f.apply(iprot, msg.seqid);
} else {
TProtocolUtil.skip(iprot, TType.STRUCT);
return exception(msg.name, msg.seqid, TApplicationException.UNKNOWN_METHOD, "Invalid method name: '" + msg.name + "'");
}
} catch (Exception e) {
return Future.exception(e);
}
}
// ---- end boilerplate.
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy