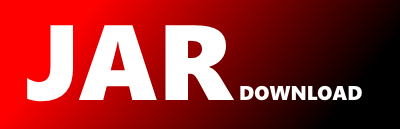
scray.service.qmodel.thrifscala.ScrayTColumnInfo.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scray-service Show documentation
Show all versions of scray-service Show documentation
scray remote query service interface
/**
* Generated by Scrooge
* version: 4.16.0
* rev: 0201cac9fdd6188248d42da91fd14c87744cc4a5
* built at: 20170421-124523
*/
package scray.service.qmodel.thrifscala
import com.twitter.scrooge.{
HasThriftStructCodec3,
LazyTProtocol,
TFieldBlob,
ThriftException,
ThriftStruct,
ThriftStructCodec3,
ThriftStructFieldInfo,
ThriftStructMetaData,
ThriftUtil
}
import org.apache.thrift.protocol._
import org.apache.thrift.transport.{TMemoryBuffer, TTransport}
import java.nio.ByteBuffer
import java.util.Arrays
import scala.collection.immutable.{Map => immutable$Map}
import scala.collection.mutable.Builder
import scala.collection.mutable.{
ArrayBuffer => mutable$ArrayBuffer, Buffer => mutable$Buffer,
HashMap => mutable$HashMap, HashSet => mutable$HashSet}
import scala.collection.{Map, Set}
/**
* Column identifier
*/
object ScrayTColumnInfo extends ThriftStructCodec3[ScrayTColumnInfo] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("ScrayTColumnInfo")
val NameField = new TField("name", TType.STRING, 1)
val NameFieldManifest = implicitly[Manifest[String]]
val ColumnTField = new TField("columnT", TType.STRING, 2)
val ColumnTFieldManifest = implicitly[Manifest[String]]
val TableIdField = new TField("tableId", TType.STRUCT, 3)
val TableIdFieldManifest = implicitly[Manifest[scray.service.qmodel.thrifscala.ScrayTTableInfo]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
NameField,
false,
false,
NameFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
),
new ThriftStructFieldInfo(
ColumnTField,
true,
false,
ColumnTFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
),
new ThriftStructFieldInfo(
TableIdField,
true,
false,
TableIdFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: ScrayTColumnInfo): Unit = {
}
def withoutPassthroughFields(original: ScrayTColumnInfo): ScrayTColumnInfo =
new Immutable(
name =
{
val field = original.name
field
},
columnT =
{
val field = original.columnT
field.map { field =>
field
}
},
tableId =
{
val field = original.tableId
field.map { field =>
scray.service.qmodel.thrifscala.ScrayTTableInfo.withoutPassthroughFields(field)
}
}
)
override def encode(_item: ScrayTColumnInfo, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
private[this] def lazyDecode(_iprot: LazyTProtocol): ScrayTColumnInfo = {
var nameOffset: Int = -1
var columnTOffset: Int = -1
var tableId: Option[scray.service.qmodel.thrifscala.ScrayTTableInfo] = None
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
val _start_offset = _iprot.offset
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 1 =>
_field.`type` match {
case TType.STRING =>
nameOffset = _iprot.offsetSkipString
case _actualType =>
val _expectedType = TType.STRING
throw new TProtocolException(
"Received wrong type for field 'name' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 2 =>
_field.`type` match {
case TType.STRING =>
columnTOffset = _iprot.offsetSkipString
case _actualType =>
val _expectedType = TType.STRING
throw new TProtocolException(
"Received wrong type for field 'columnT' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 3 =>
_field.`type` match {
case TType.STRUCT =>
tableId = Some(readTableIdValue(_iprot))
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'tableId' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new LazyImmutable(
_iprot,
_iprot.buffer,
_start_offset,
_iprot.offset,
nameOffset,
columnTOffset,
tableId,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
override def decode(_iprot: TProtocol): ScrayTColumnInfo =
_iprot match {
case i: LazyTProtocol => lazyDecode(i)
case i => eagerDecode(i)
}
private[this] def eagerDecode(_iprot: TProtocol): ScrayTColumnInfo = {
var name: String = null
var columnT: _root_.scala.Option[String] = _root_.scala.None
var tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo] = _root_.scala.None
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 1 =>
_field.`type` match {
case TType.STRING =>
name = readNameValue(_iprot)
case _actualType =>
val _expectedType = TType.STRING
throw new TProtocolException(
"Received wrong type for field 'name' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 2 =>
_field.`type` match {
case TType.STRING =>
columnT = _root_.scala.Some(readColumnTValue(_iprot))
case _actualType =>
val _expectedType = TType.STRING
throw new TProtocolException(
"Received wrong type for field 'columnT' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 3 =>
_field.`type` match {
case TType.STRUCT =>
tableId = _root_.scala.Some(readTableIdValue(_iprot))
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'tableId' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Immutable(
name,
columnT,
tableId,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
name: String,
columnT: _root_.scala.Option[String] = _root_.scala.None,
tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo] = _root_.scala.None
): ScrayTColumnInfo =
new Immutable(
name,
columnT,
tableId
)
def unapply(_item: ScrayTColumnInfo): _root_.scala.Option[_root_.scala.Tuple3[String, Option[String], Option[scray.service.qmodel.thrifscala.ScrayTTableInfo]]] = _root_.scala.Some(_item.toTuple)
@inline private def readNameValue(_iprot: TProtocol): String = {
_iprot.readString()
}
@inline private def writeNameField(name_item: String, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(NameField)
writeNameValue(name_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeNameValue(name_item: String, _oprot: TProtocol): Unit = {
_oprot.writeString(name_item)
}
@inline private def readColumnTValue(_iprot: TProtocol): String = {
_iprot.readString()
}
@inline private def writeColumnTField(columnT_item: String, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(ColumnTField)
writeColumnTValue(columnT_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeColumnTValue(columnT_item: String, _oprot: TProtocol): Unit = {
_oprot.writeString(columnT_item)
}
@inline private def readTableIdValue(_iprot: TProtocol): scray.service.qmodel.thrifscala.ScrayTTableInfo = {
scray.service.qmodel.thrifscala.ScrayTTableInfo.decode(_iprot)
}
@inline private def writeTableIdField(tableId_item: scray.service.qmodel.thrifscala.ScrayTTableInfo, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(TableIdField)
writeTableIdValue(tableId_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeTableIdValue(tableId_item: scray.service.qmodel.thrifscala.ScrayTTableInfo, _oprot: TProtocol): Unit = {
tableId_item.write(_oprot)
}
object Immutable extends ThriftStructCodec3[ScrayTColumnInfo] {
override def encode(_item: ScrayTColumnInfo, _oproto: TProtocol): Unit = { _item.write(_oproto) }
override def decode(_iprot: TProtocol): ScrayTColumnInfo = ScrayTColumnInfo.decode(_iprot)
override lazy val metaData: ThriftStructMetaData[ScrayTColumnInfo] = ScrayTColumnInfo.metaData
}
/**
* The default read-only implementation of ScrayTColumnInfo. You typically should not need to
* directly reference this class; instead, use the ScrayTColumnInfo.apply method to construct
* new instances.
*/
class Immutable(
val name: String,
val columnT: _root_.scala.Option[String],
val tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo],
override val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ScrayTColumnInfo {
def this(
name: String,
columnT: _root_.scala.Option[String] = _root_.scala.None,
tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo] = _root_.scala.None
) = this(
name,
columnT,
tableId,
Map.empty
)
}
/**
* This is another Immutable, this however keeps strings as lazy values that are lazily decoded from the backing
* array byte on read.
*/
private[this] class LazyImmutable(
_proto: LazyTProtocol,
_buf: Array[Byte],
_start_offset: Int,
_end_offset: Int,
nameOffset: Int,
columnTOffset: Int,
val tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo],
override val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ScrayTColumnInfo {
override def write(_oprot: TProtocol): Unit = {
_oprot match {
case i: LazyTProtocol => i.writeRaw(_buf, _start_offset, _end_offset - _start_offset)
case _ => super.write(_oprot)
}
}
lazy val name: String =
if (nameOffset == -1)
null
else {
_proto.decodeString(_buf, nameOffset)
}
lazy val columnT: _root_.scala.Option[String] =
if (columnTOffset == -1)
None
else {
Some(_proto.decodeString(_buf, columnTOffset))
}
/**
* Override the super hash code to make it a lazy val rather than def.
*
* Calculating the hash code can be expensive, caching it where possible
* can provide significant performance wins. (Key in a hash map for instance)
* Usually not safe since the normal constructor will accept a mutable map or
* set as an arg
* Here however we control how the class is generated from serialized data.
* With the class private and the contract that we throw away our mutable references
* having the hash code lazy here is safe.
*/
override lazy val hashCode = super.hashCode
}
/**
* This Proxy trait allows you to extend the ScrayTColumnInfo trait with additional state or
* behavior and implement the read-only methods from ScrayTColumnInfo using an underlying
* instance.
*/
trait Proxy extends ScrayTColumnInfo {
protected def _underlying_ScrayTColumnInfo: ScrayTColumnInfo
override def name: String = _underlying_ScrayTColumnInfo.name
override def columnT: _root_.scala.Option[String] = _underlying_ScrayTColumnInfo.columnT
override def tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo] = _underlying_ScrayTColumnInfo.tableId
override def _passthroughFields = _underlying_ScrayTColumnInfo._passthroughFields
}
}
trait ScrayTColumnInfo
extends ThriftStruct
with _root_.scala.Product3[String, Option[String], Option[scray.service.qmodel.thrifscala.ScrayTTableInfo]]
with HasThriftStructCodec3[ScrayTColumnInfo]
with java.io.Serializable
{
import ScrayTColumnInfo._
def name: String
def columnT: _root_.scala.Option[String]
def tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo]
def _passthroughFields: immutable$Map[Short, TFieldBlob] = immutable$Map.empty
def _1 = name
def _2 = columnT
def _3 = tableId
def toTuple: _root_.scala.Tuple3[String, Option[String], Option[scray.service.qmodel.thrifscala.ScrayTTableInfo]] = {
(
name,
columnT,
tableId
)
}
/**
* Gets a field value encoded as a binary blob using TCompactProtocol. If the specified field
* is present in the passthrough map, that value is returned. Otherwise, if the specified field
* is known and not optional and set to None, then the field is serialized and returned.
*/
def getFieldBlob(_fieldId: Short): _root_.scala.Option[TFieldBlob] = {
lazy val _buff = new TMemoryBuffer(32)
lazy val _oprot = new TCompactProtocol(_buff)
_passthroughFields.get(_fieldId) match {
case blob: _root_.scala.Some[TFieldBlob] => blob
case _root_.scala.None => {
val _fieldOpt: _root_.scala.Option[TField] =
_fieldId match {
case 1 =>
if (name ne null) {
writeNameValue(name, _oprot)
_root_.scala.Some(ScrayTColumnInfo.NameField)
} else {
_root_.scala.None
}
case 2 =>
if (columnT.isDefined) {
writeColumnTValue(columnT.get, _oprot)
_root_.scala.Some(ScrayTColumnInfo.ColumnTField)
} else {
_root_.scala.None
}
case 3 =>
if (tableId.isDefined) {
writeTableIdValue(tableId.get, _oprot)
_root_.scala.Some(ScrayTColumnInfo.TableIdField)
} else {
_root_.scala.None
}
case _ => _root_.scala.None
}
_fieldOpt match {
case _root_.scala.Some(_field) =>
val _data = Arrays.copyOfRange(_buff.getArray, 0, _buff.length)
_root_.scala.Some(TFieldBlob(_field, _data))
case _root_.scala.None =>
_root_.scala.None
}
}
}
}
/**
* Collects TCompactProtocol-encoded field values according to `getFieldBlob` into a map.
*/
def getFieldBlobs(ids: TraversableOnce[Short]): immutable$Map[Short, TFieldBlob] =
(ids flatMap { id => getFieldBlob(id) map { id -> _ } }).toMap
/**
* Sets a field using a TCompactProtocol-encoded binary blob. If the field is a known
* field, the blob is decoded and the field is set to the decoded value. If the field
* is unknown and passthrough fields are enabled, then the blob will be stored in
* _passthroughFields.
*/
def setField(_blob: TFieldBlob): ScrayTColumnInfo = {
var name: String = this.name
var columnT: _root_.scala.Option[String] = this.columnT
var tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo] = this.tableId
var _passthroughFields = this._passthroughFields
_blob.id match {
case 1 =>
name = readNameValue(_blob.read)
case 2 =>
columnT = _root_.scala.Some(readColumnTValue(_blob.read))
case 3 =>
tableId = _root_.scala.Some(readTableIdValue(_blob.read))
case _ => _passthroughFields += (_blob.id -> _blob)
}
new Immutable(
name,
columnT,
tableId,
_passthroughFields
)
}
/**
* If the specified field is optional, it is set to None. Otherwise, if the field is
* known, it is reverted to its default value; if the field is unknown, it is removed
* from the passthroughFields map, if present.
*/
def unsetField(_fieldId: Short): ScrayTColumnInfo = {
var name: String = this.name
var columnT: _root_.scala.Option[String] = this.columnT
var tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo] = this.tableId
_fieldId match {
case 1 =>
name = null
case 2 =>
columnT = _root_.scala.None
case 3 =>
tableId = _root_.scala.None
case _ =>
}
new Immutable(
name,
columnT,
tableId,
_passthroughFields - _fieldId
)
}
/**
* If the specified field is optional, it is set to None. Otherwise, if the field is
* known, it is reverted to its default value; if the field is unknown, it is removed
* from the passthroughFields map, if present.
*/
def unsetName: ScrayTColumnInfo = unsetField(1)
def unsetColumnT: ScrayTColumnInfo = unsetField(2)
def unsetTableId: ScrayTColumnInfo = unsetField(3)
override def write(_oprot: TProtocol): Unit = {
ScrayTColumnInfo.validate(this)
_oprot.writeStructBegin(Struct)
if (name ne null) writeNameField(name, _oprot)
if (columnT.isDefined) writeColumnTField(columnT.get, _oprot)
if (tableId.isDefined) writeTableIdField(tableId.get, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
name: String = this.name,
columnT: _root_.scala.Option[String] = this.columnT,
tableId: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTTableInfo] = this.tableId,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): ScrayTColumnInfo =
new Immutable(
name,
columnT,
tableId,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[ScrayTColumnInfo]
private def _equals(x: ScrayTColumnInfo, y: ScrayTColumnInfo): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[ScrayTColumnInfo]) &&
_passthroughFields == other.asInstanceOf[ScrayTColumnInfo]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 3
override def productElement(n: Int): Any = n match {
case 0 => this.name
case 1 => this.columnT
case 2 => this.tableId
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "ScrayTColumnInfo"
def _codec: ThriftStructCodec3[ScrayTColumnInfo] = ScrayTColumnInfo
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy