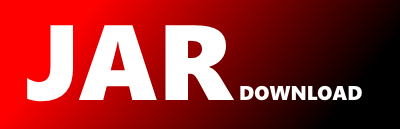
scray.service.qmodel.thrifscala.ScrayTQuery.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scray-service Show documentation
Show all versions of scray-service Show documentation
scray remote query service interface
/**
* Generated by Scrooge
* version: 4.16.0
* rev: 0201cac9fdd6188248d42da91fd14c87744cc4a5
* built at: 20170421-124523
*/
package scray.service.qmodel.thrifscala
import com.twitter.scrooge.{
HasThriftStructCodec3,
LazyTProtocol,
TFieldBlob,
ThriftException,
ThriftStruct,
ThriftStructCodec3,
ThriftStructFieldInfo,
ThriftStructMetaData,
ThriftUtil
}
import org.apache.thrift.protocol._
import org.apache.thrift.transport.{TMemoryBuffer, TTransport}
import java.nio.ByteBuffer
import java.util.Arrays
import scala.collection.immutable.{Map => immutable$Map}
import scala.collection.mutable.Builder
import scala.collection.mutable.{
ArrayBuffer => mutable$ArrayBuffer, Buffer => mutable$Buffer,
HashMap => mutable$HashMap, HashSet => mutable$HashSet}
import scala.collection.{Map, Set}
/**
* Main query type
*/
object ScrayTQuery extends ThriftStructCodec3[ScrayTQuery] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("ScrayTQuery")
val QueryInfoField = new TField("queryInfo", TType.STRUCT, 1)
val QueryInfoFieldManifest = implicitly[Manifest[scray.service.qmodel.thrifscala.ScrayTQueryInfo]]
val ValuesField = new TField("values", TType.MAP, 2)
val ValuesFieldManifest = implicitly[Manifest[Map[String, ByteBuffer]]]
val QueryExpressionField = new TField("queryExpression", TType.STRING, 3)
val QueryExpressionFieldManifest = implicitly[Manifest[String]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
QueryInfoField,
false,
false,
QueryInfoFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
),
new ThriftStructFieldInfo(
ValuesField,
false,
false,
ValuesFieldManifest,
_root_.scala.Some(implicitly[Manifest[String]]),
_root_.scala.Some(implicitly[Manifest[ByteBuffer]]),
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
),
new ThriftStructFieldInfo(
QueryExpressionField,
false,
false,
QueryExpressionFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: ScrayTQuery): Unit = {
}
def withoutPassthroughFields(original: ScrayTQuery): ScrayTQuery =
new Immutable(
queryInfo =
{
val field = original.queryInfo
scray.service.qmodel.thrifscala.ScrayTQueryInfo.withoutPassthroughFields(field)
},
values =
{
val field = original.values
field.map { case (key, value) =>
val newKey = {
val field = key
field
}
val newValue = {
val field = value
field
}
newKey -> newValue
}
},
queryExpression =
{
val field = original.queryExpression
field
}
)
override def encode(_item: ScrayTQuery, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
private[this] def lazyDecode(_iprot: LazyTProtocol): ScrayTQuery = {
var queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo = null
var values: Map[String, ByteBuffer] = Map[String, ByteBuffer]()
var queryExpressionOffset: Int = -1
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
val _start_offset = _iprot.offset
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 1 =>
_field.`type` match {
case TType.STRUCT =>
queryInfo = readQueryInfoValue(_iprot)
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'queryInfo' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 2 =>
_field.`type` match {
case TType.MAP =>
values = readValuesValue(_iprot)
case _actualType =>
val _expectedType = TType.MAP
throw new TProtocolException(
"Received wrong type for field 'values' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 3 =>
_field.`type` match {
case TType.STRING =>
queryExpressionOffset = _iprot.offsetSkipString
case _actualType =>
val _expectedType = TType.STRING
throw new TProtocolException(
"Received wrong type for field 'queryExpression' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new LazyImmutable(
_iprot,
_iprot.buffer,
_start_offset,
_iprot.offset,
queryInfo,
values,
queryExpressionOffset,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
override def decode(_iprot: TProtocol): ScrayTQuery =
_iprot match {
case i: LazyTProtocol => lazyDecode(i)
case i => eagerDecode(i)
}
private[this] def eagerDecode(_iprot: TProtocol): ScrayTQuery = {
var queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo = null
var values: Map[String, ByteBuffer] = Map[String, ByteBuffer]()
var queryExpression: String = null
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 1 =>
_field.`type` match {
case TType.STRUCT =>
queryInfo = readQueryInfoValue(_iprot)
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'queryInfo' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 2 =>
_field.`type` match {
case TType.MAP =>
values = readValuesValue(_iprot)
case _actualType =>
val _expectedType = TType.MAP
throw new TProtocolException(
"Received wrong type for field 'values' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 3 =>
_field.`type` match {
case TType.STRING =>
queryExpression = readQueryExpressionValue(_iprot)
case _actualType =>
val _expectedType = TType.STRING
throw new TProtocolException(
"Received wrong type for field 'queryExpression' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Immutable(
queryInfo,
values,
queryExpression,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo,
values: Map[String, ByteBuffer] = Map[String, ByteBuffer](),
queryExpression: String
): ScrayTQuery =
new Immutable(
queryInfo,
values,
queryExpression
)
def unapply(_item: ScrayTQuery): _root_.scala.Option[_root_.scala.Tuple3[scray.service.qmodel.thrifscala.ScrayTQueryInfo, Map[String, ByteBuffer], String]] = _root_.scala.Some(_item.toTuple)
@inline private def readQueryInfoValue(_iprot: TProtocol): scray.service.qmodel.thrifscala.ScrayTQueryInfo = {
scray.service.qmodel.thrifscala.ScrayTQueryInfo.decode(_iprot)
}
@inline private def writeQueryInfoField(queryInfo_item: scray.service.qmodel.thrifscala.ScrayTQueryInfo, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(QueryInfoField)
writeQueryInfoValue(queryInfo_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeQueryInfoValue(queryInfo_item: scray.service.qmodel.thrifscala.ScrayTQueryInfo, _oprot: TProtocol): Unit = {
queryInfo_item.write(_oprot)
}
@inline private def readValuesValue(_iprot: TProtocol): Map[String, ByteBuffer] = {
val _map = _iprot.readMapBegin()
if (_map.size == 0) {
_iprot.readMapEnd()
Map.empty[String, ByteBuffer]
} else {
val _rv = new mutable$HashMap[String, ByteBuffer]
var _i = 0
while (_i < _map.size) {
val _key = {
_iprot.readString()
}
val _value = {
_iprot.readBinary()
}
_rv(_key) = _value
_i += 1
}
_iprot.readMapEnd()
_rv
}
}
@inline private def writeValuesField(values_item: Map[String, ByteBuffer], _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(ValuesField)
writeValuesValue(values_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeValuesValue(values_item: Map[String, ByteBuffer], _oprot: TProtocol): Unit = {
_oprot.writeMapBegin(new TMap(TType.STRING, TType.STRING, values_item.size))
values_item.foreach { case (values_item_key, values_item_value) =>
_oprot.writeString(values_item_key)
_oprot.writeBinary(values_item_value)
}
_oprot.writeMapEnd()
}
@inline private def readQueryExpressionValue(_iprot: TProtocol): String = {
_iprot.readString()
}
@inline private def writeQueryExpressionField(queryExpression_item: String, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(QueryExpressionField)
writeQueryExpressionValue(queryExpression_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeQueryExpressionValue(queryExpression_item: String, _oprot: TProtocol): Unit = {
_oprot.writeString(queryExpression_item)
}
object Immutable extends ThriftStructCodec3[ScrayTQuery] {
override def encode(_item: ScrayTQuery, _oproto: TProtocol): Unit = { _item.write(_oproto) }
override def decode(_iprot: TProtocol): ScrayTQuery = ScrayTQuery.decode(_iprot)
override lazy val metaData: ThriftStructMetaData[ScrayTQuery] = ScrayTQuery.metaData
}
/**
* The default read-only implementation of ScrayTQuery. You typically should not need to
* directly reference this class; instead, use the ScrayTQuery.apply method to construct
* new instances.
*/
class Immutable(
val queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo,
val values: Map[String, ByteBuffer],
val queryExpression: String,
override val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ScrayTQuery {
def this(
queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo,
values: Map[String, ByteBuffer] = Map[String, ByteBuffer](),
queryExpression: String
) = this(
queryInfo,
values,
queryExpression,
Map.empty
)
}
/**
* This is another Immutable, this however keeps strings as lazy values that are lazily decoded from the backing
* array byte on read.
*/
private[this] class LazyImmutable(
_proto: LazyTProtocol,
_buf: Array[Byte],
_start_offset: Int,
_end_offset: Int,
val queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo,
val values: Map[String, ByteBuffer],
queryExpressionOffset: Int,
override val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ScrayTQuery {
override def write(_oprot: TProtocol): Unit = {
_oprot match {
case i: LazyTProtocol => i.writeRaw(_buf, _start_offset, _end_offset - _start_offset)
case _ => super.write(_oprot)
}
}
lazy val queryExpression: String =
if (queryExpressionOffset == -1)
null
else {
_proto.decodeString(_buf, queryExpressionOffset)
}
/**
* Override the super hash code to make it a lazy val rather than def.
*
* Calculating the hash code can be expensive, caching it where possible
* can provide significant performance wins. (Key in a hash map for instance)
* Usually not safe since the normal constructor will accept a mutable map or
* set as an arg
* Here however we control how the class is generated from serialized data.
* With the class private and the contract that we throw away our mutable references
* having the hash code lazy here is safe.
*/
override lazy val hashCode = super.hashCode
}
/**
* This Proxy trait allows you to extend the ScrayTQuery trait with additional state or
* behavior and implement the read-only methods from ScrayTQuery using an underlying
* instance.
*/
trait Proxy extends ScrayTQuery {
protected def _underlying_ScrayTQuery: ScrayTQuery
override def queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo = _underlying_ScrayTQuery.queryInfo
override def values: Map[String, ByteBuffer] = _underlying_ScrayTQuery.values
override def queryExpression: String = _underlying_ScrayTQuery.queryExpression
override def _passthroughFields = _underlying_ScrayTQuery._passthroughFields
}
}
trait ScrayTQuery
extends ThriftStruct
with _root_.scala.Product3[scray.service.qmodel.thrifscala.ScrayTQueryInfo, Map[String, ByteBuffer], String]
with HasThriftStructCodec3[ScrayTQuery]
with java.io.Serializable
{
import ScrayTQuery._
def queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo
def values: Map[String, ByteBuffer]
def queryExpression: String
def _passthroughFields: immutable$Map[Short, TFieldBlob] = immutable$Map.empty
def _1 = queryInfo
def _2 = values
def _3 = queryExpression
def toTuple: _root_.scala.Tuple3[scray.service.qmodel.thrifscala.ScrayTQueryInfo, Map[String, ByteBuffer], String] = {
(
queryInfo,
values,
queryExpression
)
}
/**
* Gets a field value encoded as a binary blob using TCompactProtocol. If the specified field
* is present in the passthrough map, that value is returned. Otherwise, if the specified field
* is known and not optional and set to None, then the field is serialized and returned.
*/
def getFieldBlob(_fieldId: Short): _root_.scala.Option[TFieldBlob] = {
lazy val _buff = new TMemoryBuffer(32)
lazy val _oprot = new TCompactProtocol(_buff)
_passthroughFields.get(_fieldId) match {
case blob: _root_.scala.Some[TFieldBlob] => blob
case _root_.scala.None => {
val _fieldOpt: _root_.scala.Option[TField] =
_fieldId match {
case 1 =>
if (queryInfo ne null) {
writeQueryInfoValue(queryInfo, _oprot)
_root_.scala.Some(ScrayTQuery.QueryInfoField)
} else {
_root_.scala.None
}
case 2 =>
if (values ne null) {
writeValuesValue(values, _oprot)
_root_.scala.Some(ScrayTQuery.ValuesField)
} else {
_root_.scala.None
}
case 3 =>
if (queryExpression ne null) {
writeQueryExpressionValue(queryExpression, _oprot)
_root_.scala.Some(ScrayTQuery.QueryExpressionField)
} else {
_root_.scala.None
}
case _ => _root_.scala.None
}
_fieldOpt match {
case _root_.scala.Some(_field) =>
val _data = Arrays.copyOfRange(_buff.getArray, 0, _buff.length)
_root_.scala.Some(TFieldBlob(_field, _data))
case _root_.scala.None =>
_root_.scala.None
}
}
}
}
/**
* Collects TCompactProtocol-encoded field values according to `getFieldBlob` into a map.
*/
def getFieldBlobs(ids: TraversableOnce[Short]): immutable$Map[Short, TFieldBlob] =
(ids flatMap { id => getFieldBlob(id) map { id -> _ } }).toMap
/**
* Sets a field using a TCompactProtocol-encoded binary blob. If the field is a known
* field, the blob is decoded and the field is set to the decoded value. If the field
* is unknown and passthrough fields are enabled, then the blob will be stored in
* _passthroughFields.
*/
def setField(_blob: TFieldBlob): ScrayTQuery = {
var queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo = this.queryInfo
var values: Map[String, ByteBuffer] = this.values
var queryExpression: String = this.queryExpression
var _passthroughFields = this._passthroughFields
_blob.id match {
case 1 =>
queryInfo = readQueryInfoValue(_blob.read)
case 2 =>
values = readValuesValue(_blob.read)
case 3 =>
queryExpression = readQueryExpressionValue(_blob.read)
case _ => _passthroughFields += (_blob.id -> _blob)
}
new Immutable(
queryInfo,
values,
queryExpression,
_passthroughFields
)
}
/**
* If the specified field is optional, it is set to None. Otherwise, if the field is
* known, it is reverted to its default value; if the field is unknown, it is removed
* from the passthroughFields map, if present.
*/
def unsetField(_fieldId: Short): ScrayTQuery = {
var queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo = this.queryInfo
var values: Map[String, ByteBuffer] = this.values
var queryExpression: String = this.queryExpression
_fieldId match {
case 1 =>
queryInfo = null
case 2 =>
values = Map[String, ByteBuffer]()
case 3 =>
queryExpression = null
case _ =>
}
new Immutable(
queryInfo,
values,
queryExpression,
_passthroughFields - _fieldId
)
}
/**
* If the specified field is optional, it is set to None. Otherwise, if the field is
* known, it is reverted to its default value; if the field is unknown, it is removed
* from the passthroughFields map, if present.
*/
def unsetQueryInfo: ScrayTQuery = unsetField(1)
def unsetValues: ScrayTQuery = unsetField(2)
def unsetQueryExpression: ScrayTQuery = unsetField(3)
override def write(_oprot: TProtocol): Unit = {
ScrayTQuery.validate(this)
_oprot.writeStructBegin(Struct)
if (queryInfo ne null) writeQueryInfoField(queryInfo, _oprot)
if (values ne null) writeValuesField(values, _oprot)
if (queryExpression ne null) writeQueryExpressionField(queryExpression, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
queryInfo: scray.service.qmodel.thrifscala.ScrayTQueryInfo = this.queryInfo,
values: Map[String, ByteBuffer] = this.values,
queryExpression: String = this.queryExpression,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): ScrayTQuery =
new Immutable(
queryInfo,
values,
queryExpression,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[ScrayTQuery]
private def _equals(x: ScrayTQuery, y: ScrayTQuery): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[ScrayTQuery]) &&
_passthroughFields == other.asInstanceOf[ScrayTQuery]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 3
override def productElement(n: Int): Any = n match {
case 0 => this.queryInfo
case 1 => this.values
case 2 => this.queryExpression
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "ScrayTQuery"
def _codec: ThriftStructCodec3[ScrayTQuery] = ScrayTQuery
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy