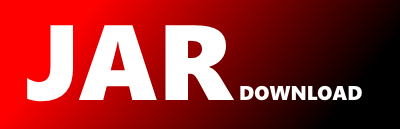
scray.service.qservice.thrifscala.ScrayCombinedStatefulTService.scala Maven / Gradle / Ivy
/**
* Generated by Scrooge
* version: 4.16.0
* rev: 0201cac9fdd6188248d42da91fd14c87744cc4a5
* built at: 20170421-124523
*/
package scray.service.qservice.thrifscala
import com.twitter.scrooge.{
LazyTProtocol,
HasThriftStructCodec3,
TFieldBlob,
ThriftService,
ThriftStruct,
ThriftStructCodec,
ThriftStructCodec3,
ThriftStructFieldInfo,
ThriftResponse,
ThriftUtil,
ToThriftService
}
import com.twitter.finagle.{service => ctfs}
import com.twitter.finagle.thrift.{Protocols, ThriftClientRequest, ThriftServiceIface}
import com.twitter.util.Future
import java.nio.ByteBuffer
import java.util.Arrays
import org.apache.thrift.protocol._
import org.apache.thrift.transport.TTransport
import org.apache.thrift.TApplicationException
import org.apache.thrift.transport.TMemoryBuffer
import scala.collection.immutable.{Map => immutable$Map}
import scala.collection.mutable.{
Builder,
ArrayBuffer => mutable$ArrayBuffer, Buffer => mutable$Buffer,
HashMap => mutable$HashMap, HashSet => mutable$HashSet}
import scala.collection.{Map, Set}
import scala.language.higherKinds
/**
* Combined Scray service
* Combines ScrayMetaTService operations with ScrayStatefulTService operations
* The combined service is thus provided on a single endpoint (port)
*/
@javax.annotation.Generated(value = Array("com.twitter.scrooge.Compiler"))
trait ScrayCombinedStatefulTService[+MM[_]] extends ThriftService {
/**
* Fetch a list of service endpoints.
* Each endpoint provides ScrayStatelessTService and ScrayStatefulTService alternatives.
* Queries can address different endpoints for load distribution.
*/
def getServiceEndpoints(): MM[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]
/**
* Add new service endpoint.
* The endpoint will be removed after a default expiration period.
*/
def addServiceEndpoint(endpoint: scray.service.qservice.thrifscala.ScrayTServiceEndpoint): MM[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]
/**
* Restore the default expiration period of an endpoint.
*/
def refreshServiceEndpoint(endpointID: scray.service.qmodel.thrifscala.ScrayUUID): MM[Unit]
/**
* Return vital sign
*/
def ping(): MM[Boolean]
/**
* Shutdown the server
*/
def shutdown(waitNanos: Option[Long] = None): MM[Unit]
/**
* Submit query
*/
def query(query: scray.service.qmodel.thrifscala.ScrayTQuery): MM[scray.service.qmodel.thrifscala.ScrayUUID]
/**
* Fetch query results
* Paging state is maintained on server side.
* The operation is neither idempotent nor safe.
*/
def getResults(queryId: scray.service.qmodel.thrifscala.ScrayUUID): MM[scray.service.qservice.thrifscala.ScrayTResultFrame]
}
/**
* Combined Scray service
* Combines ScrayMetaTService operations with ScrayStatefulTService operations
* The combined service is thus provided on a single endpoint (port)
*/
object ScrayCombinedStatefulTService { self =>
val annotations: immutable$Map[String, String] = immutable$Map.empty
trait BaseServiceIface extends ToThriftService {
def getServiceEndpoints : com.twitter.finagle.Service[self.GetServiceEndpoints.Args, self.GetServiceEndpoints.SuccessType]
def addServiceEndpoint : com.twitter.finagle.Service[self.AddServiceEndpoint.Args, self.AddServiceEndpoint.SuccessType]
def refreshServiceEndpoint : com.twitter.finagle.Service[self.RefreshServiceEndpoint.Args, self.RefreshServiceEndpoint.SuccessType]
def ping : com.twitter.finagle.Service[self.Ping.Args, self.Ping.SuccessType]
def shutdown : com.twitter.finagle.Service[self.Shutdown.Args, self.Shutdown.SuccessType]
def query : com.twitter.finagle.Service[self.Query.Args, self.Query.SuccessType]
def getResults : com.twitter.finagle.Service[self.GetResults.Args, self.GetResults.SuccessType]
def toThriftService: ThriftService = new MethodIface(this)
}
case class ServiceIface(
getServiceEndpoints : com.twitter.finagle.Service[self.GetServiceEndpoints.Args, self.GetServiceEndpoints.SuccessType],
addServiceEndpoint : com.twitter.finagle.Service[self.AddServiceEndpoint.Args, self.AddServiceEndpoint.SuccessType],
refreshServiceEndpoint : com.twitter.finagle.Service[self.RefreshServiceEndpoint.Args, self.RefreshServiceEndpoint.SuccessType],
ping : com.twitter.finagle.Service[self.Ping.Args, self.Ping.SuccessType],
shutdown : com.twitter.finagle.Service[self.Shutdown.Args, self.Shutdown.SuccessType],
query : com.twitter.finagle.Service[self.Query.Args, self.Query.SuccessType],
getResults : com.twitter.finagle.Service[self.GetResults.Args, self.GetResults.SuccessType]
) extends BaseServiceIface
with com.twitter.finagle.thrift.ThriftServiceIface.Filterable[ServiceIface] {
/**
* Prepends the given type-agnostic `Filter` to all of the `Services`
* and returns a copy of the `ServiceIface` now including the filter.
*/
def filtered(filter: com.twitter.finagle.Filter.TypeAgnostic): ServiceIface =
copy(
getServiceEndpoints = filter.toFilter.andThen(getServiceEndpoints),
addServiceEndpoint = filter.toFilter.andThen(addServiceEndpoint),
refreshServiceEndpoint = filter.toFilter.andThen(refreshServiceEndpoint),
ping = filter.toFilter.andThen(ping),
shutdown = filter.toFilter.andThen(shutdown),
query = filter.toFilter.andThen(query),
getResults = filter.toFilter.andThen(getResults)
)
}
implicit object ServiceIfaceBuilder
extends com.twitter.finagle.thrift.ServiceIfaceBuilder[ServiceIface] {
def newServiceIface(
binaryService: com.twitter.finagle.Service[ThriftClientRequest, Array[Byte]],
pf: TProtocolFactory = com.twitter.finagle.thrift.Protocols.binaryFactory(),
stats: com.twitter.finagle.stats.StatsReceiver
): ServiceIface =
ServiceIface(
getServiceEndpoints = ThriftServiceIface(self.GetServiceEndpoints, binaryService, pf, stats),
addServiceEndpoint = ThriftServiceIface(self.AddServiceEndpoint, binaryService, pf, stats),
refreshServiceEndpoint = ThriftServiceIface(self.RefreshServiceEndpoint, binaryService, pf, stats),
ping = ThriftServiceIface(self.Ping, binaryService, pf, stats),
shutdown = ThriftServiceIface(self.Shutdown, binaryService, pf, stats),
query = ThriftServiceIface(self.Query, binaryService, pf, stats),
getResults = ThriftServiceIface(self.GetResults, binaryService, pf, stats)
)
}
class MethodIface(serviceIface: BaseServiceIface)
extends ScrayCombinedStatefulTService[Future] {
def getServiceEndpoints(): Future[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] =
serviceIface.getServiceEndpoints(self.GetServiceEndpoints.Args())
def addServiceEndpoint(endpoint: scray.service.qservice.thrifscala.ScrayTServiceEndpoint): Future[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] =
serviceIface.addServiceEndpoint(self.AddServiceEndpoint.Args(endpoint))
def refreshServiceEndpoint(endpointID: scray.service.qmodel.thrifscala.ScrayUUID): Future[Unit] =
serviceIface.refreshServiceEndpoint(self.RefreshServiceEndpoint.Args(endpointID)).unit
def ping(): Future[Boolean] =
serviceIface.ping(self.Ping.Args())
def shutdown(waitNanos: Option[Long] = None): Future[Unit] =
serviceIface.shutdown(self.Shutdown.Args(waitNanos)).unit
def query(query: scray.service.qmodel.thrifscala.ScrayTQuery): Future[scray.service.qmodel.thrifscala.ScrayUUID] =
serviceIface.query(self.Query.Args(query))
def getResults(queryId: scray.service.qmodel.thrifscala.ScrayUUID): Future[scray.service.qservice.thrifscala.ScrayTResultFrame] =
serviceIface.getResults(self.GetResults.Args(queryId))
}
implicit object MethodIfaceBuilder
extends com.twitter.finagle.thrift.MethodIfaceBuilder[ServiceIface, ScrayCombinedStatefulTService[Future]] {
def newMethodIface(serviceIface: ServiceIface): MethodIface =
new MethodIface(serviceIface)
}
object GetServiceEndpoints extends com.twitter.scrooge.ThriftMethod {
object Args extends ThriftStructCodec3[Args] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("getServiceEndpoints_args")
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Args): Unit = {
}
def withoutPassthroughFields(original: Args): Args =
new Args(
)
override def encode(_item: Args, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Args = {
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Args(
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
): Args =
new Args(
)
def unapply(_item: Args): Boolean = true
}
class Args(
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftStruct
with _root_.scala.Product
with HasThriftStructCodec3[Args]
with java.io.Serializable
{
import Args._
def this(
) = this(
Map.empty
)
override def write(_oprot: TProtocol): Unit = {
Args.validate(this)
_oprot.writeStructBegin(Struct)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Args =
new Args(
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Args]
private def _equals(x: Args, y: Args): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Args]) &&
_passthroughFields == other.asInstanceOf[Args]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 0
override def productElement(n: Int): Any = n match {
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Args"
def _codec: ThriftStructCodec3[Args] = Args
}
type SuccessType = Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]
object Result extends ThriftStructCodec3[Result] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("getServiceEndpoints_result")
val SuccessField = new TField("success", TType.LIST, 0)
val SuccessFieldManifest = implicitly[Manifest[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
SuccessField,
true,
false,
SuccessFieldManifest,
_root_.scala.None,
_root_.scala.Some(implicitly[Manifest[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]),
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Result): Unit = {
}
def withoutPassthroughFields(original: Result): Result =
new Result(
success =
{
val field = original.success
field.map { field =>
field.map { field =>
scray.service.qservice.thrifscala.ScrayTServiceEndpoint.withoutPassthroughFields(field)
}
}
}
)
override def encode(_item: Result, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Result = {
var success: _root_.scala.Option[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] = _root_.scala.None
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 0 =>
_field.`type` match {
case TType.LIST =>
success = _root_.scala.Some(readSuccessValue(_iprot))
case _actualType =>
val _expectedType = TType.LIST
throw new TProtocolException(
"Received wrong type for field 'success' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Result(
success,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
success: _root_.scala.Option[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] = _root_.scala.None
): Result =
new Result(
success
)
def unapply(_item: Result): _root_.scala.Option[_root_.scala.Option[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]] = _root_.scala.Some(_item.success)
@inline private def readSuccessValue(_iprot: TProtocol): Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] = {
val _list = _iprot.readListBegin()
if (_list.size == 0) {
_iprot.readListEnd()
Nil
} else {
val _rv = new mutable$ArrayBuffer[scray.service.qservice.thrifscala.ScrayTServiceEndpoint](_list.size)
var _i = 0
while (_i < _list.size) {
_rv += {
scray.service.qservice.thrifscala.ScrayTServiceEndpoint.decode(_iprot)
}
_i += 1
}
_iprot.readListEnd()
_rv
}
}
@inline private def writeSuccessField(success_item: Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint], _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(SuccessField)
writeSuccessValue(success_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeSuccessValue(success_item: Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint], _oprot: TProtocol): Unit = {
_oprot.writeListBegin(new TList(TType.STRUCT, success_item.size))
success_item match {
case _: IndexedSeq[_] =>
var _i = 0
val _size = success_item.size
while (_i < _size) {
val success_item_element = success_item(_i)
success_item_element.write(_oprot)
_i += 1
}
case _ =>
success_item.foreach { success_item_element =>
success_item_element.write(_oprot)
}
}
_oprot.writeListEnd()
}
}
class Result(
val success: _root_.scala.Option[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]],
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftResponse[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] with ThriftStruct
with _root_.scala.Product1[Option[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]]
with HasThriftStructCodec3[Result]
with java.io.Serializable
{
import Result._
def this(
success: _root_.scala.Option[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] = _root_.scala.None
) = this(
success,
Map.empty
)
def _1 = success
def successField: Option[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] = success
def exceptionFields: Iterable[Option[com.twitter.scrooge.ThriftException]] = Seq()
override def write(_oprot: TProtocol): Unit = {
Result.validate(this)
_oprot.writeStructBegin(Struct)
if (success.isDefined) writeSuccessField(success.get, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
success: _root_.scala.Option[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] = this.success,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Result =
new Result(
success,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Result]
private def _equals(x: Result, y: Result): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Result]) &&
_passthroughFields == other.asInstanceOf[Result]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 1
override def productElement(n: Int): Any = n match {
case 0 => this.success
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Result"
def _codec: ThriftStructCodec3[Result] = Result
}
val annotations: immutable$Map[String, String] = immutable$Map.empty
type FunctionType = Function1[Args,Future[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]]
type ServiceType = com.twitter.finagle.Service[Args, Result]
type ServiceIfaceServiceType = com.twitter.finagle.Service[Args, SuccessType]
def toServiceIfaceService(f: FunctionType): ServiceIfaceServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args)
}
private[this] val toResult = (res: SuccessType) => Result(Some(res))
def functionToService(f: FunctionType): ServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args).map(toResult)
}
def serviceToFunction(svc: ServiceType): FunctionType = { args: Args =>
com.twitter.finagle.thrift.ThriftServiceIface.resultFilter(this).andThen(svc).apply(args)
}
val name: String = "getServiceEndpoints"
val serviceName: String = "ScrayCombinedStatefulTService"
val argsCodec = Args
val responseCodec = Result
val oneway: Boolean = false
}
// Compatibility aliases.
val getServiceEndpoints$args = GetServiceEndpoints.Args
type getServiceEndpoints$args = GetServiceEndpoints.Args
val getServiceEndpoints$result = GetServiceEndpoints.Result
type getServiceEndpoints$result = GetServiceEndpoints.Result
object AddServiceEndpoint extends com.twitter.scrooge.ThriftMethod {
object Args extends ThriftStructCodec3[Args] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("addServiceEndpoint_args")
val EndpointField = new TField("endpoint", TType.STRUCT, -1)
val EndpointFieldManifest = implicitly[Manifest[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
EndpointField,
false,
false,
EndpointFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Args): Unit = {
}
def withoutPassthroughFields(original: Args): Args =
new Args(
endpoint =
{
val field = original.endpoint
scray.service.qservice.thrifscala.ScrayTServiceEndpoint.withoutPassthroughFields(field)
}
)
override def encode(_item: Args, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Args = {
var endpoint: scray.service.qservice.thrifscala.ScrayTServiceEndpoint = null
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case -1 =>
_field.`type` match {
case TType.STRUCT =>
endpoint = readEndpointValue(_iprot)
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'endpoint' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Args(
endpoint,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
endpoint: scray.service.qservice.thrifscala.ScrayTServiceEndpoint
): Args =
new Args(
endpoint
)
def unapply(_item: Args): _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] = _root_.scala.Some(_item.endpoint)
@inline private def readEndpointValue(_iprot: TProtocol): scray.service.qservice.thrifscala.ScrayTServiceEndpoint = {
scray.service.qservice.thrifscala.ScrayTServiceEndpoint.decode(_iprot)
}
@inline private def writeEndpointField(endpoint_item: scray.service.qservice.thrifscala.ScrayTServiceEndpoint, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(EndpointField)
writeEndpointValue(endpoint_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeEndpointValue(endpoint_item: scray.service.qservice.thrifscala.ScrayTServiceEndpoint, _oprot: TProtocol): Unit = {
endpoint_item.write(_oprot)
}
}
class Args(
val endpoint: scray.service.qservice.thrifscala.ScrayTServiceEndpoint,
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftStruct
with _root_.scala.Product1[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]
with HasThriftStructCodec3[Args]
with java.io.Serializable
{
import Args._
def this(
endpoint: scray.service.qservice.thrifscala.ScrayTServiceEndpoint
) = this(
endpoint,
Map.empty
)
def _1 = endpoint
override def write(_oprot: TProtocol): Unit = {
Args.validate(this)
_oprot.writeStructBegin(Struct)
if (endpoint ne null) writeEndpointField(endpoint, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
endpoint: scray.service.qservice.thrifscala.ScrayTServiceEndpoint = this.endpoint,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Args =
new Args(
endpoint,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Args]
private def _equals(x: Args, y: Args): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Args]) &&
_passthroughFields == other.asInstanceOf[Args]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 1
override def productElement(n: Int): Any = n match {
case 0 => this.endpoint
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Args"
def _codec: ThriftStructCodec3[Args] = Args
}
type SuccessType = scray.service.qservice.thrifscala.ScrayTServiceEndpoint
object Result extends ThriftStructCodec3[Result] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("addServiceEndpoint_result")
val SuccessField = new TField("success", TType.STRUCT, 0)
val SuccessFieldManifest = implicitly[Manifest[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
SuccessField,
true,
false,
SuccessFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Result): Unit = {
}
def withoutPassthroughFields(original: Result): Result =
new Result(
success =
{
val field = original.success
field.map { field =>
scray.service.qservice.thrifscala.ScrayTServiceEndpoint.withoutPassthroughFields(field)
}
}
)
override def encode(_item: Result, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Result = {
var success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] = _root_.scala.None
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 0 =>
_field.`type` match {
case TType.STRUCT =>
success = _root_.scala.Some(readSuccessValue(_iprot))
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'success' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Result(
success,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] = _root_.scala.None
): Result =
new Result(
success
)
def unapply(_item: Result): _root_.scala.Option[_root_.scala.Option[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] = _root_.scala.Some(_item.success)
@inline private def readSuccessValue(_iprot: TProtocol): scray.service.qservice.thrifscala.ScrayTServiceEndpoint = {
scray.service.qservice.thrifscala.ScrayTServiceEndpoint.decode(_iprot)
}
@inline private def writeSuccessField(success_item: scray.service.qservice.thrifscala.ScrayTServiceEndpoint, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(SuccessField)
writeSuccessValue(success_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeSuccessValue(success_item: scray.service.qservice.thrifscala.ScrayTServiceEndpoint, _oprot: TProtocol): Unit = {
success_item.write(_oprot)
}
}
class Result(
val success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTServiceEndpoint],
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftResponse[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] with ThriftStruct
with _root_.scala.Product1[Option[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]
with HasThriftStructCodec3[Result]
with java.io.Serializable
{
import Result._
def this(
success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] = _root_.scala.None
) = this(
success,
Map.empty
)
def _1 = success
def successField: Option[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] = success
def exceptionFields: Iterable[Option[com.twitter.scrooge.ThriftException]] = Seq()
override def write(_oprot: TProtocol): Unit = {
Result.validate(this)
_oprot.writeStructBegin(Struct)
if (success.isDefined) writeSuccessField(success.get, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] = this.success,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Result =
new Result(
success,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Result]
private def _equals(x: Result, y: Result): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Result]) &&
_passthroughFields == other.asInstanceOf[Result]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 1
override def productElement(n: Int): Any = n match {
case 0 => this.success
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Result"
def _codec: ThriftStructCodec3[Result] = Result
}
val annotations: immutable$Map[String, String] = immutable$Map.empty
type FunctionType = Function1[Args,Future[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]
type ServiceType = com.twitter.finagle.Service[Args, Result]
type ServiceIfaceServiceType = com.twitter.finagle.Service[Args, SuccessType]
def toServiceIfaceService(f: FunctionType): ServiceIfaceServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args)
}
private[this] val toResult = (res: SuccessType) => Result(Some(res))
def functionToService(f: FunctionType): ServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args).map(toResult)
}
def serviceToFunction(svc: ServiceType): FunctionType = { args: Args =>
com.twitter.finagle.thrift.ThriftServiceIface.resultFilter(this).andThen(svc).apply(args)
}
val name: String = "addServiceEndpoint"
val serviceName: String = "ScrayCombinedStatefulTService"
val argsCodec = Args
val responseCodec = Result
val oneway: Boolean = false
}
// Compatibility aliases.
val addServiceEndpoint$args = AddServiceEndpoint.Args
type addServiceEndpoint$args = AddServiceEndpoint.Args
val addServiceEndpoint$result = AddServiceEndpoint.Result
type addServiceEndpoint$result = AddServiceEndpoint.Result
object RefreshServiceEndpoint extends com.twitter.scrooge.ThriftMethod {
object Args extends ThriftStructCodec3[Args] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("refreshServiceEndpoint_args")
val EndpointIDField = new TField("endpointID", TType.STRUCT, -1)
val EndpointIDFieldManifest = implicitly[Manifest[scray.service.qmodel.thrifscala.ScrayUUID]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
EndpointIDField,
false,
false,
EndpointIDFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Args): Unit = {
}
def withoutPassthroughFields(original: Args): Args =
new Args(
endpointID =
{
val field = original.endpointID
scray.service.qmodel.thrifscala.ScrayUUID.withoutPassthroughFields(field)
}
)
override def encode(_item: Args, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Args = {
var endpointID: scray.service.qmodel.thrifscala.ScrayUUID = null
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case -1 =>
_field.`type` match {
case TType.STRUCT =>
endpointID = readEndpointIDValue(_iprot)
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'endpointID' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Args(
endpointID,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
endpointID: scray.service.qmodel.thrifscala.ScrayUUID
): Args =
new Args(
endpointID
)
def unapply(_item: Args): _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayUUID] = _root_.scala.Some(_item.endpointID)
@inline private def readEndpointIDValue(_iprot: TProtocol): scray.service.qmodel.thrifscala.ScrayUUID = {
scray.service.qmodel.thrifscala.ScrayUUID.decode(_iprot)
}
@inline private def writeEndpointIDField(endpointID_item: scray.service.qmodel.thrifscala.ScrayUUID, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(EndpointIDField)
writeEndpointIDValue(endpointID_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeEndpointIDValue(endpointID_item: scray.service.qmodel.thrifscala.ScrayUUID, _oprot: TProtocol): Unit = {
endpointID_item.write(_oprot)
}
}
class Args(
val endpointID: scray.service.qmodel.thrifscala.ScrayUUID,
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftStruct
with _root_.scala.Product1[scray.service.qmodel.thrifscala.ScrayUUID]
with HasThriftStructCodec3[Args]
with java.io.Serializable
{
import Args._
def this(
endpointID: scray.service.qmodel.thrifscala.ScrayUUID
) = this(
endpointID,
Map.empty
)
def _1 = endpointID
override def write(_oprot: TProtocol): Unit = {
Args.validate(this)
_oprot.writeStructBegin(Struct)
if (endpointID ne null) writeEndpointIDField(endpointID, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
endpointID: scray.service.qmodel.thrifscala.ScrayUUID = this.endpointID,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Args =
new Args(
endpointID,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Args]
private def _equals(x: Args, y: Args): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Args]) &&
_passthroughFields == other.asInstanceOf[Args]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 1
override def productElement(n: Int): Any = n match {
case 0 => this.endpointID
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Args"
def _codec: ThriftStructCodec3[Args] = Args
}
type SuccessType = Unit
object Result extends ThriftStructCodec3[Result] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("refreshServiceEndpoint_result")
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Result): Unit = {
}
def withoutPassthroughFields(original: Result): Result =
new Result(
)
override def encode(_item: Result, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Result = {
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Result(
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
): Result =
new Result(
)
def unapply(_item: Result): Boolean = true
}
class Result(
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftResponse[Unit] with ThriftStruct
with _root_.scala.Product
with HasThriftStructCodec3[Result]
with java.io.Serializable
{
import Result._
def this(
) = this(
Map.empty
)
def successField: Option[Unit] = Some(Unit)
def exceptionFields: Iterable[Option[com.twitter.scrooge.ThriftException]] = Seq()
override def write(_oprot: TProtocol): Unit = {
Result.validate(this)
_oprot.writeStructBegin(Struct)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Result =
new Result(
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Result]
private def _equals(x: Result, y: Result): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Result]) &&
_passthroughFields == other.asInstanceOf[Result]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 0
override def productElement(n: Int): Any = n match {
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Result"
def _codec: ThriftStructCodec3[Result] = Result
}
val annotations: immutable$Map[String, String] = immutable$Map.empty
type FunctionType = Function1[Args,Future[Unit]]
type ServiceType = com.twitter.finagle.Service[Args, Result]
type ServiceIfaceServiceType = com.twitter.finagle.Service[Args, SuccessType]
def toServiceIfaceService(f: FunctionType): ServiceIfaceServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args)
}
private[this] val toResult = (res: SuccessType) => Result()
def functionToService(f: FunctionType): ServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args).map(toResult)
}
def serviceToFunction(svc: ServiceType): FunctionType = { args: Args =>
com.twitter.finagle.thrift.ThriftServiceIface.resultFilter(this).andThen(svc).apply(args)
}
val name: String = "refreshServiceEndpoint"
val serviceName: String = "ScrayCombinedStatefulTService"
val argsCodec = Args
val responseCodec = Result
val oneway: Boolean = false
}
// Compatibility aliases.
val refreshServiceEndpoint$args = RefreshServiceEndpoint.Args
type refreshServiceEndpoint$args = RefreshServiceEndpoint.Args
val refreshServiceEndpoint$result = RefreshServiceEndpoint.Result
type refreshServiceEndpoint$result = RefreshServiceEndpoint.Result
object Ping extends com.twitter.scrooge.ThriftMethod {
object Args extends ThriftStructCodec3[Args] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("ping_args")
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Args): Unit = {
}
def withoutPassthroughFields(original: Args): Args =
new Args(
)
override def encode(_item: Args, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Args = {
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Args(
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
): Args =
new Args(
)
def unapply(_item: Args): Boolean = true
}
class Args(
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftStruct
with _root_.scala.Product
with HasThriftStructCodec3[Args]
with java.io.Serializable
{
import Args._
def this(
) = this(
Map.empty
)
override def write(_oprot: TProtocol): Unit = {
Args.validate(this)
_oprot.writeStructBegin(Struct)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Args =
new Args(
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Args]
private def _equals(x: Args, y: Args): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Args]) &&
_passthroughFields == other.asInstanceOf[Args]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 0
override def productElement(n: Int): Any = n match {
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Args"
def _codec: ThriftStructCodec3[Args] = Args
}
type SuccessType = Boolean
object Result extends ThriftStructCodec3[Result] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("ping_result")
val SuccessField = new TField("success", TType.BOOL, 0)
val SuccessFieldManifest = implicitly[Manifest[Boolean]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
SuccessField,
true,
false,
SuccessFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Result): Unit = {
}
def withoutPassthroughFields(original: Result): Result =
new Result(
success =
{
val field = original.success
field.map { field =>
field
}
}
)
override def encode(_item: Result, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Result = {
var success: _root_.scala.Option[Boolean] = _root_.scala.None
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 0 =>
_field.`type` match {
case TType.BOOL =>
success = _root_.scala.Some(readSuccessValue(_iprot))
case _actualType =>
val _expectedType = TType.BOOL
throw new TProtocolException(
"Received wrong type for field 'success' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Result(
success,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
success: _root_.scala.Option[Boolean] = _root_.scala.None
): Result =
new Result(
success
)
def unapply(_item: Result): _root_.scala.Option[_root_.scala.Option[Boolean]] = _root_.scala.Some(_item.success)
@inline private def readSuccessValue(_iprot: TProtocol): Boolean = {
_iprot.readBool()
}
@inline private def writeSuccessField(success_item: Boolean, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(SuccessField)
writeSuccessValue(success_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeSuccessValue(success_item: Boolean, _oprot: TProtocol): Unit = {
_oprot.writeBool(success_item)
}
}
class Result(
val success: _root_.scala.Option[Boolean],
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftResponse[Boolean] with ThriftStruct
with _root_.scala.Product1[Option[Boolean]]
with HasThriftStructCodec3[Result]
with java.io.Serializable
{
import Result._
def this(
success: _root_.scala.Option[Boolean] = _root_.scala.None
) = this(
success,
Map.empty
)
def _1 = success
def successField: Option[Boolean] = success
def exceptionFields: Iterable[Option[com.twitter.scrooge.ThriftException]] = Seq()
override def write(_oprot: TProtocol): Unit = {
Result.validate(this)
_oprot.writeStructBegin(Struct)
if (success.isDefined) writeSuccessField(success.get, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
success: _root_.scala.Option[Boolean] = this.success,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Result =
new Result(
success,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Result]
private def _equals(x: Result, y: Result): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Result]) &&
_passthroughFields == other.asInstanceOf[Result]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 1
override def productElement(n: Int): Any = n match {
case 0 => this.success
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Result"
def _codec: ThriftStructCodec3[Result] = Result
}
val annotations: immutable$Map[String, String] = immutable$Map.empty
type FunctionType = Function1[Args,Future[Boolean]]
type ServiceType = com.twitter.finagle.Service[Args, Result]
type ServiceIfaceServiceType = com.twitter.finagle.Service[Args, SuccessType]
def toServiceIfaceService(f: FunctionType): ServiceIfaceServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args)
}
private[this] val toResult = (res: SuccessType) => Result(Some(res))
def functionToService(f: FunctionType): ServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args).map(toResult)
}
def serviceToFunction(svc: ServiceType): FunctionType = { args: Args =>
com.twitter.finagle.thrift.ThriftServiceIface.resultFilter(this).andThen(svc).apply(args)
}
val name: String = "ping"
val serviceName: String = "ScrayCombinedStatefulTService"
val argsCodec = Args
val responseCodec = Result
val oneway: Boolean = false
}
// Compatibility aliases.
val ping$args = Ping.Args
type ping$args = Ping.Args
val ping$result = Ping.Result
type ping$result = Ping.Result
object Shutdown extends com.twitter.scrooge.ThriftMethod {
object Args extends ThriftStructCodec3[Args] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("shutdown_args")
val WaitNanosField = new TField("waitNanos", TType.I64, -1)
val WaitNanosFieldManifest = implicitly[Manifest[Long]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
WaitNanosField,
true,
false,
WaitNanosFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Args): Unit = {
}
def withoutPassthroughFields(original: Args): Args =
new Args(
waitNanos =
{
val field = original.waitNanos
field.map { field =>
field
}
}
)
override def encode(_item: Args, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Args = {
var waitNanos: _root_.scala.Option[Long] = _root_.scala.None
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case -1 =>
_field.`type` match {
case TType.I64 =>
waitNanos = _root_.scala.Some(readWaitNanosValue(_iprot))
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'waitNanos' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Args(
waitNanos,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
waitNanos: _root_.scala.Option[Long] = _root_.scala.None
): Args =
new Args(
waitNanos
)
def unapply(_item: Args): _root_.scala.Option[_root_.scala.Option[Long]] = _root_.scala.Some(_item.waitNanos)
@inline private def readWaitNanosValue(_iprot: TProtocol): Long = {
_iprot.readI64()
}
@inline private def writeWaitNanosField(waitNanos_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(WaitNanosField)
writeWaitNanosValue(waitNanos_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeWaitNanosValue(waitNanos_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeI64(waitNanos_item)
}
}
class Args(
val waitNanos: _root_.scala.Option[Long],
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftStruct
with _root_.scala.Product1[Option[Long]]
with HasThriftStructCodec3[Args]
with java.io.Serializable
{
import Args._
def this(
waitNanos: _root_.scala.Option[Long] = _root_.scala.None
) = this(
waitNanos,
Map.empty
)
def _1 = waitNanos
override def write(_oprot: TProtocol): Unit = {
Args.validate(this)
_oprot.writeStructBegin(Struct)
if (waitNanos.isDefined) writeWaitNanosField(waitNanos.get, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
waitNanos: _root_.scala.Option[Long] = this.waitNanos,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Args =
new Args(
waitNanos,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Args]
private def _equals(x: Args, y: Args): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Args]) &&
_passthroughFields == other.asInstanceOf[Args]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 1
override def productElement(n: Int): Any = n match {
case 0 => this.waitNanos
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Args"
def _codec: ThriftStructCodec3[Args] = Args
}
type SuccessType = Unit
object Result extends ThriftStructCodec3[Result] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("shutdown_result")
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Result): Unit = {
}
def withoutPassthroughFields(original: Result): Result =
new Result(
)
override def encode(_item: Result, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Result = {
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Result(
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
): Result =
new Result(
)
def unapply(_item: Result): Boolean = true
}
class Result(
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftResponse[Unit] with ThriftStruct
with _root_.scala.Product
with HasThriftStructCodec3[Result]
with java.io.Serializable
{
import Result._
def this(
) = this(
Map.empty
)
def successField: Option[Unit] = Some(Unit)
def exceptionFields: Iterable[Option[com.twitter.scrooge.ThriftException]] = Seq()
override def write(_oprot: TProtocol): Unit = {
Result.validate(this)
_oprot.writeStructBegin(Struct)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Result =
new Result(
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Result]
private def _equals(x: Result, y: Result): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Result]) &&
_passthroughFields == other.asInstanceOf[Result]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 0
override def productElement(n: Int): Any = n match {
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Result"
def _codec: ThriftStructCodec3[Result] = Result
}
val annotations: immutable$Map[String, String] = immutable$Map.empty
type FunctionType = Function1[Args,Future[Unit]]
type ServiceType = com.twitter.finagle.Service[Args, Result]
type ServiceIfaceServiceType = com.twitter.finagle.Service[Args, SuccessType]
def toServiceIfaceService(f: FunctionType): ServiceIfaceServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args)
}
private[this] val toResult = (res: SuccessType) => Result()
def functionToService(f: FunctionType): ServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args).map(toResult)
}
def serviceToFunction(svc: ServiceType): FunctionType = { args: Args =>
com.twitter.finagle.thrift.ThriftServiceIface.resultFilter(this).andThen(svc).apply(args)
}
val name: String = "shutdown"
val serviceName: String = "ScrayCombinedStatefulTService"
val argsCodec = Args
val responseCodec = Result
val oneway: Boolean = false
}
// Compatibility aliases.
val shutdown$args = Shutdown.Args
type shutdown$args = Shutdown.Args
val shutdown$result = Shutdown.Result
type shutdown$result = Shutdown.Result
object Query extends com.twitter.scrooge.ThriftMethod {
object Args extends ThriftStructCodec3[Args] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("query_args")
val QueryField = new TField("query", TType.STRUCT, 1)
val QueryFieldManifest = implicitly[Manifest[scray.service.qmodel.thrifscala.ScrayTQuery]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
QueryField,
false,
false,
QueryFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Args): Unit = {
}
def withoutPassthroughFields(original: Args): Args =
new Args(
query =
{
val field = original.query
scray.service.qmodel.thrifscala.ScrayTQuery.withoutPassthroughFields(field)
}
)
override def encode(_item: Args, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Args = {
var query: scray.service.qmodel.thrifscala.ScrayTQuery = null
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 1 =>
_field.`type` match {
case TType.STRUCT =>
query = readQueryValue(_iprot)
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'query' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Args(
query,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
query: scray.service.qmodel.thrifscala.ScrayTQuery
): Args =
new Args(
query
)
def unapply(_item: Args): _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayTQuery] = _root_.scala.Some(_item.query)
@inline private def readQueryValue(_iprot: TProtocol): scray.service.qmodel.thrifscala.ScrayTQuery = {
scray.service.qmodel.thrifscala.ScrayTQuery.decode(_iprot)
}
@inline private def writeQueryField(query_item: scray.service.qmodel.thrifscala.ScrayTQuery, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(QueryField)
writeQueryValue(query_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeQueryValue(query_item: scray.service.qmodel.thrifscala.ScrayTQuery, _oprot: TProtocol): Unit = {
query_item.write(_oprot)
}
}
class Args(
val query: scray.service.qmodel.thrifscala.ScrayTQuery,
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftStruct
with _root_.scala.Product1[scray.service.qmodel.thrifscala.ScrayTQuery]
with HasThriftStructCodec3[Args]
with java.io.Serializable
{
import Args._
def this(
query: scray.service.qmodel.thrifscala.ScrayTQuery
) = this(
query,
Map.empty
)
def _1 = query
override def write(_oprot: TProtocol): Unit = {
Args.validate(this)
_oprot.writeStructBegin(Struct)
if (query ne null) writeQueryField(query, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
query: scray.service.qmodel.thrifscala.ScrayTQuery = this.query,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Args =
new Args(
query,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Args]
private def _equals(x: Args, y: Args): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Args]) &&
_passthroughFields == other.asInstanceOf[Args]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 1
override def productElement(n: Int): Any = n match {
case 0 => this.query
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Args"
def _codec: ThriftStructCodec3[Args] = Args
}
type SuccessType = scray.service.qmodel.thrifscala.ScrayUUID
object Result extends ThriftStructCodec3[Result] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("query_result")
val SuccessField = new TField("success", TType.STRUCT, 0)
val SuccessFieldManifest = implicitly[Manifest[scray.service.qmodel.thrifscala.ScrayUUID]]
val ExField = new TField("ex", TType.STRUCT, 1)
val ExFieldManifest = implicitly[Manifest[scray.service.qservice.thrifscala.ScrayTException]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
SuccessField,
true,
false,
SuccessFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
),
new ThriftStructFieldInfo(
ExField,
true,
false,
ExFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Result): Unit = {
}
def withoutPassthroughFields(original: Result): Result =
new Result(
success =
{
val field = original.success
field.map { field =>
scray.service.qmodel.thrifscala.ScrayUUID.withoutPassthroughFields(field)
}
},
ex =
{
val field = original.ex
field.map { field =>
scray.service.qservice.thrifscala.ScrayTException.withoutPassthroughFields(field)
}
}
)
override def encode(_item: Result, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Result = {
var success: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayUUID] = _root_.scala.None
var ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException] = _root_.scala.None
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 0 =>
_field.`type` match {
case TType.STRUCT =>
success = _root_.scala.Some(readSuccessValue(_iprot))
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'success' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 1 =>
_field.`type` match {
case TType.STRUCT =>
ex = _root_.scala.Some(readExValue(_iprot))
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'ex' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Result(
success,
ex,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
success: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayUUID] = _root_.scala.None,
ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException] = _root_.scala.None
): Result =
new Result(
success,
ex
)
def unapply(_item: Result): _root_.scala.Option[_root_.scala.Tuple2[Option[scray.service.qmodel.thrifscala.ScrayUUID], Option[scray.service.qservice.thrifscala.ScrayTException]]] = _root_.scala.Some(_item.toTuple)
@inline private def readSuccessValue(_iprot: TProtocol): scray.service.qmodel.thrifscala.ScrayUUID = {
scray.service.qmodel.thrifscala.ScrayUUID.decode(_iprot)
}
@inline private def writeSuccessField(success_item: scray.service.qmodel.thrifscala.ScrayUUID, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(SuccessField)
writeSuccessValue(success_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeSuccessValue(success_item: scray.service.qmodel.thrifscala.ScrayUUID, _oprot: TProtocol): Unit = {
success_item.write(_oprot)
}
@inline private def readExValue(_iprot: TProtocol): scray.service.qservice.thrifscala.ScrayTException = {
scray.service.qservice.thrifscala.ScrayTException.decode(_iprot)
}
@inline private def writeExField(ex_item: scray.service.qservice.thrifscala.ScrayTException, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(ExField)
writeExValue(ex_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeExValue(ex_item: scray.service.qservice.thrifscala.ScrayTException, _oprot: TProtocol): Unit = {
ex_item.write(_oprot)
}
}
class Result(
val success: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayUUID],
val ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException],
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftResponse[scray.service.qmodel.thrifscala.ScrayUUID] with ThriftStruct
with _root_.scala.Product2[Option[scray.service.qmodel.thrifscala.ScrayUUID], Option[scray.service.qservice.thrifscala.ScrayTException]]
with HasThriftStructCodec3[Result]
with java.io.Serializable
{
import Result._
def this(
success: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayUUID] = _root_.scala.None,
ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException] = _root_.scala.None
) = this(
success,
ex,
Map.empty
)
def _1 = success
def _2 = ex
def toTuple: _root_.scala.Tuple2[Option[scray.service.qmodel.thrifscala.ScrayUUID], Option[scray.service.qservice.thrifscala.ScrayTException]] = {
(
success,
ex
)
}
def successField: Option[scray.service.qmodel.thrifscala.ScrayUUID] = success
def exceptionFields: Iterable[Option[com.twitter.scrooge.ThriftException]] = Seq(ex)
override def write(_oprot: TProtocol): Unit = {
Result.validate(this)
_oprot.writeStructBegin(Struct)
if (success.isDefined) writeSuccessField(success.get, _oprot)
if (ex.isDefined) writeExField(ex.get, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
success: _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayUUID] = this.success,
ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException] = this.ex,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Result =
new Result(
success,
ex,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Result]
private def _equals(x: Result, y: Result): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Result]) &&
_passthroughFields == other.asInstanceOf[Result]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 2
override def productElement(n: Int): Any = n match {
case 0 => this.success
case 1 => this.ex
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Result"
def _codec: ThriftStructCodec3[Result] = Result
}
val annotations: immutable$Map[String, String] = immutable$Map.empty
type FunctionType = Function1[Args,Future[scray.service.qmodel.thrifscala.ScrayUUID]]
type ServiceType = com.twitter.finagle.Service[Args, Result]
type ServiceIfaceServiceType = com.twitter.finagle.Service[Args, SuccessType]
def toServiceIfaceService(f: FunctionType): ServiceIfaceServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args)
}
private[this] val toResult = (res: SuccessType) => Result(Some(res))
def functionToService(f: FunctionType): ServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args).map(toResult)
}
def serviceToFunction(svc: ServiceType): FunctionType = { args: Args =>
com.twitter.finagle.thrift.ThriftServiceIface.resultFilter(this).andThen(svc).apply(args)
}
val name: String = "query"
val serviceName: String = "ScrayCombinedStatefulTService"
val argsCodec = Args
val responseCodec = Result
val oneway: Boolean = false
}
// Compatibility aliases.
val query$args = Query.Args
type query$args = Query.Args
val query$result = Query.Result
type query$result = Query.Result
object GetResults extends com.twitter.scrooge.ThriftMethod {
object Args extends ThriftStructCodec3[Args] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("getResults_args")
val QueryIdField = new TField("queryId", TType.STRUCT, 1)
val QueryIdFieldManifest = implicitly[Manifest[scray.service.qmodel.thrifscala.ScrayUUID]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
QueryIdField,
false,
false,
QueryIdFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Args): Unit = {
}
def withoutPassthroughFields(original: Args): Args =
new Args(
queryId =
{
val field = original.queryId
scray.service.qmodel.thrifscala.ScrayUUID.withoutPassthroughFields(field)
}
)
override def encode(_item: Args, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Args = {
var queryId: scray.service.qmodel.thrifscala.ScrayUUID = null
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 1 =>
_field.`type` match {
case TType.STRUCT =>
queryId = readQueryIdValue(_iprot)
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'queryId' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Args(
queryId,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
queryId: scray.service.qmodel.thrifscala.ScrayUUID
): Args =
new Args(
queryId
)
def unapply(_item: Args): _root_.scala.Option[scray.service.qmodel.thrifscala.ScrayUUID] = _root_.scala.Some(_item.queryId)
@inline private def readQueryIdValue(_iprot: TProtocol): scray.service.qmodel.thrifscala.ScrayUUID = {
scray.service.qmodel.thrifscala.ScrayUUID.decode(_iprot)
}
@inline private def writeQueryIdField(queryId_item: scray.service.qmodel.thrifscala.ScrayUUID, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(QueryIdField)
writeQueryIdValue(queryId_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeQueryIdValue(queryId_item: scray.service.qmodel.thrifscala.ScrayUUID, _oprot: TProtocol): Unit = {
queryId_item.write(_oprot)
}
}
class Args(
val queryId: scray.service.qmodel.thrifscala.ScrayUUID,
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftStruct
with _root_.scala.Product1[scray.service.qmodel.thrifscala.ScrayUUID]
with HasThriftStructCodec3[Args]
with java.io.Serializable
{
import Args._
def this(
queryId: scray.service.qmodel.thrifscala.ScrayUUID
) = this(
queryId,
Map.empty
)
def _1 = queryId
override def write(_oprot: TProtocol): Unit = {
Args.validate(this)
_oprot.writeStructBegin(Struct)
if (queryId ne null) writeQueryIdField(queryId, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
queryId: scray.service.qmodel.thrifscala.ScrayUUID = this.queryId,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Args =
new Args(
queryId,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Args]
private def _equals(x: Args, y: Args): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Args]) &&
_passthroughFields == other.asInstanceOf[Args]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 1
override def productElement(n: Int): Any = n match {
case 0 => this.queryId
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Args"
def _codec: ThriftStructCodec3[Args] = Args
}
type SuccessType = scray.service.qservice.thrifscala.ScrayTResultFrame
object Result extends ThriftStructCodec3[Result] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("getResults_result")
val SuccessField = new TField("success", TType.STRUCT, 0)
val SuccessFieldManifest = implicitly[Manifest[scray.service.qservice.thrifscala.ScrayTResultFrame]]
val ExField = new TField("ex", TType.STRUCT, 1)
val ExFieldManifest = implicitly[Manifest[scray.service.qservice.thrifscala.ScrayTException]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
SuccessField,
true,
false,
SuccessFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
),
new ThriftStructFieldInfo(
ExField,
true,
false,
ExFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String],
None
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Result): Unit = {
}
def withoutPassthroughFields(original: Result): Result =
new Result(
success =
{
val field = original.success
field.map { field =>
scray.service.qservice.thrifscala.ScrayTResultFrame.withoutPassthroughFields(field)
}
},
ex =
{
val field = original.ex
field.map { field =>
scray.service.qservice.thrifscala.ScrayTException.withoutPassthroughFields(field)
}
}
)
override def encode(_item: Result, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
override def decode(_iprot: TProtocol): Result = {
var success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTResultFrame] = _root_.scala.None
var ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException] = _root_.scala.None
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 0 =>
_field.`type` match {
case TType.STRUCT =>
success = _root_.scala.Some(readSuccessValue(_iprot))
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'success' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 1 =>
_field.`type` match {
case TType.STRUCT =>
ex = _root_.scala.Some(readExValue(_iprot))
case _actualType =>
val _expectedType = TType.STRUCT
throw new TProtocolException(
"Received wrong type for field 'ex' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Result(
success,
ex,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTResultFrame] = _root_.scala.None,
ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException] = _root_.scala.None
): Result =
new Result(
success,
ex
)
def unapply(_item: Result): _root_.scala.Option[_root_.scala.Tuple2[Option[scray.service.qservice.thrifscala.ScrayTResultFrame], Option[scray.service.qservice.thrifscala.ScrayTException]]] = _root_.scala.Some(_item.toTuple)
@inline private def readSuccessValue(_iprot: TProtocol): scray.service.qservice.thrifscala.ScrayTResultFrame = {
scray.service.qservice.thrifscala.ScrayTResultFrame.decode(_iprot)
}
@inline private def writeSuccessField(success_item: scray.service.qservice.thrifscala.ScrayTResultFrame, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(SuccessField)
writeSuccessValue(success_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeSuccessValue(success_item: scray.service.qservice.thrifscala.ScrayTResultFrame, _oprot: TProtocol): Unit = {
success_item.write(_oprot)
}
@inline private def readExValue(_iprot: TProtocol): scray.service.qservice.thrifscala.ScrayTException = {
scray.service.qservice.thrifscala.ScrayTException.decode(_iprot)
}
@inline private def writeExField(ex_item: scray.service.qservice.thrifscala.ScrayTException, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(ExField)
writeExValue(ex_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeExValue(ex_item: scray.service.qservice.thrifscala.ScrayTException, _oprot: TProtocol): Unit = {
ex_item.write(_oprot)
}
}
class Result(
val success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTResultFrame],
val ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException],
val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends ThriftResponse[scray.service.qservice.thrifscala.ScrayTResultFrame] with ThriftStruct
with _root_.scala.Product2[Option[scray.service.qservice.thrifscala.ScrayTResultFrame], Option[scray.service.qservice.thrifscala.ScrayTException]]
with HasThriftStructCodec3[Result]
with java.io.Serializable
{
import Result._
def this(
success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTResultFrame] = _root_.scala.None,
ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException] = _root_.scala.None
) = this(
success,
ex,
Map.empty
)
def _1 = success
def _2 = ex
def toTuple: _root_.scala.Tuple2[Option[scray.service.qservice.thrifscala.ScrayTResultFrame], Option[scray.service.qservice.thrifscala.ScrayTException]] = {
(
success,
ex
)
}
def successField: Option[scray.service.qservice.thrifscala.ScrayTResultFrame] = success
def exceptionFields: Iterable[Option[com.twitter.scrooge.ThriftException]] = Seq(ex)
override def write(_oprot: TProtocol): Unit = {
Result.validate(this)
_oprot.writeStructBegin(Struct)
if (success.isDefined) writeSuccessField(success.get, _oprot)
if (ex.isDefined) writeExField(ex.get, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
success: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTResultFrame] = this.success,
ex: _root_.scala.Option[scray.service.qservice.thrifscala.ScrayTException] = this.ex,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Result =
new Result(
success,
ex,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Result]
private def _equals(x: Result, y: Result): Boolean =
x.productArity == y.productArity &&
x.productIterator.sameElements(y.productIterator)
override def equals(other: Any): Boolean =
canEqual(other) &&
_equals(this, other.asInstanceOf[Result]) &&
_passthroughFields == other.asInstanceOf[Result]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 2
override def productElement(n: Int): Any = n match {
case 0 => this.success
case 1 => this.ex
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Result"
def _codec: ThriftStructCodec3[Result] = Result
}
val annotations: immutable$Map[String, String] = immutable$Map.empty
type FunctionType = Function1[Args,Future[scray.service.qservice.thrifscala.ScrayTResultFrame]]
type ServiceType = com.twitter.finagle.Service[Args, Result]
type ServiceIfaceServiceType = com.twitter.finagle.Service[Args, SuccessType]
def toServiceIfaceService(f: FunctionType): ServiceIfaceServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args)
}
private[this] val toResult = (res: SuccessType) => Result(Some(res))
def functionToService(f: FunctionType): ServiceType =
com.twitter.finagle.Service.mk { args: Args =>
f(args).map(toResult)
}
def serviceToFunction(svc: ServiceType): FunctionType = { args: Args =>
com.twitter.finagle.thrift.ThriftServiceIface.resultFilter(this).andThen(svc).apply(args)
}
val name: String = "getResults"
val serviceName: String = "ScrayCombinedStatefulTService"
val argsCodec = Args
val responseCodec = Result
val oneway: Boolean = false
}
// Compatibility aliases.
val getResults$args = GetResults.Args
type getResults$args = GetResults.Args
val getResults$result = GetResults.Result
type getResults$result = GetResults.Result
trait FutureIface
extends ScrayCombinedStatefulTService[Future] {
/**
* Fetch a list of service endpoints.
* Each endpoint provides ScrayStatelessTService and ScrayStatefulTService alternatives.
* Queries can address different endpoints for load distribution.
*/
def getServiceEndpoints(): Future[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]]
/**
* Add new service endpoint.
* The endpoint will be removed after a default expiration period.
*/
def addServiceEndpoint(endpoint: scray.service.qservice.thrifscala.ScrayTServiceEndpoint): Future[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]
/**
* Restore the default expiration period of an endpoint.
*/
def refreshServiceEndpoint(endpointID: scray.service.qmodel.thrifscala.ScrayUUID): Future[Unit]
/**
* Return vital sign
*/
def ping(): Future[Boolean]
/**
* Shutdown the server
*/
def shutdown(waitNanos: Option[Long] = None): Future[Unit]
/**
* Submit query
*/
def query(query: scray.service.qmodel.thrifscala.ScrayTQuery): Future[scray.service.qmodel.thrifscala.ScrayUUID]
/**
* Fetch query results
* Paging state is maintained on server side.
* The operation is neither idempotent nor safe.
*/
def getResults(queryId: scray.service.qmodel.thrifscala.ScrayUUID): Future[scray.service.qservice.thrifscala.ScrayTResultFrame]
}
class FinagledClient(
service: com.twitter.finagle.Service[ThriftClientRequest, Array[Byte]],
protocolFactory: org.apache.thrift.protocol.TProtocolFactory = Protocols.binaryFactory(),
serviceName: String = "ScrayCombinedStatefulTService",
stats: com.twitter.finagle.stats.StatsReceiver = com.twitter.finagle.stats.NullStatsReceiver,
responseClassifier: ctfs.ResponseClassifier = ctfs.ResponseClassifier.Default)
extends ScrayCombinedStatefulTService$FinagleClient(
service,
protocolFactory,
serviceName,
stats,
responseClassifier)
with FutureIface {
def this(
service: com.twitter.finagle.Service[ThriftClientRequest, Array[Byte]],
protocolFactory: TProtocolFactory,
serviceName: String,
stats: com.twitter.finagle.stats.StatsReceiver
) = this(service, protocolFactory, serviceName, stats, ctfs.ResponseClassifier.Default)
}
class FinagledService(
iface: FutureIface,
protocolFactory: org.apache.thrift.protocol.TProtocolFactory)
extends ScrayCombinedStatefulTService$FinagleService(
iface,
protocolFactory)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy