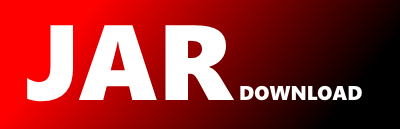
scray.service.qservice.thrifscala.ScrayMetaTService$FinagleClient.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scray-service Show documentation
Show all versions of scray-service Show documentation
scray remote query service interface
/**
* Generated by Scrooge
* version: 4.16.0
* rev: 0201cac9fdd6188248d42da91fd14c87744cc4a5
* built at: 20170421-124523
*/
package scray.service.qservice.thrifscala
import com.twitter.finagle.SourcedException
import com.twitter.finagle.{service => ctfs}
import com.twitter.finagle.stats.{NullStatsReceiver, StatsReceiver}
import com.twitter.finagle.thrift.{Protocols, ThriftClientRequest}
import com.twitter.scrooge.{ThriftStruct, ThriftStructCodec}
import com.twitter.util.{Future, Return, Throw, Throwables}
import java.nio.ByteBuffer
import java.util.Arrays
import org.apache.thrift.protocol._
import org.apache.thrift.TApplicationException
import org.apache.thrift.transport.{TMemoryBuffer, TMemoryInputTransport}
import scala.collection.{Map, Set}
import scala.language.higherKinds
/**
* Scray meta service
* The service is provided by scray seed nodes
*/
@javax.annotation.Generated(value = Array("com.twitter.scrooge.Compiler"))
class ScrayMetaTService$FinagleClient(
val service: com.twitter.finagle.Service[ThriftClientRequest, Array[Byte]],
val protocolFactory: TProtocolFactory,
val serviceName: String,
stats: StatsReceiver,
responseClassifier: ctfs.ResponseClassifier)
extends ScrayMetaTService[Future] {
def this(
service: com.twitter.finagle.Service[ThriftClientRequest, Array[Byte]],
protocolFactory: TProtocolFactory = Protocols.binaryFactory(),
serviceName: String = "ScrayMetaTService",
stats: StatsReceiver = NullStatsReceiver
) = this(
service,
protocolFactory,
serviceName,
stats,
ctfs.ResponseClassifier.Default
)
import ScrayMetaTService._
protected def encodeRequest(name: String, args: ThriftStruct) = {
val buf = new TMemoryBuffer(512)
val oprot = protocolFactory.getProtocol(buf)
oprot.writeMessageBegin(new TMessage(name, TMessageType.CALL, 0))
args.write(oprot)
oprot.writeMessageEnd()
val bytes = Arrays.copyOfRange(buf.getArray, 0, buf.length)
new ThriftClientRequest(bytes, false)
}
protected def decodeResponse[T <: ThriftStruct](resBytes: Array[Byte], codec: ThriftStructCodec[T]) = {
val iprot = protocolFactory.getProtocol(new TMemoryInputTransport(resBytes))
val msg = iprot.readMessageBegin()
try {
if (msg.`type` == TMessageType.EXCEPTION) {
val exception = TApplicationException.read(iprot) match {
case sourced: SourcedException =>
if (serviceName != "") sourced.serviceName = serviceName
sourced
case e => e
}
throw exception
} else {
codec.decode(iprot)
}
} finally {
iprot.readMessageEnd()
}
}
protected def missingResult(name: String) = {
new TApplicationException(
TApplicationException.MISSING_RESULT,
name + " failed: unknown result"
)
}
protected def setServiceName(ex: Throwable): Throwable =
if (this.serviceName == "") ex
else {
ex match {
case se: SourcedException =>
se.serviceName = this.serviceName
se
case _ => ex
}
}
// ----- end boilerplate.
private[this] val scopedStats = if (serviceName != "") stats.scope(serviceName) else stats
private[this] object __stats_getServiceEndpoints {
val RequestsCounter = scopedStats.scope("getServiceEndpoints").counter("requests")
val SuccessCounter = scopedStats.scope("getServiceEndpoints").counter("success")
val FailuresCounter = scopedStats.scope("getServiceEndpoints").counter("failures")
val FailuresScope = scopedStats.scope("getServiceEndpoints").scope("failures")
}
/**
* Fetch a list of service endpoints.
* Each endpoint provides ScrayStatelessTService and ScrayStatefulTService alternatives.
* Queries can address different endpoints for load distribution.
*/
def getServiceEndpoints(): Future[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] = {
__stats_getServiceEndpoints.RequestsCounter.incr()
val inputArgs = GetServiceEndpoints.Args()
val replyDeserializer: Array[Byte] => _root_.com.twitter.util.Try[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]] =
response => {
val result = decodeResponse(response, GetServiceEndpoints.Result)
val exception: Throwable =
null
if (result.success.isDefined)
_root_.com.twitter.util.Return(result.success.get)
else if (exception != null)
_root_.com.twitter.util.Throw(exception)
else
_root_.com.twitter.util.Throw(missingResult("getServiceEndpoints"))
}
val serdeCtx = new _root_.com.twitter.finagle.thrift.DeserializeCtx[Seq[scray.service.qservice.thrifscala.ScrayTServiceEndpoint]](inputArgs, replyDeserializer)
_root_.com.twitter.finagle.context.Contexts.local.let(
_root_.com.twitter.finagle.thrift.DeserializeCtx.Key,
serdeCtx
) {
val serialized = encodeRequest("getServiceEndpoints", inputArgs)
this.service(serialized).flatMap { response =>
Future.const(serdeCtx.deserialize(response))
}.respond { response =>
val responseClass = responseClassifier.applyOrElse(
ctfs.ReqRep(inputArgs, response),
ctfs.ResponseClassifier.Default)
responseClass match {
case ctfs.ResponseClass.Successful(_) =>
__stats_getServiceEndpoints.SuccessCounter.incr()
case ctfs.ResponseClass.Failed(_) =>
__stats_getServiceEndpoints.FailuresCounter.incr()
response match {
case Throw(ex) =>
setServiceName(ex)
__stats_getServiceEndpoints.FailuresScope.counter(Throwables.mkString(ex): _*).incr()
case _ =>
}
}
}
}
}
private[this] object __stats_addServiceEndpoint {
val RequestsCounter = scopedStats.scope("addServiceEndpoint").counter("requests")
val SuccessCounter = scopedStats.scope("addServiceEndpoint").counter("success")
val FailuresCounter = scopedStats.scope("addServiceEndpoint").counter("failures")
val FailuresScope = scopedStats.scope("addServiceEndpoint").scope("failures")
}
/**
* Add new service endpoint.
* The endpoint will be removed after a default expiration period.
*/
def addServiceEndpoint(endpoint: scray.service.qservice.thrifscala.ScrayTServiceEndpoint): Future[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] = {
__stats_addServiceEndpoint.RequestsCounter.incr()
val inputArgs = AddServiceEndpoint.Args(endpoint)
val replyDeserializer: Array[Byte] => _root_.com.twitter.util.Try[scray.service.qservice.thrifscala.ScrayTServiceEndpoint] =
response => {
val result = decodeResponse(response, AddServiceEndpoint.Result)
val exception: Throwable =
null
if (result.success.isDefined)
_root_.com.twitter.util.Return(result.success.get)
else if (exception != null)
_root_.com.twitter.util.Throw(exception)
else
_root_.com.twitter.util.Throw(missingResult("addServiceEndpoint"))
}
val serdeCtx = new _root_.com.twitter.finagle.thrift.DeserializeCtx[scray.service.qservice.thrifscala.ScrayTServiceEndpoint](inputArgs, replyDeserializer)
_root_.com.twitter.finagle.context.Contexts.local.let(
_root_.com.twitter.finagle.thrift.DeserializeCtx.Key,
serdeCtx
) {
val serialized = encodeRequest("addServiceEndpoint", inputArgs)
this.service(serialized).flatMap { response =>
Future.const(serdeCtx.deserialize(response))
}.respond { response =>
val responseClass = responseClassifier.applyOrElse(
ctfs.ReqRep(inputArgs, response),
ctfs.ResponseClassifier.Default)
responseClass match {
case ctfs.ResponseClass.Successful(_) =>
__stats_addServiceEndpoint.SuccessCounter.incr()
case ctfs.ResponseClass.Failed(_) =>
__stats_addServiceEndpoint.FailuresCounter.incr()
response match {
case Throw(ex) =>
setServiceName(ex)
__stats_addServiceEndpoint.FailuresScope.counter(Throwables.mkString(ex): _*).incr()
case _ =>
}
}
}
}
}
private[this] object __stats_refreshServiceEndpoint {
val RequestsCounter = scopedStats.scope("refreshServiceEndpoint").counter("requests")
val SuccessCounter = scopedStats.scope("refreshServiceEndpoint").counter("success")
val FailuresCounter = scopedStats.scope("refreshServiceEndpoint").counter("failures")
val FailuresScope = scopedStats.scope("refreshServiceEndpoint").scope("failures")
}
/**
* Restore the default expiration period of an endpoint.
*/
def refreshServiceEndpoint(endpointID: scray.service.qmodel.thrifscala.ScrayUUID): Future[Unit] = {
__stats_refreshServiceEndpoint.RequestsCounter.incr()
val inputArgs = RefreshServiceEndpoint.Args(endpointID)
val replyDeserializer: Array[Byte] => _root_.com.twitter.util.Try[Unit] =
response => {
val result = decodeResponse(response, RefreshServiceEndpoint.Result)
val exception: Throwable =
null
if (exception != null) _root_.com.twitter.util.Throw(exception) else Return.Unit
}
val serdeCtx = new _root_.com.twitter.finagle.thrift.DeserializeCtx[Unit](inputArgs, replyDeserializer)
_root_.com.twitter.finagle.context.Contexts.local.let(
_root_.com.twitter.finagle.thrift.DeserializeCtx.Key,
serdeCtx
) {
val serialized = encodeRequest("refreshServiceEndpoint", inputArgs)
this.service(serialized).flatMap { response =>
Future.const(serdeCtx.deserialize(response))
}.respond { response =>
val responseClass = responseClassifier.applyOrElse(
ctfs.ReqRep(inputArgs, response),
ctfs.ResponseClassifier.Default)
responseClass match {
case ctfs.ResponseClass.Successful(_) =>
__stats_refreshServiceEndpoint.SuccessCounter.incr()
case ctfs.ResponseClass.Failed(_) =>
__stats_refreshServiceEndpoint.FailuresCounter.incr()
response match {
case Throw(ex) =>
setServiceName(ex)
__stats_refreshServiceEndpoint.FailuresScope.counter(Throwables.mkString(ex): _*).incr()
case _ =>
}
}
}
}
}
private[this] object __stats_ping {
val RequestsCounter = scopedStats.scope("ping").counter("requests")
val SuccessCounter = scopedStats.scope("ping").counter("success")
val FailuresCounter = scopedStats.scope("ping").counter("failures")
val FailuresScope = scopedStats.scope("ping").scope("failures")
}
/**
* Return vital sign
*/
def ping(): Future[Boolean] = {
__stats_ping.RequestsCounter.incr()
val inputArgs = Ping.Args()
val replyDeserializer: Array[Byte] => _root_.com.twitter.util.Try[Boolean] =
response => {
val result = decodeResponse(response, Ping.Result)
val exception: Throwable =
null
if (result.success.isDefined)
_root_.com.twitter.util.Return(result.success.get)
else if (exception != null)
_root_.com.twitter.util.Throw(exception)
else
_root_.com.twitter.util.Throw(missingResult("ping"))
}
val serdeCtx = new _root_.com.twitter.finagle.thrift.DeserializeCtx[Boolean](inputArgs, replyDeserializer)
_root_.com.twitter.finagle.context.Contexts.local.let(
_root_.com.twitter.finagle.thrift.DeserializeCtx.Key,
serdeCtx
) {
val serialized = encodeRequest("ping", inputArgs)
this.service(serialized).flatMap { response =>
Future.const(serdeCtx.deserialize(response))
}.respond { response =>
val responseClass = responseClassifier.applyOrElse(
ctfs.ReqRep(inputArgs, response),
ctfs.ResponseClassifier.Default)
responseClass match {
case ctfs.ResponseClass.Successful(_) =>
__stats_ping.SuccessCounter.incr()
case ctfs.ResponseClass.Failed(_) =>
__stats_ping.FailuresCounter.incr()
response match {
case Throw(ex) =>
setServiceName(ex)
__stats_ping.FailuresScope.counter(Throwables.mkString(ex): _*).incr()
case _ =>
}
}
}
}
}
private[this] object __stats_shutdown {
val RequestsCounter = scopedStats.scope("shutdown").counter("requests")
val SuccessCounter = scopedStats.scope("shutdown").counter("success")
val FailuresCounter = scopedStats.scope("shutdown").counter("failures")
val FailuresScope = scopedStats.scope("shutdown").scope("failures")
}
/**
* Shutdown the server
*/
def shutdown(waitNanos: Option[Long] = None): Future[Unit] = {
__stats_shutdown.RequestsCounter.incr()
val inputArgs = Shutdown.Args(waitNanos)
val replyDeserializer: Array[Byte] => _root_.com.twitter.util.Try[Unit] =
response => {
val result = decodeResponse(response, Shutdown.Result)
val exception: Throwable =
null
if (exception != null) _root_.com.twitter.util.Throw(exception) else Return.Unit
}
val serdeCtx = new _root_.com.twitter.finagle.thrift.DeserializeCtx[Unit](inputArgs, replyDeserializer)
_root_.com.twitter.finagle.context.Contexts.local.let(
_root_.com.twitter.finagle.thrift.DeserializeCtx.Key,
serdeCtx
) {
val serialized = encodeRequest("shutdown", inputArgs)
this.service(serialized).flatMap { response =>
Future.const(serdeCtx.deserialize(response))
}.respond { response =>
val responseClass = responseClassifier.applyOrElse(
ctfs.ReqRep(inputArgs, response),
ctfs.ResponseClassifier.Default)
responseClass match {
case ctfs.ResponseClass.Successful(_) =>
__stats_shutdown.SuccessCounter.incr()
case ctfs.ResponseClass.Failed(_) =>
__stats_shutdown.FailuresCounter.incr()
response match {
case Throw(ex) =>
setServiceName(ex)
__stats_shutdown.FailuresScope.counter(Throwables.mkString(ex): _*).incr()
case _ =>
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy