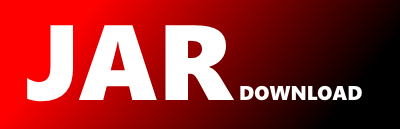
scray.service.qservice.thrifscala.ScrayStatefulTService$FinagleClient.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scray-service Show documentation
Show all versions of scray-service Show documentation
scray remote query service interface
/**
* Generated by Scrooge
* version: 4.16.0
* rev: 0201cac9fdd6188248d42da91fd14c87744cc4a5
* built at: 20170421-124523
*/
package scray.service.qservice.thrifscala
import com.twitter.finagle.SourcedException
import com.twitter.finagle.{service => ctfs}
import com.twitter.finagle.stats.{NullStatsReceiver, StatsReceiver}
import com.twitter.finagle.thrift.{Protocols, ThriftClientRequest}
import com.twitter.scrooge.{ThriftStruct, ThriftStructCodec}
import com.twitter.util.{Future, Return, Throw, Throwables}
import java.nio.ByteBuffer
import java.util.Arrays
import org.apache.thrift.protocol._
import org.apache.thrift.TApplicationException
import org.apache.thrift.transport.{TMemoryBuffer, TMemoryInputTransport}
import scala.collection.{Map, Set}
import scala.language.higherKinds
/**
* Stateful query service with continuous result pages
*/
@javax.annotation.Generated(value = Array("com.twitter.scrooge.Compiler"))
class ScrayStatefulTService$FinagleClient(
val service: com.twitter.finagle.Service[ThriftClientRequest, Array[Byte]],
val protocolFactory: TProtocolFactory,
val serviceName: String,
stats: StatsReceiver,
responseClassifier: ctfs.ResponseClassifier)
extends ScrayStatefulTService[Future] {
def this(
service: com.twitter.finagle.Service[ThriftClientRequest, Array[Byte]],
protocolFactory: TProtocolFactory = Protocols.binaryFactory(),
serviceName: String = "ScrayStatefulTService",
stats: StatsReceiver = NullStatsReceiver
) = this(
service,
protocolFactory,
serviceName,
stats,
ctfs.ResponseClassifier.Default
)
import ScrayStatefulTService._
protected def encodeRequest(name: String, args: ThriftStruct) = {
val buf = new TMemoryBuffer(512)
val oprot = protocolFactory.getProtocol(buf)
oprot.writeMessageBegin(new TMessage(name, TMessageType.CALL, 0))
args.write(oprot)
oprot.writeMessageEnd()
val bytes = Arrays.copyOfRange(buf.getArray, 0, buf.length)
new ThriftClientRequest(bytes, false)
}
protected def decodeResponse[T <: ThriftStruct](resBytes: Array[Byte], codec: ThriftStructCodec[T]) = {
val iprot = protocolFactory.getProtocol(new TMemoryInputTransport(resBytes))
val msg = iprot.readMessageBegin()
try {
if (msg.`type` == TMessageType.EXCEPTION) {
val exception = TApplicationException.read(iprot) match {
case sourced: SourcedException =>
if (serviceName != "") sourced.serviceName = serviceName
sourced
case e => e
}
throw exception
} else {
codec.decode(iprot)
}
} finally {
iprot.readMessageEnd()
}
}
protected def missingResult(name: String) = {
new TApplicationException(
TApplicationException.MISSING_RESULT,
name + " failed: unknown result"
)
}
protected def setServiceName(ex: Throwable): Throwable =
if (this.serviceName == "") ex
else {
ex match {
case se: SourcedException =>
se.serviceName = this.serviceName
se
case _ => ex
}
}
// ----- end boilerplate.
private[this] val scopedStats = if (serviceName != "") stats.scope(serviceName) else stats
private[this] object __stats_query {
val RequestsCounter = scopedStats.scope("query").counter("requests")
val SuccessCounter = scopedStats.scope("query").counter("success")
val FailuresCounter = scopedStats.scope("query").counter("failures")
val FailuresScope = scopedStats.scope("query").scope("failures")
}
/**
* Submit query
*/
def query(query: scray.service.qmodel.thrifscala.ScrayTQuery): Future[scray.service.qmodel.thrifscala.ScrayUUID] = {
__stats_query.RequestsCounter.incr()
val inputArgs = Query.Args(query)
val replyDeserializer: Array[Byte] => _root_.com.twitter.util.Try[scray.service.qmodel.thrifscala.ScrayUUID] =
response => {
val result = decodeResponse(response, Query.Result)
val exception: Throwable =
if (false)
null // can never happen, but needed to open a block
else if (result.ex.isDefined)
setServiceName(result.ex.get)
else
null
if (result.success.isDefined)
_root_.com.twitter.util.Return(result.success.get)
else if (exception != null)
_root_.com.twitter.util.Throw(exception)
else
_root_.com.twitter.util.Throw(missingResult("query"))
}
val serdeCtx = new _root_.com.twitter.finagle.thrift.DeserializeCtx[scray.service.qmodel.thrifscala.ScrayUUID](inputArgs, replyDeserializer)
_root_.com.twitter.finagle.context.Contexts.local.let(
_root_.com.twitter.finagle.thrift.DeserializeCtx.Key,
serdeCtx
) {
val serialized = encodeRequest("query", inputArgs)
this.service(serialized).flatMap { response =>
Future.const(serdeCtx.deserialize(response))
}.respond { response =>
val responseClass = responseClassifier.applyOrElse(
ctfs.ReqRep(inputArgs, response),
ctfs.ResponseClassifier.Default)
responseClass match {
case ctfs.ResponseClass.Successful(_) =>
__stats_query.SuccessCounter.incr()
case ctfs.ResponseClass.Failed(_) =>
__stats_query.FailuresCounter.incr()
response match {
case Throw(ex) =>
setServiceName(ex)
__stats_query.FailuresScope.counter(Throwables.mkString(ex): _*).incr()
case _ =>
}
}
}
}
}
private[this] object __stats_getResults {
val RequestsCounter = scopedStats.scope("getResults").counter("requests")
val SuccessCounter = scopedStats.scope("getResults").counter("success")
val FailuresCounter = scopedStats.scope("getResults").counter("failures")
val FailuresScope = scopedStats.scope("getResults").scope("failures")
}
/**
* Fetch query results
* Paging state is maintained on server side.
* The operation is neither idempotent nor safe.
*/
def getResults(queryId: scray.service.qmodel.thrifscala.ScrayUUID): Future[scray.service.qservice.thrifscala.ScrayTResultFrame] = {
__stats_getResults.RequestsCounter.incr()
val inputArgs = GetResults.Args(queryId)
val replyDeserializer: Array[Byte] => _root_.com.twitter.util.Try[scray.service.qservice.thrifscala.ScrayTResultFrame] =
response => {
val result = decodeResponse(response, GetResults.Result)
val exception: Throwable =
if (false)
null // can never happen, but needed to open a block
else if (result.ex.isDefined)
setServiceName(result.ex.get)
else
null
if (result.success.isDefined)
_root_.com.twitter.util.Return(result.success.get)
else if (exception != null)
_root_.com.twitter.util.Throw(exception)
else
_root_.com.twitter.util.Throw(missingResult("getResults"))
}
val serdeCtx = new _root_.com.twitter.finagle.thrift.DeserializeCtx[scray.service.qservice.thrifscala.ScrayTResultFrame](inputArgs, replyDeserializer)
_root_.com.twitter.finagle.context.Contexts.local.let(
_root_.com.twitter.finagle.thrift.DeserializeCtx.Key,
serdeCtx
) {
val serialized = encodeRequest("getResults", inputArgs)
this.service(serialized).flatMap { response =>
Future.const(serdeCtx.deserialize(response))
}.respond { response =>
val responseClass = responseClassifier.applyOrElse(
ctfs.ReqRep(inputArgs, response),
ctfs.ResponseClassifier.Default)
responseClass match {
case ctfs.ResponseClass.Successful(_) =>
__stats_getResults.SuccessCounter.incr()
case ctfs.ResponseClass.Failed(_) =>
__stats_getResults.FailuresCounter.incr()
response match {
case Throw(ex) =>
setServiceName(ex)
__stats_getResults.FailuresScope.counter(Throwables.mkString(ex): _*).incr()
case _ =>
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy