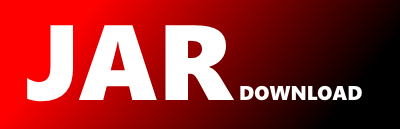
sculptormetamodel.Module Maven / Gradle / Ivy
/**
*/
package sculptormetamodel;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Module'.
*
*
*
* The following features are supported:
*
* - {@link sculptormetamodel.Module#getBasePackage Base Package}
* - {@link sculptormetamodel.Module#getApplication Application}
* - {@link sculptormetamodel.Module#getDomainObjects Domain Objects}
* - {@link sculptormetamodel.Module#getConsumers Consumers}
* - {@link sculptormetamodel.Module#getServices Services}
* - {@link sculptormetamodel.Module#isExternal External}
* - {@link sculptormetamodel.Module#getPersistenceUnit Persistence Unit}
* - {@link sculptormetamodel.Module#getResources Resources}
*
*
*
* @see sculptormetamodel.SculptormetamodelPackage#getModule()
* @model
* @generated
*/
public interface Module extends NamedElement
{
/**
* Returns the value of the 'Base Package' attribute.
*
*
* If the meaning of the 'Base Package' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Base Package' attribute.
* @see #setBasePackage(String)
* @see sculptormetamodel.SculptormetamodelPackage#getModule_BasePackage()
* @model
* @generated
*/
String getBasePackage();
/**
* Sets the value of the '{@link sculptormetamodel.Module#getBasePackage Base Package}' attribute.
*
*
* @param value the new value of the 'Base Package' attribute.
* @see #getBasePackage()
* @generated
*/
void setBasePackage(String value);
/**
* Returns the value of the 'Application' container reference.
* It is bidirectional and its opposite is '{@link sculptormetamodel.Application#getModules Modules}'.
*
*
* If the meaning of the 'Application' container reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Application' container reference.
* @see #setApplication(Application)
* @see sculptormetamodel.SculptormetamodelPackage#getModule_Application()
* @see sculptormetamodel.Application#getModules
* @model opposite="modules" transient="false"
* @generated
*/
Application getApplication();
/**
* Sets the value of the '{@link sculptormetamodel.Module#getApplication Application}' container reference.
*
*
* @param value the new value of the 'Application' container reference.
* @see #getApplication()
* @generated
*/
void setApplication(Application value);
/**
* Returns the value of the 'Domain Objects' containment reference list.
* The list contents are of type {@link sculptormetamodel.DomainObject}.
* It is bidirectional and its opposite is '{@link sculptormetamodel.DomainObject#getModule Module}'.
*
*
* If the meaning of the 'Domain Objects' containment reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Domain Objects' containment reference list.
* @see sculptormetamodel.SculptormetamodelPackage#getModule_DomainObjects()
* @see sculptormetamodel.DomainObject#getModule
* @model opposite="module" containment="true"
* @generated
*/
EList getDomainObjects();
/**
* Returns the value of the 'Consumers' containment reference list.
* The list contents are of type {@link sculptormetamodel.Consumer}.
* It is bidirectional and its opposite is '{@link sculptormetamodel.Consumer#getModule Module}'.
*
*
* If the meaning of the 'Consumers' containment reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Consumers' containment reference list.
* @see sculptormetamodel.SculptormetamodelPackage#getModule_Consumers()
* @see sculptormetamodel.Consumer#getModule
* @model opposite="module" containment="true"
* @generated
*/
EList getConsumers();
/**
* Returns the value of the 'Services' containment reference list.
* The list contents are of type {@link sculptormetamodel.Service}.
* It is bidirectional and its opposite is '{@link sculptormetamodel.Service#getModule Module}'.
*
*
* If the meaning of the 'Services' containment reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Services' containment reference list.
* @see sculptormetamodel.SculptormetamodelPackage#getModule_Services()
* @see sculptormetamodel.Service#getModule
* @model opposite="module" containment="true"
* @generated
*/
EList getServices();
/**
* Returns the value of the 'External' attribute.
*
*
* If the meaning of the 'External' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'External' attribute.
* @see #setExternal(boolean)
* @see sculptormetamodel.SculptormetamodelPackage#getModule_External()
* @model
* @generated
*/
boolean isExternal();
/**
* Sets the value of the '{@link sculptormetamodel.Module#isExternal External}' attribute.
*
*
* @param value the new value of the 'External' attribute.
* @see #isExternal()
* @generated
*/
void setExternal(boolean value);
/**
* Returns the value of the 'Persistence Unit' attribute.
*
*
* If the meaning of the 'Persistence Unit' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Persistence Unit' attribute.
* @see #setPersistenceUnit(String)
* @see sculptormetamodel.SculptormetamodelPackage#getModule_PersistenceUnit()
* @model
* @generated
*/
String getPersistenceUnit();
/**
* Sets the value of the '{@link sculptormetamodel.Module#getPersistenceUnit Persistence Unit}' attribute.
*
*
* @param value the new value of the 'Persistence Unit' attribute.
* @see #getPersistenceUnit()
* @generated
*/
void setPersistenceUnit(String value);
/**
* Returns the value of the 'Resources' containment reference list.
* The list contents are of type {@link sculptormetamodel.Resource}.
* It is bidirectional and its opposite is '{@link sculptormetamodel.Resource#getModule Module}'.
*
*
* If the meaning of the 'Resources' containment reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Resources' containment reference list.
* @see sculptormetamodel.SculptormetamodelPackage#getModule_Resources()
* @see sculptormetamodel.Resource#getModule
* @model opposite="module" containment="true"
* @generated
*/
EList getResources();
} // Module