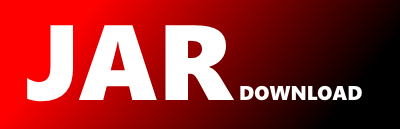
sculptormetamodel.impl.AttributeImpl Maven / Gradle / Ivy
/**
*/
package sculptormetamodel.impl;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import sculptormetamodel.Attribute;
import sculptormetamodel.SculptormetamodelPackage;
/**
*
* An implementation of the model object 'Attribute'.
*
*
* The following features are implemented:
*
* - {@link sculptormetamodel.impl.AttributeImpl#isChangeable Changeable}
* - {@link sculptormetamodel.impl.AttributeImpl#getDatabaseType Database Type}
* - {@link sculptormetamodel.impl.AttributeImpl#isNullable Nullable}
* - {@link sculptormetamodel.impl.AttributeImpl#isNaturalKey Natural Key}
* - {@link sculptormetamodel.impl.AttributeImpl#getVisibility Visibility}
* - {@link sculptormetamodel.impl.AttributeImpl#isRequired Required}
* - {@link sculptormetamodel.impl.AttributeImpl#getLength Length}
* - {@link sculptormetamodel.impl.AttributeImpl#isIndex Index}
* - {@link sculptormetamodel.impl.AttributeImpl#getDatabaseColumn Database Column}
* - {@link sculptormetamodel.impl.AttributeImpl#getValidate Validate}
* - {@link sculptormetamodel.impl.AttributeImpl#isTransient Transient}
*
*
*
* @generated
*/
public class AttributeImpl extends TypedElementImpl implements Attribute
{
/**
* The default value of the '{@link #isChangeable() Changeable}' attribute.
*
*
* @see #isChangeable()
* @generated
* @ordered
*/
protected static final boolean CHANGEABLE_EDEFAULT = true;
/**
* The cached value of the '{@link #isChangeable() Changeable}' attribute.
*
*
* @see #isChangeable()
* @generated
* @ordered
*/
protected boolean changeable = CHANGEABLE_EDEFAULT;
/**
* The default value of the '{@link #getDatabaseType() Database Type}' attribute.
*
*
* @see #getDatabaseType()
* @generated
* @ordered
*/
protected static final String DATABASE_TYPE_EDEFAULT = null;
/**
* The cached value of the '{@link #getDatabaseType() Database Type}' attribute.
*
*
* @see #getDatabaseType()
* @generated
* @ordered
*/
protected String databaseType = DATABASE_TYPE_EDEFAULT;
/**
* The default value of the '{@link #isNullable() Nullable}' attribute.
*
*
* @see #isNullable()
* @generated
* @ordered
*/
protected static final boolean NULLABLE_EDEFAULT = false;
/**
* The cached value of the '{@link #isNullable() Nullable}' attribute.
*
*
* @see #isNullable()
* @generated
* @ordered
*/
protected boolean nullable = NULLABLE_EDEFAULT;
/**
* The default value of the '{@link #isNaturalKey() Natural Key}' attribute.
*
*
* @see #isNaturalKey()
* @generated
* @ordered
*/
protected static final boolean NATURAL_KEY_EDEFAULT = false;
/**
* The cached value of the '{@link #isNaturalKey() Natural Key}' attribute.
*
*
* @see #isNaturalKey()
* @generated
* @ordered
*/
protected boolean naturalKey = NATURAL_KEY_EDEFAULT;
/**
* The default value of the '{@link #getVisibility() Visibility}' attribute.
*
*
* @see #getVisibility()
* @generated
* @ordered
*/
protected static final String VISIBILITY_EDEFAULT = null;
/**
* The cached value of the '{@link #getVisibility() Visibility}' attribute.
*
*
* @see #getVisibility()
* @generated
* @ordered
*/
protected String visibility = VISIBILITY_EDEFAULT;
/**
* The default value of the '{@link #isRequired() Required}' attribute.
*
*
* @see #isRequired()
* @generated
* @ordered
*/
protected static final boolean REQUIRED_EDEFAULT = false;
/**
* The cached value of the '{@link #isRequired() Required}' attribute.
*
*
* @see #isRequired()
* @generated
* @ordered
*/
protected boolean required = REQUIRED_EDEFAULT;
/**
* The default value of the '{@link #getLength() Length}' attribute.
*
*
* @see #getLength()
* @generated
* @ordered
*/
protected static final String LENGTH_EDEFAULT = null;
/**
* The cached value of the '{@link #getLength() Length}' attribute.
*
*
* @see #getLength()
* @generated
* @ordered
*/
protected String length = LENGTH_EDEFAULT;
/**
* The default value of the '{@link #isIndex() Index}' attribute.
*
*
* @see #isIndex()
* @generated
* @ordered
*/
protected static final boolean INDEX_EDEFAULT = false;
/**
* The cached value of the '{@link #isIndex() Index}' attribute.
*
*
* @see #isIndex()
* @generated
* @ordered
*/
protected boolean index = INDEX_EDEFAULT;
/**
* The default value of the '{@link #getDatabaseColumn() Database Column}' attribute.
*
*
* @see #getDatabaseColumn()
* @generated
* @ordered
*/
protected static final String DATABASE_COLUMN_EDEFAULT = null;
/**
* The cached value of the '{@link #getDatabaseColumn() Database Column}' attribute.
*
*
* @see #getDatabaseColumn()
* @generated
* @ordered
*/
protected String databaseColumn = DATABASE_COLUMN_EDEFAULT;
/**
* The default value of the '{@link #getValidate() Validate}' attribute.
*
*
* @see #getValidate()
* @generated
* @ordered
*/
protected static final String VALIDATE_EDEFAULT = null;
/**
* The cached value of the '{@link #getValidate() Validate}' attribute.
*
*
* @see #getValidate()
* @generated
* @ordered
*/
protected String validate = VALIDATE_EDEFAULT;
/**
* The default value of the '{@link #isTransient() Transient}' attribute.
*
*
* @see #isTransient()
* @generated
* @ordered
*/
protected static final boolean TRANSIENT_EDEFAULT = false;
/**
* The cached value of the '{@link #isTransient() Transient}' attribute.
*
*
* @see #isTransient()
* @generated
* @ordered
*/
protected boolean transient_ = TRANSIENT_EDEFAULT;
/**
*
*
* @generated
*/
protected AttributeImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass()
{
return SculptormetamodelPackage.Literals.ATTRIBUTE;
}
/**
*
*
* @generated
*/
public boolean isChangeable()
{
return changeable;
}
/**
*
*
* @generated
*/
public void setChangeable(boolean newChangeable)
{
boolean oldChangeable = changeable;
changeable = newChangeable;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__CHANGEABLE, oldChangeable, changeable));
}
/**
*
*
* @generated
*/
public String getDatabaseType()
{
return databaseType;
}
/**
*
*
* @generated
*/
public void setDatabaseType(String newDatabaseType)
{
String oldDatabaseType = databaseType;
databaseType = newDatabaseType;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__DATABASE_TYPE, oldDatabaseType, databaseType));
}
/**
*
*
* @generated
*/
public boolean isNullable()
{
return nullable;
}
/**
*
*
* @generated
*/
public void setNullable(boolean newNullable)
{
boolean oldNullable = nullable;
nullable = newNullable;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__NULLABLE, oldNullable, nullable));
}
/**
*
*
* @generated
*/
public boolean isNaturalKey()
{
return naturalKey;
}
/**
*
*
* @generated
*/
public void setNaturalKey(boolean newNaturalKey)
{
boolean oldNaturalKey = naturalKey;
naturalKey = newNaturalKey;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__NATURAL_KEY, oldNaturalKey, naturalKey));
}
/**
*
*
* @generated
*/
public String getVisibility()
{
return visibility;
}
/**
*
*
* @generated
*/
public void setVisibility(String newVisibility)
{
String oldVisibility = visibility;
visibility = newVisibility;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__VISIBILITY, oldVisibility, visibility));
}
/**
*
*
* @generated
*/
public boolean isRequired()
{
return required;
}
/**
*
*
* @generated
*/
public void setRequired(boolean newRequired)
{
boolean oldRequired = required;
required = newRequired;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__REQUIRED, oldRequired, required));
}
/**
*
*
* @generated
*/
public String getLength()
{
return length;
}
/**
*
*
* @generated
*/
public void setLength(String newLength)
{
String oldLength = length;
length = newLength;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__LENGTH, oldLength, length));
}
/**
*
*
* @generated
*/
public boolean isIndex()
{
return index;
}
/**
*
*
* @generated
*/
public void setIndex(boolean newIndex)
{
boolean oldIndex = index;
index = newIndex;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__INDEX, oldIndex, index));
}
/**
*
*
* @generated
*/
public String getDatabaseColumn()
{
return databaseColumn;
}
/**
*
*
* @generated
*/
public void setDatabaseColumn(String newDatabaseColumn)
{
String oldDatabaseColumn = databaseColumn;
databaseColumn = newDatabaseColumn;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__DATABASE_COLUMN, oldDatabaseColumn, databaseColumn));
}
/**
*
*
* @generated
*/
public String getValidate()
{
return validate;
}
/**
*
*
* @generated
*/
public void setValidate(String newValidate)
{
String oldValidate = validate;
validate = newValidate;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__VALIDATE, oldValidate, validate));
}
/**
*
*
* @generated
*/
public boolean isTransient()
{
return transient_;
}
/**
*
*
* @generated
*/
public void setTransient(boolean newTransient)
{
boolean oldTransient = transient_;
transient_ = newTransient;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, SculptormetamodelPackage.ATTRIBUTE__TRANSIENT, oldTransient, transient_));
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType)
{
switch (featureID)
{
case SculptormetamodelPackage.ATTRIBUTE__CHANGEABLE:
return isChangeable();
case SculptormetamodelPackage.ATTRIBUTE__DATABASE_TYPE:
return getDatabaseType();
case SculptormetamodelPackage.ATTRIBUTE__NULLABLE:
return isNullable();
case SculptormetamodelPackage.ATTRIBUTE__NATURAL_KEY:
return isNaturalKey();
case SculptormetamodelPackage.ATTRIBUTE__VISIBILITY:
return getVisibility();
case SculptormetamodelPackage.ATTRIBUTE__REQUIRED:
return isRequired();
case SculptormetamodelPackage.ATTRIBUTE__LENGTH:
return getLength();
case SculptormetamodelPackage.ATTRIBUTE__INDEX:
return isIndex();
case SculptormetamodelPackage.ATTRIBUTE__DATABASE_COLUMN:
return getDatabaseColumn();
case SculptormetamodelPackage.ATTRIBUTE__VALIDATE:
return getValidate();
case SculptormetamodelPackage.ATTRIBUTE__TRANSIENT:
return isTransient();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue)
{
switch (featureID)
{
case SculptormetamodelPackage.ATTRIBUTE__CHANGEABLE:
setChangeable((Boolean)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__DATABASE_TYPE:
setDatabaseType((String)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__NULLABLE:
setNullable((Boolean)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__NATURAL_KEY:
setNaturalKey((Boolean)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__VISIBILITY:
setVisibility((String)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__REQUIRED:
setRequired((Boolean)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__LENGTH:
setLength((String)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__INDEX:
setIndex((Boolean)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__DATABASE_COLUMN:
setDatabaseColumn((String)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__VALIDATE:
setValidate((String)newValue);
return;
case SculptormetamodelPackage.ATTRIBUTE__TRANSIENT:
setTransient((Boolean)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID)
{
switch (featureID)
{
case SculptormetamodelPackage.ATTRIBUTE__CHANGEABLE:
setChangeable(CHANGEABLE_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__DATABASE_TYPE:
setDatabaseType(DATABASE_TYPE_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__NULLABLE:
setNullable(NULLABLE_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__NATURAL_KEY:
setNaturalKey(NATURAL_KEY_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__VISIBILITY:
setVisibility(VISIBILITY_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__REQUIRED:
setRequired(REQUIRED_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__LENGTH:
setLength(LENGTH_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__INDEX:
setIndex(INDEX_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__DATABASE_COLUMN:
setDatabaseColumn(DATABASE_COLUMN_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__VALIDATE:
setValidate(VALIDATE_EDEFAULT);
return;
case SculptormetamodelPackage.ATTRIBUTE__TRANSIENT:
setTransient(TRANSIENT_EDEFAULT);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID)
{
switch (featureID)
{
case SculptormetamodelPackage.ATTRIBUTE__CHANGEABLE:
return changeable != CHANGEABLE_EDEFAULT;
case SculptormetamodelPackage.ATTRIBUTE__DATABASE_TYPE:
return DATABASE_TYPE_EDEFAULT == null ? databaseType != null : !DATABASE_TYPE_EDEFAULT.equals(databaseType);
case SculptormetamodelPackage.ATTRIBUTE__NULLABLE:
return nullable != NULLABLE_EDEFAULT;
case SculptormetamodelPackage.ATTRIBUTE__NATURAL_KEY:
return naturalKey != NATURAL_KEY_EDEFAULT;
case SculptormetamodelPackage.ATTRIBUTE__VISIBILITY:
return VISIBILITY_EDEFAULT == null ? visibility != null : !VISIBILITY_EDEFAULT.equals(visibility);
case SculptormetamodelPackage.ATTRIBUTE__REQUIRED:
return required != REQUIRED_EDEFAULT;
case SculptormetamodelPackage.ATTRIBUTE__LENGTH:
return LENGTH_EDEFAULT == null ? length != null : !LENGTH_EDEFAULT.equals(length);
case SculptormetamodelPackage.ATTRIBUTE__INDEX:
return index != INDEX_EDEFAULT;
case SculptormetamodelPackage.ATTRIBUTE__DATABASE_COLUMN:
return DATABASE_COLUMN_EDEFAULT == null ? databaseColumn != null : !DATABASE_COLUMN_EDEFAULT.equals(databaseColumn);
case SculptormetamodelPackage.ATTRIBUTE__VALIDATE:
return VALIDATE_EDEFAULT == null ? validate != null : !VALIDATE_EDEFAULT.equals(validate);
case SculptormetamodelPackage.ATTRIBUTE__TRANSIENT:
return transient_ != TRANSIENT_EDEFAULT;
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString()
{
if (eIsProxy()) return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (changeable: ");
result.append(changeable);
result.append(", databaseType: ");
result.append(databaseType);
result.append(", nullable: ");
result.append(nullable);
result.append(", naturalKey: ");
result.append(naturalKey);
result.append(", visibility: ");
result.append(visibility);
result.append(", required: ");
result.append(required);
result.append(", length: ");
result.append(length);
result.append(", index: ");
result.append(index);
result.append(", databaseColumn: ");
result.append(databaseColumn);
result.append(", validate: ");
result.append(validate);
result.append(", transient: ");
result.append(transient_);
result.append(')');
return result.toString();
}
} //AttributeImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy