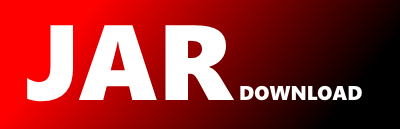
org.sdmlib.models.classes.FeatureProperty Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SDMLib Show documentation
Show all versions of SDMLib Show documentation
SDMLib is a light weight modeling library. SDMLib intentionally comes without any tool or editor.
package org.sdmlib.models.classes;
import java.util.HashSet;
import de.uniks.networkparser.graph.Clazz;
public class FeatureProperty {
public static final Clazz ALL = new Clazz().with("*");
private HashSet includeClazz = new HashSet();
private HashSet excludeClazz = new HashSet();
private HashSet path = new HashSet();
public FeatureProperty(){
includeClazz.add(ALL);
}
public boolean match(String clazzName){
// if Clazz is positive
boolean result=false;
for(Clazz item : includeClazz) {
if(item == null) {
continue;
}
if(ALL.getName(false).equals(item.getName(false))) {
result = true;
break;
} else if(item.getName(false).equals(clazzName)) {
result = true;
break;
}
}
for(Clazz item : excludeClazz) {
if(item == null) {
continue;
}
if(ALL.getName(false).equals(item.getName(false))) {
result = false;
break;
} else if(item.getName(false).equals(clazzName)) {
result = false;
break;
}
}
return result;
}
public FeatureProperty withPath(String... value) {
if(value == null) {
return this;
}
for(String item : value) {
if(item != null) {
path.add(item);
}
}
return this;
}
public FeatureProperty withInclude(String... value) {
if(value == null) {
return this;
}
for(String item : value) {
if(item != null) {
excludeClazz.add(new Clazz().with(item));
}
}
return this;
}
public FeatureProperty withExclude(String... value) {
if(value == null) {
return this;
}
if(value.length > 0 ){
// remove ALL
includeClazz.remove(ALL);
}
for(String item : value) {
if(item != null) {
includeClazz.add(new Clazz().with(item));
}
}
return this;
}
public FeatureProperty withExclude(Clazz... value) {
if(value == null) {
return this;
}
if(value.length > 0 ){
// remove ALL
includeClazz.remove(ALL);
}
for(Clazz item : value) {
if(item != null) {
includeClazz.add(item);
}
}
return this;
}
public HashSet getPath() {
return path;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy