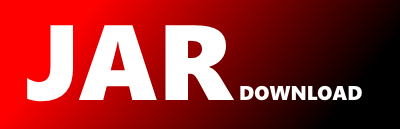
org.sdmlib.models.pattern.util.GenericConstraintPO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SDMLib Show documentation
Show all versions of SDMLib Show documentation
SDMLib is a light weight modeling library. SDMLib intentionally comes without any tool or editor.
package org.sdmlib.models.pattern.util;
import org.sdmlib.models.pattern.AttributeConstraint;
import org.sdmlib.models.pattern.GenericConstraint;
import org.sdmlib.models.pattern.Pattern;
import org.sdmlib.models.pattern.PatternElement;
import org.sdmlib.models.pattern.PatternObject;
public class GenericConstraintPO extends PatternObject
{
public GenericConstraintPO(){
newInstance(CreatorCreator.createIdMap("PatternObjectType"));
}
public GenericConstraintPO(GenericConstraint... hostGraphObject) {
if(hostGraphObject==null || hostGraphObject.length<1){
return ;
}
newInstance(CreatorCreator.createIdMap("PatternObjectType"), hostGraphObject);
}
public GenericConstraintSet allMatches()
{
this.setDoAllMatches(true);
GenericConstraintSet matches = new GenericConstraintSet();
while (this.getPattern().getHasMatch())
{
matches.add((GenericConstraint) this.getCurrentMatch());
this.getPattern().findMatch();
}
return matches;
}
public GenericConstraintPO hasModifier(String value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_MODIFIER)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public String getModifier()
{
if (this.getPattern().getHasMatch())
{
return ((GenericConstraint) getCurrentMatch()).getModifier();
}
return null;
}
public GenericConstraintPO withModifier(String value)
{
if (this.getPattern().getHasMatch())
{
((GenericConstraint) getCurrentMatch()).setModifier(value);
}
return this;
}
public GenericConstraintPO hasHasMatch(boolean value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_HASMATCH)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public boolean getHasMatch()
{
if (this.getPattern().getHasMatch())
{
return ((GenericConstraint) getCurrentMatch()).getHasMatch();
}
return false;
}
public GenericConstraintPO withHasMatch(boolean value)
{
if (this.getPattern().getHasMatch())
{
((GenericConstraint) getCurrentMatch()).setHasMatch(value);
}
return this;
}
public GenericConstraintPO hasPatternObjectName(String value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_PATTERNOBJECTNAME)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public String getPatternObjectName()
{
if (this.getPattern().getHasMatch())
{
return ((GenericConstraint) getCurrentMatch()).getPatternObjectName();
}
return null;
}
public GenericConstraintPO withPatternObjectName(String value)
{
if (this.getPattern().getHasMatch())
{
((GenericConstraint) getCurrentMatch()).setPatternObjectName(value);
}
return this;
}
public GenericConstraintPO hasDoAllMatches(boolean value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_DOALLMATCHES)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public boolean getDoAllMatches()
{
if (this.getPattern().getHasMatch())
{
return ((GenericConstraint) getCurrentMatch()).getDoAllMatches();
}
return false;
}
public GenericConstraintPO withDoAllMatches(boolean value)
{
if (this.getPattern().getHasMatch())
{
((GenericConstraint) getCurrentMatch()).setDoAllMatches(value);
}
return this;
}
public PatternPO hasPattern()
{
PatternPO result = new PatternPO(new Pattern[]{});
result.setModifier(this.getPattern().getModifier());
super.hasLink(PatternElement.PROPERTY_PATTERN, result);
return result;
}
public GenericConstraintPO hasPattern(PatternPO tgt)
{
return hasLinkConstraint(tgt, PatternElement.PROPERTY_PATTERN);
}
public Pattern getPattern()
{
if (super.getPattern().getHasMatch())
{
return ((PatternElement) this.getCurrentMatch()).getPattern();
}
return super.getPattern();
}
public GenericConstraintPO hasModifier(String lower, String upper)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_MODIFIER)
.withTgtValue(lower)
.withUpperTgtValue(upper)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public GenericConstraintPO hasHasMatch(boolean lower, boolean upper)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_HASMATCH)
.withTgtValue(lower)
.withUpperTgtValue(upper)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public GenericConstraintPO hasPatternObjectName(String lower, String upper)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_PATTERNOBJECTNAME)
.withTgtValue(lower)
.withUpperTgtValue(upper)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public GenericConstraintPO hasDoAllMatches(boolean lower, boolean upper)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_DOALLMATCHES)
.withTgtValue(lower)
.withUpperTgtValue(upper)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public GenericConstraintPO createModifier(String value)
{
this.startCreate().hasModifier(value).endCreate();
return this;
}
public GenericConstraintPO createHasMatch(boolean value)
{
this.startCreate().hasHasMatch(value).endCreate();
return this;
}
public GenericConstraintPO createPatternObjectName(String value)
{
this.startCreate().hasPatternObjectName(value).endCreate();
return this;
}
public GenericConstraintPO createDoAllMatches(boolean value)
{
this.startCreate().hasDoAllMatches(value).endCreate();
return this;
}
public PatternPO createPattern()
{
return this.startCreate().hasPattern().endCreate();
}
public GenericConstraintPO createPattern(PatternPO tgt)
{
return this.startCreate().hasPattern(tgt).endCreate();
}
public GenericConstraintPO hasText(String value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_TEXT)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public GenericConstraintPO hasText(String lower, String upper)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_TEXT)
.withTgtValue(lower)
.withUpperTgtValue(upper)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
this.getPattern().findMatch();
return this;
}
public GenericConstraintPO createText(String value)
{
this.startCreate().hasText(value).endCreate();
return this;
}
public String getText()
{
if (this.getPattern().getHasMatch())
{
return ((GenericConstraint) getCurrentMatch()).getText();
}
return null;
}
public GenericConstraintPO withText(String value)
{
if (this.getPattern().getHasMatch())
{
((GenericConstraint) getCurrentMatch()).setText(value);
}
return this;
}
public GenericConstraintPO filterText(String value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_TEXT)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
super.filterAttr();
return this;
}
public GenericConstraintPO filterText(String lower, String upper)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_TEXT)
.withTgtValue(lower)
.withUpperTgtValue(upper)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
super.filterAttr();
return this;
}
public GenericConstraintPO filterModifier(String value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_MODIFIER)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
super.filterAttr();
return this;
}
public GenericConstraintPO filterModifier(String lower, String upper)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_MODIFIER)
.withTgtValue(lower)
.withUpperTgtValue(upper)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
super.filterAttr();
return this;
}
public GenericConstraintPO filterHasMatch(boolean value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_HASMATCH)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
super.filterAttr();
return this;
}
public GenericConstraintPO filterPatternObjectName(String value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_PATTERNOBJECTNAME)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
super.filterAttr();
return this;
}
public GenericConstraintPO filterPatternObjectName(String lower, String upper)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_PATTERNOBJECTNAME)
.withTgtValue(lower)
.withUpperTgtValue(upper)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
super.filterAttr();
return this;
}
public GenericConstraintPO filterDoAllMatches(boolean value)
{
new AttributeConstraint()
.withAttrName(GenericConstraint.PROPERTY_DOALLMATCHES)
.withTgtValue(value)
.withSrc(this)
.withModifier(this.getPattern().getModifier())
.withPattern(this.getPattern());
super.filterAttr();
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy