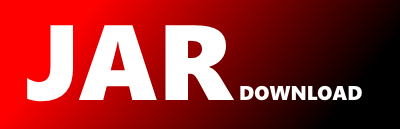
org.sdmlib.models.pattern.util.ReachabilityGraphPO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SDMLib Show documentation
Show all versions of SDMLib Show documentation
SDMLib is a light weight modeling library. SDMLib intentionally comes without any tool or editor.
package org.sdmlib.models.pattern.util;
import org.sdmlib.models.pattern.Pattern;
import org.sdmlib.models.pattern.PatternObject;
import org.sdmlib.models.pattern.ReachabilityGraph;
import org.sdmlib.models.pattern.ReachableState;
public class ReachabilityGraphPO extends PatternObject
{
public ReachabilityGraphPO(){
newInstance(null);
}
public ReachabilityGraphPO(ReachabilityGraph... hostGraphObject) {
if(hostGraphObject==null || hostGraphObject.length<1){
return ;
}
newInstance(null, hostGraphObject);
}
public ReachabilityGraphSet allMatches()
{
this.setDoAllMatches(true);
ReachabilityGraphSet matches = new ReachabilityGraphSet();
while (this.getPattern().getHasMatch())
{
matches.add((ReachabilityGraph) this.getCurrentMatch());
this.getPattern().findMatch();
}
return matches;
}
public ReachableStatePO filterStates()
{
ReachableStatePO result = new ReachableStatePO(new ReachableState[]{});
result.setModifier(this.getPattern().getModifier());
super.hasLink(ReachabilityGraph.PROPERTY_STATES, result);
return result;
}
public ReachabilityGraphPO hasStates(ReachableStatePO tgt)
{
return hasLinkConstraint(tgt, ReachabilityGraph.PROPERTY_STATES);
}
public ReachableStateSet getStates()
{
if (this.getPattern().getHasMatch())
{
return ((ReachabilityGraph) this.getCurrentMatch()).getStates();
}
return null;
}
public ReachableStatePO hasTodo()
{
ReachableStatePO result = new ReachableStatePO(new ReachableState[]{});
result.setModifier(this.getPattern().getModifier());
super.hasLink(ReachabilityGraph.PROPERTY_TODO, result);
return result;
}
public ReachabilityGraphPO hasTodo(ReachableStatePO tgt)
{
return hasLinkConstraint(tgt, ReachabilityGraph.PROPERTY_TODO);
}
public ReachableStateSet getTodo()
{
// if (this.getPattern().getHasMatch())
// {
// return ((ReachabilityGraph) this.getCurrentMatch()).getTodo();
// }
return null;
}
public PatternPO hasRules()
{
PatternPO result = new PatternPO(new Pattern[]{});
result.setModifier(this.getPattern().getModifier());
super.hasLink(ReachabilityGraph.PROPERTY_RULES, result);
return result;
}
public ReachabilityGraphPO hasRules(PatternPO tgt)
{
return hasLinkConstraint(tgt, ReachabilityGraph.PROPERTY_RULES);
}
public PatternSet getRules()
{
if (this.getPattern().getHasMatch())
{
return ((ReachabilityGraph) this.getCurrentMatch()).getRules();
}
return null;
}
public ReachableStatePO createStates()
{
return this.startCreate().filterStates().endCreate();
}
public ReachabilityGraphPO createStates(ReachableStatePO tgt)
{
return this.startCreate().hasStates(tgt).endCreate();
}
public ReachableStatePO createTodo()
{
return this.startCreate().hasTodo().endCreate();
}
public ReachabilityGraphPO createTodo(ReachableStatePO tgt)
{
return this.startCreate().hasTodo(tgt).endCreate();
}
public PatternPO createRules()
{
return this.startCreate().hasRules().endCreate();
}
public ReachabilityGraphPO createRules(PatternPO tgt)
{
return this.startCreate().hasRules(tgt).endCreate();
}
public ReachabilityGraphPO filterStates(ReachableStatePO tgt)
{
return hasLinkConstraint(tgt, ReachabilityGraph.PROPERTY_STATES);
}
public ReachableStatePO filterTodo()
{
ReachableStatePO result = new ReachableStatePO(new ReachableState[]{});
result.setModifier(this.getPattern().getModifier());
super.hasLink(ReachabilityGraph.PROPERTY_TODO, result);
return result;
}
public ReachabilityGraphPO filterTodo(ReachableStatePO tgt)
{
return hasLinkConstraint(tgt, ReachabilityGraph.PROPERTY_TODO);
}
public PatternPO filterRules()
{
PatternPO result = new PatternPO(new Pattern[]{});
result.setModifier(this.getPattern().getModifier());
super.hasLink(ReachabilityGraph.PROPERTY_RULES, result);
return result;
}
public ReachabilityGraphPO filterRules(PatternPO tgt)
{
return hasLinkConstraint(tgt, ReachabilityGraph.PROPERTY_RULES);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy