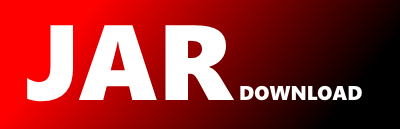
org.securegraph.accumulo.AccumuloVertex Maven / Gradle / Ivy
The newest version!
package org.securegraph.accumulo;
import org.apache.hadoop.io.Text;
import org.securegraph.*;
import org.securegraph.mutation.ExistingElementMutation;
import org.securegraph.mutation.ExistingElementMutationImpl;
import org.securegraph.mutation.PropertyRemoveMutation;
import org.securegraph.query.VertexQuery;
import org.securegraph.util.ConvertingIterable;
import org.securegraph.util.JoinIterable;
import org.securegraph.util.LookAheadIterable;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import static org.securegraph.util.IterableUtils.count;
import static org.securegraph.util.IterableUtils.toSet;
public class AccumuloVertex extends AccumuloElement implements Vertex {
public static final Text CF_SIGNAL = new Text("V");
public static final Text CF_OUT_EDGE = new Text("EOUT");
public static final Text CF_OUT_EDGE_HIDDEN = new Text("EOUTH");
public static final Text CF_IN_EDGE = new Text("EIN");
public static final Text CF_IN_EDGE_HIDDEN = new Text("EINH");
private final Map inEdges;
private final Map outEdges;
public AccumuloVertex(
AccumuloGraph graph,
String vertexId,
Visibility vertexVisibility,
Iterable properties,
Iterable propertyRemoveMutations,
Iterable hiddenVisibilities,
Authorizations authorizations,
long timestamp
) {
this(
graph,
vertexId,
vertexVisibility,
properties,
propertyRemoveMutations,
hiddenVisibilities,
new HashMap(),
new HashMap(),
authorizations,
timestamp
);
}
AccumuloVertex(
AccumuloGraph graph,
String vertexId,
Visibility vertexVisibility,
Iterable properties,
Iterable propertyRemoveMutations,
Iterable hiddenVisibilities,
Map inEdges,
Map outEdges,
Authorizations authorizations,
long timestamp
) {
super(graph, vertexId, vertexVisibility, properties, propertyRemoveMutations, hiddenVisibilities, authorizations, timestamp);
this.inEdges = inEdges;
this.outEdges = outEdges;
}
@Override
public Iterable getEdges(Direction direction, Authorizations authorizations) {
return getEdges(direction, FetchHint.ALL, authorizations);
}
@Override
public Iterable getEdges(Direction direction, EnumSet fetchHints, Authorizations authorizations) {
return getGraph().getEdges(getEdgeIds(direction, authorizations), fetchHints, authorizations);
}
@Override
public Iterable getEdgeIds(Direction direction, Authorizations authorizations) {
return getEdgeIdsWithOtherVertexId(null, direction, null, authorizations);
}
@Override
public Iterable getEdges(Direction direction, String label, Authorizations authorizations) {
return getEdges(direction, label, FetchHint.ALL, authorizations);
}
@Override
public Iterable getEdges(Direction direction, String label, EnumSet fetchHints, Authorizations authorizations) {
return getGraph().getEdges(getEdgeIds(direction, labelToArrayOrNull(label), authorizations), fetchHints, authorizations);
}
@Override
public Iterable getEdgeIds(Direction direction, String label, Authorizations authorizations) {
return getEdgeIdsWithOtherVertexId(null, direction, labelToArrayOrNull(label), authorizations);
}
@Override
public Iterable getEdges(Direction direction, String[] labels, Authorizations authorizations) {
return getEdges(direction, labels, FetchHint.ALL, authorizations);
}
@Override
public Iterable getEdges(Direction direction, final String[] labels, EnumSet fetchHints, Authorizations authorizations) {
return getGraph().getEdges(getEdgeIdsWithOtherVertexId(null, direction, labels, authorizations), fetchHints, authorizations);
}
@Override
public Iterable getEdgeIds(final Direction direction, final String[] labels, final Authorizations authorizations) {
return getEdgeIdsWithOtherVertexId(null, direction, labels, authorizations);
}
@Override
public Iterable getEdges(Vertex otherVertex, Direction direction, Authorizations authorizations) {
return getEdges(otherVertex, direction, FetchHint.ALL, authorizations);
}
@Override
public Iterable getEdges(final Vertex otherVertex, Direction direction, EnumSet fetchHints, Authorizations authorizations) {
return getGraph().getEdges(getEdgeIdsWithOtherVertexId(otherVertex.getId(), direction, null, authorizations), fetchHints, authorizations);
}
@Override
public Iterable getEdgeIds(Vertex otherVertex, Direction direction, Authorizations authorizations) {
return getEdgeIdsWithOtherVertexId(otherVertex.getId(), direction, null, authorizations);
}
@Override
public Iterable getEdges(Vertex otherVertex, Direction direction, String label, Authorizations authorizations) {
return getEdges(otherVertex, direction, label, FetchHint.ALL, authorizations);
}
@Override
public Iterable getEdges(final Vertex otherVertex, Direction direction, String label, EnumSet fetchHints, Authorizations authorizations) {
return getGraph().getEdges(getEdgeIdsWithOtherVertexId(otherVertex.getId(), direction, labelToArrayOrNull(label), authorizations), fetchHints, authorizations);
}
@Override
public Iterable getEdgeIds(Vertex otherVertex, Direction direction, String label, Authorizations authorizations) {
return getEdgeIdsWithOtherVertexId(otherVertex.getId(), direction, labelToArrayOrNull(label), authorizations);
}
@Override
public Iterable getEdges(Vertex otherVertex, Direction direction, String[] labels, Authorizations authorizations) {
return getEdges(otherVertex, direction, labels, FetchHint.ALL, authorizations);
}
@Override
public Iterable getEdges(final Vertex otherVertex, Direction direction, String[] labels, EnumSet fetchHints, Authorizations authorizations) {
return getGraph().getEdges(getEdgeIdsWithOtherVertexId(otherVertex.getId(), direction, labels, authorizations), fetchHints, authorizations);
}
@Override
public Iterable getEdgeIds(final Vertex otherVertex, final Direction direction, final String[] labels, final Authorizations authorizations) {
return getEdgeIdsWithOtherVertexId(otherVertex.getId(), direction, labels, authorizations);
}
@Override
public int getEdgeCount(Direction direction, Authorizations authorizations) {
return count(getEdgeIds(direction, authorizations));
}
@Override
public Iterable getEdgeLabels(Direction direction, Authorizations authorizations) {
return toSet(new ConvertingIterable, String>(getEdgeInfos(direction)) {
@Override
protected String convert(Map.Entry o) {
return o.getValue().getLabel();
}
});
}
@Override
public Iterable getVertices(Direction direction, Authorizations authorizations) {
return getVertices(direction, FetchHint.ALL, authorizations);
}
@SuppressWarnings("unused")
public Iterable getEdgeIdsWithOtherVertexId(final String otherVertexId, final Direction direction, final String[] labels, final Authorizations authorizations) {
return new LookAheadIterable, String>() {
@Override
protected boolean isIncluded(Map.Entry edgeInfo, String edgeId) {
if (otherVertexId != null) {
if (!otherVertexId.equals(edgeInfo.getValue().getVertexId())) {
return false;
}
}
if (labels == null || labels.length == 0) {
return true;
}
for (String label : labels) {
if (label.equals(edgeInfo.getValue().getLabel())) {
return true;
}
}
return false;
}
@Override
protected String convert(Map.Entry edgeInfo) {
return edgeInfo.getKey();
}
@Override
protected Iterator> createIterator() {
return getEdgeInfos(direction).iterator();
}
};
}
private Iterable> getEdgeInfos(Direction direction) {
switch (direction) {
case IN:
return this.inEdges.entrySet();
case OUT:
return this.outEdges.entrySet();
case BOTH:
return new JoinIterable<>(this.inEdges.entrySet(), this.outEdges.entrySet());
default:
throw new SecureGraphException("Unexpected direction: " + direction);
}
}
@Override
public Iterable getVertices(Direction direction, EnumSet fetchHints, final Authorizations authorizations) {
return getGraph().getVertices(getVertexIds(direction, authorizations), fetchHints, authorizations);
}
@Override
public Iterable getVertices(Direction direction, String label, Authorizations authorizations) {
return getVertices(direction, label, FetchHint.ALL, authorizations);
}
@Override
public Iterable getVertices(Direction direction, String label, EnumSet fetchHints, Authorizations authorizations) {
return getVertices(direction, labelToArrayOrNull(label), fetchHints, authorizations);
}
@Override
public Iterable getVertices(Direction direction, String[] labels, Authorizations authorizations) {
return getVertices(direction, labels, FetchHint.ALL, authorizations);
}
@Override
public Iterable getVertices(Direction direction, String[] labels, EnumSet fetchHints, final Authorizations authorizations) {
return getGraph().getVertices(getVertexIds(direction, labels, authorizations), fetchHints, authorizations);
}
@Override
public Iterable getVertexIds(Direction direction, String label, Authorizations authorizations) {
return getVertexIds(direction, labelToArrayOrNull(label), authorizations);
}
@Override
public Iterable getVertexIds(Direction direction, Authorizations authorizations) {
return getVertexIds(direction, (String[]) null, authorizations);
}
@Override
public Iterable getVertexIds(Direction direction, String[] labels, Authorizations authorizations) {
switch (direction) {
case BOTH:
Iterable inVertexIds = getVertexIds(Direction.IN, labels, authorizations);
Iterable outVertexIds = getVertexIds(Direction.OUT, labels, authorizations);
return new JoinIterable<>(inVertexIds, outVertexIds);
case IN:
return new GetVertexIdsIterable(this.inEdges.values(), labels);
case OUT:
return new GetVertexIdsIterable(this.outEdges.values(), labels);
default:
throw new SecureGraphException("Unexpected direction: " + direction);
}
}
@Override
public VertexQuery query(Authorizations authorizations) {
return query(null, authorizations);
}
@Override
public VertexQuery query(String queryString, Authorizations authorizations) {
return getGraph().getSearchIndex().queryVertex(getGraph(), this, queryString, authorizations);
}
void addOutEdge(Edge edge) {
this.outEdges.put(edge.getId(), new EdgeInfo(edge.getLabel(), edge.getVertexId(Direction.IN)));
}
void removeOutEdge(Edge edge) {
this.outEdges.remove(edge.getId());
}
void addInEdge(Edge edge) {
this.inEdges.put(edge.getId(), new EdgeInfo(edge.getLabel(), edge.getVertexId(Direction.OUT)));
}
void removeInEdge(Edge edge) {
this.inEdges.remove(edge.getId());
}
@Override
@SuppressWarnings("unchecked")
public ExistingElementMutation prepareMutation() {
return new ExistingElementMutationImpl(this) {
@Override
public Vertex save(Authorizations authorizations) {
saveExistingElementMutation(this, authorizations);
return getElement();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy