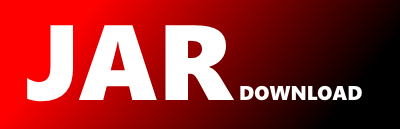
org.securegraph.GraphBase Maven / Gradle / Ivy
The newest version!
package org.securegraph;
import org.securegraph.event.GraphEvent;
import org.securegraph.event.GraphEventListener;
import org.securegraph.path.PathFindingAlgorithm;
import org.securegraph.path.RecursivePathFindingAlgorithm;
import org.securegraph.query.GraphQuery;
import org.securegraph.util.LookAheadIterable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
import static org.securegraph.util.IterableUtils.toList;
public abstract class GraphBase implements Graph {
private static final Logger LOGGER = LoggerFactory.getLogger(GraphBase.class);
private final PathFindingAlgorithm pathFindingAlgorithm = new RecursivePathFindingAlgorithm();
private final List graphEventListeners = new ArrayList();
protected GraphBase() {
}
@Override
public Vertex addVertex(Visibility visibility, Authorizations authorizations) {
return prepareVertex(visibility).save(authorizations);
}
@Override
public Vertex addVertex(String vertexId, Visibility visibility, Authorizations authorizations) {
return prepareVertex(vertexId, visibility).save(authorizations);
}
@Override
public Iterable addVertices(Iterable> vertices, Authorizations authorizations) {
List addedVertices = new ArrayList();
for (ElementBuilder vertexBuilder : vertices) {
addedVertices.add(vertexBuilder.save(authorizations));
}
return addedVertices;
}
@Override
public VertexBuilder prepareVertex(Visibility visibility) {
return prepareVertex(getIdGenerator().nextId(), visibility);
}
@Override
public boolean doesVertexExist(String vertexId, Authorizations authorizations) {
return getVertex(vertexId, FetchHint.NONE, authorizations) != null;
}
@Override
public Vertex getVertex(String vertexId, EnumSet fetchHints, Authorizations authorizations) {
LOGGER.warn("Performing scan of all vertices! Override getVertex.");
for (Vertex vertex : getVertices(fetchHints, authorizations)) {
if (vertex.getId().equals(vertexId)) {
return vertex;
}
}
return null;
}
@Override
public Vertex getVertex(String vertexId, Authorizations authorizations) throws SecureGraphException {
return getVertex(vertexId, FetchHint.ALL, authorizations);
}
@Override
public Iterable getVertices(final Iterable ids, EnumSet fetchHints, final Authorizations authorizations) {
LOGGER.warn("Getting each vertex one by one! Override getVertices(java.lang.Iterable, org.securegraph.Authorizations)");
return new LookAheadIterable() {
@Override
protected boolean isIncluded(String src, Vertex vertex) {
return vertex != null;
}
@Override
protected Vertex convert(String id) {
return getVertex(id, authorizations);
}
@Override
protected Iterator createIterator() {
return ids.iterator();
}
};
}
@Override
public Map doVerticesExist(List ids, Authorizations authorizations) {
Map results = new HashMap();
for (String id : ids) {
results.put(id, false);
}
for (Vertex vertex : getVertices(ids, FetchHint.NONE, authorizations)) {
results.put(vertex.getId(), true);
}
return results;
}
@Override
public Iterable getVertices(final Iterable ids, final Authorizations authorizations) {
return getVertices(ids, FetchHint.ALL, authorizations);
}
@Override
public List getVerticesInOrder(Iterable ids, EnumSet fetchHints, Authorizations authorizations) {
final List vertexIds = toList(ids);
List vertices = toList(getVertices(vertexIds, authorizations));
Collections.sort(vertices, new Comparator() {
@Override
public int compare(Vertex v1, Vertex v2) {
Integer i1 = vertexIds.indexOf(v1.getId());
Integer i2 = vertexIds.indexOf(v2.getId());
return i1.compareTo(i2);
}
});
return vertices;
}
@Override
public List getVerticesInOrder(Iterable ids, Authorizations authorizations) {
return getVerticesInOrder(ids, FetchHint.ALL, authorizations);
}
@Override
public Iterable getVertices(Authorizations authorizations) throws SecureGraphException {
return getVertices(FetchHint.ALL, authorizations);
}
@Override
public abstract Iterable getVertices(EnumSet fetchHints, Authorizations authorizations);
@Override
public abstract void removeVertex(Vertex vertex, Authorizations authorizations);
@Override
public Edge addEdge(Vertex outVertex, Vertex inVertex, String label, Visibility visibility, Authorizations authorizations) {
return prepareEdge(outVertex, inVertex, label, visibility).save(authorizations);
}
@Override
public Edge addEdge(String edgeId, Vertex outVertex, Vertex inVertex, String label, Visibility visibility, Authorizations authorizations) {
return prepareEdge(edgeId, outVertex, inVertex, label, visibility).save(authorizations);
}
@Override
public Edge addEdge(String outVertexId, String inVertexId, String label, Visibility visibility, Authorizations authorizations) {
return prepareEdge(outVertexId, inVertexId, label, visibility).save(authorizations);
}
@Override
public Edge addEdge(String edgeId, String outVertexId, String inVertexId, String label, Visibility visibility, Authorizations authorizations) {
return prepareEdge(edgeId, outVertexId, inVertexId, label, visibility).save(authorizations);
}
@Override
public EdgeBuilderByVertexId prepareEdge(String outVertexId, String inVertexId, String label, Visibility visibility) {
return prepareEdge(getIdGenerator().nextId(), outVertexId, inVertexId, label, visibility);
}
@Override
public EdgeBuilder prepareEdge(Vertex outVertex, Vertex inVertex, String label, Visibility visibility) {
return prepareEdge(getIdGenerator().nextId(), outVertex, inVertex, label, visibility);
}
@Override
public boolean doesEdgeExist(String edgeId, Authorizations authorizations) {
return getEdge(edgeId, FetchHint.NONE, authorizations) != null;
}
@Override
public Edge getEdge(String edgeId, EnumSet fetchHints, Authorizations authorizations) {
LOGGER.warn("Performing scan of all edges! Override getEdge.");
for (Edge edge : getEdges(fetchHints, authorizations)) {
if (edge.getId().equals(edgeId)) {
return edge;
}
}
return null;
}
@Override
public Edge getEdge(String edgeId, Authorizations authorizations) {
return getEdge(edgeId, FetchHint.ALL, authorizations);
}
@Override
public Map doEdgesExist(List ids, Authorizations authorizations) {
Map results = new HashMap();
for (String id : ids) {
results.put(id, false);
}
for (Edge edge : getEdges(ids, FetchHint.NONE, authorizations)) {
results.put(edge.getId(), true);
}
return results;
}
@Override
public Iterable getEdges(final Iterable ids, EnumSet fetchHints, final Authorizations authorizations) {
LOGGER.warn("Getting each edge one by one! Override getEdges(java.lang.Iterable, org.securegraph.Authorizations)");
return new LookAheadIterable() {
@Override
protected boolean isIncluded(String src, Edge edge) {
return edge != null;
}
@Override
protected Edge convert(String id) {
return getEdge(id, authorizations);
}
@Override
protected Iterator createIterator() {
return ids.iterator();
}
};
}
@Override
public Iterable getEdges(final Iterable ids, final Authorizations authorizations) {
return getEdges(ids, FetchHint.ALL, authorizations);
}
@Override
public Iterable getEdges(Authorizations authorizations) {
return getEdges(FetchHint.ALL, authorizations);
}
@Override
public abstract Iterable getEdges(EnumSet fetchHints, Authorizations authorizations);
@Override
public abstract void removeEdge(Edge edge, Authorizations authorizations);
@Override
public Iterable findPaths(Vertex sourceVertex, Vertex destVertex, int maxHops, Authorizations authorizations) {
ProgressCallback progressCallback = new ProgressCallback() {
@Override
public void progress(double progressPercent, Step step, Integer edgeIndex, Integer vertexCount) {
LOGGER.debug(String.format("findPaths progress %d%%: %s", (int) (progressPercent * 100.0), step.formatMessage(edgeIndex,vertexCount)));
}
};
return findPaths(sourceVertex, destVertex, maxHops, progressCallback, authorizations);
}
@Override
public Iterable findPaths(Vertex sourceVertex, Vertex destVertex, int maxHops, ProgressCallback progressCallback, Authorizations authorizations) {
return pathFindingAlgorithm.findPaths(this, sourceVertex, destVertex, maxHops, progressCallback, authorizations);
}
@Override
public Iterable findPaths(String sourceVertexId, String destVertexId, int maxHops, ProgressCallback progressCallback, Authorizations authorizations) {
EnumSet fetchHints = FetchHint.EDGE_REFS;
Vertex sourceVertex = getVertex(sourceVertexId, fetchHints, authorizations);
if (sourceVertex == null) {
throw new IllegalArgumentException("Could not find vertex with id: " + sourceVertexId);
}
Vertex destVertex = getVertex(destVertexId, fetchHints, authorizations);
if (destVertex == null) {
throw new IllegalArgumentException("Could not find vertex with id: " + destVertexId);
}
return findPaths(sourceVertex, destVertex, maxHops, progressCallback, authorizations);
}
@Override
public Iterable findPaths(String sourceVertexId, String destVertexId, int maxHops, Authorizations authorizations) {
EnumSet fetchHints = FetchHint.EDGE_REFS;
Vertex sourceVertex = getVertex(sourceVertexId, fetchHints, authorizations);
if (sourceVertex == null) {
throw new IllegalArgumentException("Could not find vertex with id: " + sourceVertexId);
}
Vertex destVertex = getVertex(destVertexId, fetchHints, authorizations);
if (destVertex == null) {
throw new IllegalArgumentException("Could not find vertex with id: " + destVertexId);
}
return findPaths(sourceVertex, destVertex, maxHops, authorizations);
}
@Override
public Iterable findRelatedEdges(Iterable vertexIds, Authorizations authorizations) {
Set results = new HashSet();
List vertices = toList(getVertices(vertexIds, authorizations));
// since we are checking bi-directional edges we should only have to check v1->v2 and not v2->v1
Map checkedCombinations = new HashMap();
for (Vertex sourceVertex : vertices) {
for (Vertex destVertex : vertices) {
if (checkedCombinations.containsKey(sourceVertex.getId() + destVertex.getId())) {
continue;
}
Iterable edgeIds = sourceVertex.getEdgeIds(destVertex, Direction.BOTH, authorizations);
for (String edgeId : edgeIds) {
results.add(edgeId);
}
checkedCombinations.put(sourceVertex.getId() + destVertex.getId(), "");
checkedCombinations.put(destVertex.getId() + sourceVertex.getId(), "");
}
}
return results;
}
@Override
public void removeEdge(String edgeId, Authorizations authorizations) {
Edge edge = getEdge(edgeId, authorizations);
if (edge == null) {
throw new IllegalArgumentException("Could not find edge with id: " + edgeId);
}
removeEdge(edge, authorizations);
}
@Override
public abstract Iterable getMetadata();
@Override
public Object getMetadata(String key) {
for (GraphMetadataEntry e : getMetadata()) {
if (e.getKey().equals(key)) {
return e.getValue();
}
}
return null;
}
@Override
public abstract void setMetadata(String key, Object value);
@Override
public abstract GraphQuery query(Authorizations authorizations);
@Override
public abstract GraphQuery query(String queryString, Authorizations authorizations);
@Override
public abstract void reindex(Authorizations authorizations);
@Override
public abstract void flush();
@Override
public abstract void shutdown();
@Override
public abstract DefinePropertyBuilder defineProperty(String propertyName);
@Override
public abstract boolean isFieldBoostSupported();
@Override
public abstract SearchIndexSecurityGranularity getSearchIndexSecurityGranularity();
@Override
public void addGraphEventListener(GraphEventListener graphEventListener) {
this.graphEventListeners.add(graphEventListener);
}
protected boolean hasEventListeners() {
return this.graphEventListeners.size() > 0;
}
protected void fireGraphEvent(GraphEvent graphEvent) {
for (GraphEventListener graphEventListener : this.graphEventListeners) {
graphEventListener.onGraphEvent(graphEvent);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy