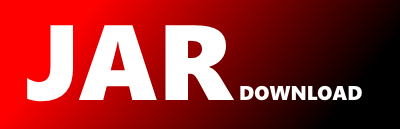
org.securegraph.query.VertexQueryBase Maven / Gradle / Ivy
The newest version!
package org.securegraph.query;
import org.securegraph.*;
import org.securegraph.util.FilterIterable;
import java.util.EnumSet;
import java.util.Map;
public abstract class VertexQueryBase extends QueryBase implements VertexQuery {
private final Vertex sourceVertex;
protected VertexQueryBase(Graph graph, Vertex sourceVertex, String queryString, Map propertyDefinitions, Authorizations authorizations) {
super(graph, queryString, propertyDefinitions, authorizations);
this.sourceVertex = sourceVertex;
}
@Override
public abstract Iterable vertices(EnumSet fetchHints);
@Override
public abstract Iterable edges(EnumSet fetchHints);
@Override
public Iterable edges(final Direction direction, EnumSet fetchHints) {
return new FilterIterable(edges(fetchHints)) {
@Override
protected boolean isIncluded(Edge edge) {
switch (direction) {
case BOTH:
return true;
case IN:
return edge.getVertexId(Direction.IN).equals(sourceVertex.getId());
case OUT:
return edge.getVertexId(Direction.OUT).equals(sourceVertex.getId());
default:
throw new RuntimeException("Unexpected direction: " + direction);
}
}
};
}
@Override
public Iterable edges(final Direction direction) {
return edges(direction, FetchHint.ALL);
}
@Override
public Iterable edges(Direction direction, final String label, EnumSet fetchHints) {
return new FilterIterable(edges(direction, fetchHints)) {
@Override
protected boolean isIncluded(Edge o) {
return label.equals(o.getLabel());
}
};
}
@Override
public Iterable edges(Direction direction, final String label) {
return edges(direction, label, FetchHint.ALL);
}
public Vertex getSourceVertex() {
return sourceVertex;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy