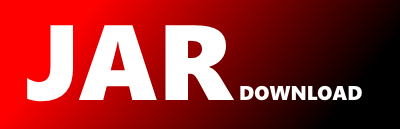
org.seedstack.netflix.hystrix.internal.CoffigHystrixDynamicProperties Maven / Gradle / Ivy
/**
* Copyright (c) 2013-2016, The SeedStack authors
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package org.seedstack.netflix.hystrix.internal;
import com.netflix.hystrix.strategy.properties.HystrixDynamicProperties;
import com.netflix.hystrix.strategy.properties.HystrixDynamicProperty;
import java.util.HashMap;
import java.util.Map;
import java.util.function.Function;
import org.seedstack.netflix.hystrix.HystrixConfig;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class CoffigHystrixDynamicProperties implements HystrixDynamicProperties {
private static final Logger LOGGER = LoggerFactory.getLogger(CoffigHystrixDynamicProperties.class);
private volatile Map properties;
@Override
public HystrixDynamicProperty getInteger(final String name, final Integer fallback) {
return new CoffigHystrixDynamicProperty<>(name, Integer::valueOf, fallback);
}
@Override
public HystrixDynamicProperty getString(final String name, final String fallback) {
return new CoffigHystrixDynamicProperty<>(name, Function.identity(), fallback);
}
@Override
public HystrixDynamicProperty getLong(final String name, final Long fallback) {
return new CoffigHystrixDynamicProperty<>(name, Long::valueOf, fallback);
}
@Override
public HystrixDynamicProperty getBoolean(final String name, final Boolean fallback) {
return new CoffigHystrixDynamicProperty<>(name, Boolean::valueOf, fallback);
}
void setHystrixConfig(HystrixConfig hystrixConfig) {
this.properties = new HashMap<>(hystrixConfig.getProperties());
}
private class CoffigHystrixDynamicProperty implements HystrixDynamicProperty {
private final String name;
private final Function converter;
private final T fallback;
private T value;
CoffigHystrixDynamicProperty(String name, Function converter, T fallback) {
this.name = name.substring(8); // removes the hystrix. prefix
this.converter = converter;
this.fallback = fallback;
fetchValue();
}
@Override
public String getName() {
return name;
}
@Override
public T get() {
return value;
}
@Override
public void addCallback(Runnable callback) {
// no support for dynamic properties yet
}
private void fetchValue() {
String valueAsString = properties.get(this.name);
this.value = valueAsString != null ? converter.apply(valueAsString) : fallback;
LOGGER.trace("Fetched hystrix property {}: {}", this.name, this.value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy