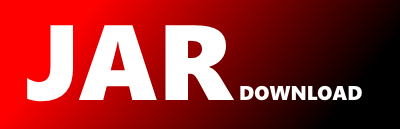
org.seedstack.netflix.hystrix.internal.command.GenericCommand Maven / Gradle / Ivy
/**
* Copyright (c) 2013-2016, The SeedStack authors
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package org.seedstack.netflix.hystrix.internal.command;
import rx.Observable;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.concurrent.Future;
class GenericCommand extends com.netflix.hystrix.HystrixCommand
© 2015 - 2025 Weber Informatics LLC | Privacy Policy