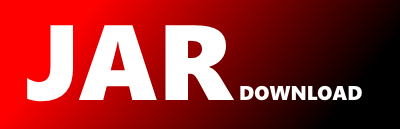
org.seedstack.business.Service Maven / Gradle / Ivy
/*
* Copyright © 2013-2024, The SeedStack authors
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package org.seedstack.business;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* A service is a stateless object that implements domain, applicative, infrastructure or interface
* logic.
*
* This annotation can be applied to an interface to declare a service. Any class implementing
* the annotated interface will be registered by the framework as an implementation of this service.
*
*
* Considering the following service:
*
* {@literal @}Service
* public interface MoneyTransferService {
* void transfer(Account source, Account target);
* }
*
* Its implementation is declared as:
*
* public class ElectronicMoneyTransferService implements MoneyTransferService {
* public void transfer(Account source, Account target) {
* // implementation
* }
* }
*
* The implementation can then be injected as follows:
*
* public class SomeClass {
* {@literal @}Inject
* MoneyTransferService moneyTransferService;
* }
*
*
* When a service interface has multiple implementations, it is necessary to differentiate them
* by using a different {@code javax.inject.Qualifier} annotation on each. This qualifier can then
* be used at the injection point to specify which implementation is required. Considering the
* additional implementation of the MoneyTransferService below:
*
* {@literal @}Named("solidGold")
* public class SolidGoldMoneyTransferService implements MoneyTransferService {
* public void transfer(Account source, Account target) {
* // implementation
* }
* }
*
* This implementation can be injected as follows:
*
* public class SomeClass {
* {@literal @}Inject
* {@literal @}Named("solidGold")
* TransferService transferService;
* }
*
*/
@Documented
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.TYPE, ElementType.ANNOTATION_TYPE})
public @interface Service {
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy