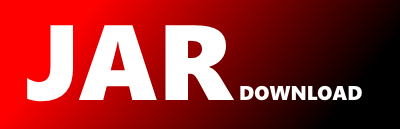
org.seedstack.business.assembler.dsl.AssembleMultiple Maven / Gradle / Ivy
/*
* Copyright © 2013-2024, The SeedStack authors
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package org.seedstack.business.assembler.dsl;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import java.util.function.Supplier;
import java.util.stream.Stream;
import org.seedstack.business.pagination.Slice;
/**
* An element of the {@link FluentAssembler} DSL allowing to assemble to multiple DTO in various
* forms (stream, list, set, array, ...).
*/
public interface AssembleMultiple {
/**
* Assembles to a {@link Stream} of DTO.
*
* @param the type of the DTO.
* @param dtoClass the DTO class to assemble.
* @return the list of DTO.
*/
Stream toStreamOf(Class dtoClass);
/**
* Assembles to the supplied {@link Collection}.
*
* @param the type of the DTO.
* @param the type of the collection.
* @param dtoClass the DTO class to assemble.
* @param collectionSupplier the provider of a (preferably empty) collection.
* @return the collection of DTO.
*/
> C toCollectionOf(Class dtoClass, Supplier collectionSupplier);
/**
* Assembles to a {@link List} of DTO.
*
* @param the type of the DTO.
* @param dtoClass the DTO class to assemble.
* @return the list of DTO.
*/
List toListOf(Class dtoClass);
/**
* Assembles to a {@link Set} of DTO.
*
* @param the type of the DTO.
* @param dtoClass the DTO class to assemble.
* @return the set of DTO.
*/
Set toSetOf(Class dtoClass);
/**
* Assembles to a {@link Slice} of DTO.
*
* @param the type of the DTO.
* @param dtoClass the DTO class to assemble
* @return the list of DTO.
*/
Slice toSliceOf(Class dtoClass);
/**
* Assembles to an array of DTO.
*
* @param the type of the DTO.
* @param dtoClass the DTO class to assemble.
* @return the array of DTO.
*/
D[] toArrayOf(Class dtoClass);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy