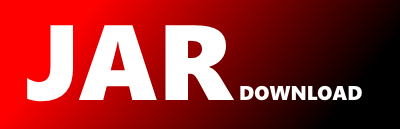
org.seedstack.seed.transaction.utils.TransactionalProxy Maven / Gradle / Ivy
The newest version!
/**
* Copyright (c) 2013-2015 by The SeedStack authors. All rights reserved.
*
* This file is part of SeedStack, An enterprise-oriented full development stack.
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package org.seedstack.seed.transaction.utils;
import org.seedstack.seed.transaction.spi.TransactionalLink;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
/**
* This proxy takes a {@link org.seedstack.seed.transaction.spi.TransactionalLink} . It intercepts method calls and apply them
* to the instance retrieved from {@link org.seedstack.seed.transaction.spi.TransactionalLink}.get().
*
* @author [email protected]
* @param the type of the transactional object.
*/
public final class TransactionalProxy implements InvocationHandler {
private final TransactionalLink transactionalLink;
private TransactionalProxy(TransactionalLink transactionalLink) {
this.transactionalLink = transactionalLink;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
if (method.getDeclaringClass().equals(Object.class)) {
return method.invoke(transactionalLink, args);
}
try {
return method.invoke(transactionalLink.get(), args);
} catch(InvocationTargetException e) { // NOSONAR
throw e.getCause();
}
}
/**
* Create a transactional proxy around the provided {@link TransactionalLink}.
*
* @param clazz the class representing the transacted resource.
* @param transactionalLink the link to access the instance of the transacted resource.
* @param type of the class representing the transacted resource.
* @return the proxy.
*/
@SuppressWarnings("unchecked")
public static T create(Class clazz, TransactionalLink transactionalLink) {
return (T) Proxy.newProxyInstance(TransactionalProxy.class.getClassLoader(), new Class>[]{clazz}, new TransactionalProxy(transactionalLink));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy