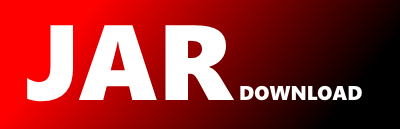
org.seekay.contract.model.tools.SetTools Maven / Gradle / Ivy
The newest version!
package org.seekay.contract.model.tools;
import java.util.*;
public class SetTools {
/**
* Private constructor for utility class
*/
private SetTools() {
throw new IllegalStateException("Utility classes should never be constructed");
}
/**
* For multiple sets, only the elements contained in all will be returned
*
* @param sets
* @param
* @return
*/
public static Set intersectingElements(Set... sets) {
assert sets.length > 1;
Set result = new HashSet();
Set head = head(sets);
List> tail = tail(sets);
for (T t : head) {
if (containedInAll(t, tail)) {
result.add(t);
}
}
return result;
}
/**
* Returns the first element in a set
*
* @param set
* @param
* @return
*/
public static T head(Set set) {
return set.iterator().next();
}
/**
* Returns the first set in a vararg of sets
*
* @param sets
* @param
* @return
*/
public static Set head(Set... sets) {
return Arrays.asList(sets).get(0);
}
/**
* Returns all but the first element of a set
*
* @param set
* @param
* @return
*/
public static Set tail(Set set) {
Set result = new HashSet();
Iterator iterator = set.iterator();
iterator.next();
while (iterator.hasNext()) {
result.add(iterator.next());
}
return result;
}
/**
* Converts a set of strings to an array of strings
*
* @param input
* @return
*/
public static String[] toArray(Set input) {
if (input == null) {
return new String[0];
}
String[] output = new String[input.size()];
int i = 0;
for (String inputString : input) {
output[i] = inputString;
i++;
}
return output;
}
/**
* Converts an array of strings to a set of strings
*
* @param input
* @return
*/
public static Set toSet(String[] input) {
Set output = new LinkedHashSet();
if (input == null) {
return output;
}
for (String inputString : input) {
output.add(inputString);
}
return output;
}
/**
* Converts a csv string to a set of strings
*
* @param csvTags
* @return
*/
public static Set toSet(String csvTags) {
if (csvTags == null) {
return new HashSet();
}
String[] tokens = csvTags.replaceAll(" ", "").split(",");
return toSet(tokens);
}
private static List> tail(Set... sets) {
if (sets.length < 2) {
throw new IllegalStateException("Matching configured incorrectly");
}
List> lists = Arrays.asList(sets);
return lists.subList(1, lists.size());
}
private static boolean containedInAll(T t, List> sets) {
boolean found = true;
for (Set set : sets) {
if (!set.contains(t)) {
found = false;
}
}
return found;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy