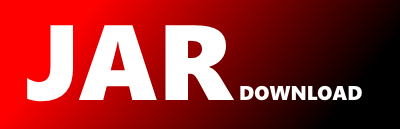
org.sejda.sambox.pdmodel.common.PDStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sambox Show documentation
Show all versions of sambox Show documentation
An Apache PDFBox fork intended to be used as PDF processor for Sejda and PDFsam
related projects
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.sejda.sambox.pdmodel.common;
import org.sejda.commons.util.IOUtils;
import org.sejda.sambox.cos.COSArray;
import org.sejda.sambox.cos.COSArrayList;
import org.sejda.sambox.cos.COSBase;
import org.sejda.sambox.cos.COSDictionary;
import org.sejda.sambox.cos.COSName;
import org.sejda.sambox.cos.COSNull;
import org.sejda.sambox.cos.COSObjectable;
import org.sejda.sambox.cos.COSStream;
import org.sejda.sambox.pdmodel.common.filespecification.FileSpecifications;
import org.sejda.sambox.pdmodel.common.filespecification.PDFileSpecification;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* A PDStream represents a stream in a PDF document. Streams are tied to a single PDF document.
*
* @author Ben Litchfield
*/
public class PDStream implements COSObjectable
{
private COSStream stream;
/**
* This will create a new PDStream object.
*/
public PDStream()
{
stream = new COSStream();
}
/**
* Constructor.
*
* @param str The stream parameter.
*/
public PDStream(COSStream str)
{
stream = str;
}
/**
* Constructor. Reads all data from the input stream and embeds it into the document, this will
* close the InputStream.
*
* @param input The stream parameter.
* @throws IOException If there is an error creating the stream in the document.
*/
public PDStream(InputStream input) throws IOException
{
this(input, (COSBase) null);
}
/**
* Constructor. Reads all data from the input stream and embeds it into the document with the
* given filter applied. This method closes the InputStream.
*
* @param input The stream parameter.
* @param filter Filter to apply to the stream.
* @throws IOException If there is an error creating the stream in the document.
*/
public PDStream(InputStream input, COSName filter) throws IOException
{
this(input, (COSBase) filter);
}
/**
* Constructor. Reads all data from the input stream and embeds it into the document with the
* given filters applied. This method closes the InputStream.
*
* @param input The stream parameter.
* @param filters Filters to apply to the stream.
* @throws IOException If there is an error creating the stream in the document.
*/
public PDStream(InputStream input, COSArray filters) throws IOException
{
this(input, (COSBase) filters);
}
/**
* Constructor. Reads all data from the input stream and embeds it into the document, this will
* close the InputStream.
*
* @param input The stream parameter.
* @param filter Filter to apply to the stream.
* @throws IOException If there is an error creating the stream in the document.
*/
public PDStream(InputStream input, COSBase filter) throws IOException
{
stream = new COSStream();
try (OutputStream output = stream.createFilteredStream(filter))
{
IOUtils.copy(input, output);
}
finally
{
IOUtils.close(input);
}
}
/**
* Convert this standard java object to a COS object.
*
* @return The cos object that matches this Java object.
*/
@Override
public COSStream getCOSObject()
{
return stream;
}
/**
* This will get a stream that can be written to.
*
* @return An output stream to write data to.
* @throws IOException If an IO error occurs during writing.
*/
public OutputStream createOutputStream()
{
return stream.createUnfilteredStream();
}
/**
* This will get a stream that can be written to, with the given filter.
*
* @param filter the filter to be used.
* @return An output stream to write data to.
* @throws IOException If an IO error occurs during writing.
*/
public OutputStream createOutputStream(COSName filter)
{
return stream.createFilteredStream(filter);
}
/**
* This will get a stream that can be read from.
*
* @return An input stream that can be read from.
* @throws IOException If an IO error occurs during reading.
*/
public InputStream createInputStream() throws IOException
{
return stream.getUnfilteredStream();
}
/**
* This will get the length of the filtered/compressed stream. This is readonly in the PD Model
* and will be managed by this class.
*
* @return The length of the filtered stream.
*/
public int getLength()
{
return stream.getInt(COSName.LENGTH, 0);
}
/**
* This will get the list of filters that are associated with this stream. Or null if there are
* none.
*
* @return A list of all encoding filters to apply to this stream.
*/
public List getFilters()
{
COSBase filters = stream.getFilters();
if (filters instanceof COSName name)
{
return new COSArrayList<>(name, name, stream, COSName.FILTER);
}
if (filters instanceof COSArray filtersArray)
{
return (List) filtersArray.toList();
}
return null;
}
/**
* This will set the filters that are part of this stream.
*
* @param filters The filters that are part of this stream.
*/
public void setFilters(List filters)
{
COSBase obj = COSArrayList.converterToCOSArray(filters);
stream.setItem(COSName.FILTER, obj);
}
/**
* Get the list of decode parameters. Each entry in the list will refer to an entry in the
* filters list.
*
* @return The list of decode parameters.
* @throws IOException if there is an error retrieving the parameters.
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy