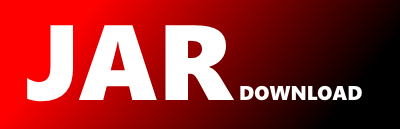
org.openqa.selenium.htmlunit.options.BrowserVersionTrait Maven / Gradle / Ivy
Show all versions of htmlunit3-driver Show documentation
// Licensed to the Software Freedom Conservancy (SFC) under one
// or more contributor license agreements. See the NOTICE file
// distributed with this work for additional information
// regarding copyright ownership. The SFC licenses this file
// to you under the Apache License, Version 2.0 (the
// "License"); you may not use this file except in compliance
// with the License. You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing,
// software distributed under the License is distributed on an
// "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
// KIND, either express or implied. See the License for the
// specific language governing permissions and limitations
// under the License.
package org.openqa.selenium.htmlunit.options;
import java.util.Map;
import java.util.TimeZone;
import org.htmlunit.BrowserVersion;
import org.htmlunit.BrowserVersion.BrowserVersionBuilder;
/**
* @author Scott Babcock
* @author Ronald Brill
*/
public enum BrowserVersionTrait implements BrowserVersionTraitNames, OptionEnum {
/**
* Returns the numeric code for the browser represented by this BrowserVersion.
*
* property: webdriver.htmlunit.numericCode
* type: {@code int}
* default: {@code 0}
*/
NUMERIC_CODE(optNumericCode, int.class, 0) {
@Override
public Object obtain(final BrowserVersion version) {
return version.getBrowserVersionNumeric();
}
},
/**
* Returns the nickname for the browser represented by this BrowserVersion.
*
* property: webdriver.htmlunit.nickname
* type: {@code String}
* default: {@code null}
*/
NICKNAME(optNickname, String.class, null) {
@Override
public Object obtain(final BrowserVersion version) {
return version.getNickname();
}
},
/**
* Returns the application version, for example "4.0 (compatible; MSIE 6.0b; Windows 98)".
*
* property: webdriver.htmlunit.applicationVersion
* type: {@code String}
* default: {@code null}
* see: MSDN documentation
*/
APPLICATION_VERSION(optApplicationVersion, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setApplicationVersion(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getApplicationVersion();
}
},
/**
* Returns the user agent string, for example "Mozilla/4.0 (compatible; MSIE 6.0b; Windows 98)".
*
* property: webdriver.htmlunit.userAgent
* type: {@link String}
* default: {@code null}
*/
USER_AGENT(optUserAgent, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setUserAgent(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getUserAgent();
}
},
/**
* Returns the application name, for example "Microsoft Internet Explorer".
*
* property: webdriver.htmlunit.applicationName
* type: {@code String}
* default: {@code null}
* @see MSDN documentation
*/
APPLICATION_NAME(optApplicationName, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setApplicationName(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getApplicationName();
}
},
/**
* Returns the application code name, for example "Mozilla".
*
* property: webdriver.htmlunit.applicationCodeName
* type: {@code String}
* default: "Mozilla"
* see: MSDN documentation
*/
APPLICATION_CODE_NAME(optApplicationCodeName, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setApplicationCodeName(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getApplicationCodeName();
}
},
/**
* Returns the application minor version, for example "0".
*
* property: webdriver.htmlunit.applicationMinorVersion
* type: {@code String}
* default: "0"
* see: MSDN documentation
*/
APPLICATION_MINOR_VERSION(optApplicationMinorVersion, String.class, "0") {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setApplicationMinorVersion(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getApplicationMinorVersion();
}
},
/**
* Returns the browser vendor, for example "Google Inc.".
*
* property: webdriver.htmlunit.vendor
* type: {@code String}
* default: ""
*/
VENDOR(optVendor, String.class, "") {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setVendor(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getVendor();
}
},
/**
* Returns the browser language, for example "en-US".
*
* property: webdriver.htmlunit.browserLanguage
* type: {@link String}
* default: "en_US"
*/
BROWSER_LANGUAGE(optBrowserLanguage, String.class, "en-US") {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setBrowserLanguage(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getBrowserLanguage();
}
},
/**
* Returns {@code true} if the browser is currently online.
*
* property: webdriver.htmlunit.isOnline
* type: {@code boolean}
* default: {@code true}
* see: MSDN documentation
*/
IS_ONLINE(optIsOnline, boolean.class, true) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setOnLine(TypeCodec.decodeBoolean(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.isOnLine();
}
},
/**
* Returns the platform on which the application is running, for example "Win32".
*
* property: webdriver.htmlunit.platform
* type: {@code String}
* default: "Win32"
* see: MSDN documentation
*/
PLATFORM(optPlatform, String.class, "Win32") {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setPlatform(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getPlatform();
}
},
/**
* Returns the system {@link TimeZone}.
*
* property: webdriver.htmlunit.systemTimezone
* type: {@link TimeZone}
* default: TimeZone.getTimeZone("America/New_York")
*/
SYSTEM_TIMEZONE(optSystemTimezone, TimeZone.class, TimeZone.getTimeZone("America/New_York")) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setSystemTimezone(TypeCodec.decodeTimeZone(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getSystemTimezone();
}
},
/**
* Returns the value used by the browser for the {@code Accept-Encoding} header.
*
* property: webdriver.htmlunit.acceptEncodingHeader
* type: {@link String}
* default: {@code null}
*/
ACCEPT_ENCODING_HEADER(optAcceptEncodingHeader, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setAcceptEncodingHeader(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getAcceptEncodingHeader();
}
},
/**
* Returns the value used by the browser for the {@code Accept-Language} header.
*
* property: webdriver.htmlunit.acceptLanguageHeader
* type: {@link String}
* default: {@code null}
*/
ACCEPT_LANGUAGE_HEADER(optAcceptLanguageHeader, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setAcceptLanguageHeader(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getAcceptLanguageHeader();
}
},
/**
* Returns the value used by the browser for the {@code Accept} header if requesting a page.
*
* property: webdriver.htmlunit.htmlAcceptHeader
* type: {@link String}
* default: {@code null}
*/
HTML_ACCEPT_HEADER(optHtmlAcceptHeader, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setHtmlAcceptHeader(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getHtmlAcceptHeader();
}
},
/**
* Returns the value used by the browser for the {@code Accept} header
* if requesting an image.
*
* property: webdriver.htmlunit.imgAcceptHeader
* type: {@link String}
* default: {@code null}
*/
IMG_ACCEPT_HEADER(optImgAcceptHeader, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setImgAcceptHeader(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getImgAcceptHeader();
}
},
/**
* Returns the value used by the browser for the {@code Accept} header
* if requesting a CSS declaration.
*
* property: webdriver.htmlunit.cssAcceptHeader
* type: {@link String}
* default: {@code null}
*/
CSS_ACCEPT_HEADER(optCssAcceptHeader, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setCssAcceptHeader(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getCssAcceptHeader();
}
},
/**
* Returns the value used by the browser for the {@code Accept} header
* if requesting a script.
*
* property: webdriver.htmlunit.scriptAcceptHeader
* type: {@link String}
* default: {@code null}
*/
SCRIPT_ACCEPT_HEADER(optScriptAcceptHeader, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setScriptAcceptHeader(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getScriptAcceptHeader();
}
},
/**
* Returns the value used by the browser for the {@code Accept} header
* if performing an XMLHttpRequest.
*
* property: webdriver.htmlunit.xmlHttpRequestAcceptHeader
* type: {@link String}
* default: {@code null}
*/
XML_HTTP_REQUEST_ACCEPT_HEADER(optXmlHttpRequestAcceptHeader, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setXmlHttpRequestAcceptHeader(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getXmlHttpRequestAcceptHeader();
}
},
/**
* Returns the value used by the browser for the {@code Sec-CH-UA} header.
*
* property: webdriver.htmlunit.secClientHintUserAgentHeader
* type: {@link String}
* default: {@code null}
*/
SEC_CLIENT_HINT_USER_AGENT_HEADER(optSecClientHintUserAgentHeader, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setSecClientHintUserAgentHeader(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getSecClientHintUserAgentHeader();
}
},
/**
* Returns the value used by the browser for the {@code Sec-CH-UA-Platform} header.
*
* property: webdriver.htmlunit.secClientHintUserAgentPlatformHeader
* type: {@link String}
* default: {@code null}
*/
SEC_CLIENT_HINT_USER_AGENT_PLATFORM_HEADER(optSecClientHintUserAgentPlatformHeader, String.class, null) {
@Override
public void apply(final Object value, final BrowserVersionBuilder builder) {
builder.setSecClientHintUserAgentPlatformHeader(TypeCodec.decodeString(value));
}
@Override
public Object obtain(final BrowserVersion version) {
return version.getSecClientHintUserAgentPlatformHeader();
}
};
private final String capabilityKey_;
private final String propertyName_;
private final Class> optionType_;
private final Object defaultValue_;
BrowserVersionTrait(final String key, final Class> type, final Object initial) {
capabilityKey_ = key;
propertyName_ = "webdriver.htmlunit.browserVersionTrait." + key;
optionType_ = type;
defaultValue_ = initial;
}
@Override
public String getCapabilityKey() {
return capabilityKey_;
}
@Override
public String getPropertyName() {
return propertyName_;
}
@Override
public Class> getOptionType() {
return optionType_;
}
@Override
public Object getDefaultValue() {
return defaultValue_;
}
/**
* Determine if the specified value matches the default for this trait.
*
* @param value value to be evaluated
* @return {@code true} if specified value matches the default value; otherwise {@code false}
*/
@Override
public boolean isDefaultValue(final Object value) {
if (defaultValue_ == null) {
return value == null;
}
if (value == null) {
return false;
}
return value.equals(defaultValue_);
}
/**
* Apply the value of the system property associated with this trait to the specified options map.
*
* @param optionsMap browser version options map
*/
@Override
public void applyPropertyTo(final Map optionsMap) {
final String value = System.getProperty(propertyName_);
if (value != null) {
optionsMap.put(capabilityKey_, decode(value));
System.clearProperty(capabilityKey_);
}
}
/**
* Encode the specified value according to the type of this trait.
*
* @param value value to be encoded
* @return trait-specific encoding for specified value
*/
@Override
public Object encode(final Object value) {
switch (optionType_.getName()) {
case "boolean":
case "int":
case "java.lang.String":
return value;
case "java.util.TimeZone":
return TypeCodec.encodeTimeZone(value);
default:
throw new IllegalStateException(
String.format("Unsupported type '%s' specified for option [%s]; value is of type: %s",
optionType_.getName(), toString(), TypeCodec.getClassName(value)));
}
}
/**
* Decode the specified value according to the type of this trait.
*
* @param value value to be decoded
* @return trait-specific decoding for specified value
*/
@Override
public Object decode(final Object value) {
switch (optionType_.getName()) {
case "boolean":
return TypeCodec.decodeBoolean(value);
case "int":
return TypeCodec.decodeInt(value);
case "java.lang.String":
return TypeCodec.decodeString(value);
case "java.util.TimeZone":
return TypeCodec.decodeTimeZone(value);
default:
throw new IllegalStateException(
String.format("Unsupported type '%s' specified for option [%s]; value is of type: %s",
optionType_.getName(), toString(), TypeCodec.getClassName(value)));
}
}
/**
* Apply the specified value for this trait into the provided browser version builder.
*
* @param value value to be inserted
* @param builder {@link BrowserVersionBuilder} object
*/
public void apply(final Object value, final BrowserVersionBuilder builder) {
throw new UnsupportedOperationException(
String.format("Trait '%s' does not support value insertion", toString()));
}
/**
* Obtain the value for this trait from the specified browser version object.
*
* @param version {@link BrowserVersion} object
* @return value for this trait
*/
public Object obtain(final BrowserVersion version) {
return null;
}
public static BrowserVersionTrait fromCapabilityKey(final String key) {
for (final BrowserVersionTrait trait : BrowserVersionTrait.values()) {
if (trait.capabilityKey_.equals(key)) {
return trait;
}
}
return null;
}
public static BrowserVersionTrait fromPropertyName(final String name) {
for (final BrowserVersionTrait trait : BrowserVersionTrait.values()) {
if (trait.propertyName_.equals(name)) {
return trait;
}
}
return null;
}
}