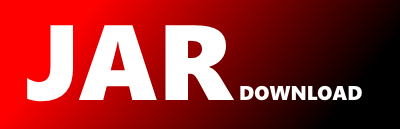
org.openqa.selenium.grid.node.remote.RemoteNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-grid Show documentation
Show all versions of selenium-grid Show documentation
Selenium automates browsers. That's it! What you do with that power is entirely up to you.
The newest version!
// Licensed to the Software Freedom Conservancy (SFC) under one
// or more contributor license agreements. See the NOTICE file
// distributed with this work for additional information
// regarding copyright ownership. The SFC licenses this file
// to you under the Apache License, Version 2.0 (the
// "License"); you may not use this file except in compliance
// with the License. You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing,
// software distributed under the License is distributed on an
// "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
// KIND, either express or implied. See the License for the
// specific language governing permissions and limitations
// under the License.
package org.openqa.selenium.grid.node.remote;
import static java.net.HttpURLConnection.HTTP_OK;
import static org.openqa.selenium.grid.data.Availability.DOWN;
import static org.openqa.selenium.grid.data.Availability.DRAINING;
import static org.openqa.selenium.grid.data.Availability.UP;
import static org.openqa.selenium.net.Urls.fromUri;
import static org.openqa.selenium.remote.http.ClientConfig.defaultConfig;
import static org.openqa.selenium.remote.http.Contents.asJson;
import static org.openqa.selenium.remote.http.Contents.reader;
import static org.openqa.selenium.remote.http.HttpMethod.DELETE;
import static org.openqa.selenium.remote.http.HttpMethod.GET;
import static org.openqa.selenium.remote.http.HttpMethod.POST;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import java.io.Closeable;
import java.io.IOException;
import java.io.Reader;
import java.io.UncheckedIOException;
import java.net.URI;
import java.time.Duration;
import java.util.Collection;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import org.openqa.selenium.Capabilities;
import org.openqa.selenium.NoSuchSessionException;
import org.openqa.selenium.RetrySessionRequestException;
import org.openqa.selenium.SessionNotCreatedException;
import org.openqa.selenium.WebDriverException;
import org.openqa.selenium.grid.data.CreateSessionRequest;
import org.openqa.selenium.grid.data.CreateSessionResponse;
import org.openqa.selenium.grid.data.NodeId;
import org.openqa.selenium.grid.data.NodeStatus;
import org.openqa.selenium.grid.data.Session;
import org.openqa.selenium.grid.node.HealthCheck;
import org.openqa.selenium.grid.node.Node;
import org.openqa.selenium.grid.security.AddSecretFilter;
import org.openqa.selenium.grid.security.Secret;
import org.openqa.selenium.grid.web.Values;
import org.openqa.selenium.internal.Either;
import org.openqa.selenium.internal.Require;
import org.openqa.selenium.json.Json;
import org.openqa.selenium.json.JsonInput;
import org.openqa.selenium.remote.SessionId;
import org.openqa.selenium.remote.http.ClientConfig;
import org.openqa.selenium.remote.http.Filter;
import org.openqa.selenium.remote.http.HttpClient;
import org.openqa.selenium.remote.http.HttpHandler;
import org.openqa.selenium.remote.http.HttpRequest;
import org.openqa.selenium.remote.http.HttpResponse;
import org.openqa.selenium.remote.tracing.HttpTracing;
import org.openqa.selenium.remote.tracing.Tracer;
public class RemoteNode extends Node implements Closeable {
public static final Json JSON = new Json();
private final HttpHandler client;
private final URI externalUri;
private final Set capabilities;
private final HealthCheck healthCheck;
private final Filter addSecret;
public RemoteNode(
Tracer tracer,
HttpClient.Factory clientFactory,
NodeId id,
URI externalUri,
Secret registrationSecret,
Duration sessionTimeout,
Collection capabilities) {
super(tracer, id, externalUri, registrationSecret, sessionTimeout);
this.externalUri = Require.nonNull("External URI", externalUri);
this.capabilities = ImmutableSet.copyOf(capabilities);
ClientConfig clientConfig =
defaultConfig().readTimeout(this.getSessionTimeout()).baseUrl(fromUri(externalUri));
this.client = Require.nonNull("HTTP client factory", clientFactory).createClient(clientConfig);
this.healthCheck = new RemoteCheck();
Require.nonNull("Registration secret", registrationSecret);
this.addSecret = new AddSecretFilter(registrationSecret);
}
@Override
public boolean isReady() {
try {
return client.execute(new HttpRequest(GET, "/readyz")).isSuccessful();
} catch (Exception e) {
return false;
}
}
@Override
public boolean isSupporting(Capabilities capabilities) {
return this.capabilities.stream()
.anyMatch(
caps ->
caps.getCapabilityNames().stream()
.allMatch(
name ->
Objects.equals(
caps.getCapability(name), capabilities.getCapability(name))));
}
@Override
public Either newSession(
CreateSessionRequest sessionRequest) {
Require.nonNull("Capabilities for session", sessionRequest);
HttpRequest req = new HttpRequest(POST, "/se/grid/node/session");
HttpTracing.inject(tracer, tracer.getCurrentContext(), req);
req.setContent(asJson(sessionRequest));
HttpResponse httpResponse = client.with(addSecret).execute(req);
Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy