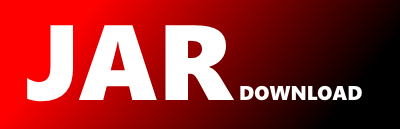
org.openqa.selenium.grid.sessionmap.SessionMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-grid Show documentation
Show all versions of selenium-grid Show documentation
Selenium automates browsers. That's it! What you do with that power is entirely up to you.
The newest version!
// Licensed to the Software Freedom Conservancy (SFC) under one
// or more contributor license agreements. See the NOTICE file
// distributed with this work for additional information
// regarding copyright ownership. The SFC licenses this file
// to you under the Apache License, Version 2.0 (the
// "License"); you may not use this file except in compliance
// with the License. You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing,
// software distributed under the License is distributed on an
// "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
// KIND, either express or implied. See the License for the
// specific language governing permissions and limitations
// under the License.
package org.openqa.selenium.grid.sessionmap;
import static org.openqa.selenium.remote.http.Route.combine;
import static org.openqa.selenium.remote.http.Route.delete;
import static org.openqa.selenium.remote.http.Route.post;
import java.net.URI;
import java.util.Map;
import org.openqa.selenium.NoSuchSessionException;
import org.openqa.selenium.grid.data.Session;
import org.openqa.selenium.internal.Require;
import org.openqa.selenium.json.Json;
import org.openqa.selenium.remote.SessionId;
import org.openqa.selenium.remote.http.HttpRequest;
import org.openqa.selenium.remote.http.HttpResponse;
import org.openqa.selenium.remote.http.Routable;
import org.openqa.selenium.remote.http.Route;
import org.openqa.selenium.remote.tracing.Tracer;
import org.openqa.selenium.status.HasReadyState;
/**
* Provides a stable API for looking up where on the Grid a particular webdriver instance is
* running.
*
* This class responds to the following URLs:
*
*
*
* Verb
* URL Template
* Meaning
*
*
* DELETE
* /se/grid/session/{sessionId}
* Removes a {@link URI} from the session map. Calling this method more than once for the same
* {@link SessionId} will not throw an error.
*
*
* GET
* /se/grid/session/{sessionId}
* Retrieves the {@link URI} associated the {@link SessionId}, or throws a
* {@link org.openqa.selenium.NoSuchSessionException} should the session not be present.
*
*
* POST
* /se/grid/session/{sessionId}
* Registers the session with session map. In theory, the session map never expires a session
* from its mappings, but realistically, sessions may end up being removed for many reasons.
*
*
*
*/
public abstract class SessionMap implements HasReadyState, Routable {
protected final Tracer tracer;
private final Route routes;
public abstract boolean add(Session session);
public abstract Session get(SessionId id) throws NoSuchSessionException;
public abstract void remove(SessionId id);
public URI getUri(SessionId id) throws NoSuchSessionException {
return get(id).getUri();
}
public SessionMap(Tracer tracer) {
this.tracer = Require.nonNull("Tracer", tracer);
Json json = new Json();
routes =
combine(
Route.get("/se/grid/session/{sessionId}/uri")
.to(params -> new GetSessionUri(this, sessionIdFrom(params))),
post("/se/grid/session").to(() -> new AddToSessionMap(tracer, json, this)),
Route.get("/se/grid/session/{sessionId}")
.to(params -> new GetFromSessionMap(tracer, this, sessionIdFrom(params))),
delete("/se/grid/session/{sessionId}")
.to(params -> new RemoveFromSession(tracer, this, sessionIdFrom(params))));
}
private SessionId sessionIdFrom(Map params) {
return new SessionId(params.get("sessionId"));
}
@Override
public boolean matches(HttpRequest req) {
return routes.matches(req);
}
@Override
public HttpResponse execute(HttpRequest req) {
return routes.execute(req);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy