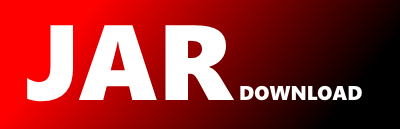
org.openqa.selenium.server.htmlrunner.HTMLTestResults Maven / Gradle / Ivy
// Licensed to the Software Freedom Conservancy (SFC) under one
// or more contributor license agreements. See the NOTICE file
// distributed with this work for additional information
// regarding copyright ownership. The SFC licenses this file
// to you under the Apache License, Version 2.0 (the
// "License"); you may not use this file except in compliance
// with the License. You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing,
// software distributed under the License is distributed on an
// "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
// KIND, either express or implied. See the License for the
// specific language governing permissions and limitations
// under the License.
package org.openqa.selenium.server.htmlrunner;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.io.Writer;
import java.net.URLDecoder;
import java.text.MessageFormat;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
/**
* A data model class for the results of the Selenium HTMLRunner (aka TestRunner, FITRunner)
*
* @author Darren Cotterill
* @author Ajit George
*/
public class HTMLTestResults {
private final String result;
private final String totalTime;
private final String numTestTotal;
private final String numTestPasses;
private final String numTestFailures;
private final String numCommandPasses;
private final String numCommandFailures;
private final String numCommandErrors;
private final String seleniumVersion;
private final String seleniumRevision;
private final String log;
private final HTMLSuiteResult suite;
private static final String HEADER = "\n" +
"Test suite results \n" +
"\nTest suite results
";
private static final String SUMMARY_HTML =
"\n\n\n" +
"\nresult: \n{0} \n \n" +
"\ntotalTime: \n{1} \n \n" +
"\nnumTestTotal: \n{2} \n \n" +
"\nnumTestPasses: \n{3} \n \n" +
"\nnumTestFailures: \n{4} \n \n" +
"\nnumCommandPasses: \n{5} \n \n" +
"\nnumCommandFailures: \n{6} \n \n" +
"\nnumCommandErrors: \n{7} \n \n" +
"\nSelenium Version: \n{8} \n \n" +
"\nSelenium Revision: \n{9} \n \n" +
"\n{10} \n \n \n
";
private static final String SUITE_HTML =
"\n{1}
{2} \n \n ";
private final List testTables;
public HTMLTestResults(String postedSeleniumVersion, String postedSeleniumRevision,
String postedResult, String postedTotalTime,
String postedNumTestTotal, String postedNumTestPasses,
String postedNumTestFailures, String postedNumCommandPasses, String postedNumCommandFailures,
String postedNumCommandErrors, String postedSuite, List postedTestTables,
String postedLog) {
result = postedResult;
numCommandFailures = postedNumCommandFailures;
numCommandErrors = postedNumCommandErrors;
suite = new HTMLSuiteResult(postedSuite);
totalTime = postedTotalTime;
numTestTotal = postedNumTestTotal;
numTestPasses = postedNumTestPasses;
numTestFailures = postedNumTestFailures;
numCommandPasses = postedNumCommandPasses;
testTables = postedTestTables;
seleniumVersion = postedSeleniumVersion;
seleniumRevision = postedSeleniumRevision;
log = postedLog;
}
public String getResult() {
return result;
}
public String getNumCommandErrors() {
return numCommandErrors;
}
public String getNumCommandFailures() {
return numCommandFailures;
}
public String getNumCommandPasses() {
return numCommandPasses;
}
public String getNumTestFailures() {
return numTestFailures;
}
public String getNumTestPasses() {
return numTestPasses;
}
public Collection getTestTables() {
return testTables;
}
public String getTotalTime() {
return totalTime;
}
public int getNumTotalTests() {
return Integer.parseInt(numTestPasses) + Integer.parseInt(numTestFailures);
}
public void write(Writer out) throws IOException {
out.write(HEADER);
out.write(MessageFormat.format(SUMMARY_HTML,
result,
totalTime,
numTestTotal,
numTestPasses,
numTestFailures,
numCommandPasses,
numCommandFailures,
numCommandErrors,
seleniumVersion,
seleniumRevision,
suite.getUpdatedSuite()));
out.write("");
for (int i = 0; i < testTables.size(); i++) {
String table = testTables.get(i).replace("\u00a0", " ");
out.write(MessageFormat.format(SUITE_HTML, i, suite.getHref(i), table));
}
out.write("
\n");
if (log != null) {
out.write(quoteCharacters(log));
}
out.write("
");
out.flush();
}
public static String quoteCharacters(String s) {
StringBuffer result = null;
for (int i = 0, max = s.length(), delta = 0; i < max; i++) {
char c = s.charAt(i);
String replacement = null;
if (c == '&') {
replacement = "&";
} else if (c == '<') {
replacement = "<";
} else if (c == '>') {
replacement = ">";
} else if (c == '"') {
replacement = """;
} else if (c == '\'') {
replacement = "'";
}
if (replacement != null) {
if (result == null) {
result = new StringBuffer(s);
}
result.replace(i + delta, i + delta + 1, replacement);
delta += (replacement.length() - 1);
}
}
if (result == null) {
return s;
}
return result.toString();
}
class UrlDecoder {
public String decode(String string) {
try {
return URLDecoder.decode(string, System.getProperty("file.encoding"));
} catch (UnsupportedEncodingException e) {
return string;
}
}
public List decodeListOfStrings(List list) {
List decodedList = new LinkedList<>();
for (String o : list) {
decodedList.add(decode(o));
}
return decodedList;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy