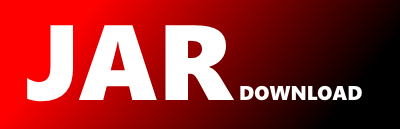
org.openqa.selenium.firefox.FirefoxWebElement Maven / Gradle / Ivy
The newest version!
/*
Copyright 2007-2009 WebDriver committers
Copyright 2007-2009 Google Inc.
Portions copyright 2007 ThoughtWorks, Inc
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package org.openqa.selenium.firefox;
import org.json.JSONException;
import org.json.JSONObject;
import org.json.JSONWriter;
import org.openqa.selenium.By;
import org.openqa.selenium.NoSuchElementException;
import org.openqa.selenium.RenderedWebElement;
import org.openqa.selenium.SearchContext;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.WebDriverException;
import org.openqa.selenium.internal.FindsByClassName;
import org.openqa.selenium.internal.FindsById;
import org.openqa.selenium.internal.FindsByLinkText;
import org.openqa.selenium.internal.FindsByName;
import org.openqa.selenium.internal.FindsByTagName;
import org.openqa.selenium.internal.FindsByXPath;
import org.openqa.selenium.internal.Locatable;
import org.openqa.selenium.internal.WrapsElement;
import java.awt.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.HashMap;
import java.io.PrintWriter;
import java.io.StringWriter;
public class FirefoxWebElement implements RenderedWebElement, Locatable,
FindsByXPath, FindsByLinkText, FindsById, FindsByName, FindsByTagName, FindsByClassName, SearchContext {
private final FirefoxDriver parent;
private final String elementId;
public FirefoxWebElement(FirefoxDriver parent, String elementId) {
this.parent = parent;
this.elementId = elementId;
}
public void click() {
sendMessage(UnsupportedOperationException.class, "click");
}
public void hover() {
sendMessage(WebDriverException.class, "hover");
}
public void submit() {
sendMessage(WebDriverException.class, "submitElement");
}
public String getValue() {
try {
return sendMessage(WebDriverException.class, "getElementValue");
} catch (WebDriverException e) {
return null;
}
}
public void clear() {
sendMessage(UnsupportedOperationException.class, "clear");
}
public void sendKeys(CharSequence... value) {
StringBuilder builder = new StringBuilder();
for (CharSequence seq : value) {
builder.append(seq);
}
sendMessage(UnsupportedOperationException.class, "sendKeys", builder.toString());
}
public String getTagName() {
String name = sendMessage(WebDriverException.class, "getTagName");
return name;
}
/**
* @deprecated Use {@link #getTagName()} instead, this method will be removed in the near future.
*/
public String getElementName() {
return getTagName();
}
public String getAttribute(String name) {
try {
return sendMessage(WebDriverException.class, "getElementAttribute", name);
} catch (WebDriverException e) {
return null;
}
}
public boolean toggle() {
sendMessage(UnsupportedOperationException.class, "toggleElement");
return isSelected();
}
public boolean isSelected() {
String value = sendMessage(WebDriverException.class, "getElementSelected");
return Boolean.parseBoolean(value);
}
public void setSelected() {
sendMessage(UnsupportedOperationException.class, "setElementSelected");
}
public boolean isEnabled() {
String value = getAttribute("disabled");
return !Boolean.parseBoolean(value);
}
public String getText() {
return sendMessage(WebDriverException.class, "getElementText");
}
public boolean isDisplayed() {
return Boolean.parseBoolean(sendMessage(WebDriverException.class, "isElementDisplayed"));
}
public Point getLocation() {
String result = sendMessage(WebDriverException.class, "getElementLocation");
String[] parts = result.split(",");
int x = Integer.parseInt(parts[0].trim());
int y = Integer.parseInt(parts[1].trim());
return new Point(x, y);
}
public Dimension getSize() {
String result = sendMessage(WebDriverException.class, "getElementSize");
String[] parts = result.split(",");
int x = Math.round(Float.parseFloat(parts[0].trim()));
int y = Math.round(Float.parseFloat(parts[1].trim()));
return new Dimension(x, y);
}
public void dragAndDropBy(int moveRight, int moveDown) {
sendMessage(UnsupportedOperationException.class, "dragAndDrop", moveRight, moveDown);
}
public void dragAndDropOn(RenderedWebElement element) {
Point currentLocation = getLocation();
Point destination = element.getLocation();
dragAndDropBy(destination.x - currentLocation.x, destination.y - currentLocation.y);
}
public WebElement findElement(By by) {
return by.findElement(this);
}
public List findElements(By by) {
return by.findElements(this);
}
public WebElement findElementByXPath(String xpath) {
return findChildElement("xpath", xpath);
}
public List findElementsByXPath(String xpath) {
return findChildElements("xpath", xpath);
}
public WebElement findElementByLinkText(String linkText) {
return findChildElement("link text", linkText);
}
public List findElementsByLinkText(String linkText) {
return findChildElements("link text", linkText);
}
public WebElement findElementByPartialLinkText(String text) {
return findChildElement("partial link text", text);
}
public List findElementsByPartialLinkText(String text) {
return findChildElements("partial link text", text);
}
public WebElement findElementById(String id) {
return findChildElement("id", id);
}
public List findElementsById(String id) {
return findChildElements("id", id);
}
public WebElement findElementByName(String name) {
return findChildElement("name", name);
}
public List findElementsByName(String name) {
return findChildElements("name", name);
}
public WebElement findElementByTagName(String tagName) {
return findChildElement("tag name", tagName);
}
public List findElementsByTagName(String tagName) {
return findChildElements("tag name", tagName);
}
public WebElement findElementByClassName(String className) {
return findChildElement("class name", className);
}
public List findElementsByClassName(String className) {
return findChildElements("class name", className);
}
private WebElement findChildElement(String using, String value) {
String id = sendMessage(NoSuchElementException.class,
"findChildElement", buildSearchParamsMap(using, value));
return new FirefoxWebElement(parent, id);
}
private List findChildElements(String using, String value) {
String indices = sendMessage(WebDriverException.class,
"findChildElements", buildSearchParamsMap(using, value));
List elements = new ArrayList();
if (indices.length() == 0) {
return elements;
}
String[] ids = indices.split(",");
for (String id : ids) {
elements.add(new FirefoxWebElement(parent, id));
}
return elements;
}
private Map buildSearchParamsMap(String using, String value) {
Map map = new HashMap();
map.put("id", elementId);
map.put("using", using);
map.put("value", value);
return map;
}
public String getValueOfCssProperty(String propertyName) {
return sendMessage(WebDriverException.class,"getElementCssProperty", propertyName);
}
private String sendMessage(Class extends RuntimeException> throwOnFailure, String methodName, Object... parameters) {
return parent.sendMessage(throwOnFailure, new Command(parent.context, elementId, methodName, parameters));
}
public String getElementId() {
return elementId;
}
public Point getLocationOnScreenOnceScrolledIntoView() {
String json = sendMessage(WebDriverException.class, "getLocationOnceScrolledIntoView");
if (json == null) {
return null;
}
try {
JSONObject mapped = new JSONObject(json);
return new Point(mapped.getInt("x"), mapped.getInt("y"));
} catch (JSONException e) {
throw new RuntimeException(e);
}
}
@Override
public boolean equals(Object obj) {
if (!(obj instanceof WebElement)) {
return false;
}
WebElement other = (WebElement) obj;
if (other instanceof WrapsElement) {
other = ((WrapsElement) obj).getWrappedElement();
}
if (!(other instanceof FirefoxWebElement)) {
return false;
}
Object result = parent.executeScript("return arguments[0] === arguments[1]", this, other);
return result != null && result instanceof Boolean && (Boolean) result;
}
@Override
public int hashCode() {
// Distinctly sub-optimal
return elementId.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy