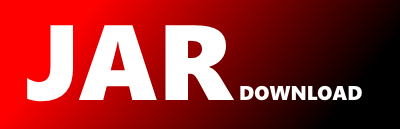
org.semantictools.uml.model.UmlClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of semantictools-context-renderer Show documentation
Show all versions of semantictools-context-renderer Show documentation
A library used to generate documentation for media types associated with a JSON-LD context
The newest version!
/*******************************************************************************
* Copyright 2012 Pearson Education
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package org.semantictools.uml.model;
import java.util.ArrayList;
import java.util.List;
import org.semantictools.frame.model.Datatype;
import org.semantictools.frame.model.Field;
import org.semantictools.frame.model.Frame;
import org.semantictools.frame.model.NamedIndividual;
import org.semantictools.frame.model.RdfType;
import com.hp.hpl.jena.vocabulary.RDF;
import com.hp.hpl.jena.vocabulary.RDFS;
public class UmlClass {
private RdfType type;
private String stereotype;
private UmlManager manager;
private List fieldList = new ArrayList();
private List supertypeList = null;
private List subtypeList = null;
private List children = new ArrayList();
private List parentList = new ArrayList();
public UmlClass(RdfType type, UmlManager manager) {
this.type = type;
this.manager = manager;
}
public String getDescription() {
String comment =
type.canAsFrame() ? type.asFrame().getComment() :
null;
return comment;
}
public List listInstances(boolean direct) {
return type.asFrame().listInstances(direct);
}
public void addSupertype(UmlClass type) {
List list = getSupertypeList();
for (UmlClass other : list) {
if (other.getURI().equals(type.getURI())) {
return;
}
}
list.add(type);
}
public void addSubtype(UmlClass type) {
List list = getSubtypeList();
for (UmlClass other : list) {
if (other.getURI().equals(type.getURI())) {
return;
}
}
list.add(type);
}
public void add(Field field) {
fieldList.add(field);
}
public String getURI() {
return type.getUri();
}
public String getLocalName() {
return type.getLocalName();
}
public RdfType getType() {
return type;
}
public String getStereotype() {
return stereotype;
}
public void setStereotype(String stereotype) {
this.stereotype = stereotype;
}
public List getFieldList() {
return fieldList;
}
public List listAllSupertypes() {
List list = new ArrayList();
if (type.canAsFrame()) {
List frameList = type.asFrame().listAllSupertypes();
for (Frame frame : frameList) {
String uri = frame.getUri();
if (uri.startsWith(RDF.getURI())) continue;
if (uri.startsWith(RDFS.getURI())) continue;
UmlClass superClass = manager.getUmlClassByURI(frame.getUri());
if (superClass == null) {
superClass = new UmlClass(frame, manager);
}
list.add(superClass);
}
}
return list;
}
public List getSupertypeList() {
if (supertypeList == null) {
supertypeList = new ArrayList();
if (type.canAsFrame()) {
List list = type.asFrame().getSupertypeList();
for (Frame frame : list) {
String uri = frame.getUri();
if (uri.startsWith(RDF.getURI())) continue;
if (uri.startsWith(RDFS.getURI())) continue;
UmlClass superClass = manager.getUmlClassByURI(frame.getUri());
if (superClass == null) {
superClass = new UmlClass(frame, manager);
}
supertypeList.add(superClass);
}
}
}
return supertypeList;
}
public List getSubtypeList() {
if (subtypeList == null) {
subtypeList = new ArrayList();
if (type.canAsFrame()) {
List list = type.asFrame().getSubtypeList();
for (Frame frame : list) {
String uri = frame.getUri();
if (uri.startsWith(RDF.getURI())) continue;
if (uri.startsWith(RDFS.getURI())) continue;
UmlClass subclass = manager.getUmlClassByURI(frame.getUri());
if (subclass == null) {
throw new RuntimeException("UmlClass not found: " + frame.getUri());
}
subtypeList.add(subclass);
}
List datatypeList = type.asFrame().getSubdatatypeList();
for (Datatype type : datatypeList) {
String uri = type.getUri();
UmlClass subclass = manager.getUmlClassByURI(uri);
if (subclass == null) {
subclass = new UmlClass(type, manager);
manager.add(subclass);
}
subtypeList.add(subclass);
}
}
}
return subtypeList;
}
public void setSubtypeList(List subtypeList) {
this.subtypeList = subtypeList;
}
/**
* Associations where this UmlClass is the subject.
*/
public List getChildren() {
return children;
}
public void addChild(UmlAssociation child) {
addUnique(children, child);
}
public void addParent(UmlAssociation parent) {
addUnique(parentList, parent);
}
private void addUnique(List list, UmlAssociation element) {
for (UmlAssociation a : list) {
if (a.equals(element)) return;
}
list.add(element);
}
/**
* Associations where this UmlClass is the object.
*/
public List getParentList() {
return parentList;
}
//
// /**
// * Returns the child association (where this UmlClass is the subject)
// * for the specified property, or null if no such association is found.
// * @param propertyURI
// * @return
// */
// public UmlAssociation getChildByURI(String propertyURI) {
//
// for (UmlAssociation assoc : children) {
//
// UmlAssociationEnd end = assoc.getOtherEnd(this);
// if (end.getPropertyURI().equals(propertyURI)) return assoc;
//
// }
//
// return null;
// }
public List listAllDeclaredProperties() {
List list = new ArrayList();
for (Field field : fieldList) {
list.add(createUmlProperty(field));
}
for (UmlAssociation a : children) {
UmlAssociationEnd end = a.getOtherEnd(this);
Field field = end.getField();
if (field == null) continue;
list.add(createUmlProperty(field));
}
return list;
}
private UmlProperty createUmlProperty(Field field) {
UmlProperty p = new UmlProperty();
p.setLocalName(field.getLocalName());
p.setDescription(field.getComment());
p.setMultiplicity(field.getMultiplicity());
p.setType(field.getType().getLocalName());
p.setField(field);
return p;
}
public String toString() {
return type.getLocalName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy